bundletool
एक ऐसा टूल है जो Android Studio, Android Gradle है
प्लगिन और Google Play का इस्तेमाल करके Android ऐप्लिकेशन बंडल बनाया जाता है. bundletool
अभी तक किसी भी व्यक्ति ने चेक इन नहीं किया है
किसी ऐप्लिकेशन बंडल को डिवाइसों पर डिप्लॉय किए गए अलग-अलग APK में बदल सकता है.
Android SDK बंडल (ASB) और उनके APK, bundletool
की मदद से बनाए गए हैं. हां
कमांड-लाइन टूल के तौर पर भी उपलब्ध है, ताकि आप ऐप्लिकेशन बंडल बना सकें और
SDK टूल खुद को बंडल करते हैं और अपने ऐप्लिकेशन के Google Play के सर्वर-साइड बिल्ड को फिर से बनाते हैं
APK या आपके
रनटाइम की सुविधा वाले SDK टूल के APKs.
bundletool
भाषा डाउनलोड करें
अगर आपने पहले से ऐसा नहीं किया है, तो यहां से bundletool
को डाउनलोड करें:
GitHub का डेटा स्टोर करने की जगह.
ऐप्लिकेशन बंडल बनाना और उसकी जांच करना
अपने ऐप्लिकेशन को बनाने के लिए, Android Studio या bundletool
कमांड-लाइन टूल का इस्तेमाल किया जा सकता है
Android ऐप्लिकेशन बंडल चुनें और फिर इस ऐप्लिकेशन बंडल से APK जनरेट करने की जांच करें.
ऐप्लिकेशन बंडल बनाना
Android Studio और 'Android Gradle प्लग इन' का इस्तेमाल करके
Android ऐप्लिकेशन बंडल बनाएं और उस पर हस्ताक्षर करें.
हालांकि, अगर IDE का इस्तेमाल करना एक विकल्प नहीं है—उदाहरण के लिए, क्योंकि आप
लगातार चलने वाला सर्वर है, तो आपको अपने संगठन के
कमांड लाइन से अपना ऐप्लिकेशन बंडल बनाना
और इसका इस्तेमाल करके हस्ताक्षर करें
jarsigner
.
bundletool
के साथ ऐप्लिकेशन बंडल बनाने के बारे में ज़्यादा जानकारी के लिए,
देखें
बंडलटूल का इस्तेमाल करके ऐप्लिकेशन बंडल बनाएं.
अपने ऐप्लिकेशन बंडल से APKs का सेट जनरेट करें
Android ऐप्लिकेशन बंडल बनाने के बाद, देखें कि Google Play किस तरह से काम करता है इसका इस्तेमाल APK जनरेट करने के लिए करता है. साथ ही, यह भी बताता है कि डिवाइस पर डिप्लॉय किए जाने पर ये APK कैसे काम करते हैं.
अपने ऐप्लिकेशन बंडल की जांच करने के दो तरीके हैं:
bundletool
कमांड-लाइन टूल का इस्तेमाल स्थानीय तौर पर करें.- Play Console पर अपना बंडल अपलोड करना Google Play पर उपलब्ध है.
इस सेक्शन में बताया गया है कि ऐप्लिकेशन बंडल को स्थानीय तौर पर टेस्ट करने के लिए, bundletool
का इस्तेमाल कैसे किया जा सकता है.
जब bundletool
आपके ऐप्लिकेशन बंडल से APKs जनरेट करता है, तो उसमें जनरेट किए गए APK भी शामिल होते हैं
ऐसे कंटेनर में APK जिसे APK सेट संग्रह कहते हैं, जिसमें .apks
फ़ाइल का इस्तेमाल किया जाता है
एक्सटेंशन चुनें. आपका ऐप्लिकेशन सभी डिवाइस कॉन्फ़िगरेशन के लिए सेट किया गया APK जनरेट करने के लिए
को डाउनलोड करने की सुविधा देता है, तो bundletool build-apks
निर्देश का इस्तेमाल इस तरह करें
दिखाया गया:
bundletool build-apks --bundle=/MyApp/my_app.aab --output=/MyApp/my_app.apks
अगर आप किसी डिवाइस पर APK डिप्लॉय करना चाहते हैं, तो आपको अपने ऐप्लिकेशन के
जैसा कि नीचे दिए गए कमांड में दिखाया गया है. अगर आप तय नहीं करते हैं, तो
साइनिंग जानकारी, bundletool
आपके APK को डीबग कुंजी से साइन करने की कोशिश करता है
आपके लिए.
bundletool build-apks --bundle=/MyApp/my_app.aab --output=/MyApp/my_app.apks --ks=/MyApp/keystore.jks --ks-pass=file:/MyApp/keystore.pwd --ks-key-alias=MyKeyAlias --key-pass=file:/MyApp/key.pwd
नीचे दी गई टेबल में उन अलग-अलग फ़्लैग और विकल्पों के बारे में बताया गया है जिन्हें कब सेट किया जा सकता है
bundletool build-apks
कमांड का इस्तेमाल करके ज़्यादा जानकारी पाएं:
टेबल 1.
bundletool build-apks
निर्देश
चिह्नित करें | ब्यौरा |
---|---|
--bundle=path |
(ज़रूरी है) ऐप्लिकेशन बंडल का पाथ बताता है जिसे Android Studio का इस्तेमाल करके बनाया है. इस बारे में ज़्यादा जानने के लिए, पढ़ें अपना प्रोजेक्ट बनाएं. |
--output=path |
(ज़रूरी है) आउटपुट का नाम बताता है
.apks फ़ाइल मिलेगी, जिसमें आपके ऐप्लिकेशन के सभी APK आर्टफ़ैक्ट शामिल हैं
है. इस फ़ाइल में मौजूद आर्टफ़ैक्ट को किसी डिवाइस पर टेस्ट करने के लिए, इसमें दिया गया तरीका अपनाएं
APK डिप्लॉय करने के तरीके से जुड़ा सेक्शन
कनेक्ट किए गए डिवाइस पर.
|
--overwrite |
यह किसी भी मौजूदा आउटपुट फ़ाइल को इससे ओवरराइट कर देता है
ऐसा पाथ जिसे आपने --output विकल्प का इस्तेमाल करके तय किया है. अगर आपको
इस फ़्लैग को शामिल न करें और आउटपुट फ़ाइल पहले से मौजूद है, तो आपको
बिल्ड में गड़बड़ी हुई.
|
--aapt2=path |
AAPT2 के लिए पसंद के मुताबिक पाथ बताता है.
