Probar la app es una parte integral del proceso de desarrollo. Por lo general, ejecutas apps en un emulador o dispositivo para verificar de forma manual que el código funcione como se espera. Sin embargo, las pruebas manuales requieren mucho tiempo, son susceptibles de errores y, a menudo, no se pueden administrar para apps que se ejecutan en pantallas y dispositivos de varios tamaños. Los problemas de las pruebas manuales suelen ser el resultado de usar un solo dispositivo para el desarrollo. Como resultado, los errores pueden pasar desapercibidos en otros dispositivos con diferentes factores de forma.
Para identificar regresiones en diferentes tamaños de ventana y pantalla, implementa pruebas automatizadas para verificar que el comportamiento y el aspecto de la app sean coherentes en diferentes factores de forma. Las pruebas automatizadas identifican los problemas con anticipación, lo que mitiga el riesgo de que afecten la experiencia del usuario.
Qué debes probar
Cuando desarrolles IUs para diferentes tamaños de pantalla y ventana, presta especial atención a dos aspectos:
- Cómo difieren los atributos visuales de los componentes y los diseños en ventanas de diferentes tamaños
- Cómo se preserva el estado en los cambios de configuración
Atributos visuales
Independientemente de si personalizas o no las IU para diferentes tamaños de ventana, debes verificar que las IU se muestren de forma correcta. Ten en cuenta el ancho y la altura que son compactos, medianos y extendidos. Consulta las Clases de tamaño de ventana para conocer los puntos de interrupción recomendados.
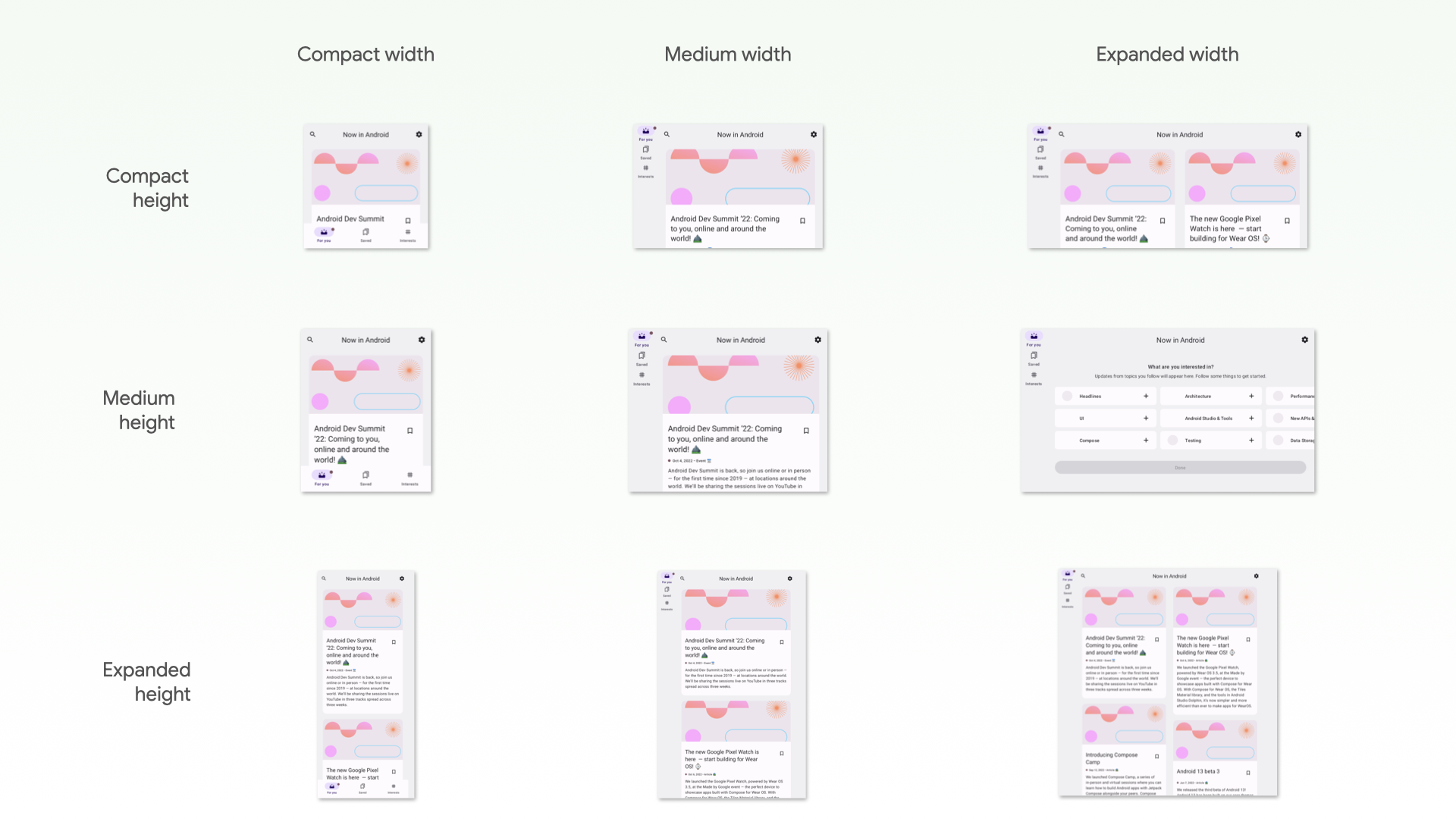
Además, es posible que tu app no renderice algunos componentes en el sistema de diseño como se espera cuando se extiendan las restricciones de tamaño.
