Key points
- A vector drawable is a vector graphic defined in an XML file as a set of points, lines, and curves along with its associated color information.
- Vector drawables are scalable, meaning they can be resized without loss of display quality. This makes them ideal for use in Android apps, as it can help to reduce the size of your APK files and improve performance.
- You can create vector drawables in Android Studio by right-clicking on the drawable folder in your project and selecting New > Vector Asset. You can also import SVG files into Android Studio as vector drawables.
Introduction
AVectorDrawable
is a vector graphic defined
in an XML file as a set of points, lines, and curves along with its associated
color information. The major advantage of using a vector drawable is image
scalability. It can be scaled without loss of display quality, which means the
same file is resized for different screen densities without loss of image quality.
This results in smaller APK files and less developer maintenance. You can also
use vector images for animation by using multiple XML files instead of multiple
images for each display resolution.
This page and the video below provide an overview of how to create vector drawables in XML. Android Studio can also convert SVG files to the vector drawable format, as described in using Add multi-density vector graphics.
Android 5.0 (API level 21) was the first version to officially support vector drawables with
VectorDrawable
and AnimatedVectorDrawable
, but
you can support older versions with the Android support library, which provides the
VectorDrawableCompat
and
AnimatedVectorDrawableCompat
classes.
About VectorDrawable class
VectorDrawable
defines a static drawable
object. Similar to the SVG format, each vector graphic is defined as a tree
hierarchy, which is made up of path
and group
objects.
Each path
contains the geometry of the object's outline and
group
contains details for transformation. All paths are drawn
in the same order as they appear in the XML file.
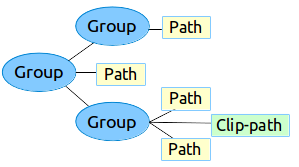
Figure 1. Sample hierarchy of a vector drawable asset
The Vector asset studio tool offers a simple way to add a vector graphic to the project as an XML file.
Example XML
Here is a sample VectorDrawable
XML file that renders an image
of a battery in the charging mode.
<!-- res/drawable/battery_charging.xml --> <vector xmlns:android="http://schemas.android.com/apk/res/android" android:height="24dp" android:width="24dp" android:viewportWidth="24.0" android:viewportHeight="24.0"> <group android:name="rotationGroup" android:pivotX="10.0" android:pivotY="10.0" android:rotation="15.0" > <path android:name="vect" android:fillColor="#FF000000" android:pathData="M15.67,4H14V2h-4v2H8.33C7.6,4 7,4.6 7,5.33V9h4.93L13,7v2h4V5.33C17,4.6 16.4,4 15.67,4z" android:fillAlpha=".3"/> <path android:name="draw" android:fillColor="#FF000000" android:pathData="M13,12.5h2L11,20v-5.5H9L11.93,9H7v11.67C7,21.4 7.6,22 8.33,22h7.33c0.74,0 1.34,-0.6 1.34,-1.33V9h-4v3.5z"/> </group> </vector>
This XML renders the following image:

About AnimatedVectorDrawable class
AnimatedVectorDrawable
adds animation to the properties of a vector
graphic. You can define an animated vector graphic as three separate
resource files or as a single XML file defining the entire drawable. Let's
look at both the approaches for better understanding: Multiple XML files and Single
XML file.
Multiple XML files
By using this approach, you can define three separate XML files:
- A
VectorDrawable
XML file. -
An
AnimatedVectorDrawable
XML file that defines the targetVectorDrawable
, the target paths and groups to animate, the properties, and the animations defined asObjectAnimator
objects orAnimatorSet
objects. - An animator XML file.
Example of multiple XML files
The following XML files demonstrate the animation of a vector graphic.
- VectorDrawable's XML file:
vd.xml
-
<vector xmlns:android="http://schemas.android.com/apk/res/android" android:height="64dp" android:width="64dp" android:viewportHeight="600" android:viewportWidth="600" > <group android:name="rotationGroup" android:pivotX="300.0" android:pivotY="300.0" android:rotation="45.0" > <path android:name="vectorPath" android:fillColor="#000000" android:pathData="M300,70 l 0,-70 70,70 0,0 -70,70z" /> </group> </vector>
- AnimatedVectorDrawable's XML file:
avd.xml
-
<animated-vector xmlns:android="http://schemas.android.com/apk/res/android" android:drawable="@drawable/vd" > <target android:name="rotationGroup" android:animation="@anim/rotation" /> <target android:name="vectorPath" android:animation="@anim/path_morph" /> </animated-vector>
- Animator XML files that are used in the AnimatedVectorDrawable's XML
file:
rotation.xml
andpath_morph.xml
-
<objectAnimator android:duration="6000" android:propertyName="rotation" android:valueFrom="0" android:valueTo="360" />
<set xmlns:android="http://schemas.android.com/apk/res/android"> <objectAnimator android:duration="3000" android:propertyName="pathData" android:valueFrom="M300,70 l 0,-70 70,70 0,0 -70,70z" android:valueTo="M300,70 l 0,-70 70,0 0,140 -70,0 z" android:valueType="pathType"/> </set>
Single XML file
By using this approach, you can merge the related XML files into a single
XML file through the XML Bundle Format. At the time of building the app, the
aapt
tag creates separate resources and references them in the
animated vector. This approach requires Build Tools 24 or higher, and the
output is backward compatible.
