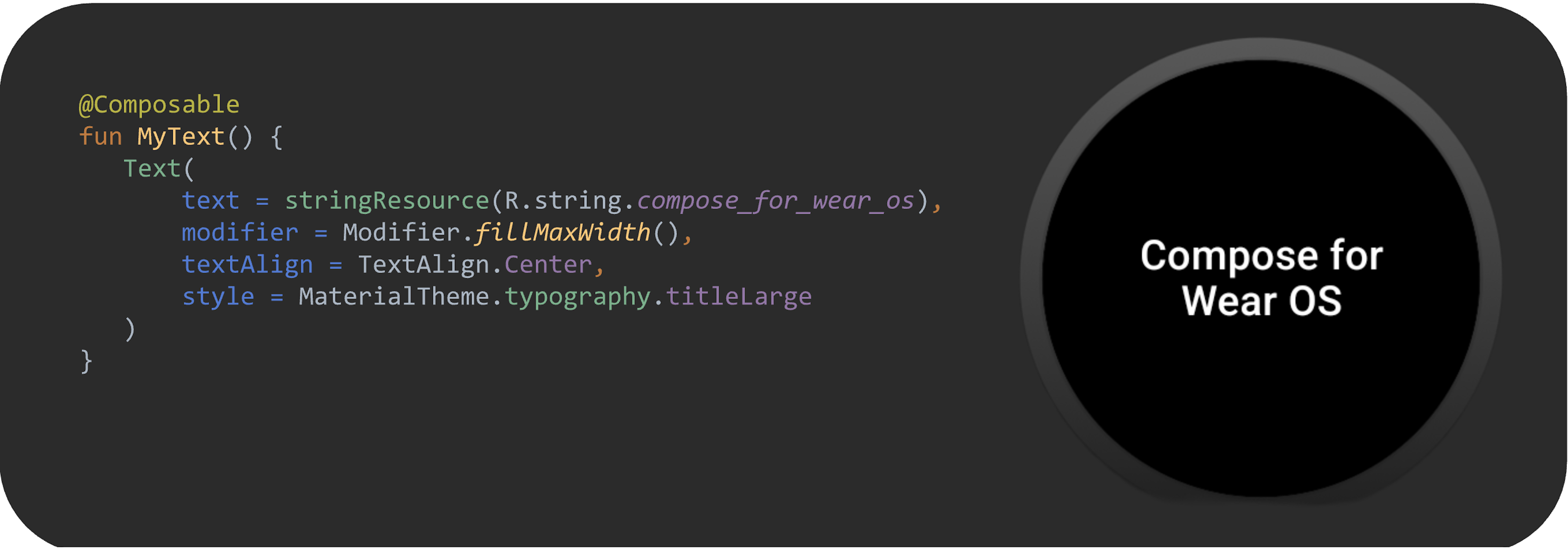
Compose for Wear OS is similar to Compose for mobile. However, there are some key differences. This guide walks you through the similarities and differences.
Compose for Wear OS is part of Android Jetpack, and like the other Wear Jetpack libraries you use, it helps you write better code faster. This is our recommended approach for building user interfaces for Wear OS apps.
If you are unfamiliar with using the Jetpack Compose toolkit, check out the Compose pathway. Many of the development principles for mobile Compose apply to Compose for Wear OS. See Why Compose for more information on the general advantages of a declarative UI framework. To learn more about Compose for Wear OS, see the Compose for Wear OS Pathway and the Wear OS samples repository on GitHub.
Material Design in Jetpack Compose on Wear OS
Jetpack Compose on Wear OS offers an implementation of Material 3, which helps you design more engaging app experiences. The Material Design components on Wear OS are built on top of Wear Material Theming. This theming is a systematic way to customize Material Design and better reflect your product's brand.
Compatibility
Compose for Wear OS works on watches that support Wear OS 3.0 (API Level 30) and watches that use Wear OS 2.0 (API level 25 and above). Using version 1.5 of Compose for Wear OS requires using version 1.8 of androidx.compose libraries and Kotlin 1.9.0. You can use the BOM mapping and Compose to Kotlin compatibility map to check Compose compatibility.Surfaces
Compose for Wear OS makes building apps on Wear OS easier. For more information see Apps. Use our built-in components to create user experiences that conform to Wear OS guidelines. For more information about components, see our design guidance.
