Following the deprecation of the
Google Sign-In
API, we are removing the games v1 SDK in 2026. After February 2025, you will be unable to publish
titles that are newly integrated with games v1 SDK, on Google Play. We recommend that you use the
games v2 SDK instead.
While existing titles with the previous games v1 integrations continue to function for a
couple of years, you are encouraged to
migrate to v2
starting June 2025.
This guide is for using the Play Games Services v1 SDK. For information
on the latest SDK version, see the
v2 documentation.
This guide shows you how to use leaderboards APIs in an Android application
to create visual leaderboards, record a player's score, and compare the score
against the player's score from previous game sessions. The APIs can be found
in the com.google.android.gms.games
and com.google.android.gms.games.leaderboards
packages.
Before you begin
If you haven't already done so, you might find it helpful to review the leaderboards game concepts.
Before you start to code using the leaderboards APIs:
- Follow the instructions for installing and setting up your app to use Google Play Games Services in the Set Up Google Play Services SDK guide.
- Define the leaderboards that you want your game to display or update, by following the instructions in the Google Play Console guide.
- Download and review the leaderboards code samples in the Android samples page.
- Familiarize yourself with the recommendations described in Quality Checklist.
Get the leaderboards client
To start using the leaderboards API, your game must first obtain a LeaderboardsClient
object.
You can do this by calling the Games.getLeadeboardsClient()
method and passing in the
activity and the GoogleSignInAccount
for the current player. To learn how to retrieve the
player account information, see Sign-in in Android Games.
Update the player's score
When the player's score changes (for example, when the player finishes the game), your
game can update their score on the leaderboard by calling LeaderboardsClient.submitScore()
,
and passing in the leaderboard ID and the raw score value.
The following code snippet shows how your app can update the player’s score:
Games.getLeaderboardsClient(this, GoogleSignIn.getLastSignedInAccount(this)) .submitScore(getString(R.string.leaderboard_id), 1337);
A good practice is to define the leaderboard ID in your strings.xml
file, so
your game can reference the leaderboards by resource ID. When making calls to
update and load player scores, make sure to also follow these
best practices to avoid exceeding your API quota.
Display a leaderboard
To display leaderboard, call LeaderboardsClient.getLeaderboardIntent()
to get an
Intent
to
create the default leaderboard user interface. Your game can then bring up the UI by calling
startActivityForResult
.
The following code snippet shows how your app can update the player’s score. In the
code snippet, RC_LEADERBOARD_UI
is an arbitrary integer for the request code.
private static final int RC_LEADERBOARD_UI = 9004; private void showLeaderboard() { Games.getLeaderboardsClient(this, GoogleSignIn.getLastSignedInAccount(this)) .getLeaderboardIntent(getString(R.string.leaderboard_id)) .addOnSuccessListener(new OnSuccessListener<Intent>() { @Override public void onSuccess(Intent intent) { startActivityForResult(intent, RC_LEADERBOARD_UI); } }); }
Notice that even though no result is returned, we have to use
startActivityForResult
so that the API can obtain the identity of the calling package. An example of the default
leaderboard UI is shown below.
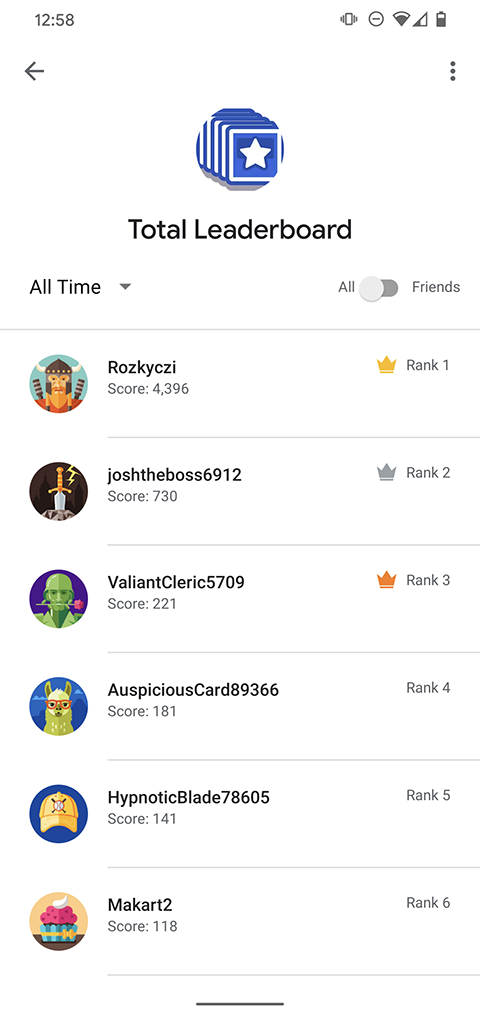