Mặt đồng hồ Wear OS liên tục hoạt động nên phải sử dụng nguồn điện một cách hiệu quả.
Tối ưu hoá hết mức hiệu suất của mặt đồng hồ. Trang này cung cấp các phương pháp hay nhất cho quá trình tối ưu hoá tổng thể mặt đồng hồ cũng như các phương pháp hay nhất được nhắm mục tiêu cho ảnh động và hình ảnh.
Tối ưu hoá những yếu tố cơ bản
Phần này có chứa các phương pháp hay nhất để cải thiện hiệu quả tổng thể cho mặt đồng hồ.
Màu và độ sáng của mặt đồng hồ
Khi sử dụng màu tối cho mặt đồng hồ, đồng hồ của người dùng sẽ tiêu tốn ít pin hơn. Dưới đây là những đề xuất về cách đặt nền cho mặt đồng hồ để tối ưu hoá mức sử dụng pin của mặt đồng hồ:
- Màu: bất cứ khi nào có thể, hãy dùng nền màu đen.
- Độ sáng: khi không thể dùng nền màu đen, hãy duy trì độ sáng của màu nền ở mức bằng hoặc dưới 25% trên thang tông màu, độ bão hoà màu, giá trị màu (HSV) hay thang tông màu, độ bão hoà màu, độ sáng (HSB). Ví dụ: nếu bạn sử dụng lớp
Color
để đặt màu nền được xác định theo thang HSV, hãy sử dụng giá trị 25 trở xuống cho chế độ cài đặt giá trị giúp kiểm soát độ sáng.
Sử dụng các tính năng linh hoạt để tương tác với điện thoại
Khi mặt đồng hồ yêu cầu một thao tác để chạy trên điện thoại, nhớ chỉ thực thi mã khi mặt đồng hồ đang hoạt động. Sử dụng phương thức đề xuất API CapabilityClient để giúp ứng dụng trên điện thoại biết rằng mặt đồng hồ tương ứng đang hoạt động.
Theo dõi mức tiêu thụ pin
Ứng dụng đồng hành Wear OS giúp các nhà phát triển và người dùng xem mức tiêu thụ pin của nhiều quy trình trên thiết bị đeo. Để xem thông tin này, hãy chuyển tới phần Cài đặt > Pin đồng hồ.
Đăng ký mặt đồng hồ có khả năng nhận biết quá trình mã hoá
Android 7.0 trở lên có hỗ trợ quá trình mã hoá dựa trên tệp và cho phép các ứng dụng có khả năng nhận biết quá trình mã hoá chạy trước khi người dùng cung cấp mật mã giải mã khi khởi động. Điều này có thể làm giảm thời lượng chuyển đổi từ ảnh động khởi động sang mặt đồng hồ tới 30 giây.
Để giúp khởi động nhanh hơn, hãy thêm android:directBootAware="true"
vào tệp kê khai của mặt đồng hồ.
Lưu ý: Hãy dùng tính năng này với mặt đồng hồ không sử dụng bộ nhớ đã mã hoá thông tin xác thực.
Các phương pháp hay nhất về ảnh động
Các phương pháp hay nhất trong phần này giúp giảm mức tiêu thụ pin liên quan đến ảnh động.
Giảm tốc độ khung hình của ảnh động
Ảnh động thường tiêu tốn tài nguyên tính toán và dùng một lượng pin đáng kể. Hầu hết các ảnh động đều trông mượt mà ở mức 30 khung hình/giây. Vì vậy, bạn nên tránh chạy ảnh động ở tốc độ khung hình cao hơn. Thay vào đó, bạn có thể sử dụng tốc độ khung hình linh động. Để biết thêm thông tin, hãy xem Canvas mẫu cho mặt đồng hồ.
Cho phép CPU ngủ giữa các ảnh động
Để tăng tối đa thời lượng pin, hãy hạn chế dùng ảnh động. Ngay cả dấu hai chấm nhấp nháy cũng làm tốn pin mỗi khi nhấp nháy.
Ảnh động và các thay đổi nhỏ về nội dung của mặt đồng hồ sẽ đánh thức CPU. Cho phép CPU ngủ giữa các ảnh động, chẳng hạn như sử dụng các loạt ảnh động ngắn mỗi giây ở chế độ tương tác, sau đó để CPU ngủ cho đến giây tiếp theo. Việc để CPU thường xuyên ngủ, thậm chí trong một thời gian ngắn, có thể làm giảm đáng kể mức tiêu thụ pin.
Các phương pháp hay nhất về hình ảnh
Các phương pháp hay nhất trong phần này giúp giảm mức tiêu thụ pin có liên quan đến hình ảnh.
