Thiết bị TV cần có thiết bị phần cứng phụ để tương tác với ứng dụng — đây là một bộ điều khiển từ xa hoặc tay điều khiển trò chơi. Do đó, ứng dụng của bạn phải hỗ trợ phương thức nhập bằng bàn phím di chuyển (D-pad). Ngoài ra, ứng dụng của bạn có thể cần phải xử lý các bộ điều khiển có kết nối mạng và nhập dữ liệu từ nhiều bộ điều khiển loại bộ điều khiển.
Hướng dẫn này thảo luận các yêu cầu đối với việc xử lý tay điều khiển cho thiết bị TV.
Các nút điều khiển tối thiểu trên D-pad
Bộ điều khiển mặc định của thiết bị TV là D-pad. Nhìn chung, ứng dụng của bạn phải hoạt động được từ bộ điều khiển từ xa chỉ có các nút lên, xuống, trái, phải, chọn, Quay lại và Màn hình chính. Nếu ứng dụng của bạn là một trò chơi thường yêu cầu tay điều khiển trò chơi có các nút điều khiển bổ sung, cố gắng hỗ trợ chơi trò chơi bằng các nút điều khiển D-pad này. Nếu không, hãy cảnh báo người dùng rằng bạn cần có tay điều khiển để thoát khỏi trò chơi của bạn bằng tay điều khiển D-pad một cách dễ dàng.
Để biết thêm thông tin về cách điều hướng bằng bộ điều khiển D-pad cho thiết bị TV, hãy xem Điều hướng trên TV.
Xử lý việc ngắt kết nối bộ điều khiển
Bộ điều khiển cho TV thường là thiết bị Bluetooth nên có thể tìm cách tiết kiệm điện năng bằng cách định kỳ chuyển sang chế độ ngủ và ngắt kết nối khỏi thiết bị TV. Điều này có nghĩa là một ứng dụng có thể bị gián đoạn hoặc khởi động lại nếu nó không được định cấu hình để xử lý các sự kiện kết nối lại này. Các sự kiện này có thể xảy ra trong bất kỳ trường hợp nào sau đây:
- Khi video dài vài phút phát, D-pad hoặc trò chơi bộ điều khiển có thể chuyển sang chế độ ngủ, ngắt kết nối khỏi thiết bị TV rồi kết nối lại sau.
- Trong quá trình chơi, người chơi mới có thể tham gia trò chơi bằng tay điều khiển trò chơi chưa được kết nối.
- Trong khi chơi, người chơi có thể rời khỏi trò chơi và ngắt kết nối tay điều khiển trò chơi.
Mọi hoạt động trong ứng dụng TV có sự kiện ngắt kết nối và kết nối lại đều phải được định cấu hình để xử lý các sự kiện kết nối lại trong tệp kê khai ứng dụng. Mã mẫu sau đây minh hoạ cách bật một hoạt động để xử lý các thay đổi về cấu hình, bao gồm cả bàn phím hoặc thiết bị điều hướng cách kết nối, ngắt kết nối hoặc kết nối lại:
<activity android:name="com.example.android.TvActivity" android:label="@string/app_name" android:configChanges="keyboard|keyboardHidden|navigation" android:theme="@style/Theme.Leanback"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LEANBACK_LAUNCHER" /> </intent-filter> ... </activity>
Thay đổi về cấu hình này cho phép ứng dụng tiếp tục chạy thông qua một quá trình kết nối lại thay vì được khung Android khởi động lại, đây không phải là trải nghiệm người dùng tốt.
Xử lý các biến thể phương thức nhập bằng D-pad
Người dùng thiết bị TV có thể dùng nhiều loại bộ điều khiển cho TV. Cho ví dụ: người dùng có thể có cả tay điều khiển D-pad cơ bản và tay điều khiển trò chơi. Mã phím do tay điều khiển trò chơi cung cấp khi sử dụng cho các chức năng của D-pad có thể khác với phím mã do D-pad cơ bản gửi.
Xử lý các biến thể trong phương thức nhập bằng D-pad để người dùng không phải chuyển đổi bộ điều khiển để vận hành ứng dụng. Để biết thêm thông tin về cách xử lý biến thể đầu vào, hãy xem Xử lý phương thức nhập bằng bàn phím di chuyển.
Xử lý sự kiện nút
Khi người dùng nhấp vào một nút trên tay điều khiển, ứng dụng của bạn sẽ nhận được một sự kiện có
KeyEvent
. Ý định
hành vi của nút có thể là một sự kiện đa phương tiện, chẳng hạn như phát, tạm dừng hoặc dừng, hoặc đó có thể là một sự kiện trên TV, chẳng hạn như
lựa chọn hoặc điều hướng. Để mang lại trải nghiệm tốt cho người dùng, hãy chỉ định
hành vi cho các nút trên bộ điều khiển.
