Hầu hết trải nghiệm trên nhiều thiết bị đều bắt đầu bằng việc tìm thiết bị có sẵn. Để đơn giản hoá tác vụ thường dùng này, chúng tôi cung cấp API Khám phá thiết bị.
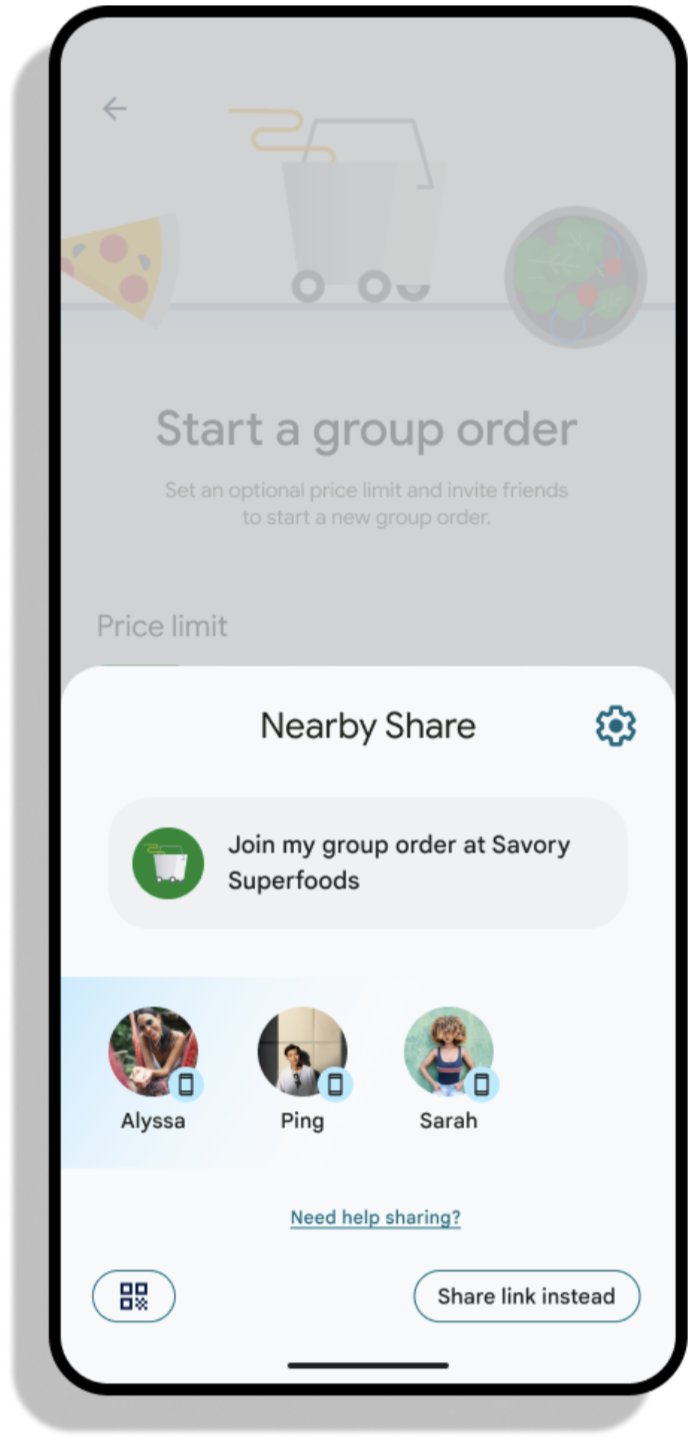
Chạy hộp thoại chọn thiết bị
Tính năng Khám phá thiết bị sử dụng hộp thoại hệ thống để cho phép người dùng chọn thiết bị mục tiêu. Để bắt đầu hộp thoại chọn thiết bị, trước tiên, bạn cần tải một ứng dụng khám phá thiết bị và đăng ký bộ nhận kết quả. Lưu ý tương tự như registerForActivityResult
, receiver này phải được đăng ký vô điều kiện trong đường dẫn khởi chạy mảnh hoặc hoạt động.
