5G network slicing gives carriers the ability to provide network performance boosts for specific use cases. This guide explains how an app can trigger the network slicing upsell UX flow and request a premium connection if the user decides to purchase one.
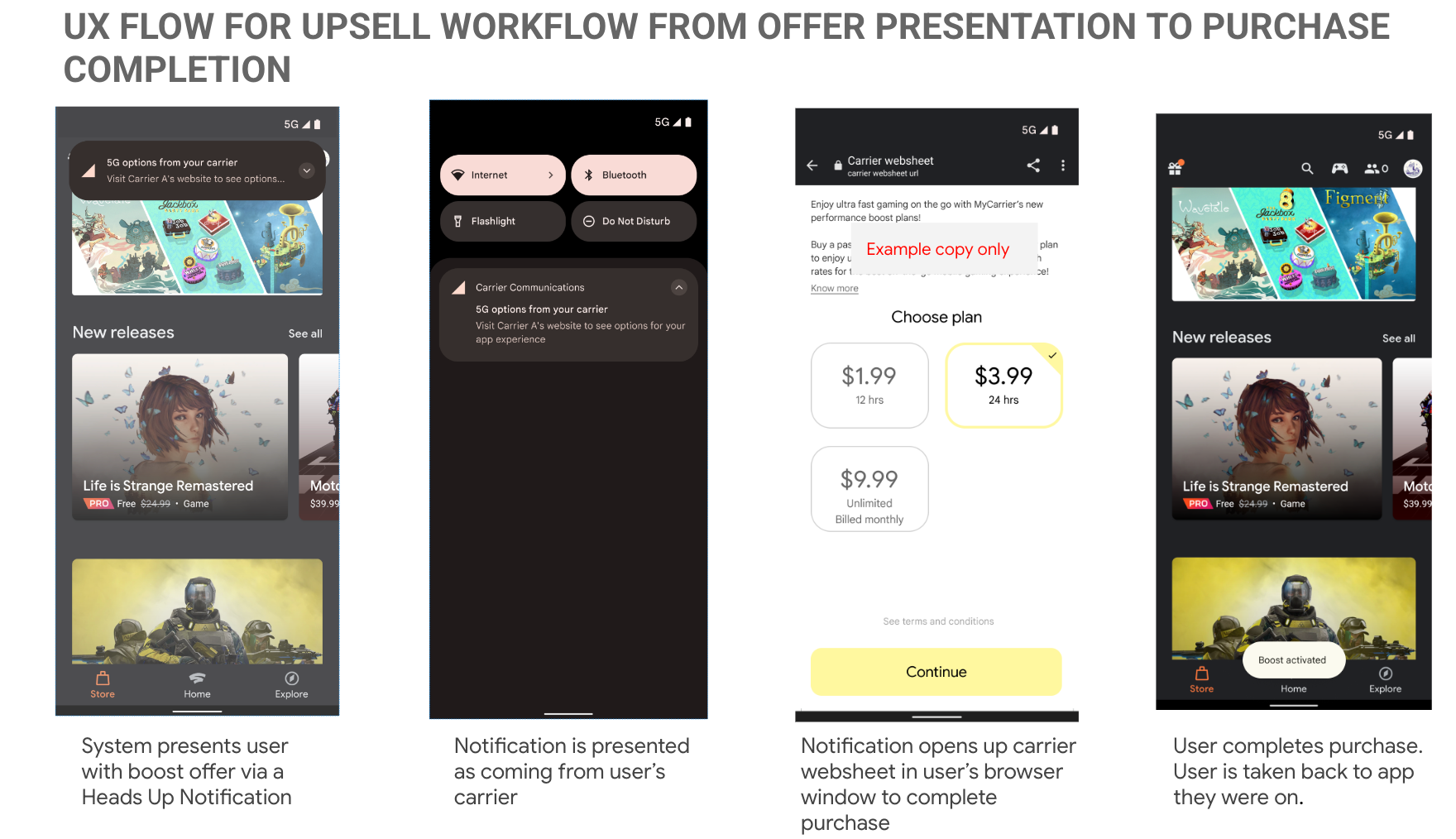
Declare premium capability intents
In order for your app's request for a network capability to be honored, your app
must declare its intent to request that capability in the app manifest.
Otherwise, the network request fails throwing a SecurityException
.
To do this, your app must declare the
PackageManager.PROPERTY_SELF_CERTIFIED_NETWORK_CAPABILITIES
property in the AndroidManifest.xml
file and include a corresponding XML
resource file.
A capability declaration in the manifest file looks like this:
<property android:name="android.net.PROPERTY_SELF_CERTIFIED_NETWORK_CAPABILITIES"
android:resource="@xml/network_capabilities" />
The corresponding network_capabilities.xml
resource file looks like this:
<network-capabilities-declaration> xmlns:android="http://schemas.android.com/apk/res/android">
<uses-network-capability android:name="NET_CAPABILITY_PRIORITIZE_LATENCY"/>
</network-capabilities-declaration>
Trigger the network slicing upsell flow
This code example demonstrates how to trigger the upsell flow and request the purchased premium capability.