Sự kiện giao diện người dùng TV
Gán hành vi giao diện người dùng TV cho các nút tạo KeyEvent
như được hiển thị trong bảng sau:
KeyEvent | Hành vi |
---|---|
KEYCODE_BUTTON_B , KEYCODE_BACK | Quay lại |
KEYCODE_BUTTON_SELECT , KEYCODE_BUTTON_A , KEYCODE_ENTER
KEYCODE_NUMPAD_ENTER , KEYCODE_DPAD_CENTER | Lựa chọn |
KEYCODE_DPAD_UP , KEYCODE_DPAD_DOWN , KEYCODE_DPAD_LEFT
KEYCODE_DPAD_RIGHT | Di chuyển |
Sự kiện truyền thông
Khi người dùng đang xem nội dung nghe nhìn, hãy chỉ định hành vi cho các nút tạo
KeyEvent
loại như minh hoạ trong bảng sau. Nếu ứng dụng của bạn đang kiểm soát một
MediaSession
!
sử dụng MediaControllerAdapter
để gọi một trong những MediaControllerCompat.TransportControls
được hiển thị trong bảng. Lưu ý rằng các nút lựa chọn hoạt động như Phát hoặc Tạm dừng
trong ngữ cảnh này.
KeyEvent | Lệnh gọi TransportControls | Hành vi |
---|---|---|
BUTTON_SELECT , BUTTON_A , ENTER
KEYCODE_NUMPAD_ENTER , DPAD_CENTER |
play() | Phát |
BUTTON_START , BUTTON_SELECT , BUTTON_A
ENTER , DPAD_CENTER , KEYCODE_NUMPAD_ENTER |
pause() | Tạm dừng |
BUTTON_R1 | skipToNext() | Chuyển tới mục tiếp theo |
BUTTON_L1 | skipToPrevious() | Chuyển về mục trước |
DPAD_RIGHT , BUTTON_R2 , AXIS_RTRIGGER
AXIS_THROTTLE | fastForward() | Tua đi |
DPAD_LEFT , BUTTON_L2 , AXIS_LTRIGGER
AXIS_BRAKE | rewind() | Tua lại |
Không có thời gian | stop() | Dừng |
Lưu ý: Khi bạn sử dụng MediaSession
, không ghi đè quá trình xử lý
các nút dành riêng cho phương tiện truyền thông, chẳng hạn như
KEYCODE_MEDIA_PLAY
hoặc KEYCODE_MEDIA_PAUSE
.
Hệ thống sẽ tự động kích hoạt
MediaSession.Callback
.
Cung cấp hành vi thích hợp cho nút Quay lại
Nút Quay lại tuyệt đối không được hoạt động như một nút bật/tắt. Ví dụ: không sử dụng thanh công cụ này để mở và đóng . Chỉ sử dụng thanh này để điều hướng lùi lại theo kiểu breadcrumb (tập hợp liên kết phân cấp) thông qua các màn hình trước đó, trình phát đã được bật.
Vì nút Quay lại chỉ thực hiện việc điều hướng quay lại theo đường thẳng, nên bạn có thể sử dụng nó để lại một trình đơn trong ứng dụng được mở bởi một nút khác và quay lại ứng dụng. Việc nhấn nút Quay lại liên tục phải luôn dẫn đến màn hình chính của Android TV. Ví dụ: chơi trò chơi > màn hình tạm dừng trò chơi > màn hình chính của trò chơi > Màn hình chính của Android TV hoặc Phát chương trình truyền hình > Màn hình chính của ứng dụng TV > Màn hình chính của Android TV.
Để biết thêm thông tin về thiết kế cho mục đích điều hướng, hãy xem Thiết kế thao tác Quay lại và di chuyển lên. Để tìm hiểu về cách triển khai, hãy tham khảo Cung cấp tính năng điều hướng quay lại thích hợp.
Tay điều khiển trò chơi
Hỗ trợ các nút điều khiển trên D-pad
Lập sơ đồ điều khiển xung quanh một bộ điều khiển D-pad, vì bộ điều khiển này là mặc định cho thiết bị Android TV. Người chơi cần sử dụng được D-pad cho mọi khía cạnh của trò chơi – không chỉ điều khiển lối chơi cốt lõi mà còn điều hướng các trình đơn và quảng cáo. Vì lý do này, đảm bảo trò chơi trên Android TV không tham chiếu đến giao diện cảm ứng có ngôn ngữ như "Nhấn vào đây để tiếp tục".
Cách bạn định hình tương tác của người chơi với bộ điều khiển có thể là chìa khoá để thu hút người chơi của bạn. Hãy cân nhắc các phương pháp hay nhất sau đây:
- Truyền đạt trước các yêu cầu về tay điều khiển: sử dụng phần mô tả của bạn trên Google Play để cho người chơi biết bất kỳ kỳ vọng nào về tay điều khiển. Nếu một trò chơi phù hợp hơn với một tay điều khiển trò chơi có cần điều khiển khác với một tay điều khiển chỉ có D-pad, hãy làm rõ điều này. Người chơi sử dụng một tay điều khiển không phù hợp với trò chơi có thể mang lại trải nghiệm không tốt và có điểm xếp hạng thấp.
