Votre application peut comporter des tâches en plusieurs étapes pour les utilisateurs. Par exemple, votre application peut avoir besoin de guider les utilisateurs dans l'achat de contenu supplémentaire, la configuration d'un paramètre de configuration complexe ou simplement la confirmation d'une décision. Toutes ces tâches nécessitent de guider les utilisateurs à travers une ou plusieurs étapes ou décisions ordonnées.
La bibliothèque androidx Leanback fournit des classes permettant d'implémenter des tâches utilisateur en plusieurs étapes. Cette page explique comment utiliser la classe GuidedStepSupportFragment
pour guider un utilisateur dans une série de décisions visant à accomplir une tâche. GuidedStepSupportFragment
utilise les bonnes pratiques liées à l'interface utilisateur des téléviseurs pour faciliter la compréhension et la navigation sur les tâches en plusieurs étapes sur les téléviseurs.
Fournir les détails d'une étape
Un GuidedStepSupportFragment
représente une seule étape dans une série d'étapes. Visuellement, il fournit une vue de référence avec une liste d'actions ou de décisions possibles pour l'étape.
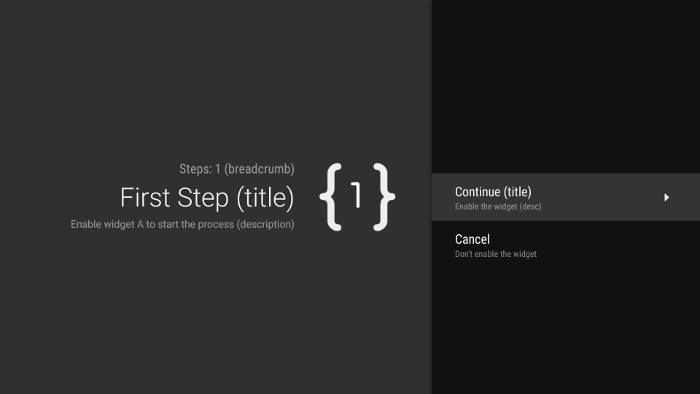
Figure 1 : Exemple d'étape guidée
Pour chaque étape de votre tâche en plusieurs étapes, développez GuidedStepSupportFragment
et fournissez des informations contextuelles sur l'étape et les actions que l'utilisateur peut effectuer. Remplacez onCreateGuidance()
et renvoyez un nouveau GuidanceStylist.Guidance
contenant des informations contextuelles, telles que le titre, la description et l'icône de l'étape, comme illustré dans l'exemple suivant:
Kotlin
override fun onCreateGuidance(savedInstanceState: Bundle?): GuidanceStylist.Guidance { return GuidanceStylist.Guidance( getString(R.string.guidedstep_first_title), getString(R.string.guidedstep_first_description), getString(R.string.guidedstep_first_breadcrumb), activity.getDrawable(R.drawable.guidedstep_main_icon_1) ) }
Java
@Override public GuidanceStylist.Guidance onCreateGuidance(Bundle savedInstanceState) { String title = getString(R.string.guidedstep_first_title); String breadcrumb = getString(R.string.guidedstep_first_breadcrumb); String description = getString(R.string.guidedstep_first_description); Drawable icon = getActivity().getDrawable(R.drawable.guidedstep_main_icon_1); return new GuidanceStylist.Guidance(title, description, breadcrumb, icon); }
Ajoutez votre sous-classe GuidedStepSupportFragment
à l'activité de votre choix en appelant GuidedStepSupportFragment.add()
dans la méthode onCreate()
de votre activité.
Si votre activité ne contient que des objets GuidedStepSupportFragment
, utilisez GuidedStepSupportFragment.addAsRoot()
au lieu de add()
pour ajouter le premier GuidedStepSupportFragment
. L'utilisation de addAsRoot()
permet de s'assurer que si l'utilisateur appuie sur le bouton "Retour" de la télécommande du téléviseur lors de l'affichage du premier GuidedStepSupportFragment
, l'activité GuidedStepSupportFragment
et l'activité parent se ferment.
Remarque:Ajoutez des objets GuidedStepSupportFragment
par programmation, et non dans vos fichiers XML de mise en page.
Créer et gérer des actions utilisateur
Ajoutez des actions utilisateur en remplaçant onCreateActions()
.
Dans votre forçage, ajoutez un nouveau GuidedAction
pour chaque action et fournissez la chaîne d'action, la description et l'ID. Utilisez GuidedAction.Builder
pour ajouter de nouvelles actions.