Si tu app tiene diseños adaptables para diferentes tamaños de ventana, debes tener pruebas automatizadas para evitar regresiones. Por ejemplo, corregir un margen en un teléfono puede generar incoherencias de diseño en una tablet. Crea pruebas de IU para verificar el comportamiento de tus diseños y componentes, o crea pruebas de captura de pantalla para verificar los diseños visualmente.
Restauración del estado
Las apps que se ejecutan en dispositivos como las tablets se rotan y cambian de tamaño con mucha más frecuencia que las apps en teléfonos. Además, los dispositivos plegables introducen nuevas capacidades de pantalla, como plegar y desplegar, que pueden activar cambios de configuración. Tu app debe poder restablecer el estado cuando se producen estos cambios de configuración. Además, debes escribir pruebas que confirmen que tu app restablece el estado correctamente.
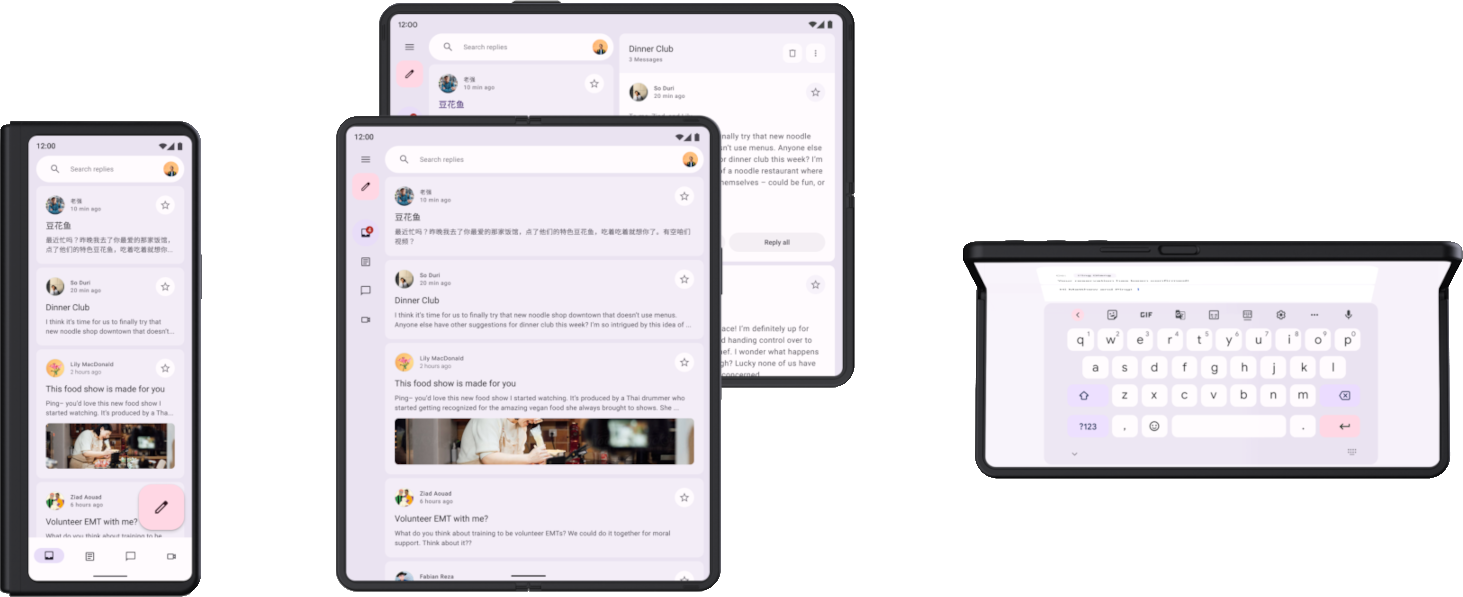
Primero, comprueba que tu app no falle cuando se produzcan cambios en la configuración. Asegúrate de que cada IU de tu app pueda controlar cualquier combinación de rotación, cambio de tamaño o plegado. Debido a que los cambios de configuración vuelven a crear la actividad de forma predeterminada, se producen algunas fallas debido a suposiciones de persistencia de la actividad.