डिफ़ॉल्ट रूप से, bundletool में AAPT2 का अपना वर्शन शामिल है.
|
--ks=path |
(ज़रूरी नहीं) डिप्लॉयमेंट कीस्टोर का पाथ बताता है, ताकि
APK साइन करना चाहते हैं. अगर इस फ़्लैग को शामिल नहीं किया जाता है,
bundletool , डीबग साइनिंग की मदद से आपके APK को साइन करने की कोशिश करता है
बटन दबाएं.
|
--ks-pass=pass:password या --ks-pass=file:/path/to/file |
यह आपके कीस्टोर पासवर्ड के बारे में बताता है. अगर आपको
पासवर्ड को सादे टेक्स्ट में लिखें और pass: से पासवर्ड क्वालीफ़ाई करें.
अगर आपने किसी ऐसी फ़ाइल का पाथ पास किया है जिसमें पासवर्ड है, तो
file: के साथ. अगर आपको किसी
--ks-pass तय किए बिना --ks फ़्लैग,
bundletool आपको कमांड लाइन से पासवर्ड डालने के लिए कहता है.
|
--ks-key-alias=alias |
आपको जिस साइनिंग पासकोड से साइन इन करना है उसका उपनाम बताता है इस्तेमाल करें. |
--key-pass=pass:password या --key-pass=file:/path/to/file |
यह साइनिंग पासकोड के लिए पासवर्ड बताता है. अगर आपको
पासवर्ड को सादे टेक्स्ट में लिखें और pass: से पासवर्ड क्वालीफ़ाई करें.
अगर आप किसी ऐसी फ़ाइल का पाथ पास करते हैं जिसमें पासवर्ड है, तो
file: के साथ. अगर यह पासवर्ड, के लिए, आप इस फ़्लैग को छोड़ सकते हैं. |
--connected-device |
यह bundletool को ऐसे APK बनाने का निर्देश देता है जो
कनेक्ट किए गए डिवाइस का कॉन्फ़िगरेशन. अगर इस फ़्लैग को शामिल नहीं किया जाता है,
bundletool , आपके सभी डिवाइस कॉन्फ़िगरेशन के लिए APKs जनरेट करता है
काम करता है.
|
--device-id=serial-number |
अगर आपके पास एक से ज़्यादा कनेक्ट किए गए डिवाइस हैं, तो उस डिवाइस का सीरियल आईडी डालें जिस पर आपको अपना ऐप्लिकेशन डिप्लॉय करना है. |
--device-spec=spec_json |
आपकी जानकारी के लिए
.json फ़ाइल, जो आपके पसंदीदा डिवाइस कॉन्फ़िगरेशन के बारे में बताती है
टारगेट करने के लिए. ज़्यादा जानने के लिए,
डिवाइस के हिसाब से JSON फ़ॉर्मैट जनरेट और इस्तेमाल करना
फ़ाइलें में सेव किया जाता है.
|
--mode=universal |
मोड को universal पर सेट करता है. अगर आप चाहें, तो इस विकल्प का इस्तेमाल करें
bundletool ऐसा सिंगल APK बनाने के लिए करें जिसमें ये सभी चीज़ें शामिल हों
आपके ऐप्लिकेशन के कोड और संसाधनों को ऐक्सेस कर सके, ताकि APK सभी
ऐसे डिवाइस कॉन्फ़िगरेशन जो आपके ऐप्लिकेशन पर काम करते हों.
ध्यान दें: ध्यान रखें कि ये APK, ऑप्टिमाइज़ किए गए APK से बड़े हैं ख़ास डिवाइस कॉन्फ़िगरेशन के लिए. हालांकि, वे आसानी से इंटरनल टेस्टर के साथ शेयर कर सकते हैं, जैसे कि कई डिवाइस कॉन्फ़िगरेशन पर आपके ऐप्लिकेशन की जांच करना चाहते हैं. |
--local-testing
|
इससे आपके ऐप्लिकेशन बंडल को स्थानीय जांच के लिए चालू किया जाता है.
लोकल टेस्टिंग की मदद से, बिना किसी ज़रूरत के तुरंत और बार-बार टेस्ट किया जा सकता है
Google Play सर्वर पर अपलोड करने के लिए.
यहां दिए गए उदाहरण का इस्तेमाल करके, मॉड्यूल इंस्टॉलेशन की जांच करने का तरीका जानें.
|
कनेक्ट किए गए डिवाइस पर APKs डिप्लॉय करना
APK का सेट जनरेट करने के बाद, bundletool
जिसमें से APK के उन वर्शन को मिलाकर बनाया गया है जो कनेक्ट किए गए डिवाइस पर सेट हैं.