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); devicePickerLauncher = Discovery.create(this).registerForResult(this, handleDevices); }
Trong đoạn mã ở trên, chúng ta có một đối tượng handleDevices
không xác định. Sau khi người dùng chọn thiết bị để kết nối và sau khi SDK kết nối thành công với các thiết bị khác, lệnh gọi lại này sẽ nhận được danh sách Participants
đã chọn.
Kotlin
handleDevices = OnDevicePickerResultListener { participants -> participants.forEach { // Use participant info } }
Java
handleDevices = participants -> { for (Participant participant : participants) { // Use participant info } }
Sau khi đăng ký bộ chọn thiết bị, hãy chạy bộ chọn đó bằng cách sử dụng thực thể devicePickerLauncher
. DevicePickerLauncher.launchDevicePicker
nhận hai tham số – danh sách bộ lọc thiết bị (xem phần dưới đây) và startComponentRequest
. startComponentRequest
dùng để cho biết hoạt động nào cần được bắt đầu trên thiết bị nhận và lý do yêu cầu hiển thị với người dùng.
Kotlin
devicePickerLauncher.launchDevicePicker( listOf(), startComponentRequest { action = "com.example.crossdevice.MAIN" reason = "I want to say hello to you" }, )
Java
devicePickerLauncher.launchDevicePickerFuture( Collections.emptyList(), new StartComponentRequest.Builder() .setAction("com.example.crossdevice.MAIN") .setReason("I want to say hello to you") .build());
Chấp nhận yêu cầu kết nối
Khi người dùng chọn một thiết bị trong bộ chọn thiết bị, một hộp thoại sẽ xuất hiện trên thiết bị nhận để yêu cầu người dùng chấp nhận kết nối. Sau khi được chấp nhận, hoạt động mục tiêu sẽ chạy. Bạn có thể xử lý hoạt động này trong onCreate
và onNewIntent
.
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) handleIntent(getIntent()) } override fun onNewIntent(intent: Intent) { super.onNewIntent(intent) handleIntent(intent) } private fun handleIntent(intent: Intent) { val participant = Discovery.create(this).getParticipantFromIntent(intent) // Accept connection from participant (see below) }
Java
@Override public void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); handleIntent(getIntent()); } @Override public void onNewIntent(Intent intent) { super.onNewIntent(intent); handleIntent(intent); } private void handleIntent(Intent intent) { Participant participant = Discovery.create(this).getParticipantFromIntent(intent); // Accept connection from participant (see below) }
Bộ lọc thiết bị
Khi khám phá thiết bị, thông thường, bạn nên lọc các thiết bị này để chỉ hiện những thiết bị liên quan đến trường hợp sử dụng. Ví dụ:
- Chỉ lọc xem các thiết bị có máy ảnh để giúp quét mã QR
- Chỉ lọc xem TV để có trải nghiệm xem trên màn hình lớn
Đối với bản dùng thử này cho nhà phát triển, chúng tôi đang bắt đầu với khả năng lọc theo các thiết bị của cùng một người dùng.
Bạn có thể chỉ định bộ lọc thiết bị bằng cách dùng lớp DeviceFilter
:
Kotlin
val deviceFilters = listOf(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY))
Java
List<DeviceFilter> deviceFilters = Arrays.asList(DeviceFilter.trustRelationshipFilter(MY_DEVICES_ONLY));
Sau khi xác định bộ lọc thiết bị, bạn có thể bắt đầu tính năng khám phá thiết bị.
Kotlin
devicePickerLauncher.launchDevicePicker(deviceFilters, startComponentRequest)
Java
Futures.addCallback( devicePickerLauncher.launchDevicePickerFuture(deviceFilters, startComponentRequest), new FutureCallback<Void>() { @Override public void onSuccess(Void result) { // do nothing, result will be returned to handleDevices callback } @Override public void onFailure(Throwable t) { // handle error } }, mainExecutor);
Lưu ý rằng launchDevicePicker
là một hàm không đồng bộ sử dụng từ khoá suspend
.