To create a Kotlin Multiplatform (KMP) module within your Android project, use the Kotlin Multiplatform Shared Module template, available in Android Studio Meerkat and Android Gradle Plugin version 8.8.0 and higher.
The module template automates the creation of a new module with the minimum configuration targeting Android and iOS platforms.
Set up the shared KMP module
To create a shared KMP module, follow these steps:
- Select File > New > New Module
- Select the Kotlin Multiplatform Shared Module template in the Templates panel:
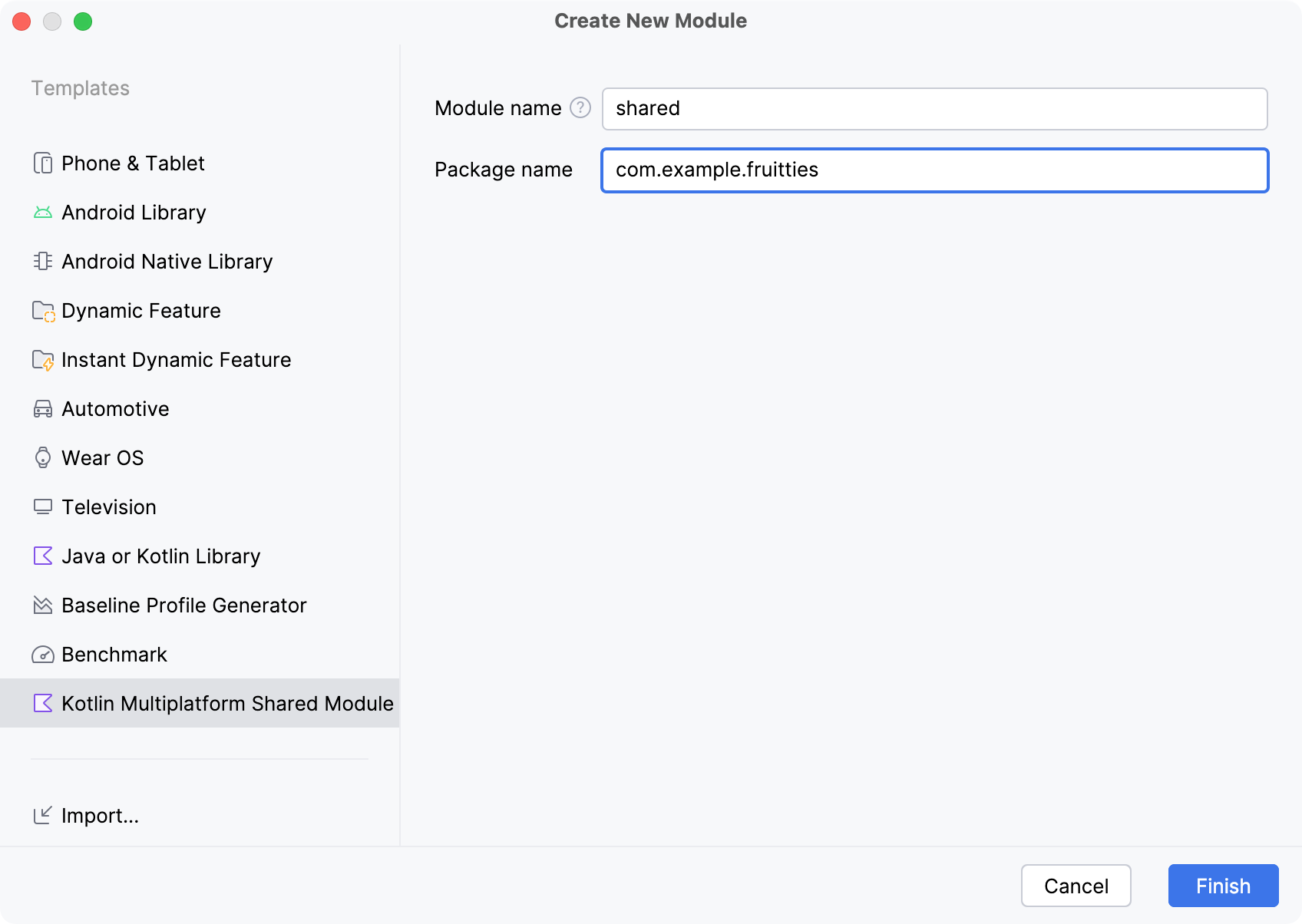
The fields in the template are the following:
- Module name – defines the Gradle module name, as well as the iOS framework name (can be changed later)
- Package name – defines the package name for files in this module
- Click Finish and allow Gradle to sync with the project. You might also be prompted to add the newly created module files to source control.