Setting up
Using Jetpack Compose with Wear OS is similar to using Jetpack Compose for
any other Android project. The main difference is that Jetpack Compose for Wear
adds Wear-specific libraries that make it easier to create user interfaces
tailored to watches.
In some cases those components share the same name as
their non-wear counterparts, such as
androidx.wear.compose.material3.Button
and
androidx.compose.material3.Button
.
Create a new app in Android Studio
To create a new project that includes Jetpack Compose, proceed as follows:
- If you’re in the Welcome to Android Studio window, click Start a new Android Studio project. If you already have an Android Studio project open, select File > New > Import Sample from the menu bar.
- Search for Compose for Wear and select Compose for Wear OS Starter.
- In the Configure your project window, do the following:
- Set the Application name.
- Choose the Project location for your sample.
- Click Finish.
- Verify that the project's
build.gradle
file is configured correctly, as described in Gradle properties files.
Now you're ready to start developing an app using Compose for Wear OS.
Jetpack Compose toolkit dependencies
To use Jetpack Compose with Wear OS, you’ll need to include Jetpack Compose
toolkit dependencies in your app’s build.gradle
file. Most of the dependency
changes related to Wear OS are in the
top architectural layers, surrounded by a red box
in the following image.
That means many of the dependencies you already use with Jetpack Compose don't change when targeting Wear OS. For example, the UI, runtime, compiler, and animation dependencies remain the same.
However, Wear OS has its own versions of material
and material3
, foundation
, and
navigation
libraries, so check that you're using the proper libraries.
Use the
WearComposeMaterial
version of APIs where possible. While it's technically possible to use the
mobile version of Compose Material, it is not optimized for the unique
requirements of Wear OS. In addition, mixing Compose Material with Compose
Material for Wear OS can result in unexpected behavior. For example, because
each library has its own MaterialTheme
class, there's the possibility of
colors, typography, or shapes being inconsistent if both versions are used.
The following table outlines the dependency differences between Wear OS and Mobile:
Wear OS Dependency
(androidx.wear.*) |
Comparison | Mobile Dependency
(androidx.*) |
androidx.wear.compose:compose-material3 | instead of | androidx.compose.material:material3 |
androidx.wear.compose:compose-navigation | instead of | androidx.navigation:navigation-compose |
androidx.wear.compose:compose-foundation | in addition to | androidx.compose.foundation:foundation |
The following snippet shows an example build.gradle
file that includes these
dependencies:
Kotlin
dependencies { val composeBom = platform("androidx.compose:compose-bom:2025.05.00") // General compose dependencies implementation(composeBom) implementation("androidx.activity:activity-compose:1.10.1") implementation("androidx.compose.ui:ui-tooling-preview:1.8.3") // Other compose dependencies // Compose for Wear OS dependencies implementation("androidx.wear.compose:compose-material3:1.5.0-beta05") // Foundation is additive, so you can use the mobile version in your Wear OS app. implementation("androidx.wear.compose:compose-foundation:1.5.0-beta05") // Wear OS preview annotations implementation("androidx.wear.compose:compose-ui-tooling:1.5.0-beta05") // If you are using Compose Navigation, use the Wear OS version (NOT THE MOBILE VERSION). // Uncomment the line below and update the version number. // implementation("androidx.wear.compose:compose-navigation:1.5.0-beta05") // Testing testImplementation("junit:junit:4.13.2") androidTestImplementation("androidx.test.ext:junit:1.1.3") androidTestImplementation("androidx.test.espresso:espresso-core:3.4.0") androidTestImplementation("androidx.compose.ui:ui-test-junit4:1.0.3") debugImplementation("androidx.compose.ui:ui-tooling:1.4.1") }
Feedback
Try out Compose for Wear OS and use the issue tracker to provide suggestion and feedback.
Join the #compose-wear channel on Kotlin Slack to connect with developer community and let us know your experience.
Recommended for you
- Note: link text is displayed when JavaScript is off
- Resources in Compose
- Material Design 3 in Compose
- Get Started with Jetpack Compose
Content and code samples on this page are subject to the licenses described in the Content License. Java and OpenJDK are trademarks or registered trademarks of Oracle and/or its affiliates.
Last updated 2025-07-02 UTC.