Kotlin
override fun onCreateActions(actions: MutableList<GuidedAction>, savedInstanceState: Bundle?) { super.onCreateActions(actions, savedInstanceState) // Add "Continue" user action for this step actions.add(GuidedAction.Builder() .id(CONTINUE) .title(getString(R.string.guidedstep_continue)) .description(getString(R.string.guidedstep_letsdoit)) .hasNext(true) .build()) ...
Java
@Override public void onCreateActions(List<GuidedAction> actions, Bundle savedInstanceState) { // Add "Continue" user action for this step actions.add(new GuidedAction.Builder() .id(CONTINUE) .title(getString(R.string.guidedstep_continue)) .description(getString(R.string.guidedstep_letsdoit)) .hasNext(true) .build()); ...
Les actions ne sont pas limitées aux sélections sur une seule ligne. Voici d'autres types d'actions que vous pouvez créer:
-
Ajoutez une action de libellé d'informations pour fournir des informations supplémentaires sur les choix des utilisateurs en définissant
infoOnly(true)
. LorsqueinfoOnly
est défini sur "true", les utilisateurs ne peuvent pas sélectionner l'action. -
Ajoutez une action textuelle modifiable en définissant
editable(true)
. Lorsqueeditable
est défini sur "true", l'utilisateur peut saisir du texte dans une action sélectionnée à l'aide de la télécommande ou d'un clavier connecté. IgnorezonGuidedActionEditedAndProceed()
pour obtenir le texte modifié saisi par l'utilisateur. Vous pouvez également ignoreronGuidedActionEditCanceled()
pour savoir quand l'utilisateur annule une entrée. -
Ajoutez un ensemble d'actions qui se comportent comme des cases d'option que l'utilisateur peut cocher en utilisant
checkSetId()
avec une valeur d'ID commune pour regrouper les actions dans un ensemble. Toutes les actions d'une même liste ayant le même ID d'ensemble de test sont considérées comme associées. Lorsque l'utilisateur sélectionne l'une des actions de cet ensemble, celle-ci est cochée et toutes les autres actions sont décochées. -
Ajoutez une action de sélecteur de date en utilisant
GuidedDatePickerAction.Builder
au lieu deGuidedAction.Builder
dansonCreateActions()
. RemplacezonGuidedActionEditedAndProceed()
pour obtenir la valeur de date de modification saisie par l'utilisateur. - Ajoutez une action qui utilise des sous-actions pour permettre à l'utilisateur de choisir parmi une liste étendue de choix. Les sous-actions sont décrites dans la section Ajouter des sous-actions.
- Ajoutez un bouton d'action qui s'affiche à droite de la liste des actions et qui est facilement accessible. Les actions de bouton sont décrites dans la section Ajouter des actions de bouton.
Vous pouvez également ajouter un indicateur visuel indiquant que la sélection d'une action entraîne une nouvelle étape en définissant hasNext(true)
.
Pour connaître les différents attributs que vous pouvez définir, consultez GuidedAction
.
Pour répondre aux actions, remplacez onGuidedActionClicked()
et traitez l'GuidedAction
transmise. Identifiez l'action sélectionnée en examinant GuidedAction.getId()
.
Ajouter des sous-actions
Certaines actions peuvent vous obliger à proposer des options supplémentaires à l'utilisateur. Un élément GuidedAction
peut spécifier une liste de sous-actions qui s'affichent sous la forme d'un menu d'actions enfants.
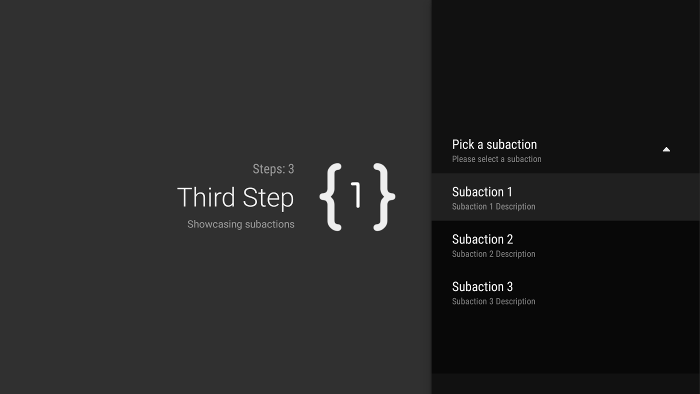
Figure 2. Sous-actions de pas guidées.
