CameraX menyediakan Extensions API untuk mengakses ekstensi yang telah diterapkan oleh produsen perangkat di berbagai perangkat Android. Untuk daftar mode ekstensi yang didukung, lihat Ekstensi kamera.
Untuk mengetahui daftar perangkat yang mendukung ekstensi, lihat Perangkat yang didukung.
Arsitektur ekstensi
Gambar berikut menampilkan arsitektur ekstensi kamera.
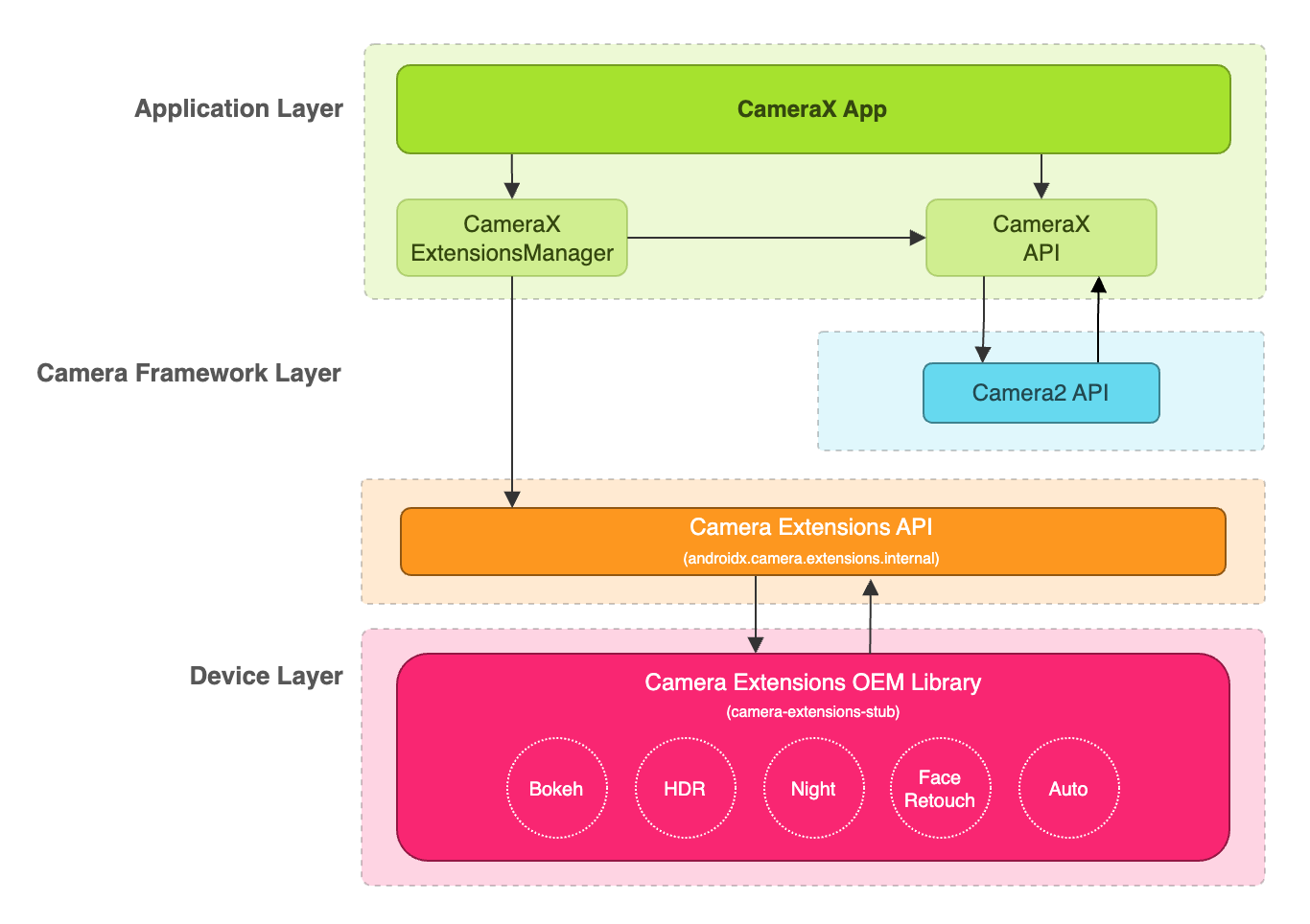
Aplikasi CameraX dapat menggunakan ekstensi melalui CameraX Extensions API. CameraX Extensions API mengelola pembuatan kueri untuk ekstensi yang tersedia, mengonfigurasi sesi kamera ekstensi, dan berkomunikasi dengan library OEM Camera Extensions. Hal ini memungkinkan aplikasi Anda menggunakan kemampuan seperti Mode Malam, HDR, Otomatis, Bokeh, atau Retouch Wajah.
Mengaktifkan ekstensi untuk pratinjau dan pengambilan gambar
Sebelum menggunakan API ekstensi, ambil instance ExtensionsManager
menggunakan metode
ExtensionsManager#getInstanceAsync(Context, CameraProvider). Ini akan memungkinkan Anda mengkueri informasi ketersediaan
ekstensi. Lalu, ambil CameraSelector
yang mengaktifkan ekstensi. Mode
ekstensi akan diterapkan pada kasus penggunaan pratinjau dan pengambilan gambar saat
memanggil metode bindToLifecycle()
dengan ekstensi CameraSelector
yang diaktifkan.
