如需直接从应用访问 Gemini API 和 Gemini 系列模型,我们建议您使用适用于 Android 的 Firebase SDK 中的 Vertex AI。此 SDK 属于更大的 Firebase 平台的一部分,可帮助您构建和运行全栈应用。
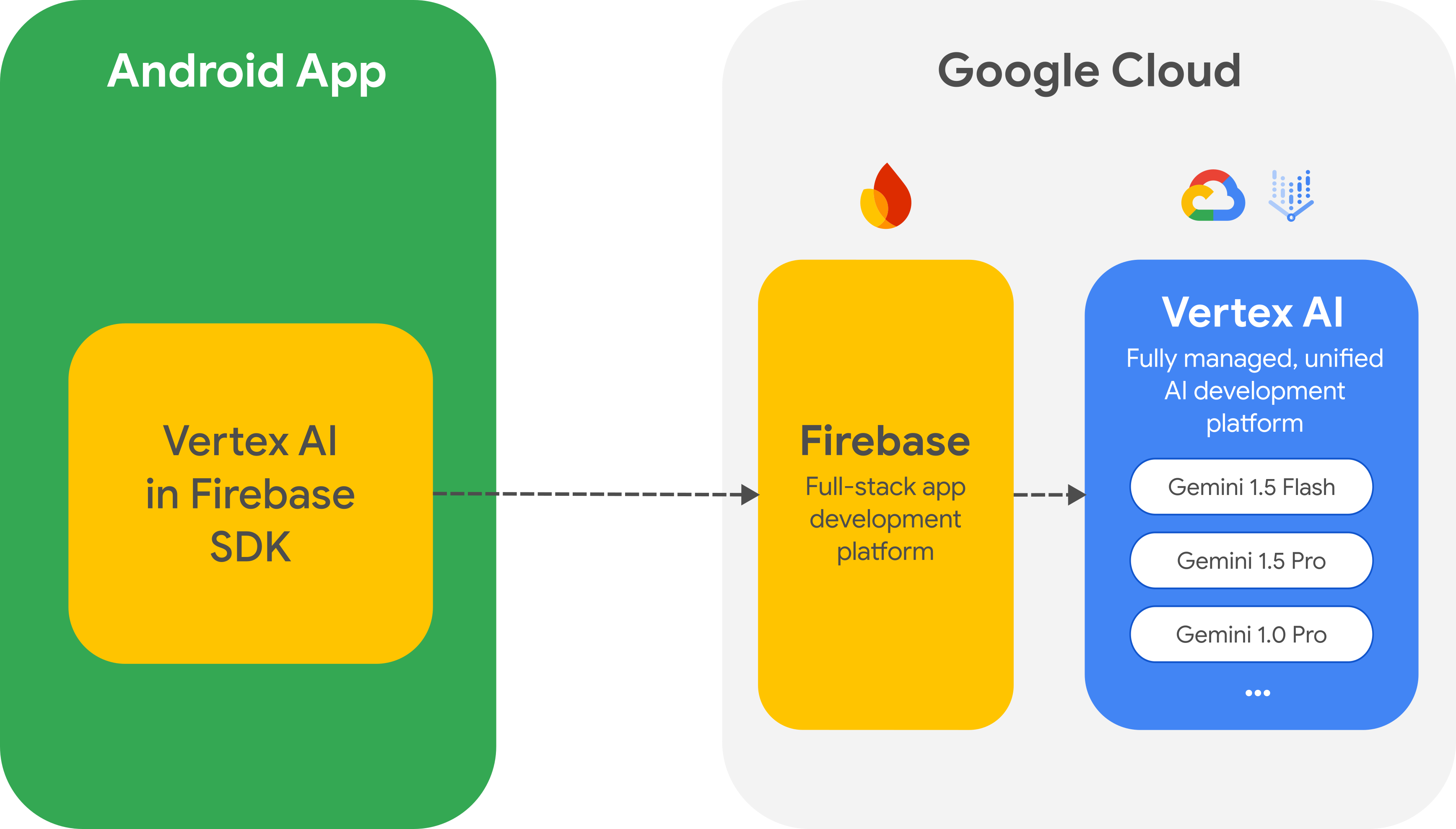
从 Google AI 客户端 SDK 迁移
Vertex AI in Firebase SDK 与 Google AI 客户端 SDK 类似,但 Vertex AI in Firebase SDK 针对生产用例提供了重要的安全选项和其他功能。例如,在 Firebase 中使用 Vertex AI 时,您还可以使用以下内容:
- Firebase App Check,以防止未经授权的客户端滥用 Gemini API。
- Firebase Remote Config,在云端动态设置和更改应用的值(例如模型名称),而无需发布应用的新版本。
- Cloud Storage for Firebase,以便在向 Gemini API 发出请求时添加大型媒体文件。
如果您已将 Google AI 客户端 SDK 集成到您的应用中,则可以迁移到 Firebase 中的 Vertex AI。
使用入门
在直接从应用中与 Gemini API 交互之前,您需要先完成一些操作,包括熟悉提示功能,以及设置 Firebase 和应用以使用 SDK。
使用提示进行实验
您可以在 Vertex AI Studio 中使用提示进行实验。Vertex AI Studio 是一个用于提示设计和原型设计的 IDE。您可以上传文件来测试包含文本和图片的提示,并保存提示以供日后再访问。
为您的用例创建合适的提示与其说是科学,不如说是艺术,因此实验至关重要。如需详细了解提示,请参阅 Firebase 文档。
设置 Firebase 项目并将您的应用连接到 Firebase
准备好从应用调用 Gemini API 后,请按照 Vertex AI in Firebase 入门指南中的说明在应用中设置 Firebase 和 SDK。该入门指南将帮助您完成本指南中的所有后续任务。
- 设置新的或现有的 Firebase 项目,包括使用随用随付 Blaze 定价方案和启用所需的 API。
- 将您的应用与 Firebase 相关联,包括注册您的应用并将 Firebase 配置文件 (
google-services.json
) 添加到您的应用。
添加 Gradle 依赖项
将以下 Gradle 依赖项添加到应用模块中:
Kotlin
dependencies {
...
// Import the BoM for the Firebase platform
implementation(platform("com.google.firebase:firebase-bom:33.12.0"))
// Add the dependency for the Vertex AI in Firebase library
// When using the BoM, you don't specify versions in Firebase library dependencies
implementation("com.google.firebase:firebase-vertexai")
}
Java
dependencies {
[...]
implementation("com.google.firebase:firebase-vertexai:16.3.0")
// Required to use `ListenableFuture` from Guava Android for one-shot generation
implementation("com.google.guava:guava:31.0.1-android")
// Required to use `Publisher` from Reactive Streams for streaming operations