Giảm kích thước của thành phần bitmap
Nhiều mặt đồng hồ hiển thị một hình nền cùng với các thành phần đồ hoạ khác được biến đổi và chồng lên trên hình nền, chẳng hạn như kim đồng hồ và các thành phần khác di chuyển theo thời gian. Các thành phần đồ hoạ này càng lớn thì càng tiêu tốn tài nguyên tính toán để chuyển đổi các thành phần đó. Thông thường, các thành phần đồ hoạ này được xoay (và đôi khi được điều chỉnh theo tỷ lệ) bên trong phương thức Render.CanvasRenderer.render()
mỗi khi hệ thống vẽ lại mặt đồng hồ, như mô tả trong bài viết Vẽ mặt đồng hồ.
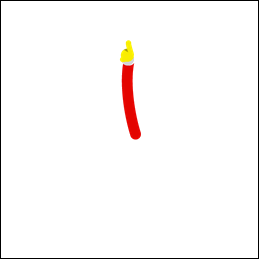

Hình 1. Cắt kim đồng hồ để loại bỏ các điểm ảnh thừa.
Việc giảm kích thước của các thành phần bitmap giúp cải thiện hiệu suất của ảnh động và tiết kiệm pin. Hãy làm theo các mẹo sau để cải thiện hiệu suất của mặt đồng hồ:
- Không được sử dụng các thành phần đồ hoạ có kích thước lớn hơn mức cần thiết.
- Loại bỏ các điểm ảnh thừa trong suốt xung quanh cạnh.
Ví dụ: có thể giảm 97% kích thước của hình ảnh kim đồng hồ ở bên trái trong hình 1 bằng cách xoá các điểm ảnh thừa trong suốt, như minh hoạ ở hình bên phải.
Kết hợp các thành phần bitmap
Nếu bạn có các bitmap thường được vẽ cùng nhau, hãy cân nhắc kết hợp các bitmap này vào một thành phần đồ hoạ. Ví dụ: bạn thường có thể kết hợp hình nền ở chế độ tương tác bằng các dấu kiểm để tránh vẽ 2 bitmap toàn màn hình mỗi khi hệ thống vẽ lại mặt đồng hồ.
Tắt tính năng khử răng cưa khi vẽ các bitmap được điều chỉnh theo tỷ lệ
Khi vẽ một bitmap được điều chỉnh theo tỷ lệ trên đối tượng Canvas
bằng cách sử dụng phương thức
Canvas.drawBitmap()
, bạn có thể cung cấp một thực thể Paint
để định cấu hình một số tùy chọn. Để cải thiện hiệu suất, hãy tắt tính năng khử răng cưa bằng phương thức
setAntiAlias()
, vì tùy chọn này không có bất kỳ ảnh hưởng nào đến bitmap.
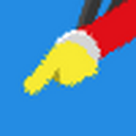
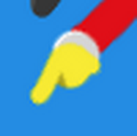
Hình 2. Ví dụ về cách lọc bitmap bị tắt (bên trái) và bật (phải).
Dùng bộ lọc bitmap
Đối với thành phần bitmap mà bạn vẽ dựa trên các phần tử khác, hãy bật tính năng lọc bitmap trên cùng một thực thể Paint
bằng cách sử dụng phương thức setFilterBitmap()
. Hình 2 cho thấy khung hiển thị phóng to của kim đồng hồ khi có và không có tính năng lọc bitmap.
Lưu ý: Khi chế độ môi trường xung quanh đang hoạt động, hãy tắt tính năng lọc bitmap. Ở chế độ môi trường xung quanh có bit thấp, hệ thống không hiển thị màu sắc trong ảnh một cách đáng tin cậy để xử lý thành công quá trình lọc bitmap.
Di chuyển các toán tử gây tốn tài nguyên ra ngoài phương thức vẽ
Hệ thống sẽ gọi phương thức Render.CanvasRenderer.render()
mỗi khi vẽ lại mặt đồng hồ. Để cải thiện hiệu suất, bạn chỉ nên đưa các thao tác có trong phương thức này. Đây là những thao tác bắt buộc để cập nhật mặt đồng hồ.
Khi có thể, hãy tránh thực hiện những thao tác sau đây trong phương thức Render.CanvasRenderer.render()
:
- Tải hình ảnh và các tài nguyên khác
- Đổi kích thước hình ảnh
- Phân bổ đối tượng
- Thao tác tính toán có kết quả không thay đổi giữa các khung hình
Để phân tích hiệu suất của mặt đồng hồ, hãy sử dụng Trình phân tích CPU. Cụ thể, hãy đảm bảo thời gian thực thi của phương thức triển khai Render.CanvasRenderer.render()
là ngắn và nhất quán giữa các lệnh gọi. Để biết thêm thông tin, hãy xem bài viết Kiểm tra hoạt động của CPU bằng Trình phân tích CPU.
Thông tin có liên quan
Ứng dụng mẫu về mặt đồng hồ minh hoạ các phương pháp hay nhất để định cấu hình mặt đồng hồ.