उदाहरण के लिए, अगर आपके पास कनेक्ट किया गया कोई डिवाइस है, जिस पर Android 5.0 (एपीआई लेवल 21) चल रहा है
या उसके बाद के वर्शन का इस्तेमाल करने पर, bundletool
बेस APK, फ़ीचर मॉड्यूल APK को पुश करता है, और
कॉन्फ़िगरेशन APKs को इंस्टॉल करना ज़रूरी है. इसके अलावा, अगर
कनेक्ट किया गया आपका डिवाइस, Android 4.4 (एपीआई लेवल 20) या इससे पहले के वर्शन पर चल रहा है,
bundletool
आपके डिवाइस पर डिप्लॉय करने के लिए, काम करने वाला मल्टी-APK खोजता है.
अपने ऐप्लिकेशन को APK सेट से डिप्लॉय करने के लिए, install-apks
निर्देश का इस्तेमाल करें और तय करें कि
का उपयोग करके सेट किए गए APK का पाथ
--apks=/path/to/apks
फ़्लैग, इसके तौर पर
जो नीचे दिए गए निर्देश में दिखाए गए हैं. अगर आपने कई डिवाइस कनेक्ट किए हुए हैं,
इसे जोड़कर लक्षित डिवाइस तय करें
--device-id=serial-id
फ़्लैग.
bundletool install-apks --apks=/MyApp/my_app.apks
डिवाइस के हिसाब से, APK का सेट जनरेट करना
अगर आपको अपने ऐप्लिकेशन के सभी डिवाइस कॉन्फ़िगरेशन के लिए APKs का सेट नहीं बनाना है, तो
का इस्तेमाल करता है, तो आप ऐसे APK बना सकते हैं जो सिर्फ़ कनेक्ट किए गए कॉन्फ़िगरेशन को टारगेट करते हैं
डिवाइस का --connected-device
विकल्प उपयोग कर रहा है, जैसा कि नीचे दिए गए आदेश में दिखाया गया है.
अगर आपने कई डिवाइस कनेक्ट किए हुए हैं, तो
--device-id=serial-id
फ़्लैग.
bundletool build-apks --connected-device --bundle=/MyApp/my_app.aab --output=/MyApp/my_app.apks
डिवाइस के हिसाब से JSON फ़ाइलें जनरेट करना और उनका इस्तेमाल करना
bundletool
, किसी डिवाइस को टारगेट करने वाला APK सेट जनरेट कर सकता है
JSON फ़ाइल में सेट किया गया कॉन्फ़िगरेशन. सबसे पहले
कनेक्ट किए गए डिवाइस के लिए, नीचे दिया गया निर्देश चलाएं:
bundletool get-device-spec --output=/tmp/device-spec.json
bundletool
, टूल की डायरेक्ट्री में आपके डिवाइस के लिए JSON फ़ाइल बनाता है. आपने लोगों तक पहुंचाया मुफ़्त में
इसके बाद, APK का सेट जनरेट करने के लिए फ़ाइल को bundletool
को पास कर सकता है
उस JSON फ़ाइल में बताए गए कॉन्फ़िगरेशन को ही टारगेट करें:
bundletool build-apks --device-spec=/MyApp/pixel2.json --bundle=/MyApp/my_app.aab --output=/MyApp/my_app.apks
मैन्युअल तरीके से डिवाइस की खास बातें JSON बनाएं
अगर आपके पास उस डिवाइस का ऐक्सेस नहीं है जिसके लिए आपको APK सेट है—उदाहरण के लिए, अगर आपको किसी ऐसे डिवाइस पर अपने ऐप्लिकेशन को आज़माना है जिस पर आपके पास उपलब्ध है—आपके पास नीचे दिए गए फ़ॉर्मैट का इस्तेमाल करके, मैन्युअल तरीके से JSON फ़ाइल बनाने का विकल्प है:
{
"supportedAbis": ["arm64-v8a", "armeabi-v7a"],
"supportedLocales": ["en", "fr"],
"screenDensity": 640,
"sdkVersion": 27
}
इसके बाद, इस JSON को bundle extract-apks
कमांड को पास किया जा सकता है. इसका तरीका बताया गया है
पर क्लिक करें.
किसी मौजूदा APK सेट से, डिवाइस के हिसाब से बनाए गए APK एक्सट्रैक्ट करना
अगर आपके पास कोई मौजूदा APK सेट है और आप उससे APK का सबसेट निकालना चाहते हैं, तो
जो खास डिवाइस कॉन्फ़िगरेशन को टारगेट करते हैं, तो आप extract-apks
का इस्तेमाल कर सकते हैं
निर्देश दें और डिवाइस स्पेसिफ़िकेशन JSON को इस तरह से बताएँ:
bundletool extract-apks --apks=/MyApp/my_existing_APK_set.apks --output-dir=/MyApp/my_pixel2_APK_set.apks --device-spec=/MyApp/bundletool/pixel2.json
किसी APK सेट में मौजूद APKs के अनुमानित डाउनलोड साइज़ का आकलन करना
किसी APK में APK के डाउनलोड साइज़ का अनुमानित साइज़ मापने के लिए, जैसे कि वे
तार की मदद से कंप्रेस किया गया हो, इसके लिए get-size total
निर्देश का इस्तेमाल करें:
bundletool get-size total --apks=/MyApp/my_app.apks
get-size total
कमांड के व्यवहार में बदलाव करने के लिए,
ये फ़्लैग मिल जाएंगे:
दूसरी टेबल.
get-size total
निर्देश
चिह्नित करें | ब्यौरा |
---|---|
--apks=path |
(ज़रूरी है) मौजूदा APK सेट फ़ाइल का पाथ बताता है जिसका डाउनलोड साइज़ मापा जा रहा है. |
--device-spec=path |
डिवाइस की खास जानकारी वाली फ़ाइल का पाथ बताता है (इससे
get-device-spec या मैन्युअल तरीके से बनाया गया हो).
