This page lists the new features introduced in Android Studio preview releases. The preview builds provide early access to the latest features and improvements in Android Studio. You can download these preview versions here. If you encounter any problems using a preview version of Android Studio, please let us know. Your bug reports help to make Android Studio better.
For the latest news on Android Studio preview releases, including a list of notable fixes in each preview release, see the Release Updates in the Android Studio blog.
Current versions of Android Studio
The following table lists the current versions of Android Studio and their respective channels.
Version | Channel |
---|---|
Android Studio Koala | 2024.1.1 | Stable |
Android Gradle plugin 8.5.0 | Stable |
Android Studio Koala Feature Drop | 2024.1.2 | Beta |
Android Studio Ladybug | 2024.1.3 | Canary |
Compatibility with Android Gradle plugin previews
Each preview version of Android Studio is published alongside a corresponding version of the Android Gradle plugin (AGP). Preview versions of Studio should work with any compatible stable version of AGP. However, if you're using a preview version of AGP, you must use the corresponding preview version of Studio (for example, Android Studio Chipmunk Canary 7 with AGP 7.2.0-alpha07). Attempts to use divergent versions (for example, Android Studio Chipmunk Beta 1 with AGP 7.2.0-alpha07) will cause a Sync failure, which results in a prompt to update to the corresponding version of AGP.
For a detailed log of Android Gradle plugin API deprecations and removals, see the Android Gradle plugin API updates.
Android Studio Koala Feature Drop | 2024.1.2
In addition to the Intellij 2024.1 platform updates, the following are new features in Android Studio Koala Feature Drop | 2024.1.2. To see what's been fixed in this version of Android Studio, see the closed issues.
Android Device Streaming: more devices and improved sign-up
Android Device Streaming now includes the following devices, in addition to the portfolio of 20+ device models already available:
- Samsung Galaxy Fold5
- Samsung Galaxy S23 Ultra
- Google Pixel 8a
Additionally, if you're new to Firebase, Android Studio automatically creates and sets up a no-cost Firebase project for you when you sign in to Koala Feature Drop to use Device Streaming. So, you can get to streaming the device you need much faster. Learn more about Android Device Streaming quotas, including promotional quota for the Firebase Blaze plan projects available for a limited time.
USB cable speed detection
Android Studio now detects when it's possible to connect your Android device with a faster USB cable, and suggests an upgrade that maximizes your device capabilities. Using an appropriate USB cable optimizes app installation time and minimizes latency when using tools such as the Android Studio debugger.
The whole USB chain leading to a device is verified. If you see a "Connection speed warning" notification, check the version certification of the cables but also any hubs, including the monitor's hub, involved in the USB chain.
USB cable speed detection is available with the following:
- Devices running API level 30 (Android 11) or higher.
- Workstations running macOS or Linux. Windows support is coming soon.
- The latest version of the SDK Platform Tools.
The information provided by Android Studio is similar to the information you can get using one of the following tools, depending on your OS:
- Mac: running
system_profiler SPUSBDataType
from the terminal - Linux: running
lsusb -vvv
from the terminal
Updated sign in flow to Google services
It's now easier to sign in to multiple Google services with one authentication step. Whether you want to use Gemini in Android Studio, Firebase for Android Device Streaming, Google Play for Android Vitals reports, or all these useful services, the new sign in flow makes it easier to get up and running. If you're new to Firebase and want to use Android Device Streaming, Android Studio automatically creates a project for you, so you can quickly start streaming a real physical Firebase device. With granular permissions scoping, you will always be in control of which services have access to your account. To get started, click the profile avatar and sign in with your developer account.
Device UI setting shortcuts
To help you build and debug your UI, we have introduced Device UI setting shortcuts in the Running Devices tool window in Android Studio. Use the shortcuts to view the effect of common UI settings such as dark theme, font size, screen size, app language and TalkBack. You can use the shortcuts with emulators, mirrored physical devices, and devices streamed from Firebase Test Lab.
Note that accessibility settings such as TalkBack and Select to Speak only show up if they are already installed on the device. If you don't see those options, download the Android Accessibility Suite app from the Play Store.
Device UI setting shortcuts are available for devices running API level 33 or higher.
