테스트는 앱 개발 프로세스에서 빼놓을 수 없는 부분입니다. 일반적으로 에뮬레이터나 기기에서 앱을 실행하여 코드가 예상대로 작동하는지 수동으로 확인합니다. 하지만 수동 테스트는 시간이 오래 걸리고 오류가 발생하기 쉬우며, 다양한 크기의 화면과 기기에서 실행되는 앱의 경우 관리하기 어려운 경우가 많습니다. 수동 테스트의 문제는 개발에 단일 기기를 사용하는 경우가 많습니다. 따라서 폼 팩터가 다른 다른 기기에서는 오류가 감지되지 않을 수 있습니다.
다양한 창 및 화면 크기에서 회귀를 식별하려면 자동 테스트를 구현하여 앱의 동작과 모양이 다양한 폼 팩터에서 일관적인지 확인합니다. 자동 테스트를 사용하면 문제를 조기에 식별하여 사용자 환경에 영향을 미치는 문제의 위험을 완화할 수 있습니다.
테스트 대상
다양한 화면과 창 크기에 맞게 만들어진 UI를 개발할 때는 다음 두 가지 측면에 특히 유의하세요.
- 크기가 다른 창에서 구성요소와 레이아웃의 시각적 속성이 어떻게 다른지
- 구성 변경 시 상태가 유지되는 방식
시각적 속성
다양한 창 크기에 맞게 UI를 맞춤설정하는지 여부와 관계없이 UI가 올바르게 표시되는지 확인해야 합니다. 소형, 중형, 확장형인 너비와 높이를 고려하세요. 권장 중단점은 창 크기 클래스를 참고하세요.
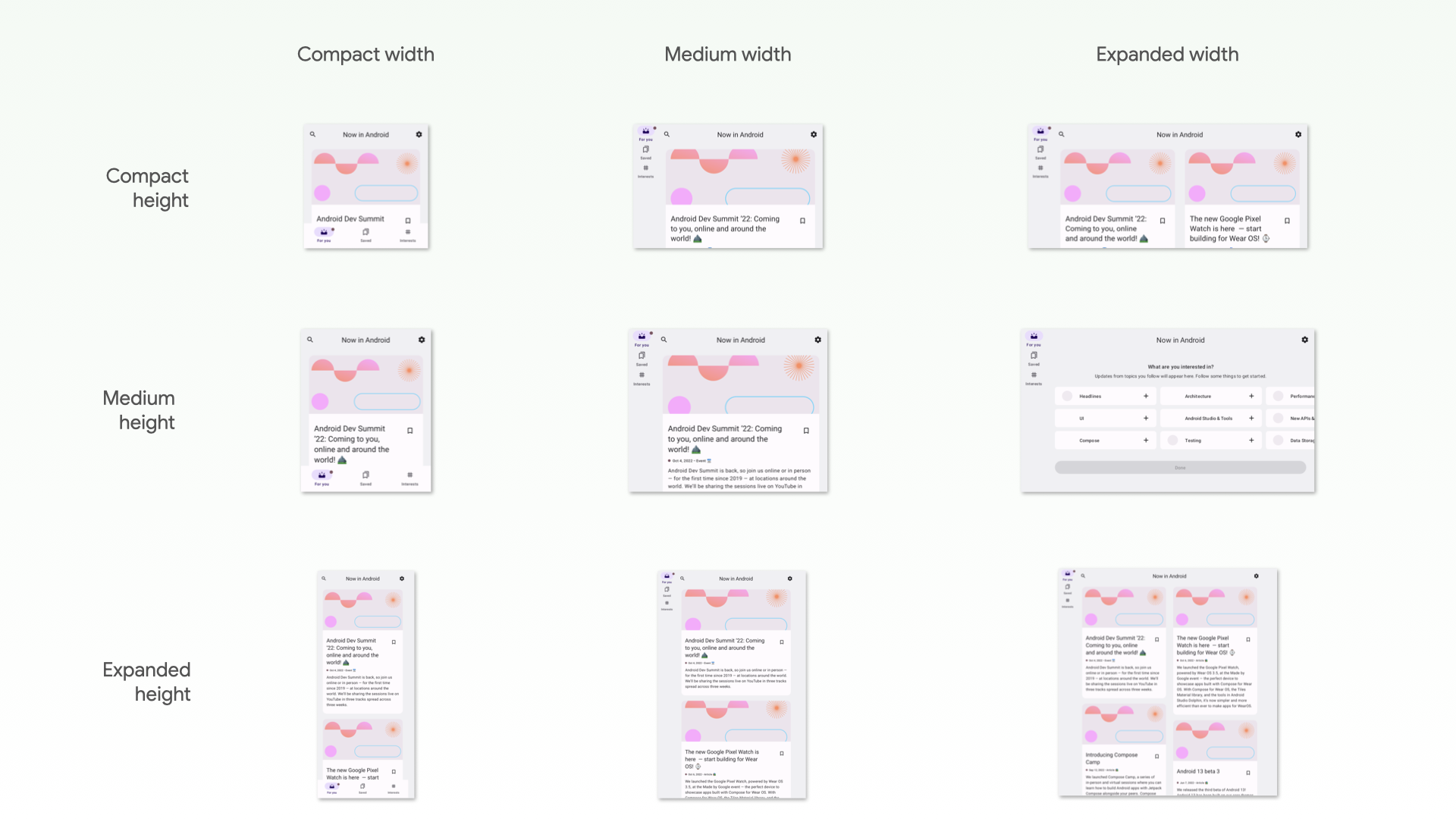
또한 크기 제약 조건이 늘어나면 앱이 디자인 시스템의 일부 구성요소를 예상대로 렌더링하지 않을 수 있습니다.