implementation("org.reactivestreams:reactive-streams:1.0.4")
}
初始化 Vertex AI 服务和生成式模型
首先,实例化 GenerativeModel
并指定模型名称:
Kotlin
val generativeModel = Firebase.vertexAI.generativeModel("gemini-2.0-flash")
Java
GenerativeModel gm = FirebaseVertexAI.getInstance().generativeModel("gemini-2.0-flash");
在 Firebase 文档中,您可以详细了解可与 Vertex AI in Firebase 搭配使用的可用模型。您还可以了解如何配置模型参数。
从您的应用与 Gemini API 交互
现在,您已设置 Firebase 和应用以使用 SDK,接下来就可以从应用与 Gemini API 交互了。
生成文本
如需生成文本回答,请使用问题调用 generateContent()
。
Kotlin
// Note: `generateContent()` is a `suspend` function, which integrates well
// with existing Kotlin code.
scope.launch {
val response = model.generateContent("Write a story about the green robot")
}
Java
// In Java, create a `GenerativeModelFutures` from the `GenerativeModel`.
// Note that `generateContent()` returns a `ListenableFuture`. Learn more:
// https://developer.android.com/develop/background-work/background-tasks/asynchronous/listenablefuture
GenerativeModelFutures model = GenerativeModelFutures.from(gm);
Content prompt = new Content.Builder()
.addText("Write a story about a green robot.")
.build();
ListenableFuture<GenerateContentResponse> response = model.generateContent(prompt);
Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() {
@Override
public void onSuccess(GenerateContentResponse result) {
String resultText = result.getText();
}
@Override
public void onFailure(Throwable t) {
t.printStackTrace();
}
}, executor);
根据图片和其他媒体内容生成文本
您还可以根据包含文字、图片或其他媒体内容的提示生成文字。调用 generateContent()
时,您可以将媒体作为内嵌数据传递(如以下示例所示)。或者,您也可以使用 Cloud Storage for Firebase 网址在请求中添加大型媒体文件。
Kotlin
scope.launch {
val response = model.generateContent(
content {
image(bitmap)
text("what is the object in the picture?")
