Este documento descreve como integrar o Gerenciador de credenciais a um app Android que usa a WebView.
Visão geral
Antes de se aprofundar no processo de integração, é importante entender o fluxo de comunicação entre o código nativo do Android, um componente da Web renderizado em uma WebView que gerencia a autenticação do app e um back-end. O fluxo envolve o registro (criação de credenciais) e a autenticação (recebimento de credenciais existentes).
Registro (criar uma chave de acesso)
- O back-end gera o arquivo JSON de registro inicial e o envia para a página da Web renderizada na WebView.
- A página da Web usa
navigator.credentials.create()
para registrar novas credenciais. Você vai usar o JavaScript injetado para substituir esse método em uma etapa posterior para enviar a solicitação ao app Android. - O app Android usa o Gerenciador de credenciais para criar a
solicitação de credencial e usá-la para
createCredential
. - O Gerenciador de credenciais compartilha a credencial de chave pública com o app.
- O app envia a credencial de chave pública de volta à página da Web para que o JavaScript injetado possa analisar as respostas.
- A página da Web envia a chave pública para o back-end, que a verifica e salva.
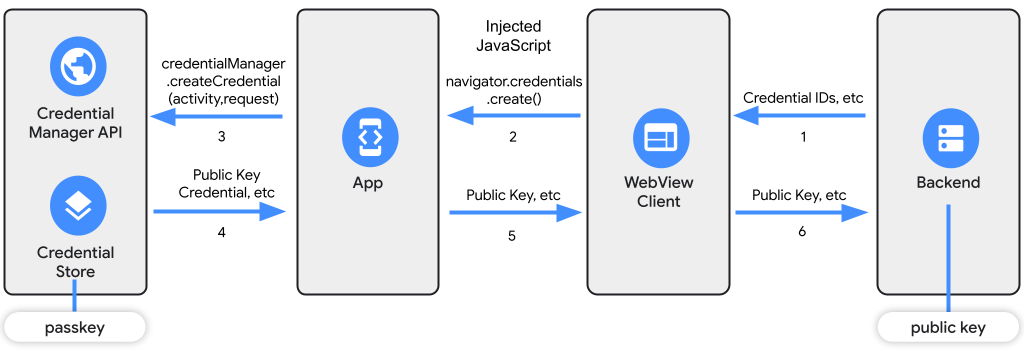
Autenticação (receber uma chave de acesso)
- O back-end gera um arquivo JSON de autenticação para receber a credencial e o envia para a página da Web renderizada no cliente da WebView.
- A página da Web usa
navigator.credentials.get
. Use o JavaScript injetado para substituir esse método para redirecionar a solicitação para o app Android. - O app recupera a credencial ao chamar
getCredential
no Gerenciador de credenciais. - O Gerenciador de credenciais retorna a credencial ao app.
- O app recebe a assinatura digital da chave privada e a envia à página da Web para que o JavaScript injetado possa analisar as respostas.
- Em seguida, a página da Web a envia ao servidor, que verifica a assinatura digital com a chave pública.
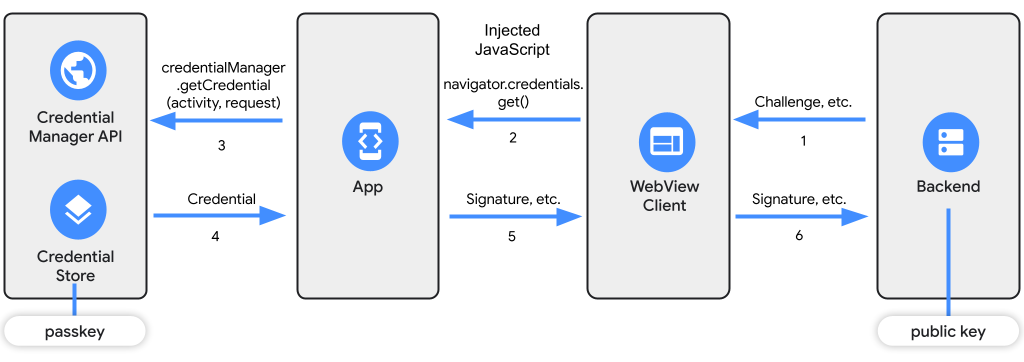
O mesmo fluxo pode ser usado para senhas ou sistemas de identidade federados.
Pré-requisitos
Para usar o Gerenciador de credenciais, conclua as etapas descritas na seção de pré-requisitos do guia da API e faça o seguinte:
- Adicione as dependências necessárias.
- Preserve as classes no arquivo do ProGuard.
- Adicione suporte ao Digital Asset Links.
