Les tests s'inscrivent dans le processus de développement d'une application. Vous exécutez généralement des applications sur un émulateur ou un appareil pour vérifier manuellement que votre code fonctionne comme prévu. Cependant, les tests manuels sont longs, sujets à des erreurs et souvent difficiles à gérer pour les applications exécutées sur des écrans et des appareils de différentes tailles. Les problèmes liés aux tests manuels sont le plus souvent le résultat de l'utilisation d'un seul appareil pour le développement. Par conséquent, les erreurs peuvent passer inaperçues sur d'autres appareils avec des facteurs de forme différents.
Pour identifier les régressions sur différentes tailles de fenêtre et d'écran, implémentez des tests automatisés afin de vérifier que le comportement et l'apparence de votre application sont cohérents sur différents facteurs de forme. Les tests automatisés identifient les problèmes dès le départ, ce qui réduit le risque qu'ils affectent l'expérience utilisateur.
Que faut-il tester ?
Lorsque vous développez des UI conçues pour différentes tailles d'écran et de fenêtre, accordez une attention particulière à deux aspects:
- Comment les attributs visuels des composants et des mises en page sont différents sur les fenêtres de différentes tailles
- Comment l'état est conservé lors des modifications de configuration
Attributs visuels
Que vous personnalisiez les UI pour différentes tailles de fenêtre ou non, vous devez vérifier qu'elles s'affichent correctement. Tenez compte des largeurs et hauteurs compactes, moyennes et étendues. Consultez la section Classes de taille de fenêtre pour connaître les points d'arrêt recommandés.
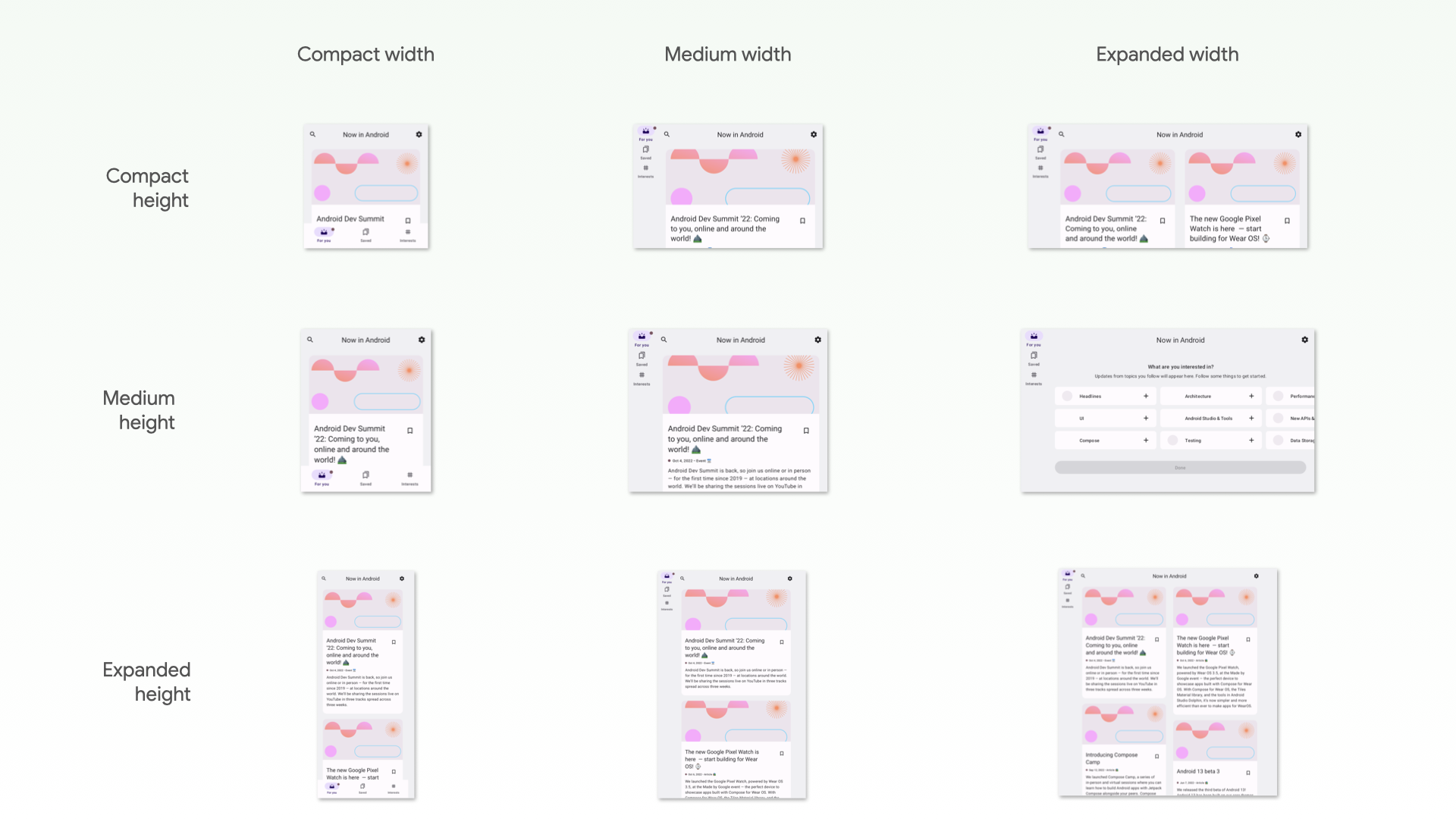
De plus, il est possible que votre application n'affiche pas certains composants de votre système de conception comme prévu lorsque leurs contraintes de taille sont étirées.
