Auf Geräten mit Android 8.0 (API-Level 26) und höher wird durch das Koppeln von Companion-Geräten im Namen deiner App eine Bluetooth- oder WLAN-Suche der Geräte in der Nähe durchgeführt, ohne dass die Berechtigung ACCESS_FINE_LOCATION
erforderlich ist. So wird der Datenschutz für Nutzer maximiert. Nachdem das Gerät gekoppelt wurde, kann es die Berechtigungen REQUEST_COMPANION_RUN_IN_BACKGROUND
und REQUEST_COMPANION_USE_DATA_IN_BACKGROUND
nutzen, um die App im Hintergrund zu starten. Verwenden Sie diese Methode, um die Erstkonfiguration des Begleitgeräts, z. B. einer BLE-fähigen Smartwatch, vorzunehmen. Für das Koppeln des Begleitgeräts müssen außerdem die Standortdienste aktiviert sein.
Beim Koppeln von Companion-Geräten werden keine eigenständigen Verbindungen hergestellt. Bluetooth- und Wi-Fi-Konnektivitäts-APIs stellen Verbindungen her. Auch das Koppeln von Begleitgeräten ermöglicht kontinuierliches Scannen.
Ein Nutzer kann ein Gerät aus einer Liste auswählen und ihm Zugriff auf eine App gewähren. Diese Berechtigungen werden widerrufen, wenn Sie die App deinstallieren oder disassociate()
aufrufen.
Eine App ist für das Löschen ihrer eigenen Verknüpfungen verantwortlich, wenn der Nutzer sie nicht mehr benötigt, z. B. wenn er sich abmeldet oder verbundene Geräte entfernt.