Existen varias formas de probar los cambios de configuración, pero, en la mayoría de los casos, existen dos maneras de probarlos:
- En Compose, usa
StateRestorationTester
para simular un cambio de configuración de manera eficiente sin reiniciar la actividad. Consulta las siguientes secciones para obtener más información. - En cualquier prueba de IU, como Espresso o Compose, llama a
Activity.recreate()
para simular un cambio de configuración.
En general, no es necesario usar diferentes dispositivos para probar el restablecimiento del estado en respuesta a los cambios de configuración. Esto se debe a que todos los cambios de configuración que recrean la actividad tienen repercusiones similares. Sin embargo, algunos cambios en la configuración pueden activar diferentes mecanismos de restablecimiento de estado en dispositivos específicos.
Por ejemplo, cuando un usuario está viendo una IU de lista-detalles en un dispositivo plegable abierto y pliega el dispositivo para cambiar a la pantalla frontal, la IU suele cambiar a la página de detalles. Una prueba automatizada debe abarcar este restablecimiento del estado de la IU, incluido el estado de navegación.
Para probar los cambios de configuración que se realizan en dispositivos que pasan de una pantalla a otra o ingresan al modo multiventana, tienes varias opciones:
- Con cualquier dispositivo, puedes cambiar el tamaño de la pantalla durante una prueba. En la mayoría de los casos, esto activa todos los mecanismos de restablecimiento de estado que debes verificar. Sin embargo, esta prueba no funcionará para la lógica que detecte posiciones específicas en dispositivos plegables, ya que los cambios de posición no activan un cambio de configuración.
- Con un dispositivo o emulador que admita las funciones que quieras probar, activa los cambios de configuración relacionados. Por ejemplo, un dispositivo plegable o una tablet se puede controlar con el dispositivo Espresso para pasar del plegado a uno plano en la orientación horizontal. Para ver ejemplos, consulta la sección Dispositivo Espresso de Bibliotecas y herramientas para probar diferentes tamaños de pantalla.
Tipos de pruebas para diferentes tamaños de pantalla y ventana
Usa el tipo de prueba adecuado para cada caso de uso y verifica que la prueba funcione correctamente en diferentes factores de forma:
Las pruebas de comportamiento de la IU inician parte de la IU de la app, como la visualización de una actividad. Las pruebas verifican que ciertos elementos existan o tengan atributos específicos . De manera opcional, las pruebas pueden realizar acciones de usuario simuladas. Para las vistas, usa Espresso. Jetpack Compose tiene sus propias APIs de prueba. Las pruebas de comportamiento de la IU pueden ser instrumentadas o locales. Las pruebas de instrumentación se ejecutan en dispositivos o emuladores, mientras que las pruebas de IU locales se ejecutan en Robolectric en la JVM.
Usa pruebas de comportamiento de la IU para verificar que la implementación de la navegación de una app sea correcta. Las pruebas realizan acciones como hacer clic y deslizar el dedo. Las pruebas de comportamiento de la IU también comprueban la existencia de ciertos elementos o propiedades. Para obtener más información, consulta Cómo automatizar pruebas de IU.
Las pruebas de captura de pantalla toman una captura de pantalla de una IU o un componente y la comparan con una captura de pantalla aprobada previamente. Esta es una forma muy eficaz de protegerse contra las regresiones, ya que una sola captura de pantalla puede abarcar una gran cantidad de elementos y sus propiedades visuales. Puedes ejecutar pruebas de capturas de pantalla en la JVM o en los dispositivos. Hay varios frameworks de prueba de capturas de pantalla disponibles.
Por último, es posible que necesites pruebas de unidades para probar la funcionalidad de las unidades de lógica que se comportan de manera diferente según el tipo de dispositivo o el tamaño de la ventana, pero las pruebas de unidades son menos comunes en esta área.
Próximos pasos
Si deseas obtener más información para implementar las verificaciones que se incluyen en este documento, consulta Bibliotecas y herramientas.