La liste de sous-actions peut contenir des actions standards ou des cases d'option, mais pas de sélecteur de date ni d'actions de texte modifiables. En outre, une sous-action ne peut pas avoir son propre ensemble de sous-actions, car le système n'accepte qu'un seul niveau de sous-actions.
Pour ajouter des sous-actions, commencez par créer et renseigner une liste d'objets GuidedAction
qui agissent en tant que sous-actions, comme illustré dans l'exemple suivant:
Kotlin
subActions.add(GuidedAction.Builder() .id(SUBACTION1) .title(getString(R.string.guidedstep_subaction1_title)) .description(getString(R.string.guidedstep_subaction1_desc)) .build()) ...
Java
List<GuidedAction> subActions = new ArrayList<GuidedAction>(); subActions.add(new GuidedAction.Builder() .id(SUBACTION1) .title(getString(R.string.guidedstep_subaction1_title)) .description(getString(R.string.guidedstep_subaction1_desc)) .build()); ...
Dans onCreateActions()
, créez un GuidedAction
de premier niveau qui affiche la liste des sous-actions lorsqu'elles sont sélectionnées:
Kotlin
... actions.add(GuidedAction.Builder() .id(SUBACTIONS) .title(getString(R.string.guidedstep_subactions_title)) .description(getString(R.string.guidedstep_subactions_desc)) .subActions(subActions) .build()) ...
Java
@Override public void onCreateActions(List<GuidedAction> actions, Bundle savedInstanceState) { ... actions.add(new GuidedAction.Builder() .id(SUBACTIONS) .title(getString(R.string.guidedstep_subactions_title)) .description(getString(R.string.guidedstep_subactions_desc)) .subActions(subActions) .build()); ... }
Enfin, répondez aux sélections de sous-actions en remplaçant onSubGuidedActionClicked()
:
Kotlin
override fun onSubGuidedActionClicked(action: GuidedAction): Boolean { // Check for which action was clicked and handle as needed when(action.id) { SUBACTION1 -> { // Subaction 1 selected } } // Return true to collapse the subactions menu or // false to keep the menu expanded return true }
Java
@Override public boolean onSubGuidedActionClicked(GuidedAction action) { // Check for which action was clicked and handle as needed if (action.getId() == SUBACTION1) { // Subaction 1 selected } // Return true to collapse the subactions menu or // false to keep the menu expanded return true; }
Ajouter des actions de bouton
Si votre étape guidée comporte une longue liste d'actions, les utilisateurs devront peut-être faire défiler la liste pour accéder aux actions les plus couramment utilisées. Utilisez les actions de bouton pour séparer les actions couramment utilisées de la liste d'actions. Les actions sur les boutons apparaissent à côté de la liste d'actions et sont faciles d'accès.
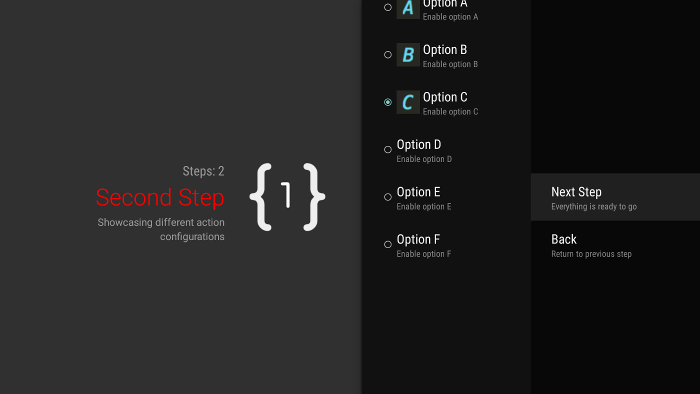
Figure 3. Actions guidées du bouton des pas.
Les actions de bouton sont créées et gérées comme les actions standards, à la différence que les actions de bouton sont créées dans onCreateButtonActions()
au lieu de onCreateActions()
. Répondez aux actions de bouton dans onGuidedActionClicked()
.
Utilisez les actions sur les boutons pour des actions simples, comme les actions de navigation entre les étapes. N'utilisez pas le sélecteur de date ni d'autres actions modifiables comme actions de boutons. De plus, les actions de bouton ne peuvent pas comporter de sous-actions.