앱에 다양한 창 크기에 맞는 적응형 레이아웃이 있는 경우 회귀를 방지하기 위한 자동화된 테스트가 있어야 합니다. 예를 들어 휴대전화에서 여백을 수정하면 태블릿에서 레이아웃 불일치가 발생할 수 있습니다. UI 테스트를 만들어 레이아웃과 구성요소의 동작을 확인하거나 스크린샷 테스트를 구성하여 레이아웃을 시각적으로 확인합니다.
상태 복원
태블릿과 같은 기기에서 실행되는 앱은 휴대전화의 앱보다 훨씬 더 자주 회전되고 크기가 조절됩니다. 또한 폴더블은 접기 및 펼치기와 같이 구성 변경을 트리거할 수 있는 새로운 디스플레이 기능을 도입합니다. 앱은 이러한 구성 변경이 발생할 때 상태를 복원할 수 있어야 합니다. 그런 다음 앱이 상태를 올바르게 복원하는지 확인하는 테스트도 작성해야 합니다.
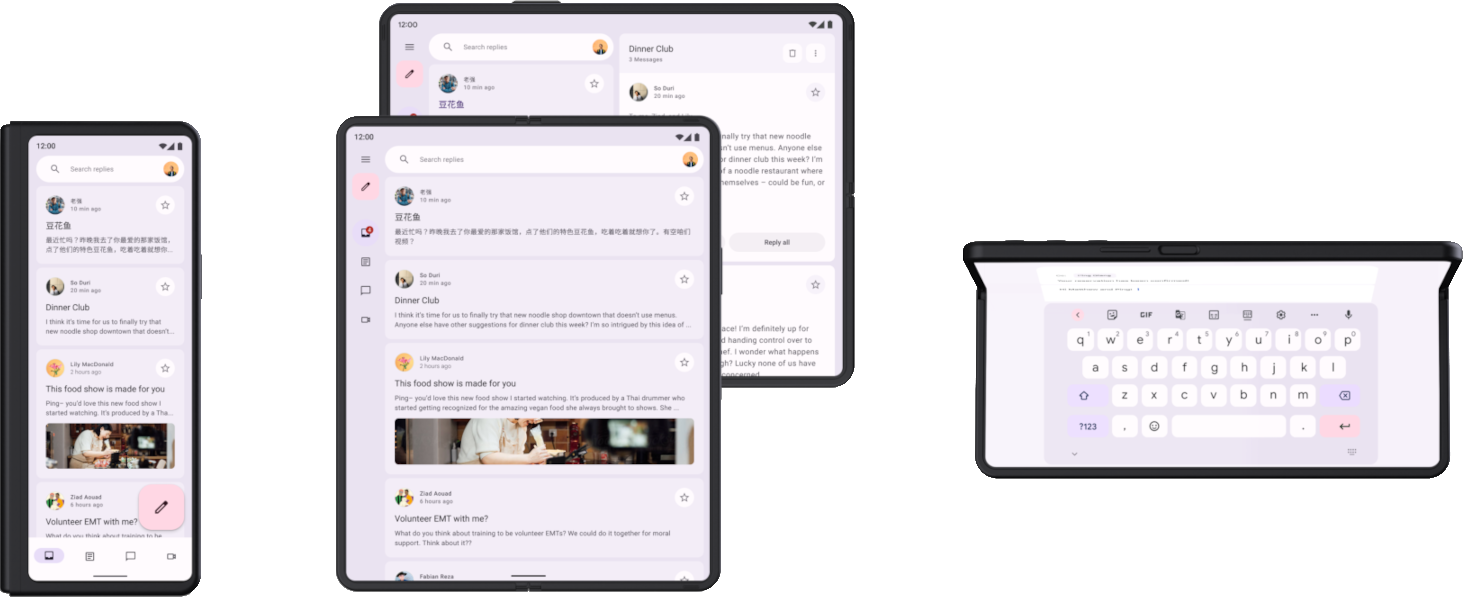
먼저 구성 변경이 발생할 때 앱이 비정상 종료되지 않는지 테스트합니다. 앱의 모든 UI가 회전, 크기 조절, 접기의 조합을 처리할 수 있는지 확인합니다. 기본적으로 구성 변경은 활동을 다시 생성하므로 활동 지속성 가정으로 인해 일부 비정상 종료가 발생합니다.