Kopplung von Companion-Geräten implementieren
Verwenden Sie den CompanionDeviceManager
, um eine Verbindung zu einem Begleitgerät herzustellen und zu verwalten.
In diesem Abschnitt wird erläutert, wie Sie das Dialogfeld für die Kopplungsanfrage anpassen, wenn Sie Ihre App über Bluetooth, BLE und WLAN mit Companion-Geräten koppeln.
Companion-Geräte angeben
Das folgende Codebeispiel zeigt, wie Sie einer Manifestdatei das Flag <uses-feature>
hinzufügen. Dadurch wird dem System mitgeteilt, dass deine App Companion-Geräte einrichten möchte.
<uses-feature android:name="android.software.companion_device_setup"/>
Geräte nach Typ auflisten
Sie können alle verfügbaren Companion-Geräte anzeigen lassen, die dem von Ihnen angegebenen Filter entsprechen, oder die Anzeige auf eine einzige Option beschränken (siehe Abbildung 1). Dazu erstellen Sie einen Filter, der angibt, nach welchen Gerätetypen Ihre App sucht, oder indem Sie setSingleDevice()
auf true
setzen (siehe Abbildung 2).
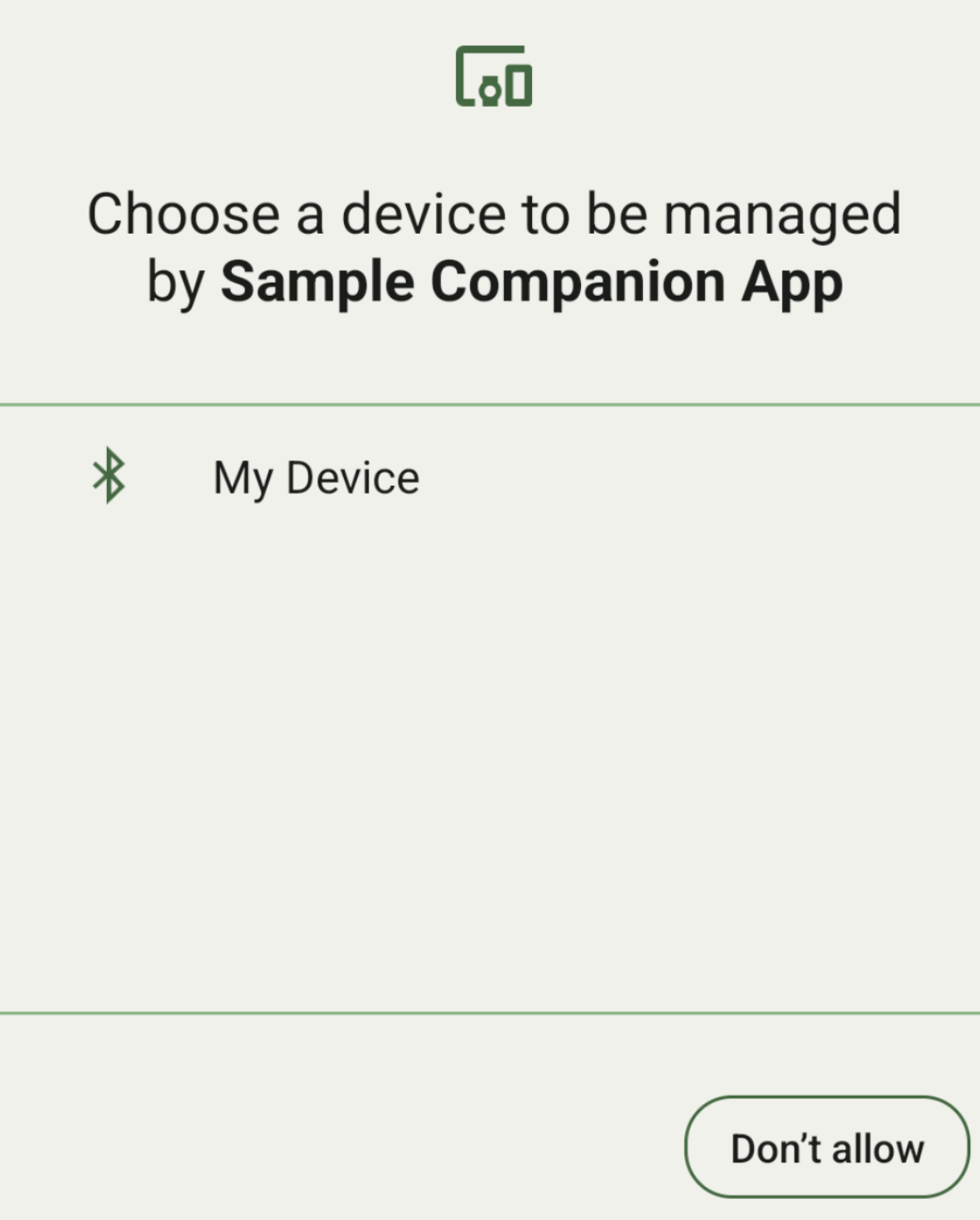
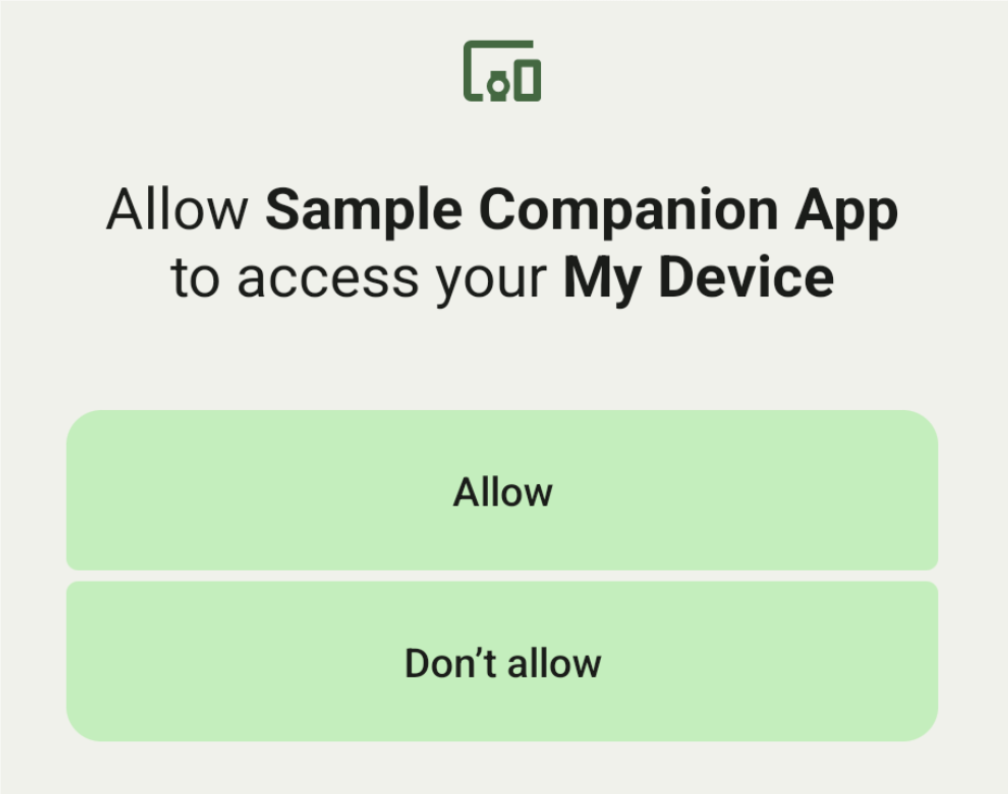
Wenn Sie einen Filter auf die Liste der Begleitgeräte anwenden möchten, die im Dialogfeld für Anfragen angezeigt wird, prüfen Sie, ob Bluetooth aktiviert ist oder prüfen Sie, ob WLAN aktiviert ist.