Comunicação com JavaScript
Para permitir que o JavaScript em uma WebView e o código nativo do Android se comuniquem entre si, envie mensagens e processe solicitações entre os dois ambientes. Para fazer isso, injete um código JavaScript personalizado em uma WebView. Isso permite modificar o comportamento do conteúdo da Web e interagir com o código nativo do Android.
Injeção de JavaScript
O código JavaScript a seguir estabelece a comunicação
entre a WebView e o app Android. Ele substitui os métodos
navigator.credentials.create()
e
navigator.credentials.get()
usados pela
API WebAuthn para os fluxos de registro e autenticação
descritos anteriormente.
Use a versão reduzida desse código JavaScript no seu aplicativo.
Criar um listener para chaves de acesso
Configure uma classe PasskeyWebListener
para processar a comunicação
com o JavaScript. Ela precisa herdar de
WebViewCompat.WebMessageListener
. Essa classe recebe mensagens do
JavaScript e executa as ações necessárias no app Android.
As seções a seguir descrevem a estrutura da classe PasskeyWebListener
, além do processamento de solicitações e respostas.
Processar a solicitação de autenticação
Para processar solicitações de operações navigator.credentials.create()
ou
navigator.credentials.get()
da WebAuthn, o método onPostMessage
da
classe PasskeyWebListener
é chamado quando o código JavaScript envia uma mensagem
ao app Android:
// The class talking to Javascript should inherit:
class PasskeyWebListener(
private val activity: Activity,
private val coroutineScope: CoroutineScope,
private val credentialManagerHandler: CredentialManagerHandler
) : WebViewCompat.WebMessageListener {
/** havePendingRequest is true if there is an outstanding WebAuthn request.
There is only ever one request outstanding at a time. */
private var havePendingRequest = false
/** pendingRequestIsDoomed is true if the WebView has navigated since
starting a request. The FIDO module cannot be canceled, but the response
will never be delivered in this case. */
private var pendingRequestIsDoomed = false
/** replyChannel is the port that the page is listening for a response on.
It is valid if havePendingRequest is true. */
private var replyChannel: ReplyChannel? = null
/**
* Called by the page during a WebAuthn request.
*
* @param view Creates the WebView.
* @param message The message sent from the client using injected JavaScript.
* @param sourceOrigin The origin of the HTTPS request. Should not be null.
* @param isMainFrame Should be set to true. Embedded frames are not
supported.
* @param replyProxy Passed in by JavaScript. Allows replying when wrapped in
the Channel.
* @return The message response.
*/
@UiThread
override fun onPostMessage(
view: WebView,
message: WebMessageCompat,
sourceOrigin: Uri,
isMainFrame: Boolean,
replyProxy: JavaScriptReplyProxy,
) {
val messageData = message.data ?: return
onRequest(
messageData,
sourceOrigin,
isMainFrame,
JavaScriptReplyChannel(replyProxy)
)
}
private fun onRequest(
msg: String,
sourceOrigin: Uri,
isMainFrame: Boolean,
reply: ReplyChannel,
) {
msg?.let {
val jsonObj = JSONObject(msg);
val type = jsonObj.getString(TYPE_KEY)
val message = jsonObj.getString(REQUEST_KEY)
if (havePendingRequest) {
postErrorMessage(reply, "The request already in progress", type)
return
}
replyChannel = reply
if (!isMainFrame) {
reportFailure("Requests from subframes are not supported", type)
return
}
val originScheme = sourceOrigin.scheme
if (originScheme == null || originScheme.lowercase() != "https") {
reportFailure("WebAuthn not permitted for current URL", type)
return
}
// Verify that origin belongs to your website,
// it's because the unknown origin may gain credential info.
// if (isUnknownOrigin(originScheme)) {
// return
// }
havePendingRequest = true
pendingRequestIsDoomed = false
// Use a temporary "replyCurrent" variable to send the data back, while
// resetting the main "replyChannel" variable to null so it’s ready for
// the next request.