Regrouper les étapes guidées dans une séquence guidée
Un GuidedStepSupportFragment
représente une seule étape. Pour créer une séquence d'étapes ordonnées, regroupez plusieurs objets GuidedStepSupportFragment
à l'aide de GuidedStepSupportFragment.add()
pour ajouter l'étape suivante de la séquence à la pile de fragments.
Kotlin
override fun onGuidedActionClicked(action: GuidedAction) { val fm = fragmentManager when(action.id) { CONTINUE -> GuidedStepSupportFragment.add(fm, SecondStepFragment()) } }
Java
@Override public void onGuidedActionClicked(GuidedAction action) { FragmentManager fm = getFragmentManager(); if (action.getId() == CONTINUE) { GuidedStepSupportFragment.add(fm, new SecondStepFragment()); } ...
Si l'utilisateur appuie sur le bouton "Retour" de la télécommande du téléviseur, l'appareil affiche l'élément GuidedStepSupportFragment
précédent sur la pile de fragments. Si vous fournissez votre propre GuidedAction
qui revient à l'étape précédente, vous pouvez implémenter le comportement "Retour" en appelant getFragmentManager().popBackStack()
.
Si vous devez renvoyer l'utilisateur à une étape encore précédente de la séquence, utilisez popBackStackToGuidedStepSupportFragment()
pour revenir à un GuidedStepSupportFragment
spécifique dans la pile de fragments.
Lorsque l'utilisateur a terminé la dernière étape de la séquence, utilisez finishGuidedStepSupportFragments()
pour supprimer toutes les instances GuidedStepSupportFragment
de la pile actuelle et revenir à l'activité parente d'origine. Si le premier GuidedStepSupportFragment
est ajouté à l'aide de addAsRoot()
, l'appel de finishGuidedStepSupportFragments()
ferme également l'activité parente.
Personnaliser la présentation des étapes
La classe GuidedStepSupportFragment
peut utiliser des thèmes personnalisés qui contrôlent des aspects de la présentation, tels que la mise en forme du texte du titre ou les animations de transition d'étape. Les thèmes personnalisés doivent hériter de Theme_Leanback_GuidedStep
et peuvent fournir des valeurs de remplacement pour les attributs définis dans GuidanceStylist
et GuidedActionsStylist
.
Pour appliquer un thème personnalisé à votre GuidedStepSupportFragment
, effectuez l'une des opérations suivantes:
-
Appliquez le thème à l'activité parente en définissant l'attribut
android:theme
sur l'élément d'activité dans le fichier manifeste Android. Définir cet attribut applique le thème à toutes les vues enfants et constitue le moyen le plus simple d'appliquer un thème personnalisé si l'activité parent ne contient que des objetsGuidedStepSupportFragment
. -
Si votre activité utilise déjà un thème personnalisé et que vous ne souhaitez pas appliquer de styles
GuidedStepSupportFragment
à d'autres vues de l'activité, ajoutez l'attributLeanbackGuidedStepTheme_guidedStepTheme
à votre thème d'activité personnalisé existant. Cet attribut pointe vers le thème personnalisé qui n'est utilisé que par les objetsGuidedStepSupportFragment
dans votre activité. -
Si vous utilisez des objets
GuidedStepSupportFragment
dans différentes activités qui font partie de la même tâche globale en plusieurs étapes et que vous souhaitez utiliser un thème visuel cohérent pour toutes les étapes, remplacezGuidedStepSupportFragment.onProvideTheme()
et renvoyez votre thème personnalisé.
Pour en savoir plus sur l'ajout de styles et de thèmes, consultez la section Styles et thèmes.
La classe GuidedStepSupportFragment
utilise des classes de styliste spéciales pour accéder aux attributs de thème et les appliquer.
La classe GuidanceStylist
utilise les informations du thème pour contrôler la présentation du guide de gauche, tandis que la classe GuidedActionsStylist
utilise les informations du thème pour contrôler la présentation de la vue d'actions appropriée.
Pour personnaliser le style visuel de vos étapes au-delà de ce que la personnalisation du thème fournit, sous-classez GuidanceStylist
ou GuidedActionsStylist
et renvoyez votre sous-classe dans GuidedStepSupportFragment.onCreateGuidanceStylist()
ou GuidedStepSupportFragment.onCreateActionsStylist()
.
Pour en savoir plus sur ce que vous pouvez personnaliser dans ces sous-classes, consultez la documentation sur GuidanceStylist
et GuidedActionsStylist
.