Nachdem die Verbindung aktiviert wurde, können Sie einen DeviceFilter
hinzufügen. Die folgenden abgeleiteten Klassen von DeviceFilter
geben die Gerätetypen an, mit denen Ihre App basierend auf einem Verbindungstyp verknüpft werden kann:
Alle drei Unterklassen verfügen über Builder, die die Konfiguration der Filter optimieren.
Im folgenden Beispiel sucht ein Gerät nach einem Bluetooth-Gerät mit einer BluetoothDeviceFilter
.
Kotlin
val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() // Match only Bluetooth devices whose name matches the pattern. .setNamePattern(Pattern.compile("My device")) // Match only Bluetooth devices whose service UUID matches this pattern. .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build()
Java
BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() // Match only Bluetooth devices whose name matches the pattern. .setNamePattern(Pattern.compile("My device")) // Match only Bluetooth devices whose service UUID matches this pattern. .addServiceUuid(new ParcelUuid(new UUID(0x123abcL, -1L)), null) .build();
Legen Sie für DeviceFilter
ein AssociationRequest
fest, damit der Gerätemanager bestimmen kann, nach welchem Gerätetyp gesucht werden soll.
Kotlin
val pairingRequest: AssociationRequest = AssociationRequest.Builder() // Find only devices that match this request filter. .addDeviceFilter(deviceFilter) // Stop scanning as soon as one device matching the filter is found. .setSingleDevice(true) .build()
Java
AssociationRequest pairingRequest = new AssociationRequest.Builder() // Find only devices that match this request filter. .addDeviceFilter(deviceFilter) // Stop scanning as soon as one device matching the filter is found. .setSingleDevice(true) .build();
Führen Sie nach dem Initialisieren eines AssociationRequest
die Funktion associate()
für CompanionDeviceManager
aus. Die Funktion associate()
koppelt Anfrageobjekt und Callback. Callbacks geben an, wenn eine App ein Gerät findet und ein Dialogfeld öffnen kann, in dem der Nutzer seine Auswahl eingeben kann.
Wenn eine App keine Geräte findet, gibt der Callback eine Fehlermeldung zurück.
Auf Geräten mit Android 13 (API-Level 33) und höher:
Kotlin
val deviceManager = requireContext().getSystemService(Context.COMPANION_DEVICE_SERVICE) val executor: Executor = Executor { it.run() } deviceManager.associate(pairingRequest, executor, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onAssociationPending(intentSender: IntentSender) { intentSender?.let { startIntentSenderForResult(it, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } } override fun onAssociationCreated(associationInfo: AssociationInfo) { // The association is created. } override fun onFailure(errorMessage: CharSequence?) { // Handle the failure. } })
Java
CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService(Context.COMPANION_DEVICE_SERVICE); Executor executor = new Executor() { @Override public void execute(Runnable runnable) { runnable.run(); } }; deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { executor, // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onAssociationCreated(AssociationInfo associationInfo) { // The association is created. } @Override public void onFailure(CharSequence errorMessage) { // Handle the failure. });
Auf Geräten mit Android 12L (API-Level 32) und niedriger (eingestellt):
Kotlin
val deviceManager = requireContext().getSystemService(Context.COMPANION_DEVICE_SERVICE) deviceManager.associate(pairingRequest, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onDeviceFound(chooserLauncher: IntentSender) { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } override fun onFailure(error: CharSequence?) { // Handle the failure. } }, null)
Java
CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService(Context.COMPANION_DEVICE_SERVICE); deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onFailure(CharSequence error) { // Handle the failure. } }, null);
Damit Nutzer auswählen können, mit welchem Gerätetyp sie eine Verbindung herstellen möchten, starten Sie mit dem Parameter intentSender
in der Funktion onAssociationPending()
eine Einstellungsaktivität. Die Ergebnisse dieser Aktion werden an das Fragment in der Funktion onActivityResult()
Ihrer Einstellungsaktivität zurückgesendet. Sie werden informiert, wenn der Nutzer anhand des Ergebnisses eine Auswahl trifft. Anschließend kannst du auf das ausgewählte Gerät zugreifen. Wenn ein Nutzer ein Bluetooth-Gerät auswählt, wird als Ergebnis ein BluetoothDevice
-Objekt gesendet.
