CameraX는 기기 제조업체가 다양한 Android 기기에 구현한 확장 프로그램에 액세스하기 위한 Extensions API를 제공합니다. 지원되는 확장 프로그램 모드 목록은 카메라 확장 프로그램을 참고하세요.
확장 프로그램을 지원하는 기기 목록은 지원되는 기기를 참고하세요.
확장 프로그램 아키텍처
다음 이미지는 카메라 확장 프로그램 아키텍처를 보여줍니다.
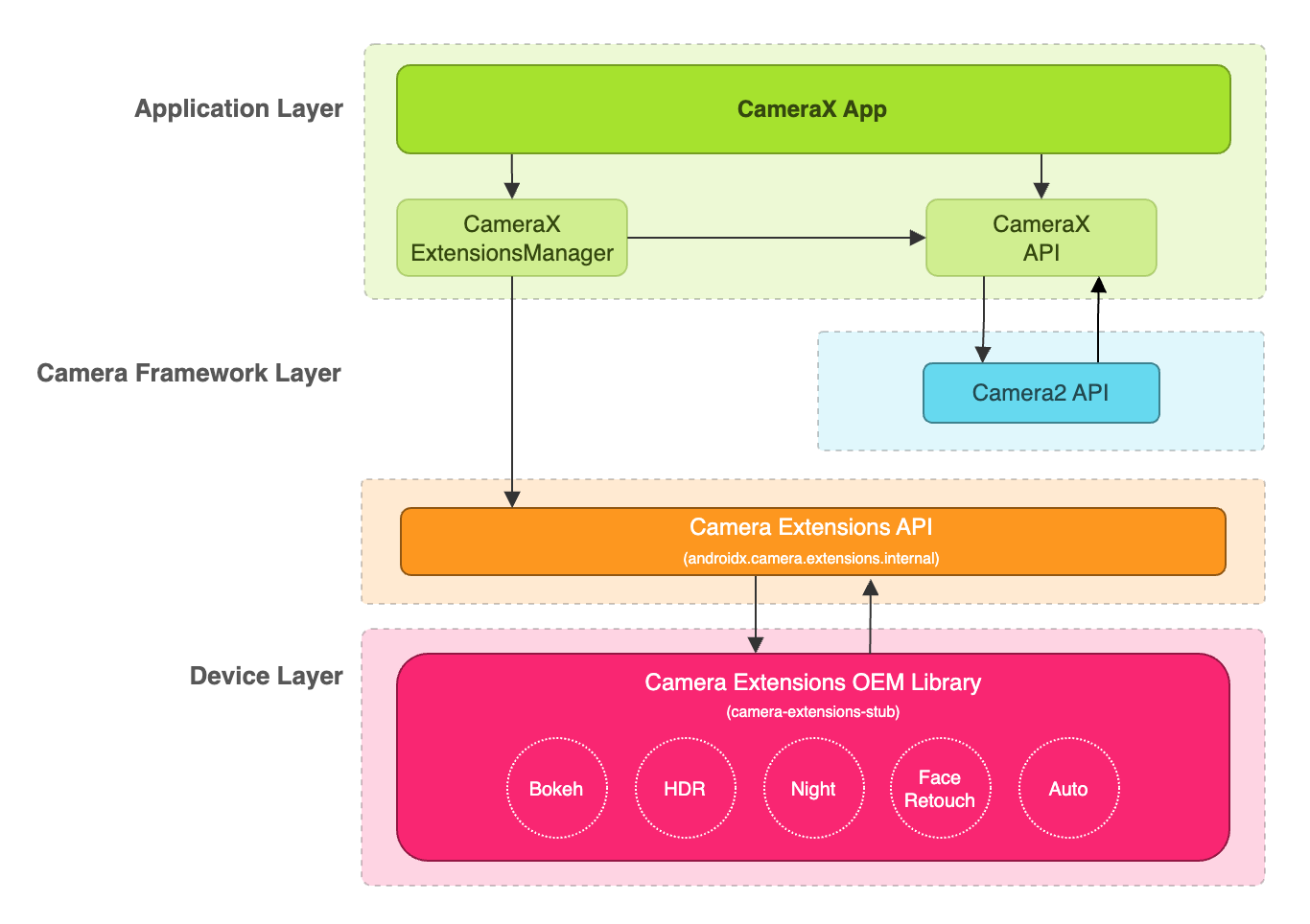
CameraX 애플리케이션은 CameraX Extensions API를 통해 확장 프로그램을 사용할 수 있습니다. CameraX Extensions API는 사용 가능한 확장 프로그램을 쿼리하고 확장 프로그램 카메라 세션을 구성하며 Camera Extensions OEM 라이브러리와 통신합니다. 이를 통해 애플리케이션이 야간, HDR, 자동, 빛망울 효과, 얼굴 보정과 같은 기능을 사용할 수 있습니다.
이미지 캡처 및 미리보기 확장 프로그램 사용
Extensions API를 사용하기 전에 ExtensionsManager#getInstanceAsync(Context, CameraProvider) 메서드를 사용하여 ExtensionsManager
인스턴스를 검색하세요. 그러면 확장 프로그램 가용성 정보를 검색할 수 있습니다. 그런 다음 확장 프로그램 지원 CameraSelector
를 검색합니다. 확장 프로그램 모드는 CameraSelector
확장 프로그램이 사용 설정된 상태로 bindToLifecycle() 메서드를 호출할 때 이미지 캡처 및 미리보기 사용 사례에 적용됩니다.