कॉन्फ़िगरेशन के सेट का आकलन करने के लिए, पार्शियल पाथ तय किया जा सकता है.
|
--dimensions=dimensions
| साइज़ के अनुमान का हिसाब लगाते समय इस्तेमाल किए गए डाइमेंशन की जानकारी देता है. स्वीकार की जाती हैं
कॉमा लगाकर अलग की गई सूची: SDK , ABI ,
SCREEN_DENSITY और LANGUAGE . इनकी मदद से,
सभी डाइमेंशन में, ALL तय करें.
|
--instant |
यह तुरंत चालू होने वाले APK के डाउनलोड साइज़ का आकलन करता है,
ऐसे APK जिन्हें इंस्टॉल किया जा सकता है. डिफ़ॉल्ट रूप से bundletool ,
इंस्टॉल किए जा सकने वाले APK का डाउनलोड साइज़.
|
--modules=modules |
APK में विचार करने के लिए सेट किए गए मॉड्यूल की ऐसी सूची तय करता है जिसे कॉमा लगाकर अलग किया गया है
को भी मापा जा सकता है. bundletool निर्देश अपने-आप चला जाता है
इसमें किसी सेट के लिए सभी डिपेंडेंट मॉड्यूल शामिल हैं. डिफ़ॉल्ट रूप से,
कमांड, इस दौरान इंस्टॉल किए गए सभी मॉड्यूल के डाउनलोड साइज़ को मापता है
पहला डाउनलोड.
|
SDK टूल बंडल डिपेंडेंसी के साथ ऐप्लिकेशन बंडल बनाना (एक्सपेरिमेंट के तौर पर उपलब्ध)
Android SDK बंडल (ASB) डिपेंडेंसी के साथ Android ऐप्लिकेशन बंडल बनाया जा सकता है कमांड लाइन से साइन किया जा सकता है और उसका इस्तेमाल करके Jarsigner.
हर ऐप्लिकेशन बंडल मॉड्यूल में, एक मॉड्यूल प्रोटोकॉल बफ़र (.pb
) फ़ाइल शामिल होती है:
runtime_enabled_sdk_config.pb
. इस फ़ाइल में SDK टूल की सूची शामिल है
जिस पर ऐप्लिकेशन बंडल मॉड्यूल निर्भर करता है. इस फ़ाइल की पूरी जानकारी के लिए,
देखें
runtime_enabled_sdk_config.proto
फ़ाइल.
SDK टूल बंडल डिपेंडेंसी के साथ ऐप्लिकेशन बंडल बनाने के लिए, इस बारे में सेक्शन में दिया गया तरीका अपनाएं
बंडलटूल का इस्तेमाल करके ऐप्लिकेशन बंडल बनाना और
runtime_enabled_sdk_config.pb
फ़ाइल को हर ऐप्लिकेशन मॉड्यूल के ज़िप में
फ़ाइल में कोड और संसाधन शामिल हैं.
runtime_enabled_sdk_config.pb
फ़ाइल में मौजूद कुछ अहम फ़ील्ड:
सर्टिफ़िकेट का ब्यौरा: कुंजी के लिए सर्टिफ़िकेट का SHA-256 डाइजेस्ट का इस्तेमाल, SDK टूल के APK को साइन करने के लिए किया जाता है. यह
SdkMetadata.pb
फ़ाइल, Android SDK आर्काइव फ़ॉर्मैट में.संसाधन पैकेज आईडी: वह पैकेज आईडी जो इस SDK टूल में मौजूद सभी संसाधन है SDK टूल को ऐप्लिकेशन में एम्बेड करने के लिए, APKs जनरेट करते समय, उन्हें फिर से मैप किया जाता है. इससे, पुराने सिस्टम के साथ काम करने की सुविधा चालू हो जाती है.
एक SDK टूल सिर्फ़ एक मॉड्यूल में दिख सकता है. यदि अनेक मॉड्यूल एक जैसे SDK टूल, इस डिपेंडेंसी को डुप्लीकेट करके बेस मॉड्यूल में ले जाना चाहिए. अलग-अलग मॉड्यूल, SDK टूल के अलग-अलग वर्शन पर निर्भर नहीं हो सकते.
SDK टूल बंडल डिपेंडेंसी वाले ऐप्लिकेशन बंडल से APK जनरेट करें (एक्सपेरिमेंट के तौर पर उपलब्ध)
अपने ऐप्लिकेशन बंडल से APK जनरेट करने के लिए, इसके बारे में सेक्शन में दिए गए चरणों को अपनाएं
अपने ऐप्लिकेशन बंडल से APKs का सेट जनरेट करना
या के बारे में
डिवाइस के हिसाब से APK का सेट जनरेट करना
और इन SDK टूल के साथ bundletool build-apks
कमांड उपलब्ध कराएं
निर्भर करता है. ये SDK टूल, SDK टूल बंडल फ़ॉर्मैट या SDK टूल में उपलब्ध कराए जा सकते हैं
संग्रह का फ़ॉर्मैट.
आपके पास SDK टूल को SDK बंडल के तौर पर उपलब्ध कराने का विकल्प होता है. ऐसा करने के लिए, --sdk-bundles
फ़्लैग जोड़ें:
अनुसरण करता है:
bundletool build-apks --bundle=app.aab --sdk-bundles=sdk1.asb,sdk2.asb \ --output=app.apks
--sdk-archives
फ़्लैग जोड़कर, SDK टूल के संग्रह के तौर पर SDK टूल उपलब्ध कराए जा सकते हैं.
