アプリの開発において、テストは非常に重要なプロセスです。通常は、エミュレータまたはデバイスでアプリを実行して、コードが想定どおりに動作することを手動で確認します。ただし、手動テストは時間がかかり、エラーが発生しやすくなります。また、さまざまなサイズの画面やデバイスで実行されるアプリでは、管理が困難になることもあります。手動テストの問題は、多くの場合、開発に単一のデバイスを使用している結果です。その結果、フォーム ファクタが異なる他のデバイスではエラーが検知されない可能性があります。
さまざまなウィンドウ サイズと画面サイズでの回帰を特定するには、自動テストを実装して、アプリの動作と外観がさまざまなフォーム ファクタで整合していることを確認します。自動テストでは問題を早期に特定できるため、ユーザー エクスペリエンスに問題が及ぶリスクを軽減できます。
テスト項目
さまざまな画面サイズとウィンドウサイズ向けの UI を開発する場合は、次の 2 つの点に特に注意してください。
- サイズの異なるウィンドウでコンポーネントとレイアウトの視覚的属性がどのように異なるか
- 構成の変更後も状態を保持する方法
視覚的属性
さまざまなウィンドウ サイズに合わせて UI をカスタマイズするかどうかにかかわらず、UI が正しく表示されることを確認する必要があります。幅と高さは、コンパクト、中程度、拡張のいずれかになります。推奨されるブレークポイントについては、ウィンドウ サイズクラスをご覧ください。
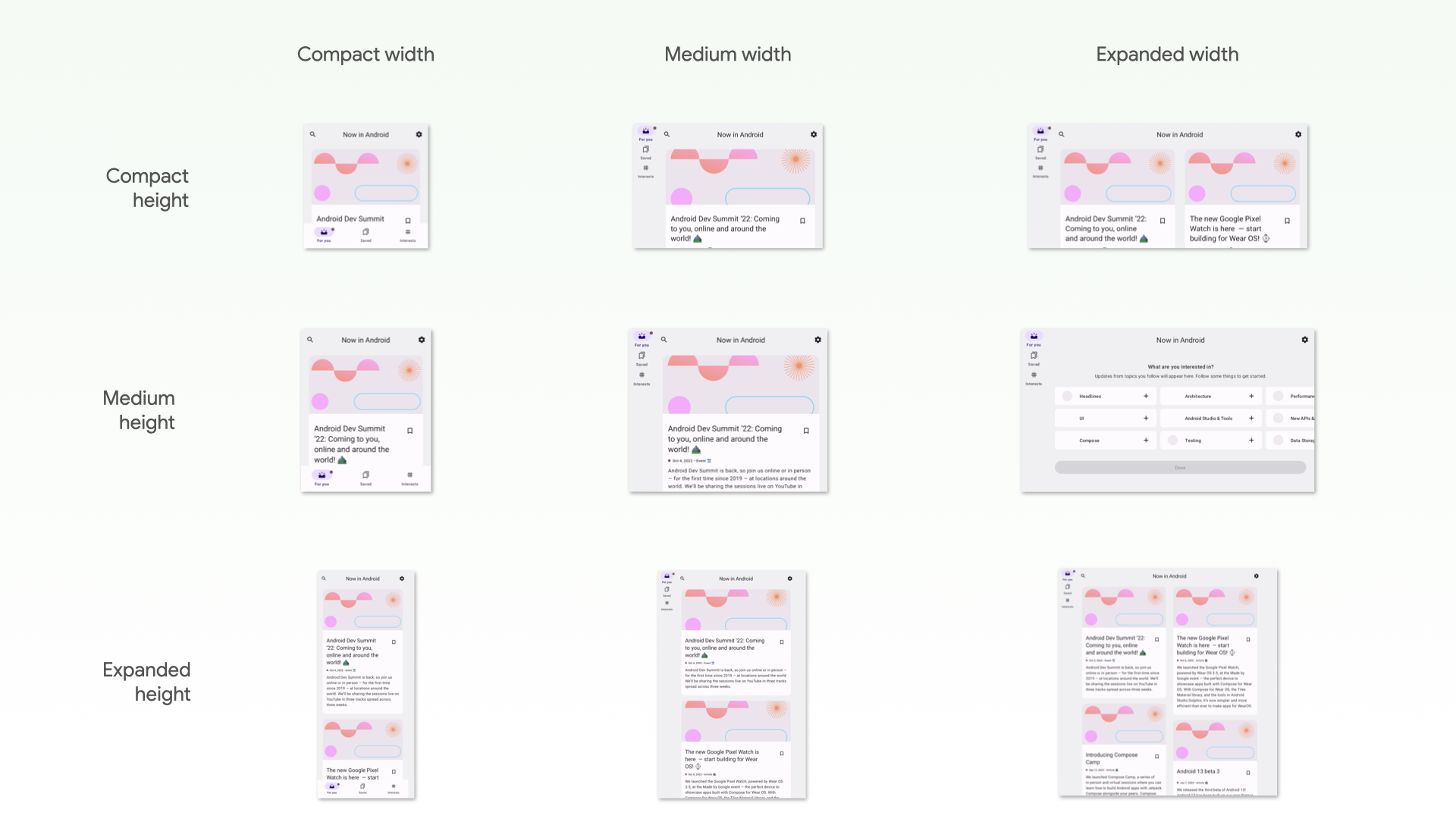
また、サイズ制約が引き伸ばされると、デザインシステムの一部のコンポーネントが想定どおりにレンダリングされない可能性があります。
アプリにさまざまなウィンドウ サイズのアダプティブ レイアウトがある場合は、リグレッションを防ぐための自動テストが必要です。たとえば、スマートフォンで余白を固定すると、タブレットでレイアウトの不整合が生じる可能性があります。UI テストを作成してレイアウトとコンポーネントの動作を確認するか、スクリーンショット テストを作成してレイアウトを視覚的に確認します。
状態の復元
タブレットなどのデバイスで実行されるアプリは、スマートフォンのアプリよりも回転とサイズ変更が頻繁に行われます。また、折りたたみ式デバイスには、折りたたみや開くなどの新しいディスプレイ機能が導入されており、構成の変更をトリガーする可能性があります。アプリは、これらの構成変更が発生したときに状態を復元できる必要があります。また、アプリの状態が正しく復元されることを確認するテストも作成する必要があります。
