Barrier
public
class
Barrier
extends View
java.lang.Object | ||
↳ | View | |
↳ | android.support.constraint.Barrier |
Added in 1.1
A Barrier references multiple widgets as input, and creates a virtual guideline based on the most extreme widget on the specified side. For example, a left barrier will align to the left of all the referenced views.
Example
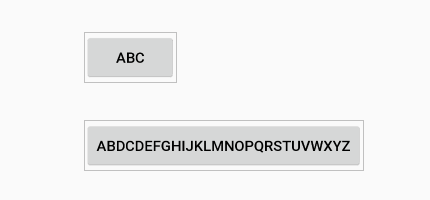
<android.support.constraint.Barrier
android:id="@+id/barrier"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
app:barrierDirection="start"
app:constraint_referenced_ids="button1,button2" />
With the barrier direction set to start, we will have the following result:
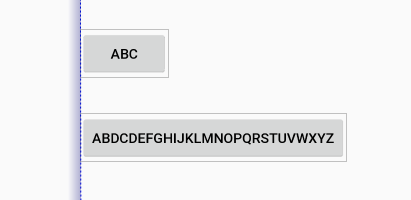
Reversely, with the direction set to end, we will have:
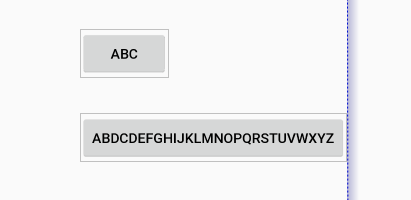
If the widgets dimensions change, the barrier will automatically move according to its direction to get the most extreme widget:
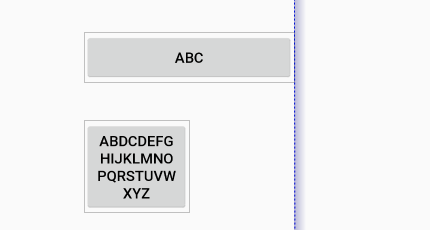
Other widgets can then be constrained to the barrier itself, instead of the individual widget. This allows a layout to automatically adapt on widget dimension changes (e.g. different languages will end up with different length for similar worlds).
GONE widgets handling
If the barrier references GONE widgets, the default behavior is to create a barrier on the resolved position of the GONE widget. If you do not want to have the barrier take GONE widgets into account, you can change this by setting the attribute barrierAllowsGoneWidgets to false (default being true).
Summary
Fields | |
---|---|
public
static
final
int |
BOTTOM
Bottom direction constant |
public
static
final
int |
END
End Barrier constant |
public
static
final
int |
LEFT
Left direction constant |
public
static
final
int |
RIGHT
Right direction constant |
public
static
final
int |
START
Start direction constant |
public
static
final
int |
TOP
Top direction constant |
Public constructors | |
---|---|
Barrier(Context context)
|
|
Barrier(Context context, AttributeSet attrs)
|
|
Barrier(Context context, AttributeSet attrs, int defStyleAttr)
|
Public methods | |
---|---|
boolean
|
allowsGoneWidget()
|
int
|
getMargin()
Returns the barrier margin |
int[]
|
getReferencedIds()
Helpers typically reference a collection of ids |
int
|
getType()
Get the barrier type ( |
void
|
loadParameters(ConstraintSet.Constraint constraint, HelperWidget child, ConstraintLayout.LayoutParams layoutParams,
|
void
|
resolveRtl(ConstraintWidget widget, boolean isRtl)
|
void
|
setAllowsGoneWidget(boolean supportGone)
|
void
|
setDpMargin(int margin)
Set a margin on the barrier |
void
|
setMargin(int margin)
Set the barrier margin |
void
|
setReferencedIds(int[] ids)
Helpers typically reference a collection of ids |
void
|
setType(int type)
Set the barrier type ( |
void
|
updatePostConstraints(ConstraintLayout constainer)
|
void
|
updatePreLayout(ConstraintWidgetContainer container, Helper helper,
|
Protected methods | |
---|---|
View[]
|
getViews(ConstraintLayout layout)
|
Inherited methods | |
---|---|
Fields
BOTTOM
public static final int BOTTOM
Bottom direction constant
END
public static final int END
End Barrier constant
LEFT
public static final int LEFT
Left direction constant
RIGHT
public static final int RIGHT
Right direction constant
START
public static final int START
Start direction constant
TOP
public static final int TOP
Top direction constant
Public constructors
Barrier
public Barrier (Context context)
Parameters | |
---|---|
context |
Context |
Barrier
public Barrier (Context context, AttributeSet attrs)
Parameters | |
---|---|
context |
Context |
attrs |
AttributeSet |
Barrier
public Barrier (Context context, AttributeSet attrs, int defStyleAttr)
Parameters | |
---|---|
context |
Context |
attrs |
AttributeSet |
defStyleAttr |
int |
Public methods
allowsGoneWidget
public boolean allowsGoneWidget ()
Returns | |
---|---|
boolean |
getMargin
public int getMargin ()
Returns the barrier margin
Returns | |
---|---|
int |
the barrier margin (in pixels) |
getReferencedIds
public int[] getReferencedIds ()
Helpers typically reference a collection of ids
Returns | |
---|---|
int[] |
ids referenced |
getType
public int getType ()
Get the barrier type (Barrier.LEFT
, Barrier.TOP
,
Barrier.RIGHT
, Barrier.BOTTOM
, Barrier.END
,
Barrier.START
)
Returns | |
---|---|
int |
loadParameters
public void loadParameters (ConstraintSet.Constraint constraint, HelperWidget child, ConstraintLayout.LayoutParams layoutParams,mapIdToWidget)
Parameters | |
---|---|
constraint |
ConstraintSet.Constraint |
child |
HelperWidget |
layoutParams |
ConstraintLayout.LayoutParams |
mapIdToWidget |
|
resolveRtl
public void resolveRtl (ConstraintWidget widget, boolean isRtl)
Parameters | |
---|---|
widget |
ConstraintWidget |
isRtl |
boolean |
setAllowsGoneWidget
public void setAllowsGoneWidget (boolean supportGone)
Parameters | |
---|---|
supportGone |
boolean |
setDpMargin
public void setDpMargin (int margin)
Set a margin on the barrier
Parameters | |
---|---|
margin |
int : in dp
|
setMargin
public void setMargin (int margin)
Set the barrier margin
Parameters | |
---|---|
margin |
int : in pixels
|
setReferencedIds
public void setReferencedIds (int[] ids)
Helpers typically reference a collection of ids
Parameters | |
---|---|
ids |
int |
Returns | |
---|---|
void |
ids referenced |
setType
public void setType (int type)
Set the barrier type (Barrier.LEFT
, Barrier.TOP
,
Barrier.RIGHT
, Barrier.BOTTOM
, Barrier.END
,
Barrier.START
)
Parameters | |
---|---|
type |
int |
updatePostConstraints
public void updatePostConstraints (ConstraintLayout constainer)
Parameters | |
---|---|
constainer |
ConstraintLayout |
updatePreLayout
public void updatePreLayout (ConstraintWidgetContainer container, Helper helper,map)
Parameters | |
---|---|
container |
ConstraintWidgetContainer |
helper |
Helper |
map |
|
Protected methods
getViews
protected View[] getViews (ConstraintLayout layout)
Parameters | |
---|---|
layout |
ConstraintLayout |
Returns | |
---|---|
View[] |