इस तरह से:
bundletool build-apks --bundle=app.aab --sdk-archives=sdk1.asar,sdk2.asar \ --output=app.apks
जिन डिवाइसों में SDK टूल की लाइब्रेरी की सुविधा नहीं है उनके लिए, SDK टूल बंडल डिपेंडेंसी वाले ऐप्लिकेशन बंडल से APK जनरेट करें
Android 13 से पहले के वर्शन वाले डिवाइसों पर, SDK टूल की लाइब्रेरी इंस्टॉल करने या उन्हें चलाने की सुविधा काम नहीं करती
का इस्तेमाल किया जा सकता है. बंडलटूल, पुराने सिस्टम के साथ काम करने की क्षमता को छिपा देता है और
जब आप एक ही ऐप्लिकेशन बंडल से आपके APK सेट के कई वैरिएंट जनरेट करते हैं
--sdk-bundles
या --sdk-archives
विकल्प के साथ bundletool build-apks
को चलाएं.
अलग-अलग वैरिएंट, अलग-अलग सुविधाओं वाले डिवाइसों को टारगेट करते हैं:
- नए डिवाइसों के लिए एक वैरिएंट उपलब्ध है, जिसमें SDK टूल को एक अलग वैरिएंट के तौर पर इंस्टॉल किया गया है ऐप्लिकेशन के पैकेज और ऐप्लिकेशन के APK में, SDK टूल से जुड़ा कोई कॉन्टेंट शामिल नहीं होता.
- पुराने डिवाइसों के लिए, एक या उससे ज़्यादा वैरिएंट उपलब्ध हैं, जिनमें SDK टूल के APKs जोड़े गए हैं स्प्लिट किए गए ऐप्लिकेशन APK के लिए भी ऐसा किया जा सकता है. SDK टूल के APK, ऐप्लिकेशन से जुड़े हैं पैकेज. इस मामले में, SDK टूल का रनटाइम, इस डिवाइस.
बिना SDK टूल की डिपेंडेंसी के ऐप्लिकेशन बंडल के लिए APK जनरेट करने के तरीके की तरह ही,
bundletool extract-apks
और bundletool install-apks
, फ़िल्टर किया गया सेट दिखाते हैं
कनेक्ट किए गए डिवाइस या उपलब्ध कराए गए डिवाइस के सबसे अच्छे वैरिएंट के APKs
कॉन्फ़िगरेशन.
बेहतर इस्तेमाल के मामलों के लिए, जहां आपकी दिलचस्पी सिर्फ़ APK स्प्लिट जनरेट करने में है
को इस्तेमाल करने के लिए, SDK टूल के संग्रह से,
bundletool build-sdk-apks-for-app
निर्देश इस तरह है:
bundletool build-sdk-apks-for-app --app-properties=app-properties.json \ --sdk-archive=sdk.asar --output=sdk.apks
app-properties
फ़ाइल में वे फ़ील्ड होने चाहिए जिनके बारे में इसमें बताया गया है
runtime_enabled_sdk_config.proto
फ़ाइल. app-properties
फ़ाइल इस तरह दिखती है:
{
"package_name": "com.my.app",
"version_code": 1234,
"min_sdk_version": 21,
"resources_package_id": 0x7e
}
bundletool build-sdk-apks-for-app
निर्देश ऐप्लिकेशन का सबसेट जनरेट करता है
ऐसे APK जो ऐप्लिकेशन के पैकेज के नाम में SDK टूल के कॉन्टेंट से मिलते-जुलते हैं. आप
इन APK को ऐसे अन्य APK के साथ मिलाना होगा जिनमें ऐप्लिकेशन का कॉन्टेंट शामिल है. उदाहरण के लिए, अगर
आपको उन्हें अलग-अलग और बढ़ाना होगा. साथ ही, डिवाइस पर एक साथ इंस्टॉल करना होगा
जो SDK टूल के रनटाइम के साथ काम नहीं करता है.
SDK टूल का बंडल बनाना और उसकी जांच करना (एक्सपेरिमेंट के तौर पर उपलब्ध है)
bundletool
का इस्तेमाल करके, ASB बनाया जा सकता है. साथ ही,
फ़ाइलें इंस्टॉल और उपलब्ध कराने के लिए ज़रूरी हैं.
SDK टूल का बंडल बनाएं
कमांड लाइन से ASB बनाया जा सकता है और उस पर हस्ताक्षर किया जा सकता है इसका उपयोग कर रहा है Jarsigner.
SDK टूल का बंडल बनाने के लिए, यह तरीका अपनाएं:
प्रोटो फ़ॉर्मैट में, SDK टूल के बंडल का मेनिफ़ेस्ट और संसाधनों को जनरेट करना इसके लिए, वही तरीका अपनाएं जो ऐप्लिकेशन बंडल के लिए इस्तेमाल किया जाता है.
अपने SDK टूल के कंपाइल किए गए कोड और संसाधनों को एक बेस ज़िप फ़ाइल में पैकेज के तौर पर इकट्ठा करना, जैसा आप किसी ऐप मॉड्यूल में करेंगे.
SdkModulesConfig.pb.json
फ़ाइल औरSdkBundleConfig.pb.json
जनरेट करें फ़ाइल, जो Android SDK बंडल की खास बातें.bundletool build-sdk-bundle
निर्देश का इस्तेमाल करके अपना ASB बनाएं: अनुसरण करता है:
bundletool build-sdk-bundle --sdk-bundle-config=SdkBundleConfig.pb.json \ --sdk-modules-config=SdkModulesConfig.pb.json \ --modules=base.zip --output=sdk.asb
नीचे दी गई टेबल में उन अलग-अलग फ़्लैग और विकल्पों के बारे में बताया गया है जिन्हें कब सेट किया जा सकता है
bundletool build-sdk-bundle
कमांड का इस्तेमाल करके, ज़्यादा जानकारी पाएं.