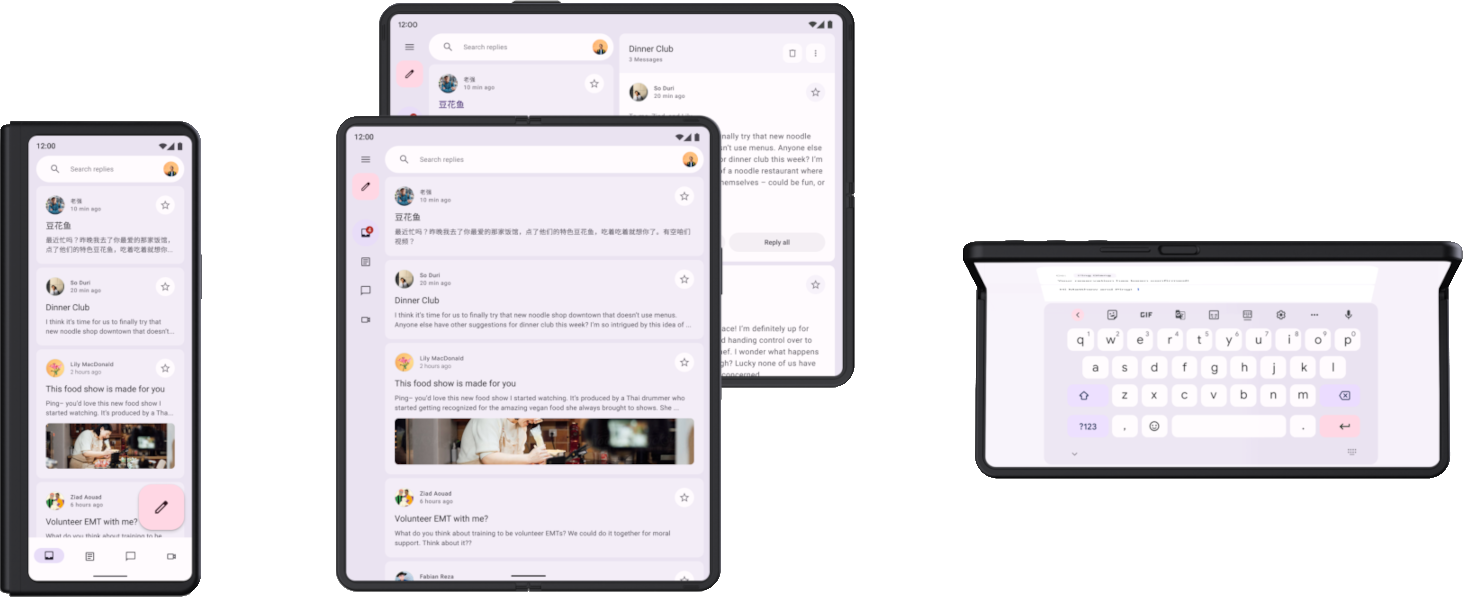
まず、構成変更が発生したときにアプリがクラッシュしないことをテストします。アプリ内のすべての UI が、回転、サイズ変更、折りたたみの組み合わせを処理できるようにします。構成変更によりアクティビティがデフォルトで再作成されるため、アクティビティの永続性が想定されているためにクラッシュが発生することがあります。
構成変更をテストする方法は複数ありますが、ほとんどの場合、次の 2 つの方法があります。
- Compose では、
StateRestorationTester
を使用して、アクティビティを再起動せずに構成変更を効率的にシミュレートします。詳細については、以下のセクションをご覧ください。 - Espresso や Compose などの UI テストでは、
Activity.recreate()
を呼び出して構成変更をシミュレートします。
通常、構成変更に応じた状態の復元をテストするために別のデバイスを使用する必要はありません。これは、アクティビティを再作成するすべての構成変更に同様の影響があるためです。ただし、一部の構成変更により、特定のデバイスで異なる状態復元メカニズムがトリガーされる場合があります。
たとえば、ユーザーが開いた折りたたみ式デバイスでリスト詳細 UI を表示していて、デバイスを折りたたんでフロント ディスプレイに切り替えると、通常、UI は詳細ページに切り替わります。自動テストでは、ナビゲーション ステータスを含む UI 状態の復元をカバーする必要があります。
デバイスが 1 つのディスプレイから別のディスプレイに移動したり、マルチウィンドウ モードに入ったりしたときに発生する構成の変更をテストするには、複数の方法があります。
- 任意のデバイスを使用して、テスト中に画面のサイズを変更します。ほとんどの場合、これにより、検証が必要なすべての状態復元メカニズムがトリガーされます。ただし、ポーズの変更が構成変更をトリガーしないため、このテストは、折りたたみ式デバイスの特定のポーズを検出するロジックでは機能しません。