Once complete, the Android Studio Project View shows the new shared module
along with a sourceset for each platform.
Link the shared module to the Android app
The module wizard doesn't add the newly created module as a dependency to any existing module. As a next step, you need to link the shared module to one of your existing Gradle modules similarly to other Android dependencies
dependencies {
...
implementation(project(":shared"))
}
Once enabled, you can access code as usual. From the Android app, you're able to access code that is available either in androidMain or in commonMain.
For more information on Kotlin Multiplatform project structure, see the basics of Kotlin Multiplatform project structure
Set up the shared module to the iOS app
Swift can't use Kotlin modules directly and requires a compiled binary framework to be produced.
The new module template in Android Studio configures the shared module to
produce a framework for each of the iOS architectures. You can find the
following code in the shared module's build.gradle.kts
file:
val xcfName = "sharedKit"
iosX64 {
binaries.framework {
baseName = xcfName
}
}
iosArm64 {
binaries.framework {
baseName = xcfName
}
}
iosSimulatorArm64 {
binaries.framework {
baseName = xcfName
}
}
See Hierarchical project structure for information on defining other architecture types.
Link the shared library in the iOS project
To enable access to the shared code from the iOS project, add a script phase to generate the Kotlin framework before compiling the Swift sources:
- Right-click the file in the Android Studio and select Open In and Open in Associated Application. This will open the iOS app in Xcode.
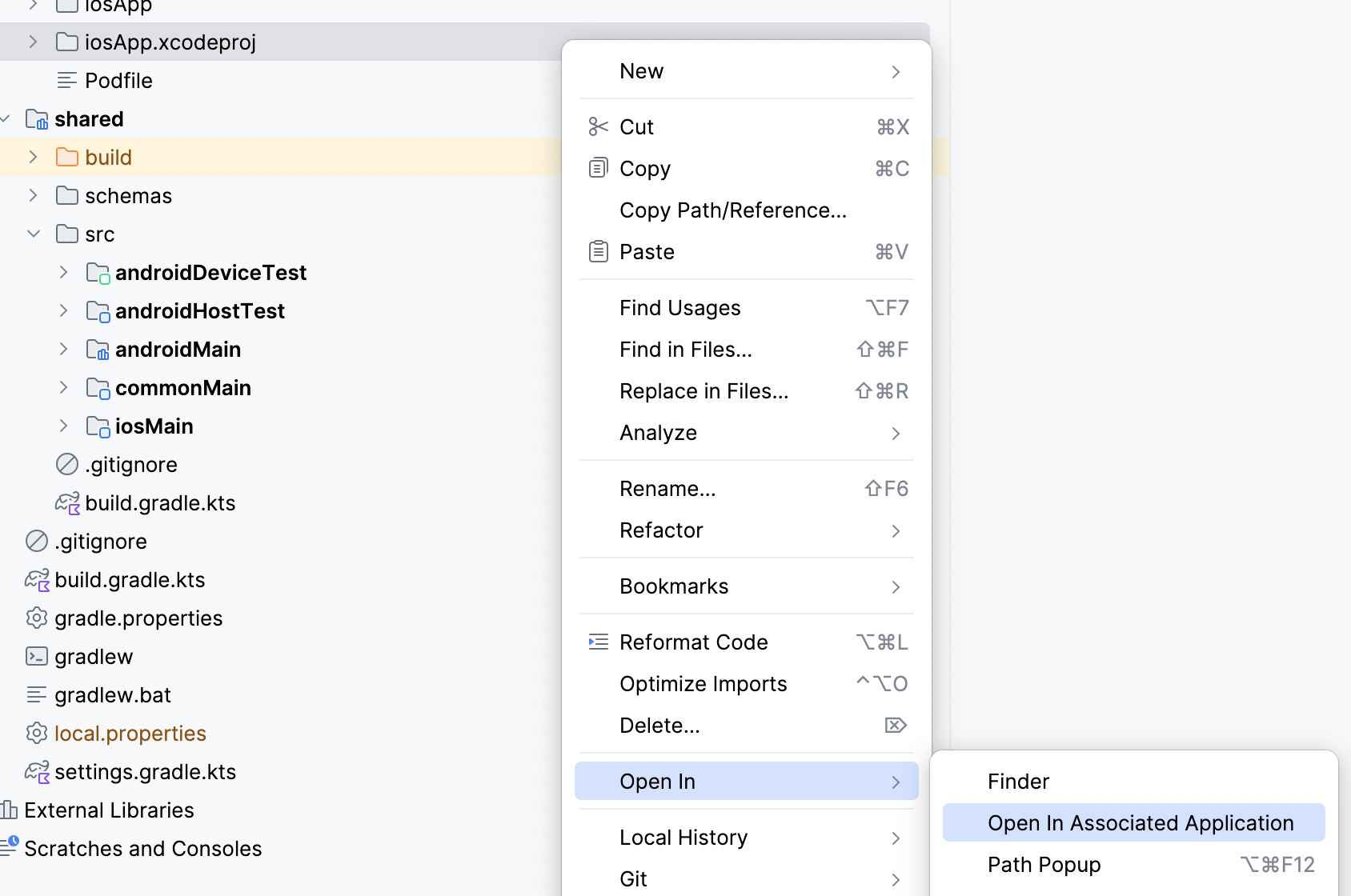
- Open the project settings by double-clicking the project name in the project navigator
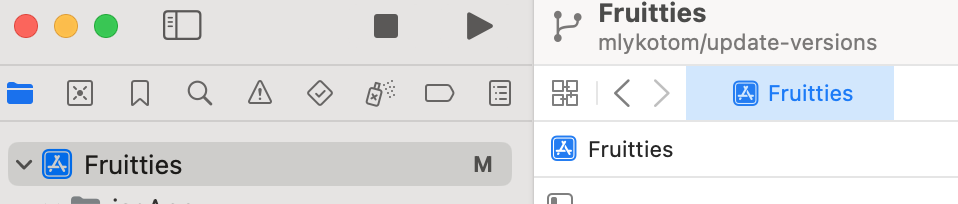
- Change the default Run Script name to Compile Kotlin Framework to better identify what this phase does. Double-click the Run Script title to edit it.
- Expand the build phase, and in the Shell text field, enter the following script code:
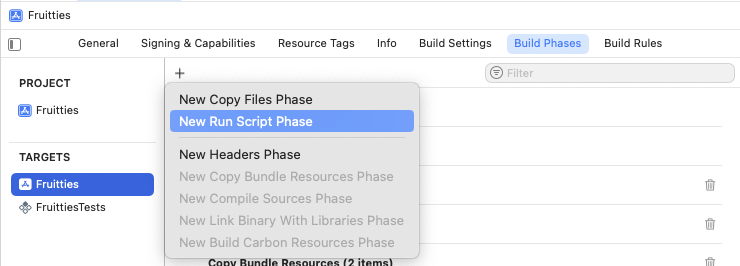
Drag the Run Script phase before the Compile Sources phase.
Figure 6. Run script build phase before compile sources Build the project in Xcode by clicking ⌘B or navigating to the Product menu and selecting Build.
When the build succeeds, you'll see the following icon.
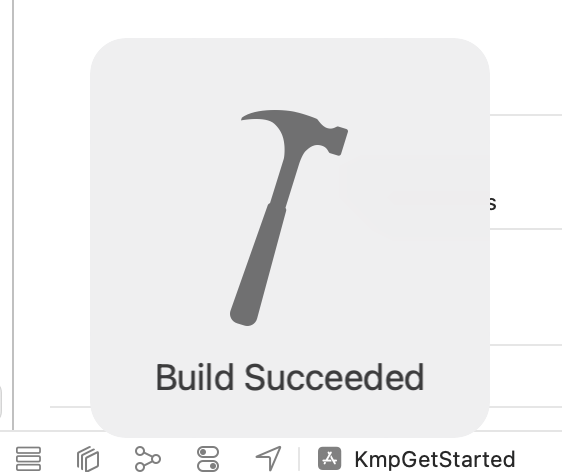
Access the shared code in the iOS app
To verify that the iOS app can successfully access code from the shared module, do the following:
- In the iOS project, open the
ContentView.swift
file at:Sources/View/ContentView.swift
- Add import
sharedKit
at the top of the file. - Modify the Text view to include the
Platform_iosKt.platform()
information in the displayed string as follows:
This update checks whether the Fruitties app can call the platform()
function
from the shared module, which should return "iOS" when running on the iOS
platform.