तीसरी टेबल.
bundletool build-sdk-bundle
निर्देश
चिह्नित करें | ब्यौरा |
---|---|
--modules |
(ज़रूरी है) वह मॉड्यूल फ़ाइल जिसे फ़ाइनल बनाना है ASB की ओर से. |
--output |
(ज़रूरी है) वह पाथ जहां आपको ASB बनाना है. |
--sdk-modules-config |
(ज़रूरी है) कॉन्फ़िगरेशन के बारे में बताने वाली JSON फ़ाइल का पाथ के सभी मॉड्यूल देखे जा सकते हैं. JSON फ़ाइल को फ़ॉर्मैट करने का तरीका जानने के लिए, यहां जाएं: Android SDK बंडल की खास बातें सेक्शन. |
--sdk-bundle-config |
JSON फ़ाइल का पाथ, जो कॉन्फ़िगरेशन के बारे में बताता है के तौर पर उपलब्ध है. JSON फ़ाइल को फ़ॉर्मैट करने का तरीका जानने के लिए, यहां जाएं: Android SDK बंडल की खास बातें सेक्शन. |
--metadata-file |
ASB के लिए मेटाडेटा शामिल करने वाली फ़ाइल.
फ़्लैग मान का फ़ॉर्मैट यह है
<bundle-path>:<physical-file> ,
जहां <bundle-path> फ़ाइल की जगह को दिखाता है
SDK टूल बंडल की मेटाडेटा डायरेक्ट्री और <physical-file>
कोई मौजूदा फ़ाइल, जिसमें स्टोर किया जाने वाला रॉ डेटा होता है. फ़्लैग ये हो सकता है:
दोहराया गया.
|
--overwrite |
अगर यह नीति सेट की जाती है, तो यह पिछले मौजूदा आउटपुट की जगह लागू हो जाती है. |
SDK टूल के बंडल से APK जनरेट करें
ASB बनाने के बाद, SDK टूल के बंडल को स्थानीय तौर पर टेस्ट किया जा सकता है. इसके लिए:
bundletool build-sdk-apks
निर्देश का इस्तेमाल करके, अपने APK जनरेट करना, जैसा कि यहां दिखाया गया है
यह कोड डालें:
bundletool build-sdk-apks --sdk-bundle=sdk.asb --output=sdk.apks
जब bundletool
आपके SDK टूल बंडल से APK जनरेट करता है, तो इस टूल में
APK सेट संग्रह नाम के कंटेनर में मौजूद APK, जिसमें .apks
फ़ाइल का इस्तेमाल किया जाता है
एक्सटेंशन चुनें. bundletool
, SDK टूल के बंडल से एक स्टैंडअलोन APK जनरेट करता है
सभी डिवाइस कॉन्फ़िगरेशन को टारगेट करती है.
अगर आपको किसी डिवाइस पर ASB को डिप्लॉय करना है, तो आपको अपने ऐप्लिकेशन की साइनिंग जानकारी, जैसा कि यहां दिए गए कमांड में दिखाया गया है:
bundletool build-sdk-apks --sdk-bundle=sdk.asb --output=sdk.apks \ --ks=keystore.jks \ --ks-pass=file:/keystore.pwd \ --ks-key-alias=KeyAlias \ --key-pass=file:/key.pwd
नीचे दी गई टेबल में उन अलग-अलग फ़्लैग और विकल्पों के बारे में बताया गया है जिन्हें कब सेट किया जा सकता है
bundletool build-sdk-apks
कमांड का इस्तेमाल करके, ज़्यादा जानकारी पाएं.
टेबल 4.
bundletool build-sdk-apks
निर्देश
चिह्नित करें | ब्यौरा |
---|---|
--sdk-bundle |
(ज़रूरी है) SDK टूल के बंडल का पाथ. एक्सटेंशन होना ज़रूरी है
.asb .
|
--output |
(ज़रूरी है) डिफ़ॉल्ट रूप से, वह पाथ जहां आप APK सेट संग्रह चाहते हैं
बनाया जाना चाहिए. वैकल्पिक रूप से, अगर आपको
--output-format=DIRECTORY ,
यह उस डायरेक्ट्री का पाथ है जहां आपको जनरेट किए गए APKs सेव करने हैं.
|
--ks |
कीस्टोर का वह पाथ जिसका इस्तेमाल आपको जनरेट किए गए APK हैं. |
--ks-key-alias |
हस्ताक्षर करने के लिए कीस्टोर में इस्तेमाल करने के लिए कुंजी का उपनाम जनरेट किए गए APK हैं. |
--key-pass |
कीस्टोर में मौजूद कुंजी का पासवर्ड, जिसका इस्तेमाल जनरेट किए गए पासकोड को साइन करने के लिए किया जा सकता है APK. अगर पासवर्ड को साफ़ टेक्स्ट में पास किया जाता है, तो आपको वैल्यू से पहले पुष्टि करनी होगी
अगर यह फ़्लैग सेट नहीं है, तो कीस्टोर पासवर्ड की कोशिश की गई है. अगर ऐसा नहीं होता है, तो कमांड-लाइन टर्मिनल आपको डालें. |
--ks-pass |
कीस्टोर का पासवर्ड, जिसका इस्तेमाल जनरेट किए गए APK पर साइन करने के लिए किया जाना है. अगर पासवर्ड को साफ़ टेक्स्ट में पास किया जाता है, तो आपको वैल्यू से पहले पुष्टि करनी होगी
अगर यह फ़्लैग सेट नहीं है, तो कमांड-लाइन टर्मिनल, आपसे पासवर्ड डालने का प्रॉम्प्ट करता है. |
--aapt2 |
इस्तेमाल करने के लिए, AAPT2 बाइनरी का पाथ. |
--output-format |
जनरेट किए गए APKs के लिए आउटपुट फ़ॉर्मैट. डिफ़ॉल्ट रूप से, यह विकल्प
APK_SET , जो APK सेट वाले संग्रह में APK को आउटपुट करता है.