- テストする機能をサポートするデバイスまたはエミュレータを使用して、関連する構成変更をトリガーします。たとえば、折りたたみ式デバイスやタブレットは、Espresso Device を使用して、折りたたみから横向きに開くように操作できます。例については、さまざまな画面サイズをテストするためのライブラリとツールの Espresso Device セクションをご覧ください。
さまざまな画面サイズとウィンドウサイズのテストタイプ
ユースケースごとに適切なタイプのテストを使用して、さまざまなフォーム ファクタでテストが正しく動作していることを確認します。
UI 動作テストは、アクティビティの表示など、アプリ UI の一部を起動します。テストでは、特定の要素が存在すること、または特定の属性を持つことを確認します。テストでは、必要に応じてシミュレートされたユーザー アクションを実行することがあります。ビューの場合は Espresso を使用します。Jetpack Compose には独自のテスト API があります。UI 動作テストは、インストルメンテーションまたはローカルにできます。インストルメンテーション テストはデバイスまたはエミュレータで実行されますが、ローカル UI テストは JVM の Robolectric で実行されます。
UI 動作テストを使用して、アプリのナビゲーションの実装が正しいことを確認します。テストでは、クリックやスワイプなどのアクションを実行します。UI 動作テストでは、特定の要素やプロパティの存在も確認します。詳細については、UI テストを自動化するをご覧ください。
スクリーンショット テストでは、UI またはコンポーネントのスクリーンショットを取得し、画像を以前に承認されたスクリーンショットと比較します。1 つのスクリーンショットで多数の要素とその視覚プロパティをカバーできるため、これは回帰を防ぐ非常に効果的な方法です。スクリーンショット テストは、JVM またはデバイスで実行できます。利用可能なスクリーンショット テスト フレームワークは複数あります。詳細については、スクリーンショット テストをご覧ください。
最後に、デバイスの種類やウィンドウサイズに応じて動作が異なるロジック単位の機能をテストするために単体テストが必要になる場合がありますが、この分野では単体テストはあまり一般的ではありません。
次のステップ
このドキュメントに記載されているチェックを実装する方法について詳しくは、ライブラリとツールをご覧ください。