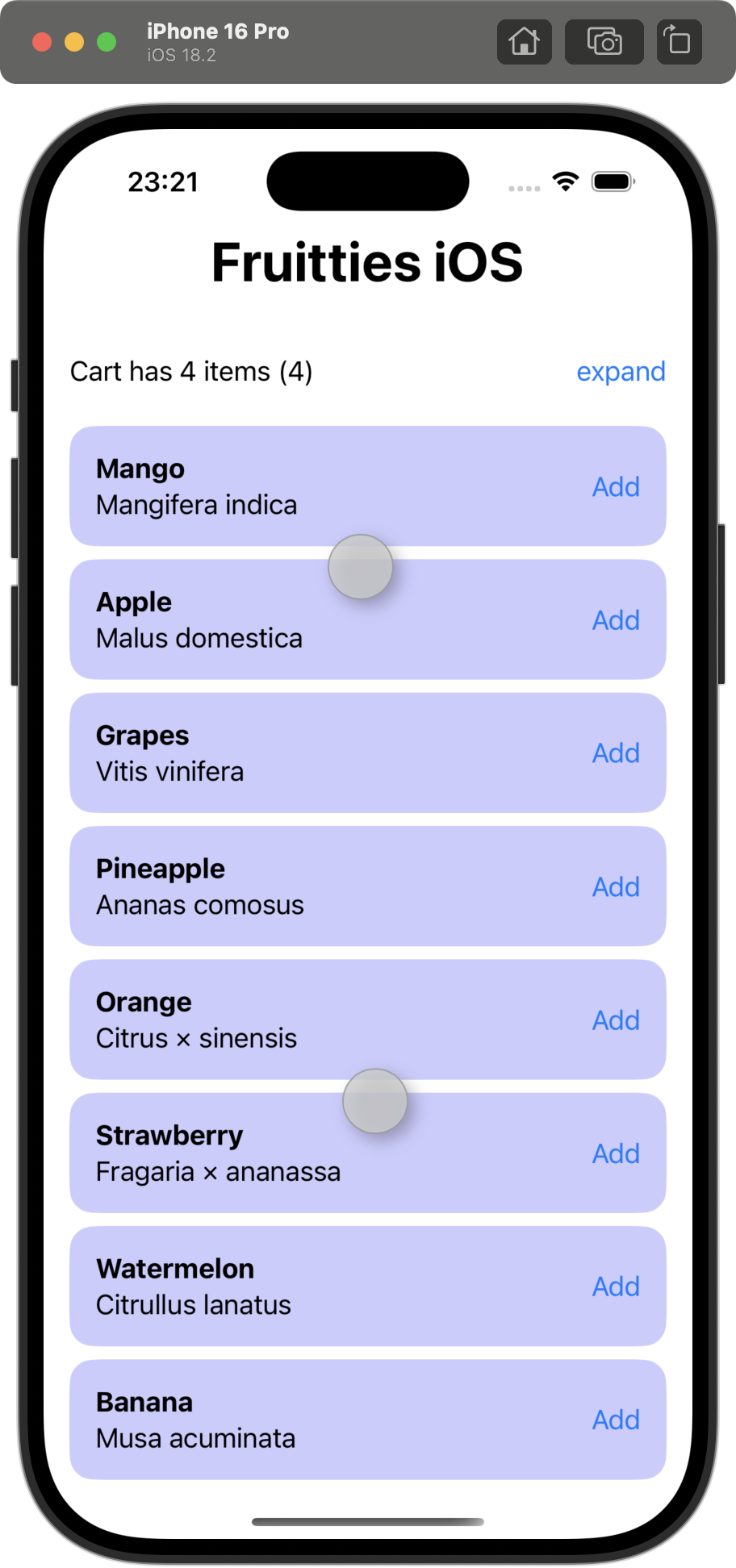
Additional Resources
If you're new to KMP development, see the official KMP documentation for more guides. If you're new to iOS development, see Swift Basics documentation..