बनाया गया. अगर इसे DIRECTORY पर सेट किया जाता है, तो यह APK को
--output के ज़रिए बताई गई डायरेक्ट्री. |
--verbose |
अगर सेट किया जाता है, तो यह विकल्प स्टैंडर्ड आउटपुट. |
--version-code |
SDK टूल का वर्शन कोड. Android प्लैटफ़ॉर्म इस वर्शन कोड का इस्तेमाल करता है APK इंस्टॉल करने के लिए ज़रूरी है, SDK टूल के वर्शन के लिए नहीं. इस विकल्प को आर्बिट्रेरी वैल्यू का इस्तेमाल करें. अगर यह नीति सेट नहीं है, तो इसकी डिफ़ॉल्ट वैल्यू 0 होती है. |
--overwrite |
अगर यह नीति सेट की जाती है, तो यह पिछले मौजूदा आउटपुट की जगह लागू हो जाती है. |
SDK टूल के APKs को डिप्लॉय करना, एक्सट्रैक्ट करना, और उनके साइज़ का आकलन करना
आप उन्हीं चरणों का पालन कर सकते हैं जिनका उपयोग ऐप्लिकेशन के कनेक्ट किए गए डिवाइस पर APKs, अलग-अलग डिवाइस के हिसाब से एक्सट्रैक्ट करें मौजूदा APK सेट के APKs और अनुमानित डेटा का आकलन करें APK सेट में APKs का साइज़ डाउनलोड करने के लिए.
SDK टूल के बंडल से SDK टूल का संग्रह जनरेट करें
अपने डिस्ट्रिब्यूशन चैनल पर एएसबी अपलोड करने के बाद,
उदाहरण के लिए, Google Play में ASB को Android SDK टूल में बदल दिया गया है
Maven के ज़रिए ऐप्लिकेशन डेवलपर को उपलब्ध कराने के लिए, संग्रहित करें (.asar
). ज़्यादा के लिए
इस बारे में जानकारी पाने के लिए,
SDK टूल संग्रह के फ़ॉर्मैट की खास बातें.
अपना ASB बनाने के बाद, आपके पास
bundletool build-sdk-asar
निर्देश का इस्तेमाल करके, इस तरह से Android SDK टूल को स्थानीय तौर पर संग्रहित करें
निम्न कोड में दिखाया गया है:
bundletool build-sdk-asar --sdk-bundle=sdk.asb --output=sdk.asar \ --apk-signing-key-certificate=keycert.txt
नीचे दी गई टेबल में उन अलग-अलग फ़्लैग और विकल्पों के बारे में बताया गया है जिन्हें कब सेट किया जा सकता है
bundletool build-sdk-asar
कमांड का इस्तेमाल करके, ज़्यादा जानकारी पाएं.
पांचवीं टेबल.
bundletool build-sdk-asar
निर्देश
चिह्नित करें | ब्यौरा |
---|---|
--apk-signing-key-certificate |
(ज़रूरी है) SDK टूल के APK साइनिंग सर्टिफ़िकेट का पाथ. यह है
का प्रमाणपत्र, जिसका उपयोग आपने
build-sdk-apks निर्देश.
|
--output |
(ज़रूरी है) वह पाथ जहां आपको .asar फ़ाइल को रखना है
बनाया गया.
|
--sdk-bundle |
(ज़रूरी है) SDK टूल के बंडल का पाथ. एक्सटेंशन होना ज़रूरी है
.asb .
|
--overwrite |
अगर यह नीति सेट की जाती है, तो यह पिछले मौजूदा आउटपुट की जगह लागू हो जाती है. |
रनटाइम के साथ काम करने वाले एसडीके फ़ॉर्मैट (एक्सपेरिमेंट के तौर पर उपलब्ध)
रनटाइम के साथ काम करने वाले SDK टूल के लिए, Android के दो फ़ाइल फ़ॉर्मैट उपलब्ध होते हैं:
- Android SDK बंडल (
.asb
), इसका इस्तेमाल रनटाइम के साथ काम करने वाला SDK टूल जोड़ दिया गया है. - Android SDK आर्काइव (
.asar
), इसका इस्तेमाल Maven पर रनटाइम के साथ काम करने वाला SDK टूल.
Android SDK बंडल फ़ॉर्मैट
SDK बंडल, रनटाइम के साथ काम करने वाले SDK टूल के लिए पब्लिश किया जाने वाला फ़ॉर्मैट है. इसमें सभी चीज़ें शामिल हैं SDK टूल का कोड और संसाधन. इसमें, SDK टूल की किसी लाइब्रेरी का कोड भी शामिल होता है निर्भर करता है. इसमें रनटाइम के साथ काम करने वाले दूसरे रनटाइम के कोड और संसाधन शामिल नहीं होते SDK टूल जिन पर निर्भर करता है.
Android SDK बंडल (ASB), एक्सटेंशन वाली एक साइन की गई ZIP फ़ाइल होती है
.asb
. SDK टूल के कोड और संसाधनों को उसी तरह व्यवस्थित किया जाता है जिस तरह
किसी APK में ढूंढें. ASB में कई कॉन्फ़िगरेशन फ़ाइलें होती हैं, जो
इंस्टॉल किए जा सकने वाले APK जनरेट करते हैं.