Example of a single XML file
<animated-vector xmlns:android="http://schemas.android.com/apk/res/android" xmlns:aapt="http://schemas.android.com/aapt"> <aapt:attr name="android:drawable"> <vector android:width="24dp" android:height="24dp" android:viewportWidth="24" android:viewportHeight="24"> <path android:name="root" android:strokeWidth="2" android:strokeLineCap="square" android:strokeColor="?android:colorControlNormal" android:pathData="M4.8,13.4 L9,17.6 M10.4,16.2 L19.6,7" /> </vector> </aapt:attr> <target android:name="root"> <aapt:attr name="android:animation"> <objectAnimator android:propertyName="pathData" android:valueFrom="M4.8,13.4 L9,17.6 M10.4,16.2 L19.6,7" android:valueTo="M6.4,6.4 L17.6,17.6 M6.4,17.6 L17.6,6.4" android:duration="300" android:interpolator="@android:interpolator/fast_out_slow_in" android:valueType="pathType" /> </aapt:attr> </target> </animated-vector>
Vector drawables backward compatibility solution
To support vector drawable and animated vector drawable on devices running platform versions lower
than Android 5.0 (API level 21), or use fillColor
, fillType
and
strokeColor
functionalities below Android 7.0 (API level 24),
VectorDrawableCompat
and AnimatedVectorDrawableCompat
are available through two support libraries:
support-vector-drawable
and animated-vector-drawable
,
respectively.
Android Studio 1.4 introduced limited compatibility support for vector
drawables by generating PNG files at build time. However, the vector drawable
and animated vector drawable support Libraries offer both flexibility and
broad compatibility — it's a support library, so you can use it with all
Android platform versions back to Android 2.1 (API level 7+). To configure your
app to use vector support libraries, add the vectorDrawables
element to your build.gradle
file in the app module.
Use the following code snippet to configure the vectorDrawables
element:
Groovy
// For Gradle Plugin 2.0+ android { defaultConfig { vectorDrawables.useSupportLibrary = true } }
Kotlin
// For Gradle Plugin 2.0+ android { defaultConfig { vectorDrawables.useSupportLibrary = true } }
Groovy
// For Gradle Plugin 1.5 or below android { defaultConfig { // Stops the Gradle plugin’s automatic rasterization of vectors generatedDensities = [] } // Flag notifies aapt to keep the attribute IDs around aaptOptions { additionalParameters "--no-version-vectors" } }
Kotlin
// For Gradle Plugin 1.5 or below android { defaultConfig { // Stops the Gradle plugin’s automatic rasterization of vectors generatedDensities() } // Flag notifies aapt to keep the attribute IDs around aaptOptions { additionalParameters("--no-version-vectors") } }
You can use VectorDrawableCompat
and
AnimatedVectorDrawableCompat
on all
on devices running Android 4.0 (API level 14) and higher. The way Android
loads drawables, not every place that accepts a drawable ID, such as in an XML
file, supports loading vector drawables. The
android.support.v7.appcompat
package has added a number
of features to make it easy to use vector drawables. Firstly, when you use
android.support.v7.appcompat
package with
ImageView
or with subclasses such as
ImageButton
and
FloatingActionButton
, you can
use the new app:srcCompat
attribute to reference vector drawables
as well as any other drawable available to android:src
:
<ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" app:srcCompat="@drawable/ic_add" />
To change drawables at runtime, you can use the
setImageResource()
method as before. Using AppCompat
and app:srcCompat
is the most foolproof method of integrating
vector drawables into your app.
Support Library 25.4.0 and higher supports the following features:
- Path Morphing (PathType evaluator) Used to morph one path into another path.
- Path Interpolation Used to define a flexible interpolator (represented as a path) instead of the system-defined interpolators like LinearInterpolator.
Support Library 26.0.0-beta1 and higher supports the following features:
- Move along path The geometry object can move around, along an arbitrary path, as part of an animation.
Example of multiple XML files using the support library
The following XML files demonstrate the approach of using multiple XML files to animate a vector graphic.
- VectorDrawable's XML file:
vd.xml
-
<vector xmlns:android="http://schemas.android.com/apk/res/android" android:height="64dp" android:width="64dp" android:viewportHeight="600" android:viewportWidth="600" > <group android:name="rotationGroup" android:pivotX="300.0" android:pivotY="300.0" android:rotation="45.0" > <path android:name="vectorPath" android:fillColor="#000000" android:pathData="M300,70 l 0,-70 70,70 0,0 -70,70z" /> </group> </vector>
- AnimatedVectorDrawable's XML file:
avd.xml
-
<animated-vector xmlns:android="http://schemas.android.com/apk/res/android" android:drawable="@drawable/vd" > <target android:name="rotationGroup" android:animation="@anim/rotation" /> </animated-vector>
- Animator XML file that is used in the AnimatedVectorDrawable's XML
file:
rotation.xml
-
<objectAnimator android:duration="6000" android:propertyName="rotation" android:valueFrom="0" android:valueTo="360" />
Single XML file
The following XML file demonstrates the approach of using a single XML file
to animate a vector graphic. At the time of building the app, the
aapt
tag creates separate resources and references them in the
animated vector. This approach requires Build Tools 24 or higher, and the
output is backward compatible.
Example of a single XML file using the support library
<animated-vector xmlns:android="http://schemas.android.com/apk/res/android" xmlns:aapt="http://schemas.android.com/aapt"> <aapt:attr name="android:drawable"> <vector xmlns:android="http://schemas.android.com/apk/res/android" android:width="64dp" android:height="64dp" android:viewportWidth="600" android:viewportHeight="600"> <group android:name="rotationGroup" android:pivotX="300" android:pivotY="300" android:rotation="45.0" > <path android:name="vectorPath" android:fillColor="#000000" android:pathData="M300,70 l 0,-70 70,70 0,0 -70,70z" /> </group> </vector> </aapt:attr> <target android:name="rotationGroup"> <aapt:attr name="android:animation"> <objectAnimator android:propertyName="rotation" android:valueFrom="0" android:valueTo="360" android:duration="6000" android:interpolator="@android:interpolator/fast_out_slow_in" /> </aapt:attr> </target> </animated-vector>