사용자는 스타일러스를 사용하여 앱에서 그리거나 쓰거나 상호작용할 때 손바닥으로 화면을 터치하기도 합니다. 시스템이 이러한 이벤트를 의도치 않은 손바닥 터치로 인식하여 무시하기 전에 터치 이벤트가 앱에 보고될 수 있습니다.
손바닥 터치를 식별하고 무시
앱은 관련 없는 터치 이벤트를 식별하여 무시해야 합니다. Android는 MotionEvent
객체를 앱에 전달하여 손바닥 터치를 취소합니다.
앱에 전달된
MotionEvent
객체를 검사합니다.MotionEvent
API를 사용하여 이벤트 속성 (작업 및 플래그)을 판단합니다.- 단일 포인터 이벤트:
ACTION_CANCEL
를 확인합니다. Android 13 이상에서는FLAG_CANCELED
도 확인합니다. - 멀티 포인터 이벤트: Android 13 이상에서는
ACTION_POINTER_UP
및FLAG_CANCELED
를 확인합니다.
- 단일 포인터 이벤트:
ACTION_CANCEL
및ACTION_POINTER_UP
/FLAG_CANCELED
속성이 있는 움직임 감지 이벤트를 무시합니다.
1. 모션 이벤트 객체 획득
앱에 OnTouchListener
를 추가합니다.
Kotlin
val myView = findViewById<View>(R.id.myView).apply { setOnTouchListener { view, event -> // Process motion event. } }
Java
View myView = findViewById(R.id.myView); myView.setOnTouchListener( (view, event) -> { // Process motion event. });
2. 이벤트 작업 및 플래그 확인
모든 API 수준에서 단일 포인터 이벤트를 나타내는 ACTION_CANCEL
을 확인합니다. Android 13 이상에서는 ACTION_POINTER_UP
에서 FLAG_CANCELED.
를 확인합니다.
Kotlin
val myView = findViewById<View>(R.id.myView).apply { setOnTouchListener { view, event -> when (event.actionMasked) { MotionEvent.ACTION_CANCEL -> { //Process canceled single-pointer motion event for all SDK versions. } MotionEvent.ACTION_POINTER_UP -> { if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU && (event.flags and MotionEvent.FLAG_CANCELED) == MotionEvent.FLAG_CANCELED) { //Process canceled multi-pointer motion event for Android 13 and higher. } } } true } }
Java
View myView = findViewById(R.id.myView); myView.setOnTouchListener( (view, event) -> { switch (event.getActionMasked()) { case MotionEvent.ACTION_CANCEL: // Process canceled single-pointer motion event for all SDK versions. case MotionEvent.ACTION_UP: if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.TIRAMISU && (event.getFlags() & MotionEvent.FLAG_CANCELED) == MotionEvent.FLAG_CANCELED) { //Process canceled multi-pointer motion event for Android 13 and higher. } } return true; });
3. 동작 실행취소
손바닥 터치를 식별한 후에는 동작이 화면에 미치는 효과를 실행취소할 수 있습니다.
손바닥 터치와 같은 의도하지 않은 입력이 실행취소될 수 있도록 앱에서는 사용자 작업 기록을 유지해야 합니다. 기록을 유지하는 방법의 예는 Android 앱에서 스타일러스 지원 개선 Codelab의 기본 그리기 앱 구현을 참고하세요.
핵심사항
MotionEvent
: 터치 및 이동 이벤트를 나타냅니다. 이벤트를 무시할지 결정하는 데 필요한 정보가 포함되어 있습니다.OnTouchListener#onTouch()
:MotionEvent
객체를 수신합니다.MotionEvent#getActionMasked()
: 모션 이벤트와 관련된 작업을 반환합니다.ACTION_CANCEL
: 동작이 실행취소되어야 함을 나타내는MotionEvent
상수입니다.ACTION_POINTER_UP
: 첫 번째 포인터 이외의 포인터가 소실되었음을, 즉 기기 화면과의 접촉을 포기했음을 나타내는MotionEvent
상수입니다.FLAG_CANCELED
: 포인터가 위로 올라가 의도치 않은 터치 이벤트가 발생했음을 나타내는MotionEvent
상수입니다. Android 13 (API 수준 33) 및 이후 버전의ACTION_POINTER_UP
및ACTION_CANCEL
이벤트에 추가되었습니다.
결과
이제 앱은 Android 13 이상 API 수준의 멀티 포인터 이벤트와 모든 API 수준의 단일 포인터 이벤트에서 손바닥 터치를 식별하고 무시할 수 있습니다.
이 가이드가 포함된 컬렉션
이 가이드는 더 광범위한 Android 개발 목표를 다루는 선별된 빠른 가이드 모음의 일부입니다.
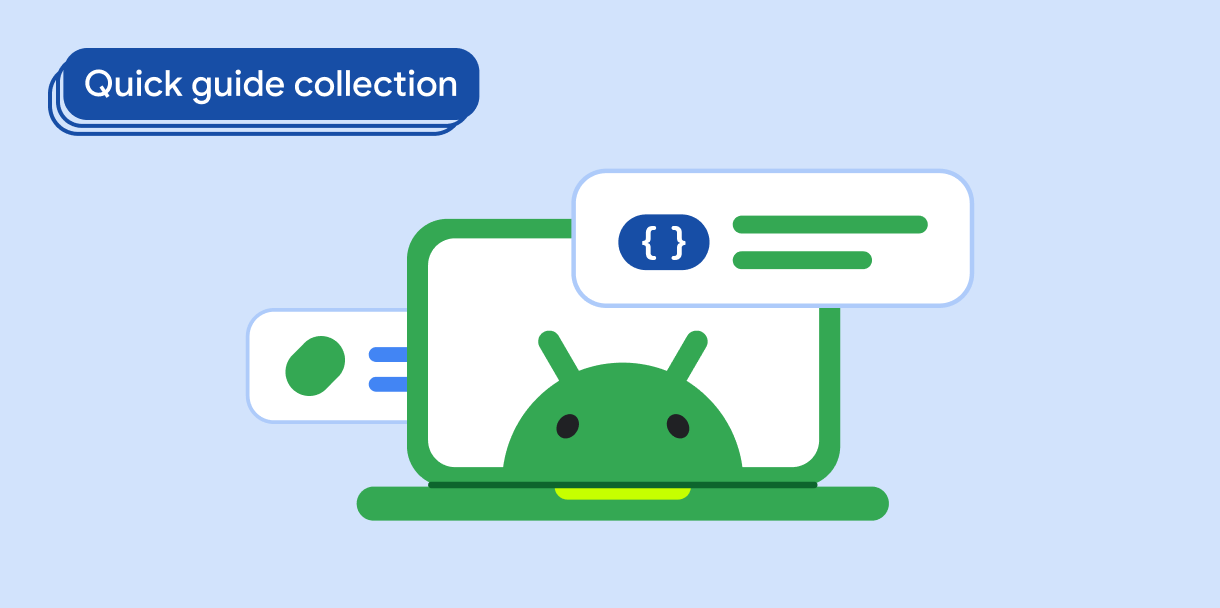