Wenn die Funktion onAssociationPending()
erkennt, dass ein Nutzer ein Bluetooth LE-Gerät auswählt, wird ein android.bluetooth.le.ScanResult
-Objekt erwartet. Für WLAN-Geräte wird ein android.net.wifi.ScanResult
-Objekt erwartet.
Kotlin
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Continue to interact with the paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } }
Java
@Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (resultCode != Activity.RESULT_OK) { return; } if (requestCode == SELECT_DEVICE_REQUEST_CODE && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE); if (deviceToPair != null) { deviceToPair.createBond(); // Continue to interact with the paired device. } } else { super.onActivityResult(requestCode, resultCode, data); } }
In den folgenden Beispielen wird die Kopplung von Companion-Geräten mit Filtern implementiert, die Geräte angeben und nach Typ auflisten können:
Auf Geräten mit Android 13 (API-Level 33) und höher:
Kotlin
private const val SELECT_DEVICE_REQUEST_CODE = 0 class MainActivity : AppCompatActivity() { private val deviceManager: CompanionDeviceManager by lazy { getSystemService(Context.COMPANION_DEVICE_SERVICE) as CompanionDeviceManager } val mBluetoothAdapter: BluetoothAdapter by lazy { val java = BluetoothManager::class.java getSystemService(java)!!.adapter } val executor: Executor = Executor { it.run() } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // To skip filters based on names and supported feature flags (UUIDs), // omit calls to setNamePattern() and addServiceUuid() // respectively, as shown in the following Bluetooth example. val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build() // The argument provided in setSingleDevice() determines whether a single // device name or a list of them appears. val pairingRequest: AssociationRequest = AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build() // When the app tries to pair with a Bluetooth device, show the // corresponding dialog box to the user. deviceManager.associate(pairingRequest, executor, object : CompanionDeviceManager.Callback() { // Called when a device is found. Launch the IntentSender so the user // can select the device they want to pair with. override fun onAssociationPending(intentSender: IntentSender) { intentSender?.let { startIntentSenderForResult(it, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } } override fun onAssociationCreated(associationInfo: AssociationInfo) { // AssociationInfo object is created and get association id and the // macAddress. var associationId: int = associationInfo.id var macAddress: MacAddress = associationInfo.deviceMacAddress } override fun onFailure(errorMessage: CharSequence?) { // Handle the failure. } ) override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Maintain continuous interaction with a paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } } }
Java
class MainActivityJava extends AppCompatActivity { private static final int SELECT_DEVICE_REQUEST_CODE = 0; Executor executor = new Executor() { @Override public void execute(Runnable runnable) { runnable.run(); } }; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService( Context.COMPANION_DEVICE_SERVICE ); // To skip filtering based on name and supported feature flags, // do not include calls to setNamePattern() and addServiceUuid(), // respectively. This example uses Bluetooth. BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid( new ParcelUuid(new UUID(0x123abcL, -1L)), null ) .build(); // The argument provided in setSingleDevice() determines whether a single // device name or a list of device names is presented to the user as // pairing options. AssociationRequest pairingRequest = new AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build(); // When the app tries to pair with the Bluetooth device, show the // appropriate pairing request dialog to the user. deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { executor, // Called when a device is found. Launch the IntentSender so the user can // select the device they want to pair with. @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult( chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0 ); } catch (IntentSender.SendIntentException e) { Log.e("MainActivity", "Failed to send intent"); } } @Override public void onAssociationCreated(AssociationInfo associationInfo) { // AssociationInfo object is created and get association id and the // macAddress. int associationId = associationInfo.getId(); MacAddress macAddress = associationInfo.getDeviceMacAddress(); } @Override public void onFailure(CharSequence errorMessage) { // Handle the failure. }); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (resultCode != Activity.RESULT_OK) { return; } if (requestCode == SELECT_DEVICE_REQUEST_CODE) { if (resultCode == Activity.RESULT_OK && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra( CompanionDeviceManager.EXTRA_DEVICE ); if (deviceToPair != null) { deviceToPair.createBond(); // ... Continue interacting with the paired device. } } } else { super.onActivityResult(requestCode, resultCode, data); } } }