Untuk mengimplementasikan ekstensi pada kasus penggunaan pratinjau dan pengambilan gambar, lihat contoh kode berikut:
Kotlin
import androidx.camera.extensions.ExtensionMode import androidx.camera.extensions.ExtensionsManager override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) val lifecycleOwner = this val cameraProviderFuture = ProcessCameraProvider.getInstance(applicationContext) cameraProviderFuture.addListener({ // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the device. // The camera obtained from the provider will be bound to the activity lifecycle. val cameraProvider = cameraProviderFuture.get() val extensionsManagerFuture = ExtensionsManager.getInstanceAsync(applicationContext, cameraProvider) extensionsManagerFuture.addListener({ // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available on // the device. val extensionsManager = extensionsManagerFuture.get() // Select the camera val cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable(cameraSelector, ExtensionMode.NIGHT)) { // Unbind all use cases before enabling different extension modes. try { cameraProvider.unbindAll() // Retrieve a night extension enabled camera selector val nightCameraSelector = extensionsManager.getExtensionEnabledCameraSelector( cameraSelector, ExtensionMode.NIGHT ) // Bind image capture and preview use cases with the extension enabled camera // selector. val imageCapture = ImageCapture.Builder().build() val preview = Preview.Builder().build() // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider) // Returns an instance of the camera bound to the lifecycle // Use this camera object to control various operations with the camera // Example: flash, zoom, focus metering etc. val camera = cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ) } catch (e: Exception) { Log.e(TAG, "Use case binding failed", e) } } }, ContextCompat.getMainExecutor(this)) }, ContextCompat.getMainExecutor(this)) }
Java
import androidx.camera.extensions.ExtensionMode; import androidx.camera.extensions.ExtensionsManager; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); final LifecycleOwner lifecycleOwner = this; final ListenableFuturecameraProviderFuture = ProcessCameraProvider.getInstance(getApplicationContext()); cameraProviderFuture.addListener(() -> { try { // Obtain an instance of a process camera provider // The camera provider provides access to the set of cameras associated with the // device. The camera obtained from the provider will be bound to the activity // lifecycle. final ProcessCameraProvider cameraProvider = cameraProviderFuture.get(); final ListenableFuture extensionsManagerFuture = ExtensionsManager.getInstanceAsync(getApplicationContext(), cameraProvider); extensionsManagerFuture.addListener(() -> { // Obtain an instance of the extensions manager // The extensions manager enables a camera to use extension capabilities available // on the device. try { final ExtensionsManager extensionsManager = extensionsManagerFuture.get(); // Select the camera final CameraSelector cameraSelector = CameraSelector.DEFAULT_BACK_CAMERA; // Query if extension is available. // Not all devices will support extensions or might only support a subset of // extensions. if (extensionsManager.isExtensionAvailable( cameraSelector, ExtensionMode.NIGHT )) { // Unbind all use cases before enabling different extension modes. cameraProvider.unbindAll(); // Retrieve extension enabled camera selector final CameraSelector nightCameraSelector = extensionsManager .getExtensionEnabledCameraSelector(cameraSelector, ExtensionMode.NIGHT); // Bind image capture and preview use cases with the extension enabled camera // selector. final ImageCapture imageCapture = new ImageCapture.Builder().build(); final Preview preview = new Preview.Builder().build(); // Connect the preview to receive the surface the camera outputs the frames // to. This will allow displaying the camera frames in either a TextureView // or SurfaceView. The SurfaceProvider can be obtained from the PreviewView. preview.setSurfaceProvider(surfaceProvider); cameraProvider.bindToLifecycle( lifecycleOwner, nightCameraSelector, imageCapture, preview ); } } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); } catch (ExecutionException | InterruptedException e) { throw new RuntimeException(e); } }, ContextCompat.getMainExecutor(this)); }
Menonaktifkan ekstensi
Untuk menonaktifkan ekstensi vendor, putuskan ikatan semua kasus penggunaan dan ikat ulang kasus penggunaan
pratinjau dan pengambilan gambar dengan pemilih kamera normal. Misalnya, ikat ulang ke
kamera belakang menggunakan CameraSelector.DEFAULT_BACK_CAMERA
.
Dependensi
Extensions API CameraX diterapkan di library camera-extensions
.
Ekstensi bergantung pada modul inti CameraX (core
, camera2
,
lifecycle
).
Groovy
dependencies { def camerax_version = "1.2.0-rc01" implementation "androidx.camera:camera-core:${camerax_version}" implementation "androidx.camera:camera-camera2:${camerax_version}" implementation "androidx.camera:camera-lifecycle:${camerax_version}" //the CameraX Extensions library implementation "androidx.camera:camera-extensions:${camerax_version}" ... }
Kotlin
dependencies { val camerax_version = "1.2.0-rc01" implementation("androidx.camera:camera-core:${camerax_version}") implementation("androidx.camera:camera-camera2:${camerax_version}") implementation("androidx.camera:camera-lifecycle:${camerax_version}") // the CameraX Extensions library implementation("androidx.camera:camera-extensions:${camerax_version}") ... }
Penghapusan API lama
Dengan Extensions API baru yang dirilis pada 1.0.0-alpha26
, Extensions API
lama yang dirilis pada bulan Agustus 2019 kini tidak digunakan lagi. Dimulai dari
versi 1.0.0-alpha28
, Extensions API lama telah dihapus dari
library. Aplikasi yang menggunakan Extensions API baru kini harus mendapatkan
CameraSelector
yang mendukung ekstensi dan menggunakannya untuk mengikat kasus penggunaan.
Aplikasi yang menggunakan Extensions API lama harus dimigrasikan ke Extensions API baru untuk memastikan kompatibilitas dengan rilis CameraX mendatang.
Referensi lainnya
Untuk mempelajari CameraX lebih lanjut, lihat referensi tambahan berikut.
Codelab
Contoh kode
Aplikasi Contoh Ekstensi CameraX
Referensi lainnya
Alat Validasi Ekstensi Vendor CameraX