Si votre application comporte des mises en page adaptatives pour différentes tailles de fenêtre, vous devez effectuer des tests automatisés pour éviter les régressions. Par exemple, fixer une marge sur un téléphone peut entraîner des incohérences de mise en page sur une tablette. Créez des tests d'interface utilisateur pour vérifier le comportement de vos mises en page et composants, ou créez des tests de capture d'écran pour vérifier les mises en page visuellement.
Restauration de l'état
Les applications exécutées sur des appareils tels que les tablettes sont pivotées et redimensionnées beaucoup plus fréquemment que les applications sur les téléphones. De plus, les appareils pliables introduisent de nouvelles fonctionnalités d'affichage, telles que le pliage et le dépliage, qui peuvent déclencher des modifications de configuration. Votre application doit pouvoir restaurer l'état lorsque ces modifications de configuration se produisent. Vous devez également écrire des tests qui confirment que votre application restaure l'état correctement.
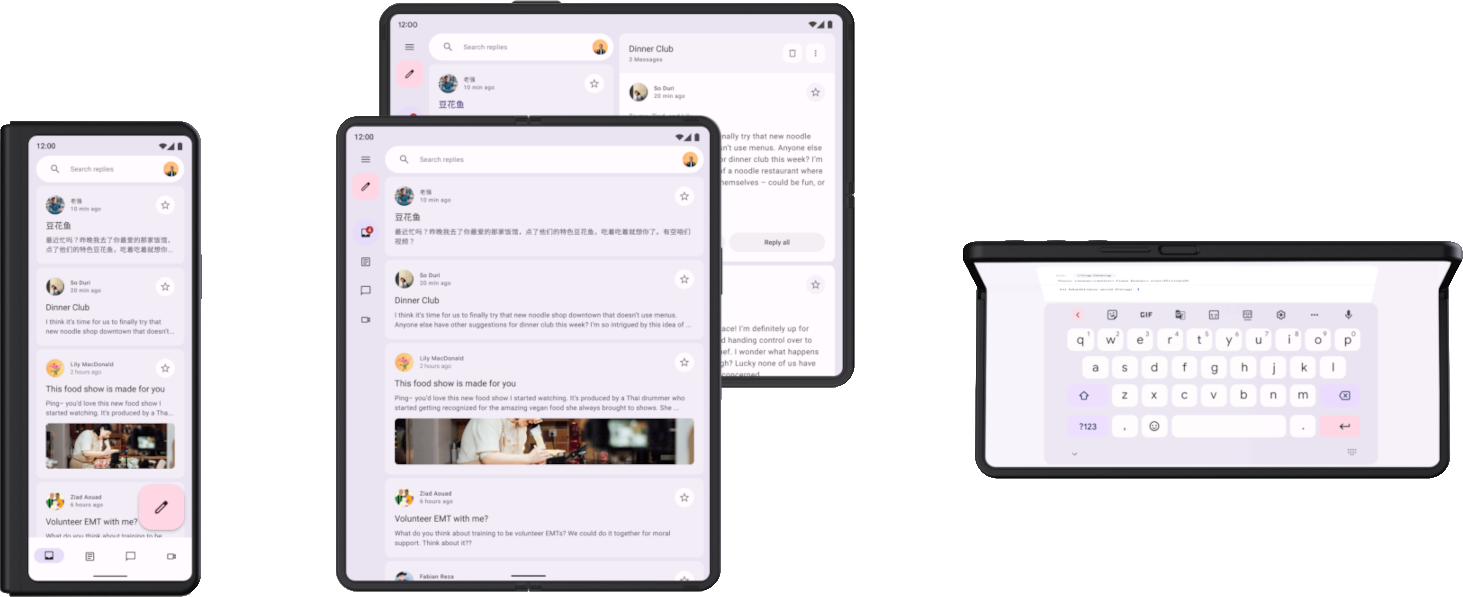
Commencez par vérifier que votre application ne plante pas lorsque la configuration change. Assurez-vous que chaque UI de votre application peut gérer n'importe quelle combinaison de rotation, de redimensionnement ou de pliage. Étant donné que les modifications de configuration recréent l'activité par défaut, certains plantages se produisent en raison d'hypothèses de persistance de l'activité.