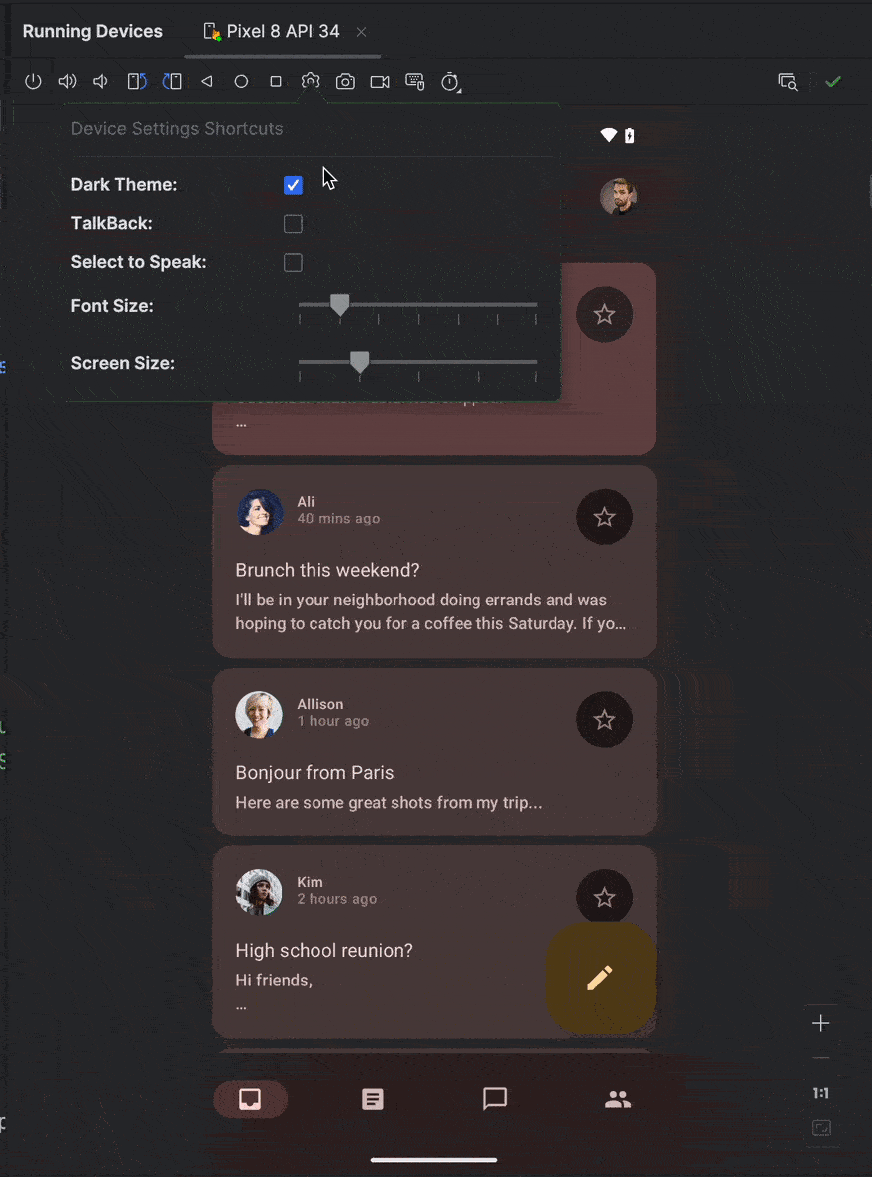
Faster and improved Profiler with a task-centric approach
We've improved the performance of the Android Studio Profiler such that popular profiling tasks like capturing a system trace with profileable apps now start up to 60% faster.
The Profiler's task-centric redesign also makes it easier to start the task you're interested in, whether it's profiling your app's CPU, memory, or power usage. For example, you can start a system trace task to profile and improve your app's startup time right from the UI as soon as you open the Profiler.