val replyCurrent = replyChannel
if (replyCurrent == null) {
Log.i(TAG, "The reply channel was null, cannot continue")
return;
}
when (type) {
CREATE_UNIQUE_KEY ->
this.coroutineScope.launch {
handleCreateFlow(credentialManagerHandler, message, replyCurrent)
}
GET_UNIQUE_KEY -> this.coroutineScope.launch {
handleGetFlow(credentialManagerHandler, message, replyCurrent)
}
else -> Log.i(TAG, "Incorrect request json")
}
}
}
private suspend fun handleCreateFlow(
credentialManagerHandler: CredentialManagerHandler,
message: String,
reply: ReplyChannel,
) {
try {
havePendingRequest = false
pendingRequestIsDoomed = false
val response = credentialManagerHandler.createPasskey(message)
val successArray = ArrayList<Any>();
successArray.add("success");
successArray.add(JSONObject(response.registrationResponseJson));
successArray.add(CREATE_UNIQUE_KEY);
reply.send(JSONArray(successArray).toString())
replyChannel = null // setting initial replyChannel for the next request
} catch (e: CreateCredentialException) {
reportFailure(
"Error: ${e.errorMessage} w type: ${e.type} w obj: $e",
CREATE_UNIQUE_KEY
)
} catch (t: Throwable) {
reportFailure("Error: ${t.message}", CREATE_UNIQUE_KEY)
}
}
companion object {
/** INTERFACE_NAME is the name of the MessagePort that must be injected into pages. */
const val INTERFACE_NAME = "__webauthn_interface__"
const val TYPE_KEY = "type"
const val REQUEST_KEY = "request"
const val CREATE_UNIQUE_KEY = "create"
const val GET_UNIQUE_KEY = "get"
/** INJECTED_VAL is the minified version of the JavaScript code described at this class
* heading. The non minified form is found at credmanweb/javascript/encode.js.*/
const val INJECTED_VAL = """
var __webauthn_interface__,__webauthn_hooks__;!function(e){console.log("In the hook."),__webauthn_interface__.addEventListener("message",function e(n){var r=JSON.parse(n.data),t=r[2];"get"===t?o(r):"create"===t?u(r):console.log("Incorrect response format for reply")});var n=null,r=null,t=null,a=null;function o(e){if(null!==n&&null!==t){if("success"!=e[0]){var r=t;n=null,t=null,r(new DOMException(e[1],"NotAllowedError"));return}var a=i(e[1]),o=n;n=null,t=null,o(a)}}function l(e){var n=e.length%4;return Uint8Array.from(atob(e.replace(/-/g,"+").replace(/_/g,"/").padEnd(e.length+(0===n?0:4-n),"=")),function(e){return e.charCodeAt(0)}).buffer}function s(e){return btoa(Array.from(new Uint8Array(e),function(e){return String.fromCharCode(e)}).join("")).replace(/\+/g,"-").replace(/\//g,"_").replace(/=+${'$'}/,"")}function u(e){if(null===r||null===a){console.log("Here: "+r+" and reject: "+a);return}if(console.log("Output back: "+e),"success"!=e[0]){var n=a;r=null,a=null,n(new DOMException(e[1],"NotAllowedError"));return}var t=i(e[1]),o=r;r=null,a=null,o(t)}function i(e){return console.log("Here is the response from credential manager: "+e),e.rawId=l(e.rawId),e.response.clientDataJSON=l(e.response.clientDataJSON),e.response.hasOwnProperty("attestationObject")&&(e.response.attestationObject=l(e.response.attestationObject)),e.response.hasOwnProperty("authenticatorData")&&(e.response.authenticatorData=l(e.response.authenticatorData)),e.response.hasOwnProperty("signature")&&(e.response.signature=l(e.response.signature)),e.response.hasOwnProperty("userHandle")&&(e.response.userHandle=l(e.response.userHandle)),e.getClientExtensionResults=function e(){return{}},e}e.create=function n(t){if(!("publicKey"in t))return e.originalCreateFunction(t);var o=new Promise(function(e,n){r=e,a=n}),l=t.publicKey;if(l.hasOwnProperty("challenge")){var u=s(l.challenge);l.challenge=u}if(l.hasOwnProperty("user")&&l.user.hasOwnProperty("id")){var i=s(l.user.id);l.user.id=i}var c=JSON.stringify({type:"create",request:l});return __webauthn_interface__.postMessage(c),o},e.get=function r(a){if(!("publicKey"in a))return e.originalGetFunction(a);var o=new Promise(function(e,r){n=e,t=r}),l=a.publicKey;if(l.hasOwnProperty("challenge")){var u=s(l.challenge);l.challenge=u}var i=JSON.stringify({type:"get",request:l});return __webauthn_interface__.postMessage(i),o},e.onReplyGet=o,e.CM_base64url_decode=l,e.CM_base64url_encode=s,e.onReplyCreate=u}(__webauthn_hooks__||(__webauthn_hooks__={})),__webauthn_hooks__.originalGetFunction=navigator.credentials.get,__webauthn_hooks__.originalCreateFunction=navigator.credentials.create,navigator.credentials.get=__webauthn_hooks__.get,navigator.credentials.create=__webauthn_hooks__.create,window.PublicKeyCredential=function(){},window.PublicKeyCredential.isUserVerifyingPlatformAuthenticatorAvailable=function(){return Promise.resolve(!1)};
"""
}
Para handleCreateFlow
e handleGetFlow
, consulte o exemplo no
GitHub (em inglês).
