รายการช่วยให้ผู้ใช้เลือกรายการจากชุดตัวเลือกได้อย่างง่ายดายในอุปกรณ์ Wear OS
ไลบรารี UI อุปกรณ์ที่สวมใส่ได้ประกอบด้วย
WearableRecyclerView
ซึ่งเป็น
RecyclerView
ในการสร้างรายการที่เพิ่มประสิทธิภาพสำหรับอุปกรณ์ที่สวมใส่ได้ คุณใช้
ในแอปที่สวมใส่ได้โดยการสร้างคอนเทนเนอร์ WearableRecyclerView
ใหม่
ใช้ WearableRecyclerView
สำหรับ
รายการง่ายๆ ที่มีมากมาย เช่น ตัวเรียกใช้งานแอปพลิเคชัน หรือรายชื่อติดต่อ แต่ละรายการอาจ
มีสตริงสั้นๆ และไอคอนที่เกี่ยวข้อง หรือแต่ละรายการอาจมีเฉพาะสตริง
หรือไอคอน
หมายเหตุ: หลีกเลี่ยงเลย์เอาต์ที่ซับซ้อน ผู้ใช้ควรแค่มองไปที่รายการเพื่อ เข้าใจว่าคืออะไร โดยเฉพาะกับอุปกรณ์ที่สวมใส่ได้" ขนาดหน้าจอที่จำกัด
เมื่อขยายชั้นเรียน RecyclerView
ที่มีอยู่แล้ว WearableRecyclerView
โดยค่าเริ่มต้น API จะแสดงรายการแบบเลื่อนได้ในแนวตั้งแบบเป็นแนวตรง คุณยังสามารถใช้
WearableRecyclerView
API สำหรับเลือกใช้การจัดวางแบบโค้ง
ท่าทางสัมผัสการเลื่อนเป็นวงกลม
ในแอปที่สวมใส่ได้
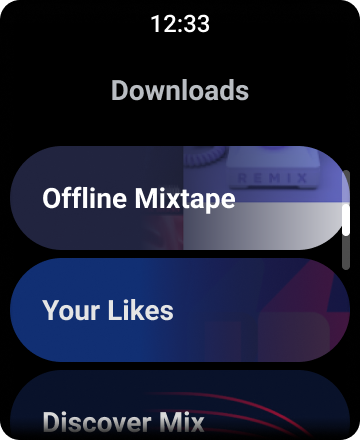
รูปที่ 1 มุมมองรายการเริ่มต้นใน Wear OS
คู่มือนี้จะแสดงวิธีใช้ชั้นเรียน WearableRecyclerView
เพื่อสร้าง
รายการในแอป Wear OS, วิธีเลือกใช้เลย์เอาต์แบบโค้ง
ของรายการที่เลื่อนได้ และวิธีปรับแต่งลักษณะของ
เด็กๆ ขณะเลื่อนหน้าจอ
เพิ่ม WearableRecyclerView ในกิจกรรมโดยใช้ XML
เลย์เอาต์ต่อไปนี้จะเพิ่ม WearableRecyclerView
ลงในกิจกรรม
<androidx.wear.widget.WearableRecyclerView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/recycler_launcher_view" android:layout_width="match_parent" android:layout_height="match_parent" android:scrollbars="vertical" />
ตัวอย่างต่อไปนี้แสดง WearableRecyclerView
ใช้กับกิจกรรม:
Kotlin
class MainActivity : Activity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) setContentView(R.layout.activity_main) } ... }
Java
public class MainActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } ... }
สร้างเลย์เอาต์แบบโค้ง
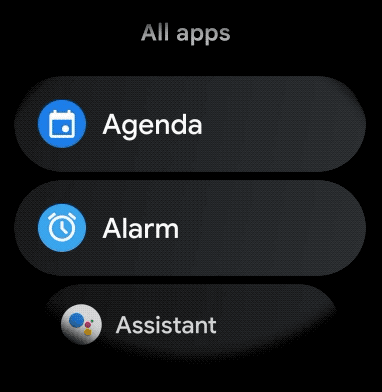
วิธีสร้างเลย์เอาต์แบบโค้งสำหรับรายการที่เลื่อนได้ในแอปอุปกรณ์ที่สวมใส่ได้
-
ใช้
WearableRecyclerView
เป็นคอนเทนเนอร์หลักในเลย์เอาต์ XML ที่เกี่ยวข้อง -
ตั้งค่า
setEdgeItemsCenteringEnabled(boolean)
ไปยังtrue
ช่วงเวลานี้ จัดวางรายการแรกและรายการสุดท้ายบนหน้าจอให้อยู่ในแนวตั้ง -
ใช้เมธอด
WearableRecyclerView.setLayoutManager()
เพื่อตั้งค่าเลย์เอาต์ของ รายการบนหน้าจอ
Kotlin
wearableRecyclerView.apply { // To align the edge children (first and last) with the center of the screen. isEdgeItemsCenteringEnabled = true ... layoutManager = WearableLinearLayoutManager(this@MainActivity) }
Java
// To align the edge children (first and last) with the center of the screen. wearableRecyclerView.setEdgeItemsCenteringEnabled(true); ... wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this));
หากแอปมีข้อกำหนดที่เฉพาะเจาะจงในการปรับแต่งรูปลักษณ์ของเด็กขณะเลื่อนดู เช่น
การปรับขนาดไอคอนและข้อความขณะที่รายการเลื่อนออกจากกึ่งกลาง - ขยาย
WearableLinearLayoutManager.LayoutCallback
และลบล้าง
onLayoutFinished
ข้อมูลโค้ดต่อไปนี้แสดงตัวอย่างของการปรับแต่งการเลื่อนรายการเพื่อปรับขนาด
ออกห่างจากศูนย์กลางโดยขยาย
WearableLinearLayoutManager.LayoutCallback
ชั้นเรียน:
Kotlin
/** How much icons should scale, at most. */ private const val MAX_ICON_PROGRESS = 0.65f class CustomScrollingLayoutCallback : WearableLinearLayoutManager.LayoutCallback() { private var progressToCenter: Float = 0f override fun onLayoutFinished(child: View, parent: RecyclerView) { child.apply { // Figure out % progress from top to bottom. val centerOffset = height.toFloat() / 2.0f / parent.height.toFloat() val yRelativeToCenterOffset = y / parent.height + centerOffset // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset) // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS) scaleX = 1 - progressToCenter scaleY = 1 - progressToCenter } } }
Java
public class CustomScrollingLayoutCallback extends WearableLinearLayoutManager.LayoutCallback { /** How much icons should scale, at most. */ private static final float MAX_ICON_PROGRESS = 0.65f; private float progressToCenter; @Override public void onLayoutFinished(View child, RecyclerView parent) { // Figure out % progress from top to bottom. float centerOffset = ((float) child.getHeight() / 2.0f) / (float) parent.getHeight(); float yRelativeToCenterOffset = (child.getY() / parent.getHeight()) + centerOffset; // Normalize for center. progressToCenter = Math.abs(0.5f - yRelativeToCenterOffset); // Adjust to the maximum scale. progressToCenter = Math.min(progressToCenter, MAX_ICON_PROGRESS); child.setScaleX(1 - progressToCenter); child.setScaleY(1 - progressToCenter); } }
Kotlin
wearableRecyclerView.layoutManager = WearableLinearLayoutManager(this, CustomScrollingLayoutCallback())
Java
CustomScrollingLayoutCallback customScrollingLayoutCallback = new CustomScrollingLayoutCallback(); wearableRecyclerView.setLayoutManager( new WearableLinearLayoutManager(this, customScrollingLayoutCallback));