구성 변경을 테스트하는 방법에는 여러 가지가 있지만 대부분의 경우 다음 두 가지 방법으로 테스트할 수 있습니다.
- Compose에서
StateRestorationTester
를 사용하여 활동을 다시 시작하지 않고도 효율적인 방식으로 구성 변경을 시뮬레이션합니다. 자세한 내용은 다음 섹션을 참고하세요. - Espresso 또는 Compose와 같은 UI 테스트에서
Activity.recreate()
를 호출하여 구성 변경을 시뮬레이션합니다.
일반적으로 구성 변경에 대한 응답으로 상태 복원을 테스트하기 위해 다른 기기를 사용할 필요는 없습니다. 활동을 다시 만드는 모든 구성 변경사항이 비슷한 영향을 미치기 때문입니다. 그러나 일부 구성 변경사항은 특정 기기에서 다른 상태 복원 메커니즘을 트리거할 수 있습니다.
예를 들어 사용자가 펼쳐진 폴더블에서 목록 세부정보 UI를 보고 있는데 기기를 접어 전면 디스플레이로 전환하면 일반적으로 UI가 세부정보 페이지로 전환됩니다. 자동 테스트는 탐색 상태를 비롯한 UI 상태의 복원을 다루어야 합니다.
한 디스플레이에서 다른 디스플레이로 전환하거나 멀티 윈도우 모드로 전환할 때 기기에서 발생하는 구성 변경사항을 테스트하려면 다음과 같은 여러 옵션이 있습니다.
- 테스트 중에 기기를 사용하여 화면 크기를 조절합니다. 대부분의 경우 이렇게 하면 확인해야 하는 모든 상태 복원 메커니즘이 트리거됩니다. 그러나 상태 변경이 구성 변경을 트리거하지 않으므로 폴더블에서 특정 상태를 감지하는 로직에는 이 테스트가 작동하지 않습니다.
- 테스트하려는 기능을 지원하는 기기 또는 에뮬레이터를 사용하여 관련 구성 변경사항을 트리거합니다. 예를 들어 폴더블 또는 태블릿은 Espresso Device를 사용하여 접힌 상태에서 평평하게 펼쳐지도록 제어할 수 있습니다. 예시는 다양한 화면 크기를 테스트하는 라이브러리 및 도구의 Espresso 기기 섹션을 참고하세요.
다양한 화면 및 창 크기에 대한 테스트 유형
각 사용 사례에 적절한 유형의 테스트를 사용하여 다양한 폼 팩터에서 테스트가 올바르게 작동하는지 확인합니다.
UI 동작 테스트는 앱 UI의 일부를 실행합니다(예: 활동 표시). 테스트는 특정 요소가 존재하거나 특정 속성을 보유하는지 확인합니다 . 테스트는 선택적으로 사용자 작업을 시뮬레이션할 수 있습니다. 뷰의 경우 Espresso를 사용하세요. Jetpack Compose에는 자체 테스트 API가 있습니다. UI 동작 테스트는 계측 또는 로컬일 수 있습니다. 계측 테스트는 기기나 에뮬레이터에서 실행되는 반면 로컬 UI 테스트는 JVM의 Robolectric에서 실행됩니다.
UI 동작 테스트를 사용하여 앱의 탐색 구현이 올바른지 확인합니다. 테스트는 클릭 및 스와이프와 같은 작업을 실행합니다. UI 동작 테스트는 특정 요소 또는 속성의 존재 여부도 확인합니다. 자세한 내용은 UI 테스트 자동화를 참고하세요.
스크린샷 테스트: UI 또는 구성요소의 스크린샷을 찍고 이미지를 이전에 승인된 스크린샷과 비교합니다. 단일 스크린샷으로 많은 수의 요소와 시각적 속성을 다룰 수 있으므로 회귀를 방지하는 데 매우 효과적인 방법입니다. JVM 또는 기기에서 스크린샷 테스트를 실행할 수 있습니다. 사용 가능한 스크린샷 테스트 프레임워크는 여러 가지가 있습니다. 자세한 내용은 스크린샷 테스트를 참고하세요.
마지막으로 기기 유형이나 창 크기에 따라 다르게 동작하는 로직 단위의 기능을 테스트하려면 단위 테스트가 필요할 수 있지만 이 영역에서는 단위 테스트가 흔하지 않습니다.
다음 단계
이 문서에 포함된 검사를 구현하는 방법에 관한 자세한 내용은 라이브러리 및 도구를 참고하세요.