Wear OS tiles preview panel
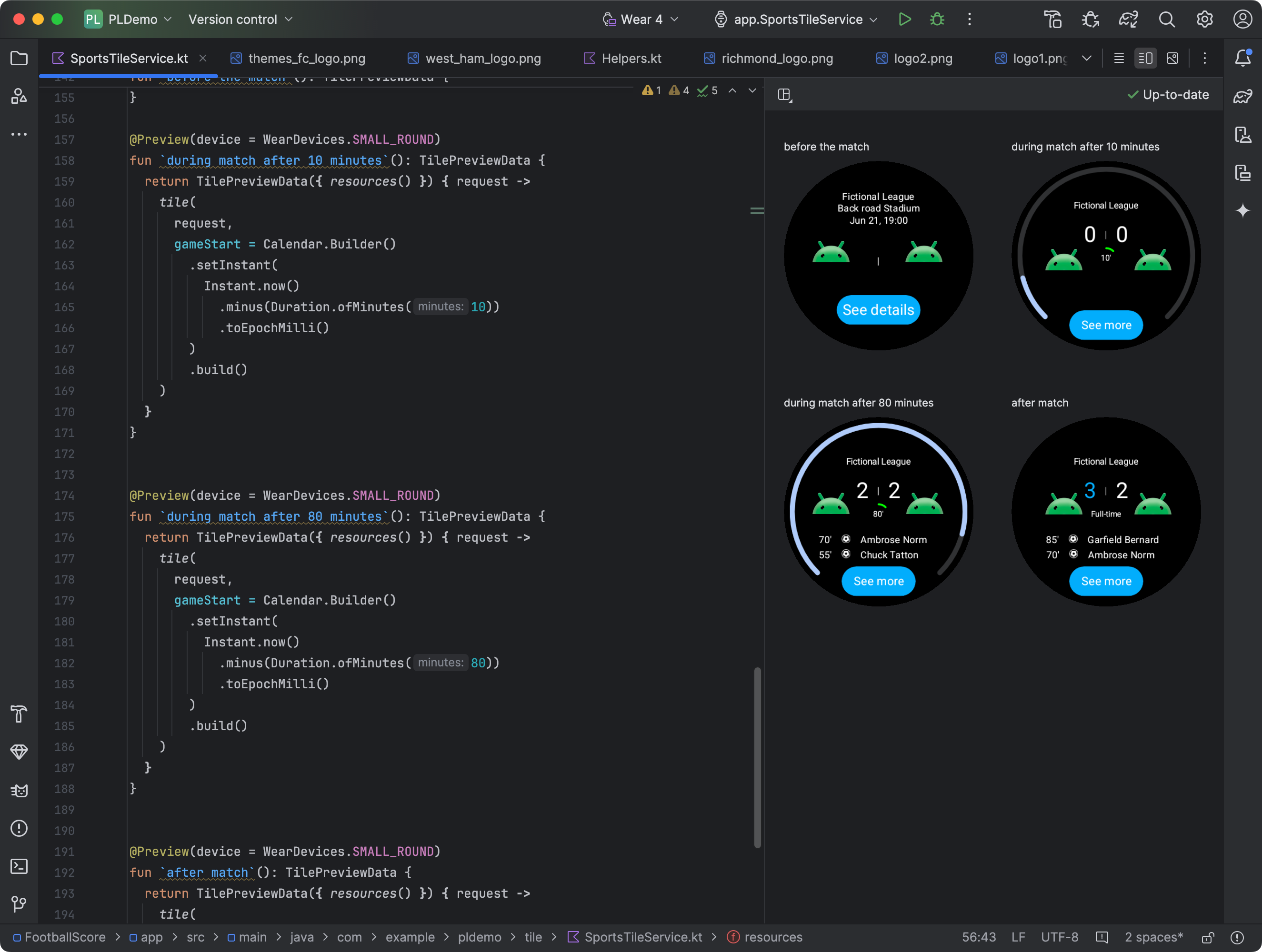
By including several dependencies on version 1.4 of the Jetpack Tiles library, you can view snapshots of your Wear OS app's tiles. This preview panel is particularly useful if your tile's appearance changes in response to conditions, such as different content depending on the device's display size, or a sports event reaching halftime.
Compose Glance widget previews
Android Studio Koala Feature Drop makes it easy to preview your Jetpack Compose Glance widgets directly within the IDE. Catch potential UI issues and fine-tune your widget's appearance early in the development process. To get started follow these steps:
- Add the dependencies.
- Add the dependencies to your version catalog:
[versions] androidx-glance-preview = "1.1.0-rc01" [libraries] androidx-glance-preview = { group = "androidx.glance", name = "glance-preview", version.ref = "androidx-glance-preview" } androidx-glance-appwidget-preview = { group = "androidx.glance", name = "glance-appwidget-preview", version.ref = "androidx-glance-preview" }
- Add the dependencies to your app-level
build.gradle.kts
file:debugImplementation(libs.androidx.glance.preview) debugImplementation(libs.androidx.glance.appwidget.preview)
- Add the dependencies to your version catalog:
- Import the dependencies in the file where you have Glance UI:
import androidx.glance.preview.ExperimentalGlancePreviewApi import androidx.glance.preview.Preview
- Create a preview of your Glance widget:
@Composable fun MyGlanceContent() { GlanceTheme { Scaffold( backgroundColor = GlanceTheme.colors.widgetBackground, titleBar = { … }, ) { … } } } @OptIn(ExperimentalGlancePreviewApi::class) @Preview(widthDp = 172, heightDp = 244) @Composable fun MyGlancePreview() { MyGlanceContent() }
Live Edit for Compose enabled by default and new shortcut
Live Edit is now enabled in manual mode by default. It has increased stability and more robust change detection, including support for import statements.
Note that starting with Android Studio Koala Feature Drop Beta 1, the default shortcut to push your changes in manual mode has been updated to Command+'. You can still customize it on the Keymap settings page.
Kotlin support for test fixtures in Android Gradle plugin
Starting with Android Gradle plugin 8.5.0-beta01, you can now use Kotlin in your
testFixtures
sources. Previously, testFixtures
sources only supported Java.