}
)
}
Java
GenerativeModelFutures model = GenerativeModelFutures.from(gm);
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.sparky);
Content prompt = new Content.Builder()
.addImage(bitmap)
.addText("What developer tool is this mascot from?")
.build();
ListenableFuture<GenerateContentResponse> response = model.generateContent(prompt);
Futures.addCallback(response, new FutureCallback<GenerateContentResponse>() {
@Override
public void onSuccess(GenerateContentResponse result) {
String resultText = result.getText();
}
@Override
public void onFailure(Throwable t) {
t.printStackTrace();
}
}, executor);
多轮聊天
您还可以支持多轮对话。使用 startChat()
函数初始化聊天。您可以选择提供消息历史记录。然后,调用 sendMessage()
函数发送聊天消息。
Kotlin
val chat = generativeModel.startChat(
history = listOf(
content(role = "user") { text("Hello, I have 2 dogs in my house.") },
content(role = "model") { text("Great to meet you. What would you like to know?") }
)
)
scope.launch {
val response = chat.sendMessage("How many paws are in my house?")
}
Java
// (Optional) create message history
Content.Builder userContentBuilder = new Content.Builder();
userContentBuilder.setRole("user");
userContentBuilder.addText("Hello, I have 2 dogs in my house.");
Content userContent = userContentBuilder.build();
Content.Builder modelContentBuilder = new Content.Builder();
modelContentBuilder.setRole("model");
modelContentBuilder.addText("Great to meet you. What would you like to know?");
Content modelContent = userContentBuilder.build();
List<Content> history = Arrays.asList(userContent, modelContent);
// Initialize the chat
ChatFutures chat = model.startChat(history);
// Create a new user message
Content.Builder messageBuilder = new Content.Builder();
messageBuilder.setRole("user");
messageBuilder.addText("How many paws are in my house?");
Content message = messageBuilder.build();
Publisher<GenerateContentResponse> streamingResponse =
chat.sendMessageStream(message);
StringBuilder outputContent = new StringBuilder();
streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() {
@Override
public void onNext(GenerateContentResponse generateContentResponse) {
String chunk = generateContentResponse.getText();
outputContent.append(chunk);
}
@Override
public void onComplete() {
// ...
}
@Override
public void onError(Throwable t) {
t.printStackTrace();
}
@Override
public void onSubscribe(Subscription s) {
s.request(Long.MAX_VALUE);
}
});
逐字逐句给出回答
您可以通过不等待模型生成的完整结果,而是使用流式处理部分结果,从而实现更快的互动。使用 generateContentStream()
流式传输响应。
Kotlin
scope.launch {
var outputContent = ""
generativeModel.generateContentStream(inputContent)
.collect { response ->
outputContent += response.text
}
}
Java
// Note that in Java the method `generateContentStream()` returns a
// Publisher from the Reactive Streams library.
// https://www.reactive-streams.org/
GenerativeModelFutures model = GenerativeModelFutures.from(gm);
// Provide a prompt that contains text
Content prompt = new Content.Builder()
.addText("Write a story about a green robot.")
.build();
Publisher<GenerateContentResponse> streamingResponse =
model.generateContentStream(prompt);
StringBuilder outputContent = new StringBuilder();
streamingResponse.subscribe(new Subscriber<GenerateContentResponse>() {
@Override
public void onNext(GenerateContentResponse generateContentResponse) {
String chunk = generateContentResponse.getText();
outputContent.append(chunk);
}
@Override
public void onComplete() {
// ...
}
@Override
public void onError(Throwable t) {
t.printStackTrace();
}
@Override
public void onSubscribe(Subscription s) {
s.request(Long.MAX_VALUE);
}
});
后续步骤
- 查看 GitHub 上的 Vertex AI in Firebase 示例应用。
- 开始考虑为正式版做好准备,包括设置 Firebase App Check,以保护 Gemini API 免遭未经授权的客户端滥用。
- 如需详细了解 Vertex AI in Firebase,请参阅 Firebase 文档。