Auf Geräten mit Android 12L (API-Level 32) und niedriger (eingestellt):
Kotlin
private const val SELECT_DEVICE_REQUEST_CODE = 0 class MainActivity : AppCompatActivity() { private val deviceManager: CompanionDeviceManager by lazy { getSystemService(Context.COMPANION_DEVICE_SERVICE) as CompanionDeviceManager } override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) // To skip filters based on names and supported feature flags (UUIDs), // omit calls to setNamePattern() and addServiceUuid() // respectively, as shown in the following Bluetooth example. val deviceFilter: BluetoothDeviceFilter = BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid(ParcelUuid(UUID(0x123abcL, -1L)), null) .build() // The argument provided in setSingleDevice() determines whether a single // device name or a list of them appears. val pairingRequest: AssociationRequest = AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build() // When the app tries to pair with a Bluetooth device, show the // corresponding dialog box to the user. deviceManager.associate(pairingRequest, object : CompanionDeviceManager.Callback() { override fun onDeviceFound(chooserLauncher: IntentSender) { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0) } override fun onFailure(error: CharSequence?) { // Handle the failure. } }, null) } override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) { when (requestCode) { SELECT_DEVICE_REQUEST_CODE -> when(resultCode) { Activity.RESULT_OK -> { // The user chose to pair the app with a Bluetooth device. val deviceToPair: BluetoothDevice? = data?.getParcelableExtra(CompanionDeviceManager.EXTRA_DEVICE) deviceToPair?.let { device -> device.createBond() // Maintain continuous interaction with a paired device. } } } else -> super.onActivityResult(requestCode, resultCode, data) } } }
Java
class MainActivityJava extends AppCompatActivity { private static final int SELECT_DEVICE_REQUEST_CODE = 0; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); CompanionDeviceManager deviceManager = (CompanionDeviceManager) getSystemService( Context.COMPANION_DEVICE_SERVICE ); // To skip filtering based on name and supported feature flags, // don't include calls to setNamePattern() and addServiceUuid(), // respectively. This example uses Bluetooth. BluetoothDeviceFilter deviceFilter = new BluetoothDeviceFilter.Builder() .setNamePattern(Pattern.compile("My device")) .addServiceUuid( new ParcelUuid(new UUID(0x123abcL, -1L)), null ) .build(); // The argument provided in setSingleDevice() determines whether a single // device name or a list of device names is presented to the user as // pairing options. AssociationRequest pairingRequest = new AssociationRequest.Builder() .addDeviceFilter(deviceFilter) .setSingleDevice(true) .build(); // When the app tries to pair with the Bluetooth device, show the // appropriate pairing request dialog to the user. deviceManager.associate(pairingRequest, new CompanionDeviceManager.Callback() { @Override public void onDeviceFound(IntentSender chooserLauncher) { try { startIntentSenderForResult(chooserLauncher, SELECT_DEVICE_REQUEST_CODE, null, 0, 0, 0); } catch (IntentSender.SendIntentException e) { // failed to send the intent } } @Override public void onFailure(CharSequence error) { // handle failure to find the companion device } }, null); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { if (requestCode == SELECT_DEVICE_REQUEST_CODE) { if (resultCode == Activity.RESULT_OK && data != null) { BluetoothDevice deviceToPair = data.getParcelableExtra( CompanionDeviceManager.EXTRA_DEVICE ); if (deviceToPair != null) { deviceToPair.createBond(); // ... Continue interacting with the paired device. } } } else { super.onActivityResult(requestCode, resultCode, data); } } }
Begleitgeräteprofile
Partner-Apps unter Android 12 (API-Level 31) und höher können Companion-Geräteprofile verwenden, um eine Verbindung zu einer Uhr herzustellen. Weitere Informationen findest du in der Anleitung zum Anfordern von Berechtigungen unter Wear OS.
Companion-Apps aktiv lassen
Unter Android 12 (API-Level 31) und höher können Sie zusätzliche APIs verwenden, damit Ihre Companion-App weiter ausgeführt wird, während sich ein Begleitgerät in Reichweite befindet. Mit diesen APIs können Sie Folgendes tun:
Der Ruhemodus der App wird beendet, wenn sich ein Begleitgerät in Reichweite befindet.
Weitere Informationen findest du unter
CompanionDeviceManager.startObservingDevicePresence()
.Es wird garantiert, dass ein Anwendungsprozess weiterläuft, solange das Begleitgerät in Reichweite bleibt.
Weitere Informationen findest du unter
CompanionDeviceService.onDeviceAppeared()
.