To use this feature, do the following.
- Ensure you're using Kotlin version 1.9.20 or higher.
- Add
android.experimental.enableTestFixturesKotlinSupport=true
to yourgradle.properties
file. Add an explicit dependency on the Kotlin standard library in your module's
build.gradle.kts
orbuild.gradle
file:dependencies { testFixturesImplementation("org.jetbrains.kotlin:kotlin-stdlib:1.9.20") }
Known limitations: There is no KAPT or KSP support for test fixtures yet.
Android Studio Ladybug | 2024.1.3
The following are new features in Android Studio Ladybug | 2024.1.3. To see what's been fixed in this version of Android Studio, see the closed issues.
Code suggestions with Gemini in Android Studio
You can now provide custom prompts for Gemini in Android Studio to generate code suggestions:
- Enable Gemini by clicking View > Tool Windows > Gemini.
- To see the prompt field, right-click in the code editor and select Gemini > Transform selected code from the context menu.
- Prompt Gemini to generate a code suggestion that either adds new code or transforms selected code. Ask Gemini to simplify complex code by rewriting it, perform very specific code transformations such as "make this code idiomatic," or generate new functions you describe. Android Studio then shows you Gemini's code suggestion as a code diff, so you can review and accept only the suggestions you want.
Analyze crash reports with Gemini in Android Studio
Use Gemini in Android Studio to analyze your App Quality Insights crash reports, generate insights, provide a crash summary, and when possible recommend next steps, including sample code and links to relevant documentation.
Generate all of this information by clicking Show Insights in the App Quality Insights tool window in Android Studio after you enable Gemini from View > Tool Windows > Gemini.
Google Play SDK Index integration
The Android Studio Google Play SDK Index integration now includes warnings from the Google Play SDK Console. This gives you a complete view of any potential version or policy issues in your dependencies before submitting your app to the Google Play Console.
Android Studio now also displays notes from SDK authors directly in the editor to save you time.
Mock sensor capabilities and values
Android Studio now includes a new sensor panel, which lets you simulate a device having or not having specific sensor capabilities, such as a heart rate sensor, as well as set specific test values for these sensors. Use this panel to test how your app handles devices that have different sensor capabilities. This panel is useful for testing health and fitness apps, especially on Wear OS devices.
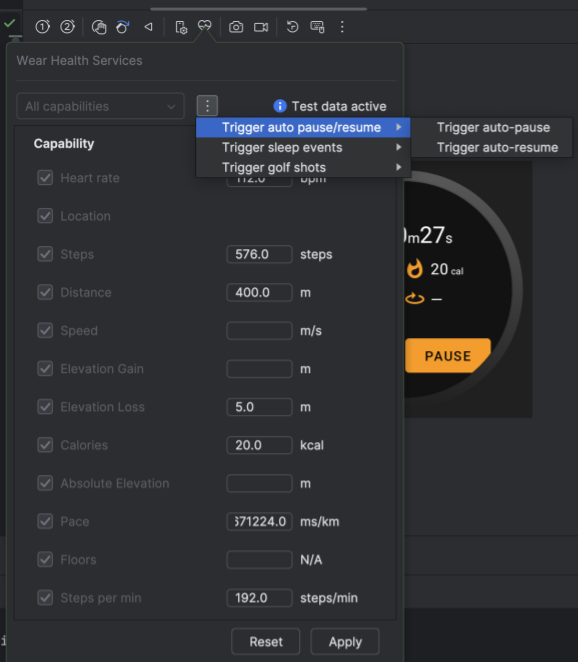
To open and use the panel, do the following:
- Create or open an Android Virtual Device (AVD) and run your app on the emulator.
- In the emulator panel, select Wear Health Services.
The Wear Health Services panel opens, showing a list of sensors that are available on different Android-powered devices.
After the panel opens, you can do the following:
- Toggle among Standard capabilities, All capabilities (default), or Custom. Select Apply to send the current list of capabilities to the emulated device, and select Reset to restore the list of capabilities to their default on-off values.
- Trigger different user events after you select the Trigger events drop-down button. From here, you can Trigger auto pause/resume of fitness activities, Trigger sleep events by the user, and Trigger golf shots that the user takes on a golf course or mini-golf course.
- Override sensor values, after you begin an exercise in an app that's installed on the emulator. After you enter new values for different exercise metrics, select Apply to sync these values with the emulator. This is useful for testing how your app handles different exercise conditions and users' fitness tendencies.
Compose Preview Screenshot Testing tool
Use the Compose Preview Screenshot Testing tool to test your Compose UIs and prevent regressions. The new tool helps you generate HTML reports that let you visually detect any changes to your app's UI. Learn more at Compose Preview Screenshot Testing.