Context mContext;
Network mNetwork;
public void purchasePremiumCapability() {
TelephonyManager tm = mContext.getSystemService(TelephonyManager.class);
int capability = TelephonyManager.PREMIUM_CAPABILITY_PRIORITIZE_LATENCY;
if (tm.isPremiumCapabilityAvailableForPurchase(capability)) {
tm.purchasePremiumCapability(capability, Runnable::run, new Consumer<Integer>() {
@Override
public void accept(Integer result) {
Log.d("Purchase premium capability result: "
+ TelephonyManager.convertPurchaseResultToString(result));
switch (result) {
case /* success or already purchased */:
requestPremiumCapabilityNetwork();
break;
case /* temporary failure */:
// TODO: wait and retry
break;
case /* hard failure */:
// TODO: handle failure
break;
default:
Log.e("Unknown purchase result: " + result);
}
}
});
} else {
Log.e("Premium capability is not available for purchase.");
}
}
public void requestPremiumCapabilityNetwork() {
ConnectvityManager cm = mContext.getSystemService(ConnectivityManager.class);
NetworkRequest request = NetworkRequest.Builder()
.addCapability(NetworkCapabilities.NET_CAPABILITY_PRIORITIZE_LATENCY)
.build();
cm.requestNetwork(request, new NetworkCallback() {
@Override
public void onAvailable(Network network) {
Log.d("Application can now use the network with the premium capability.");
mNetwork = network;
}
@Override
public void onLost(Network network) {
Log.d("Premium capability network is no longer available.");
mNetwork = null;
// TODO: clean up anything relying on the premium capability network
}
});
}
The following sections describe the steps involved in this process in greater detail.
Step 1: Verify if premium capability is available
Call the
isPremiumCapabilityAvailableForPurchase()
API method to determine
whether the selected premium capability is available. This method returns true
if the capability is available for purchase from the carrier using the upsell
notification workflow.
Step 2: Initiate the upsell notification flow
After confirming that the premium capability is available, your app should call
purchasePremiumCapability()
to initiate the upsell notification flow. If the user has not already purchased
the specified capability and all preconditions are satisfied, then the platform
shows the user a notification to let them know that performance boost options
might be available from their carrier. If the user taps the notification, the
platform opens the carrier's webview so that the purchase process can continue.
The parameter
callback passed to purchasePremiumCapability()
returns a
result code for the purchase request.
The result codes
PURCHASE_PREMIUM_CAPABILITY_RESULT_SUCCESS
and
PURCHASE_PREMIUM_CAPABILITY_RESULT_ALREADY_PURCHASED
represent successful results where your app can proceed to requesting the
selected premium capability.
The result codes in the following list represent failed purchase requests. See the API reference to learn more about them.
PURCHASE_PREMIUM_CAPABILITY_RESULT_ALREADY_IN_PROGRESS
PURCHASE_PREMIUM_CAPABILITY_RESULT_CARRIER_DISABLED
PURCHASE_PREMIUM_CAPABILITY_RESULT_CARRIER_ERROR
PURCHASE_PREMIUM_CAPABILITY_RESULT_ENTITLEMENT_CHECK_FAILED
PURCHASE_PREMIUM_CAPABILITY_RESULT_FEATURE_NOT_SUPPORTED
PURCHASE_PREMIUM_CAPABILITY_RESULT_NETWORK_NOT_AVAILABLE
PURCHASE_PREMIUM_CAPABILITY_RESULT_NOT_DEFAULT_DATA_SUBSCRIPTION
PURCHASE_PREMIUM_CAPABILITY_RESULT_NOT_FOREGROUND
PURCHASE_PREMIUM_CAPABILITY_RESULT_PENDING_NETWORK_SETUP
PURCHASE_PREMIUM_CAPABILITY_RESULT_REQUEST_FAILED
PURCHASE_PREMIUM_CAPABILITY_RESULT_THROTTLED
PURCHASE_PREMIUM_CAPABILITY_RESULT_TIMEOUT
PURCHASE_PREMIUM_CAPABILITY_RESULT_USER_CANCELED
Step 3: Request the purchased premium connection
If the upsell notification flow returns a successful code
(PURCHASE_PREMIUM_CAPABILITY_RESULT_SUCCESS
or
PURCHASE_PREMIUM_CAPABILITY_RESULT_ALREADY_PURCHASED
), your app should use
requestNetwork()
to request a network that satisfies the requested capability. Note that when you
build a NetworkRequest
object, the capability that you add is not the same
capability that you pass to the TelephonyManager
APIs in the earlier steps.
The following table maps the constants from the TelephonyManager
class to the
corresponding constants in NetworkCapabilities
.
TelephonyManager constant |
NetworkCapabilities constant |
---|---|
PREMIUM_CAPABILITY_PRIORITIZE_LATENCY |
NET_CAPABILITY_PRIORITIZE_LATENCY |
If the purchase request fails, your app should request and use the default network instead. There is no automatic fallback behavior if the premium slice request cannot be fulfilled.