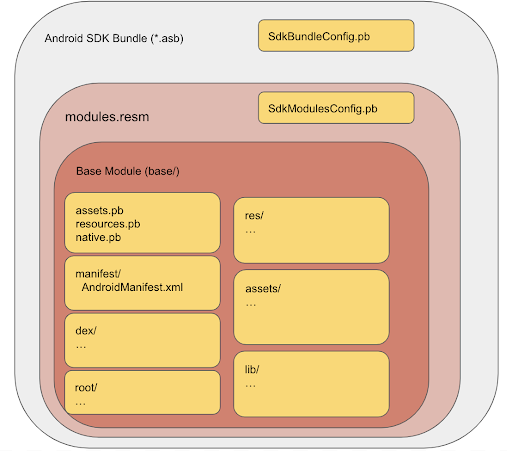
नीचे दी गई सूची में कुछ ASB फ़ाइलों के बारे में बताया गया है विवरण:
SdkBundleConfig.pb
: प्रोटो फ़ॉर्मैट में एक कॉन्फ़िगरेशन फ़ाइल, जिसमें रनटाइम के साथ काम करने वाले SDK टूल की सूची होनी चाहिए जिस पर आपका SDK टूल निर्भर करता है. पूरे के बारे में जानने के लिए,sdk_bundle_config.proto
फ़ाइल से लिए जाते हैं.modules.resm
: एक ज़िप फ़ाइल जिसमें SDK टूल के APKs.SdkModulesConfig.pb
: प्रोटो फ़ॉर्मैट में एक कॉन्फ़िगरेशन फ़ाइल. यह फ़ाइल इसमें SDK टूल के एंट्री पॉइंट का नाम, वर्शन, और क्लास का नाम शामिल होता है फ़्रेमवर्क (SandboxedSdkProvider
). पूरी परिभाषा जानने के लिए, यहां देखें:sdk_modules_config.proto
फ़ाइल का इस्तेमाल किया जाएगा.base/
: एक मॉड्यूल, जिसमें SDK टूल का कोड और संसाधन होते हैं.manifest/
: प्रोटो फ़ॉर्मैट में SDK टूल का मेनिफ़ेस्ट.dex/
: DEX फ़ॉर्मैट में कंपाइल किया गया कोड. एक से ज़्यादा DEX फ़ाइलें दी जा सकती हैं.res/
,lib/
,assets/
: ये डायरेक्ट्री, सामान्य APK. जनरेट करते समय इन डायरेक्ट्री में मौजूद पाथ सुरक्षित रखे जाते हैं: SDK टूल के APKs.root/
: यह डायरेक्ट्री उन फ़ाइलों को सेव करती है जिन्हें बाद में रूट किया जाता है APK फ़ॉर्मैट में बदलें. उदाहरण के लिए, इसमें Java-आधारित ऐसे संसाधन शामिल हो सकते हैं जो आपका SDK टूल,Class.getResource()
तरीका. इस डायरेक्ट्री में मौजूद पाथ भी सुरक्षित रखे गए हैं.
BUNDLE-METADATA
: इस डायरेक्ट्री में, ऐसी मेटाडेटा फ़ाइलें शामिल हैं जिनमें टूल या ऐप स्टोर के लिए उपयोगी जानकारी हो. ऐसी मेटाडेटा फ़ाइलें इसमें ProGuard मैपिंग और अपने SDK टूल की DEX फ़ाइलों की पूरी सूची शामिल होती है. इस डायरेक्ट्री में मौजूद फ़ाइलें, आपके SDK टूल के APKs में पैकेज नहीं की जाती हैं.
Android SDK आर्काइव का फ़ॉर्मैट
Android SDK आर्काइव, रनटाइम के साथ काम करने वाले एसडीके का डिस्ट्रिब्यूशन फ़ॉर्मैट होता है. यह
मेवन. यह एक ZIP फ़ाइल है, जिसका फ़ाइल एक्सटेंशन .asar
है. फ़ाइल
इसमें ऐसी सारी जानकारी शामिल है जो ऐप्लिकेशन बनाने वाले टूल को
एक Android ऐप्लिकेशन बंडल होना चाहिए, जो रनटाइम के साथ काम करने वाले आपके SDK टूल पर निर्भर करता हो.
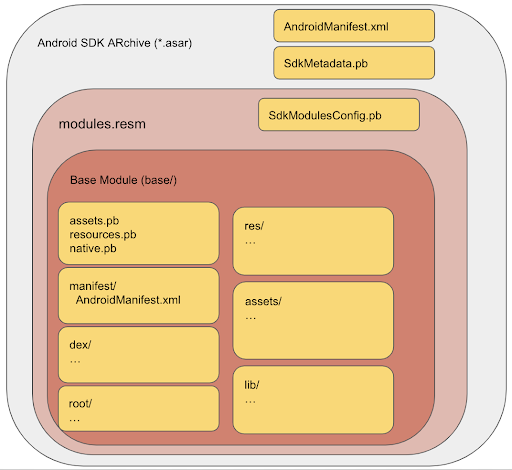
नीचे दी गई सूची में, Android SDK Archive की कुछ फ़ाइलों के बारे में बताया गया है विवरण:
SdkMetadata.pb
: प्रोटो फ़ॉर्मैट में एक कॉन्फ़िगरेशन फ़ाइल जिसमें SDK टूल का नाम, वर्शन, और हस्ताक्षर करने के लिए इस्तेमाल की गई कुंजी का सर्टिफ़िकेट डाइजेस्ट इस SDK टूल के लिए जनरेट किए गए APKs. पूरी परिभाषा जानने के लिए, यहां देखें:sdk_metadata.proto
फ़ाइल से लिए जाते हैं.modules.resm
: एक ज़िप फ़ाइल जिसमें SDK टूल के APKs. यह.resm
Android SDK बंडल.AndroidManifest.xml
: SDK टूल की मेनिफ़ेस्ट फ़ाइल, जो टेक्स्ट एक्सएमएल फ़ॉर्मैट में होती है.
अन्य संसाधन
bundletool
इस्तेमाल करने के बारे में ज़्यादा जानने के लिए, यह वीडियो देखें
ऐप्लिकेशन बंडल: बंडलटूल और Play Console के साथ टेस्टिंग बंडल.