Wear OS 中的應用程式使用的版面配置技巧與其他 Android 裝置相同,但必須根據特定手錶的限制來設計。
注意:請勿將整個功能和 UI 從行動應用程式轉移至 Wear OS,這麼做無法帶來良好的使用者體驗。
如果您將應用程式設計為矩形手持裝置,則圓形手錶上螢幕角落的內容可能會遭到裁剪。如果您使用可捲動的垂直清單,那麼受到的影響可能會較小,因為使用者能夠捲動畫面將內容置中。不過,如果是單螢幕畫面,可能會導致使用者體驗不佳。
如果您為版面配置使用下列設定,文字會在圓形螢幕的裝置上會無法正確顯示:
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:id="@+id/text" android:layout_width="0dp" android:layout_height="0dp" android:text="@string/very_long_hello_world" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
如要解決這個問題,請使用支援圓形裝置的 Wear OS UI 程式庫中的版面配置。
- 您可以使用
BoxInsetLayout
,避免裁剪到圓形螢幕邊緣附近的畫面。 - 如果您想要顯示並操控針對圓形螢幕最佳化的項目垂直清單,可以使用
WearableRecyclerView
建立曲線版面配置。
如要進一步瞭解如何設計應用程式,請參閱「Wear OS 設計指南」。
使用 BoxInsetLayout
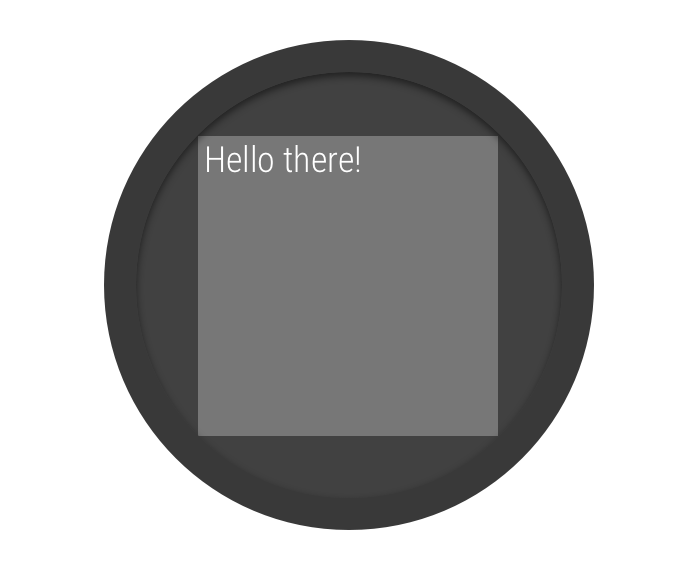
圖 2. 圓形螢幕上的視窗插邊。
Wear OS UI 程式庫中的
BoxInsetLayout
類別可讓您定義適用於圓形螢幕的版面配置。這個類別可讓您輕鬆對齊中央或螢幕邊緣附近的檢視畫面。
圖 2 中的灰色正方形會顯示在套用必要的視窗插邊後,BoxInsetLayout
可將子檢視畫面自動顯示在圓形螢幕上的區域。為了要在這個區域中顯示,子檢視畫面會使用下列值指定 layout_boxedEdges
屬性:
top
、bottom
、left
和right
的組合。舉例來說,"left|top"
值會將子項的左側和上方邊緣置於圖 2 的灰色正方形中。"all"
值會將所有子項內容置於圖 2 的灰色正方形中。這是最常見的ConstraintLayout
方法。
圖 2 所示的版面配置使用 <BoxInsetLayout>
元素,並適用於圓形螢幕:
<androidx.wear.widget.BoxInsetLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_height="match_parent" android:layout_width="match_parent" android:padding="15dp"> <androidx.constraintlayout.widget.ConstraintLayout android:layout_width="match_parent" android:layout_height="match_parent" android:padding="5dp" app:layout_boxedEdges="all"> <TextView android:layout_height="wrap_content" android:layout_width="match_parent" android:text="@string/sometext" android:textAlignment="center" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <ImageButton android:background="@android:color/transparent" android:layout_height="50dp" android:layout_width="50dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintStart_toStartOf="parent" android:src="@drawable/cancel" /> <ImageButton android:background="@android:color/transparent" android:layout_height="50dp" android:layout_width="50dp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" android:src="@drawable/ok" /> </androidx.constraintlayout.widget.ConstraintLayout> </androidx.wear.widget.BoxInsetLayout>
請注意,版面配置的部分會以粗體標示:
-
android:padding="15dp"
此行會將邊框間距指派給
<BoxInsetLayout>
元素。 -
android:padding="5dp"
此行會將邊框間距指派給內部
ConstraintLayout
元素。 -
app:layout_boxedEdges="all"
此行可確保
ConstraintLayout
元素及其子項在圓形螢幕上的視窗插邊定義區域內。
使用曲線版面配置
您可以透過 Wear OS UI 程式庫中的
WearableRecyclerView
類別,選擇採用針對圓形螢幕進行最佳化調整的曲線版面配置。如要為應用程式中的可捲動清單啟用曲線版面配置,請參閱「在 Wear OS 上建立清單」。