- Sử dụng nút ánh xạ nhất quán: nút trực quan là chìa khoá đem lại trải nghiệm tốt cho người dùng. Ví dụ: tuân thủ các phong tục được chấp nhận bằng cách sử dụng nút A để chấp nhận và nút B để huỷ. Bạn cũng có thể linh hoạt ở dạng khả năng có lại. Để biết thêm thông tin về liên kết nút, hãy xem bài viết Xử lý của tay điều khiển.
- Phát hiện khả năng của đơn vị kiểm soát và điều chỉnh cho phù hợp: truy vấn đơn vị kiểm soát đó về khả năng tối ưu hoá mức độ trùng khớp giữa tay điều khiển và trò chơi. Ví dụ: có thể bạn có ý định để người chơi điều khiển một đối tượng bằng cách vẫy bộ điều khiển trên không, nhưng nếu một bộ điều khiển của người chơi thiếu phần cứng gia tốc kế và con quay hồi chuyển, tính năng vẫy tay không hoạt động. Truy vấn bộ điều khiển và nếu tính năng phát hiện chuyển động không hoạt động được hỗ trợ, chuyển sang một lược đồ kiểm soát thay thế và có sẵn. Thông tin khác về khả năng truy vấn bộ điều khiển, hãy xem phần Hỗ trợ trên các phiên bản Android.
Sử dụng các nút thích hợp
Không phải tay điều khiển trò chơi nào cũng cung cấp nút Bắt đầu, Tìm kiếm hoặc Trình đơn. Đảm bảo giao diện người dùng không phụ thuộc về việc sử dụng các nút này.
Xử lý nhiều bộ điều khiển
Khi nhiều người chơi cùng chơi một trò chơi, mỗi người đều có tay điều khiển riêng, điều quan trọng là
liên kết từng cặp người chơi – tay điều khiển. Để biết thông tin về cách triển khai tham số của bộ điều khiển
nhận dạng, xem
getControllerNumber()
.
Xử lý việc ngắt kết nối bộ điều khiển
Khi tay điều khiển bị ngắt kết nối trong quá trình chơi, hãy tạm dừng trò chơi và hiện thông báo hộp thoại nhắc người chơi đã ngắt kết nối kết nối lại bộ điều khiển.
Ngoài ra, hãy đưa ra các mẹo khắc phục sự cố trong hộp thoại. Ví dụ: hãy cho phát "Kiểm tra kết nối Bluetooth của bạn". Để biết thêm thông tin về cách triển khai thiết bị đầu vào hỗ trợ, xem bài viết Xử lý bộ điều khiển hành động và Tổng quan về Bluetooth.
Hiện hướng dẫn tay điều khiển
Nếu trò chơi của bạn cung cấp hướng dẫn điều khiển trò chơi bằng hình ảnh, hãy sử dụng hình ảnh tay điều khiển không có thương hiệu và chỉ bao gồm các nút tương thích với Android.
Để xem hình ảnh mẫu của bộ điều khiển tương thích với Android, hãy tải Mẫu tay điều khiển trò chơi (ZIP) dành cho Android TV. Ứng dụng này có một tay điều khiển màu trắng trên nền đen và một tay điều khiển màu đen trên nền trắng nền—như trong hình 1—dưới dạng tệp PNG và dưới dạng tệp Adobe® Illustrator®.
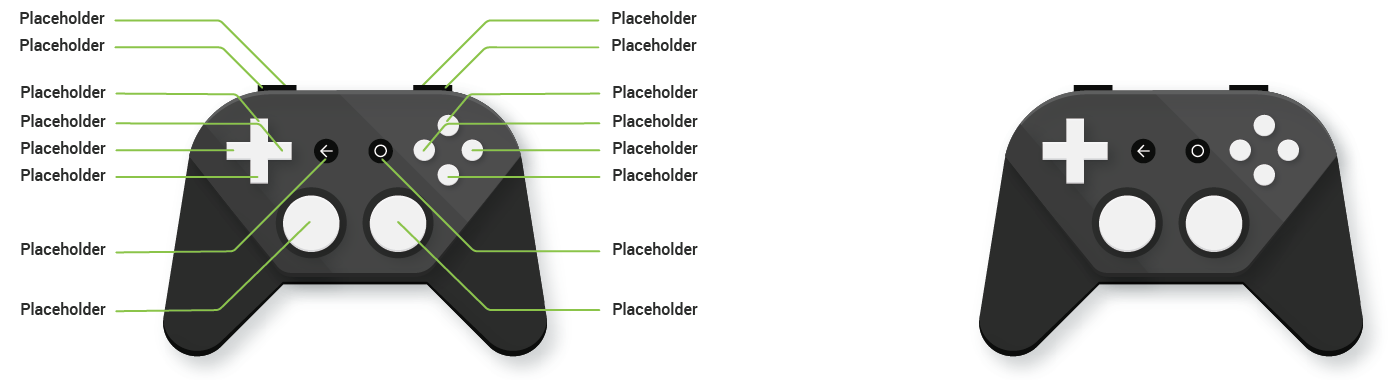
Hình 1. Ví dụ về hướng dẫn cho tay điều khiển bằng cách sử dụng Mẫu tay điều khiển trò chơi dành cho Android TV.