Il existe plusieurs façons de tester les modifications de configuration, mais dans la plupart des cas, il existe deux méthodes:
- Dans Compose, utilisez
StateRestorationTester
pour simuler un changement de configuration de manière efficace sans redémarrer l'activité. Pour en savoir plus, consultez les sections suivantes. - Dans tout test d'interface utilisateur tel qu'Espresso ou Compose, simulez un changement de configuration en appelant
Activity.recreate()
.
En règle générale, vous n'avez pas besoin d'utiliser différents appareils pour tester la restauration de l'état en réponse aux modifications de configuration. En effet, toutes les modifications de configuration qui recréent l'activité ont des répercussions similaires. Toutefois, certaines modifications de configuration peuvent déclencher différents mécanismes de restauration d'état sur des appareils spécifiques.
Par exemple, lorsqu'un utilisateur consulte une UI Liste/Détail sur un appareil pliable ouvert et qu'il plie l'appareil pour passer à l'écran avant, l'UI passe généralement à la page d'informations. Un test automatisé doit couvrir cette restauration de l'état de l'UI, y compris l'état de navigation.
Pour tester les modifications de configuration qui se produisent sur les appareils qui passent d'un écran à un autre ou qui passent en mode multifenêtre, plusieurs options s'offrent à vous:
- Sur n'importe quel appareil, redimensionnez l'écran pendant un test. Dans la plupart des cas, cela déclenche tous les mécanismes de restauration d'état que vous devez vérifier. Toutefois, ce test ne fonctionnera pas pour la logique qui détecte des postures spécifiques dans les appareils pliables, car les changements de posture ne déclenchent pas de modification de configuration.
- À l'aide d'un appareil ou d'un émulateur compatible avec les fonctionnalités que vous souhaitez tester, déclenchez les modifications de configuration associées. Par exemple, un appareil pliable ou une tablette peuvent être contrôlés à l'aide d'Espresso Device pour passer du mode plié au mode ouvert en mode paysage. Pour voir des exemples, consultez la section Appareil Espresso de la page Bibliothèques et outils pour tester différentes tailles d'écran.
Types de tests pour différentes tailles d'écran et de fenêtre
Utilisez le type de test approprié pour chaque cas d'utilisation afin de vérifier qu'il fonctionne correctement sur différents facteurs de forme:
Les tests de comportement de l'UI lancent une partie de l'UI de l'application, comme l'affichage d'une activité. Les tests vérifient que certains éléments existent ou qu'ils possèdent des attributs spécifiques . Les tests peuvent éventuellement effectuer des actions utilisateur simulées. Pour les vues, utilisez Espresso. Jetpack Compose dispose de ses propres API de test. Les tests de comportement de l'interface utilisateur peuvent être instrumentés ou locaux. Les tests d'instrumentation s'exécutent sur des appareils ou des émulateurs, tandis que les tests d'UI locaux s'exécutent sur Robolectric sur la JVM.
Utilisez des tests de comportement de l'UI pour vérifier que l'implémentation de la navigation d'une application est correcte. Les tests effectuent des actions telles que des clics et des balayages. Les tests de comportement de l'UI vérifient également l'existence de certains éléments ou propriétés. Pour en savoir plus, consultez la section Automatiser les tests de l'interface utilisateur.
Les tests de capture d'écran permettent de prendre une capture d'écran d'une UI ou d'un composant, puis de comparer l'image à une capture d'écran précédemment approuvée. Il s'agit d'un moyen très efficace de se protéger contre les régressions, car une seule capture d'écran peut couvrir un grand nombre d'éléments et leurs propriétés visuelles. Vous pouvez exécuter des tests de capture d'écran sur la JVM ou sur des appareils. Plusieurs frameworks de test de captures d'écran sont disponibles. Pour en savoir plus, consultez les tests de captures d'écran.
Enfin, vous devrez peut-être effectuer des tests unitaires pour tester le fonctionnement des unités de logique qui se comportent différemment selon le type d'appareil ou la taille de la fenêtre, mais les tests unitaires sont moins courants dans ce domaine.
Étapes suivantes
Pour en savoir plus sur l'implémentation des vérifications contenues dans ce document, consultez la section Bibliothèques et outils.