이미지 캡처 및 미리보기 사용 사례의 확장 프로그램을 구현하려면 다음 코드 샘플을 참고하세요.
Kotlin
import androidx.camera.extensions.ExtensionMode import androidx.camera.extensions.ExtensionsManager override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val lifecycleOwner = this val cameraProviderFuture = ProcessCameraProvider.getInstance(applicationContext) cameraProviderFuture.addListener({ // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the device. // The camera obtained from the provider will be bound to the activity lifecycle. val cameraProvider = cameraProviderFuture.get() val extensionsManagerFuture = ExtensionsManager.getInstanceAsync(applicationContext, cameraProvider) extensionsManagerFuture.addListener({ // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available on // the device. val extensionsManager = extensionsManagerFuture.get() // Select the camera val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable(cameraSelector, ExtensionMode.NIGHT)) { // Unbind all use cases before enabling different extension modes. try { cameraProvider.unbindAll() // Retrieve a night extension enabled camera selector val nightCameraSelector = extensionsManager.getExtensionEnabledCameraSelector( cameraSelector, ExtensionMode.NIGHT ) // Bind image capture and preview use cases with the extension enabled camera // selector. val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider) // Returns an instance of the camera bound to the lifecycle // Use this camera object to control various operations with the camera // Example: flash, zoom, focus metering etc. val camera = cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ) } catch (e: Exception) { Log.e(TAG, "Use case binding failed", e) } } }, ContextCompat.getMainExecutor(this)) }, ContextCompat.getMainExecutor(this)) }
자바
import androidx.camera.extensions.ExtensionMode; import androidx.camera.extensions.ExtensionsManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); final LifecycleOwner lifecycleOwner = this; final ListenableFuturecameraProviderFuture = ProcessCameraProvider.getInstance(getApplicationContext()); cameraProviderFuture.addListener(() -> { try { // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the // device. The camera obtained from the provider will be bound to the activity // lifecycle. final ProcessCameraProvider cameraProvider = cameraProviderFuture.get(); final ListenableFuture extensionsManagerFuture = ExtensionsManager.getInstanceAsync(getApplicationContext(), cameraProvider); extensionsManagerFuture.addListener(() -> { // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available // on the device. try { final ExtensionsManager extensionsManager = extensionsManagerFuture.get(); // Select the camera final CameraSelector cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA; // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable( cameraSelector, ExtensionMode.NIGHT )) { // Unbind all use cases before enabling different extension modes. cameraProvider.unbindAll(); // Retrieve extension enabled camera selector final CameraSelector nightCameraSelector = extensionsManager .getExtensionEnabledCameraSelector(cameraSelector, ExtensionMode.NIGHT); // Bind image capture and preview use cases with the extension enabled camera // selector. final ImageCapture imageCapture = new ImageCapture.Builder().build(); final Preview preview = new Preview.Builder().build(); // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider); cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ); } } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); }
확장 프로그램 사용 중지
공급업체 확장 프로그램을 사용 중지하려면 모든 사용 사례의 바인딩을 해제하고 이미지 캡처 및 미리보기 사용 사례를 일반 카메라 선택기로 다시 바인딩합니다. 예를 들어 CameraSelector.DEFAULT_BACK_CAMERA
를 사용하여 후면 카메라에 다시 바인딩합니다.
종속 항목
CameraX Extensions API는 camera-extensions
라이브러리에 구현됩니다.
확장 프로그램은 CameraX 핵심 모듈(core
, camera2
, lifecycle
)에 따라 다릅니다.
Groovy
dependencies { def camerax_version = "1.2.0-rc01" implementation "androidx.camera:camera-core:${camerax_version}" implementation "androidx.camera:camera-camera2:${camerax_version}" implementation "androidx.camera:camera-lifecycle:${camerax_version}" //the CameraX Extensions library implementation "androidx.camera:camera-extensions:${camerax_version}" ... }
Kotlin
dependencies { val camerax_version = "1.2.0-rc01" implementation("androidx.camera:camera-core:${camerax_version}") implementation("androidx.camera:camera-camera2:${camerax_version}") implementation("androidx.camera:camera-lifecycle:${camerax_version}") // the CameraX Extensions library implementation("androidx.camera:camera-extensions:${camerax_version}") ... }
기존 API 삭제
1.0.0-alpha26
이라는 새 Extensions API가 출시되었으므로 2019년 8월에 출시된 기존 Extensions API는 이제 지원 중단됩니다. 1.0.0-alpha28
버전부터 기존 Extensions API가 라이브러리에서 삭제되었습니다. 새로운 Extensions API를 사용하는 애플리케이션은 이제 확장 프로그램이 사용 설정된 CameraSelector
를 획득하고 이를 사용하여 사용 사례를 결합해야 합니다.
기존 Extensions API를 사용하는 애플리케이션은 향후 출시될 CameraX와의 호환성을 보장하기 위해 새로운 Extensions API로 이전해야 합니다.
추가 리소스
CameraX에 관해 자세히 알아보려면 다음 추가 리소스를 참고하세요.
Codelab
코드 샘플
기타 참고 문서
CameraX 공급업체 확장 프로그램 유효성 검사 도구