Processar a resposta
Para gerenciar as respostas enviadas do app nativo à página da Web, adicione
JavaScriptReplyProxy
ao JavaScriptReplyChannel
.
// The setup for the reply channel allows communication with JavaScript.
private class JavaScriptReplyChannel(private val reply: JavaScriptReplyProxy) :
ReplyChannel {
override fun send(message: String?) {
try {
reply.postMessage(message!!)
} catch (t: Throwable) {
Log.i(TAG, "Reply failure due to: " + t.message);
}
}
}
// ReplyChannel is the interface where replies to the embedded site are
// sent. This allows for testing since AndroidX bans mocking its objects.
interface ReplyChannel {
fun send(message: String?)
}
Capture todos os erros do app nativo e envie-os de volta para o lado do JavaScript.
Integrar com a WebView
Esta seção descreve como configurar sua integração com a WebView.
Inicializar a WebView
Na atividade do app Android, inicialize uma WebView
e configure um
WebViewClient
complementar. O WebViewClient
processa a comunicação com
o código JavaScript injetado na WebView
.
Configure a WebView e chame o Gerenciador de credenciais:
val credentialManagerHandler = CredentialManagerHandler(this)
setContent {
val coroutineScope = rememberCoroutineScope()
AndroidView(factory = {
WebView(it).apply {
settings.javaScriptEnabled = true
// Test URL:
val url = "https://passkeys-codelab.glitch.me/"
val listenerSupported = WebViewFeature.isFeatureSupported(
WebViewFeature.WEB_MESSAGE_LISTENER
)
if (listenerSupported) {
// Inject local JavaScript that calls Credential Manager.
hookWebAuthnWithListener(
this, this@WebViewMainActivity,
coroutineScope, credentialManagerHandler
)
} else {
// Fallback routine for unsupported API levels.
}
loadUrl(url)
}
}
)
}
Crie um novo objeto de cliente da WebView e injete o código JavaScript na página da Web:
val passkeyWebListener = PasskeyWebListener(activity, coroutineScope, credentialManagerHandler)
val webViewClient = object : WebViewClient() {
override fun onPageStarted(view: WebView?, url: String?, favicon: Bitmap?) {
super.onPageStarted(view, url, favicon)
webView.evaluateJavascript(PasskeyWebListener.INJECTED_VAL, null)
}
}
webView.webViewClient = webViewClient
Configurar um listener de mensagens da Web
Para permitir que mensagens sejam postadas entre o JavaScript e o app Android, configure um
listener de mensagens da Web com o método WebViewCompat.addWebMessageListener
.
val rules = setOf("*")
if (WebViewFeature.isFeatureSupported(WebViewFeature.WEB_MESSAGE_LISTENER)) {
WebViewCompat.addWebMessageListener(webView, PasskeyWebListener.INTERFACE_NAME,
rules, passkeyWebListener)
}
Integração com a Web
Para aprender a criar integração com a Web, confira as seções Criar uma chave de acesso para logins sem senha e Fazer login com uma chave de acesso usando o preenchimento automático de formulários.
Teste e implantação
Teste todo o fluxo em um ambiente controlado para garantir uma comunicação adequada entre o app Android, a página da Web e o back-end.
Implante a solução integrada na produção, garantindo que o back-end possa processar as solicitações de registro e autenticação. O código de back-end precisa gerar o arquivo JSON inicial para os processos de registro (criação) e autenticação (recebimento). Ele também precisa processar a validação e verificação das respostas recebidas da página da Web.
Verifique se a implementação corresponde às recomendações de UX.
Observações importantes
- Use o código JavaScript fornecido para processar as
operações
navigator.credentials.create()
enavigator.credentials.get()
. - A classe
PasskeyWebListener
é a ponte entre o app Android e o código JavaScript na WebView. Ela processa a transmissão de mensagens, a comunicação e a execução das ações necessárias. - Adapte os snippets de código fornecidos à estrutura, às convenções de nomenclatura e aos requisitos específicos do projeto.
- Detecte erros no app nativo e envie-os de volta para o lado do JavaScript.
Ao seguir este guia e integrar o Gerenciador de credenciais ao seu app Android que usa a WebView, você pode oferecer aos usuários uma experiência de login com chaves de acesso segura e integrada, bem como gerenciar as credenciais de maneira eficaz.