This guide shows you how to use the achievements APIs in an Android application
to unlock and display achievements in your game. The APIs can be found
in the com.google.android.gms.games
and com.google.android.gms.games.achievements
packages.
Before you begin
If you haven't already done so, you might find it helpful to review the achievements game concepts.
Before you start to code using the achievements API:
- Follow the instructions for installing and setting up your app to use Google Play Games Services in the Set Up Google Play Services SDK guide.
- Define the achievements that you want your game to unlock or display, by following the instructions in the Google Play Console guide.
- Download and review the achievements code samples in the Android samples page.
- Familiarize yourself with the recommendations described in Quality Checklist.
Get an achievements client
To start using the achievements API, your game must first obtain an
AchievementsClient
object. You can do this by calling the
Games.getAchievementClient()
method and passing in the activity and the
GoogleSignInAccount
for the current player. To learn how to retrieve the
player account information, see Sign-in in Android Games.
Unlock achievements
To unlock an achievement, call the AchievementsClient.unlock()
method and
pass in the achievement ID.
The following code snippet shows how your app can unlock achievements:
Games.getAchievementsClient(this, GoogleSignIn.getLastSignedInAccount(this)) .unlock(getString(R.string.my_achievement_id));
If the achievement is of the incremental type (that is, several steps are required to
unlock it), call AchievementsClient.increment()
instead.
The following code snippet shows how your app can increment the player’s achievement:
Games.getAchievementsClient(this, GoogleSignIn.getLastSignedInAccount(this)) .increment(getString(R.string.my_achievement_id), 1);
You do not need to write additional code to unlock the achievement; Google Play Games Services automatically unlocks the achievement once it reaches its required number of steps.
A good practice is to define the achievement IDs in the strings.xml
file, so
your game can reference the achievements by resource ID. When making calls to update and load
achievements, make sure to also follow these best practices to
avoid exceeding your API quota.
Display achievements
To show a player's achievements, call AchievementsClient.getAchievementsIntent()
to get an Intent
to create
the default achievements user interface. Your game can then bring up the UI by calling
startActivityForResult
.
The following code snippet shows how your app can display the default achievement user interface.
In the snippet, RC_ACHIEVEMENT_UI
is an arbitrary integer that the game uses as the request code.
private static final int RC_ACHIEVEMENT_UI = 9003; private void showAchievements() { Games.getAchievementsClient(this, GoogleSignIn.getLastSignedInAccount(this)) .getAchievementsIntent() .addOnSuccessListener(new OnSuccessListener<Intent>() { @Override public void onSuccess(Intent intent) { startActivityForResult(intent, RC_ACHIEVEMENT_UI); } }); }
An example of the default achievements UI is shown below.
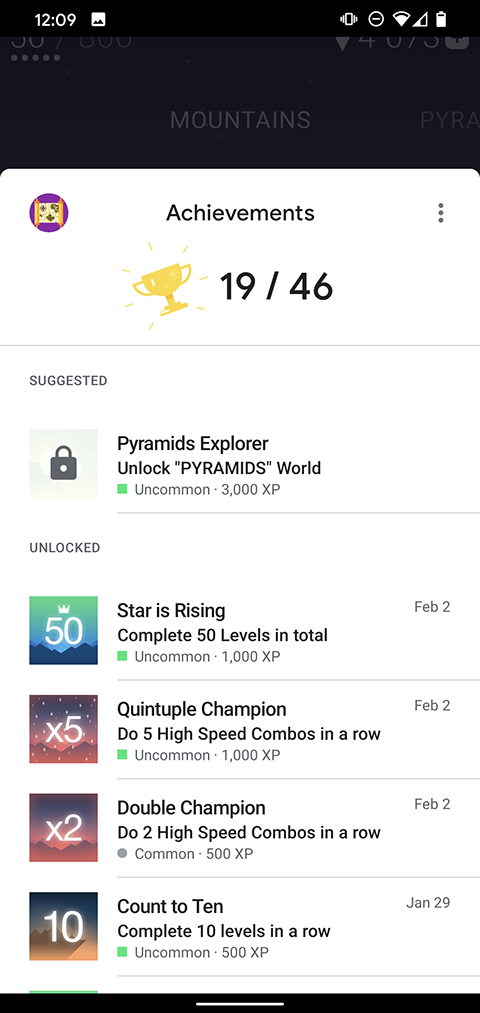