يشرح هذا المستند كيفية دمج واجهة برمجة تطبيقات مدير بيانات الاعتماد مع تطبيق Android يستخدم WebView.
نظرة عامة
قبل التعمق في عملية الدمج، من المهم فهم سير الاتصال بين رمز Android الأصلي ومكوِّن ويب معروض ضمن WebView يدير مصادقة تطبيقك وخلفية. وتنطوي هذه العملية على التسجيل (إنشاء بيانات الاعتماد) والمصادقة (الحصول على بيانات الاعتماد الحالية).
التسجيل (إنشاء مفتاح مرور)
- تنشئ الخلفية ملف JSON للتسجيل المبدئي وترسله إلى صفحة الويب المعروضة ضمن WebView.
- تستخدم صفحة الويب
navigator.credentials.create()
لتسجيل بيانات الاعتماد الجديدة. ستستخدم رمز JavaScript الذي تم إدخاله لإلغاء هذه الطريقة في خطوة لاحقة لإرسال الطلب إلى تطبيق Android. - يستخدم تطبيق Android واجهة برمجة تطبيقات مدير بيانات الاعتماد لإنشاء
طلب بيانات الاعتماد واستخدامها من أجل
createCredential
. - تشارك واجهة برمجة تطبيقات إدارة بيانات الاعتماد بيانات اعتماد المفتاح العام مع التطبيق.
- يرسل التطبيق بيانات اعتماد المفتاح العام مرة أخرى إلى صفحة الويب حتى تتمكن لغة JavaScript التي تم إدخالها من تحليل الردود.
- ترسل صفحة الويب المفتاح العام إلى الخلفية، وهي التي تتحقّق من المفتاح العام وتحفظه.
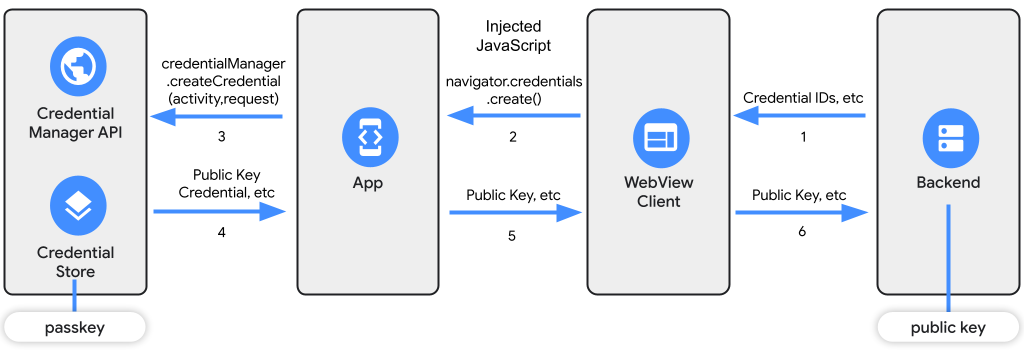
المصادقة (الحصول على مفتاح مرور)
- تنشئ الخلفية مصادقة JSON للحصول على بيانات الاعتماد وترسلها إلى صفحة الويب التي يتم عرضها في برنامج WebView.
- تستخدم صفحة الويب
navigator.credentials.get
. استخدِم رمز JavaScript الذي تم إدخاله لإلغاء هذه الطريقة لإعادة توجيه الطلب إلى تطبيق Android. - يسترد التطبيق بيانات الاعتماد باستخدام واجهة برمجة تطبيقات إدارة بيانات الاعتماد من خلال طلب الرمز
getCredential
. - تعرض Credential Manager API بيانات الاعتماد للتطبيق.
- يحصل التطبيق على التوقيع الرقمي للمفتاح الخاص ويرسله إلى صفحة الويب بحيث يمكن لبرنامج JavaScript الذي تم إدخاله تحليل الردود.
- ثم ترسلها صفحة الويب إلى الخادم الذي يتحقق من التوقيع الرقمي باستخدام المفتاح العام.
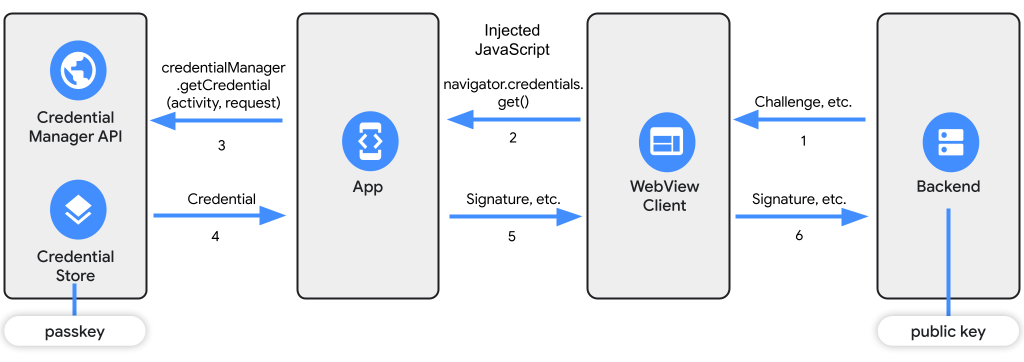
ويمكن استخدام الخطوات نفسها لكلمات المرور أو أنظمة الهوية الموحدة.
المتطلّبات الأساسية
لاستخدام واجهة برمجة تطبيقات "إدارة بيانات الاعتماد"، أكمِل الخطوات الموضّحة في قسم المتطلبات الأساسية في دليل "مدير بيانات الاعتماد"، واحرص على تنفيذ ما يلي:
اتصال JavaScript
للسماح لJavaScript في WebView ورمز Android الأصلي بالتحدث مع بعضهما البعض، عليك إرسال الرسائل والتعامل مع الطلبات بين البيئتين. لإجراء ذلك، عليك إدخال رمز JavaScript مخصّص في WebView. يتيح لك ذلك تعديل سلوك محتوى الويب والتفاعل مع رمز Android الأصلي.
إدخال JavaScript
ينشئ رمز JavaScript التالي اتصالاً
بين WebView وتطبيق Android. يؤدي ذلك إلى إلغاء طرق
navigator.credentials.create()
و
navigator.credentials.get()
التي تستخدمها
WebAuthn API لعمليات التسجيل والمصادقة
الموضّحة سابقًا.
استخدِم النسخة المصغَّرة من رمز JavaScript في تطبيقك.
إنشاء أداة معالجة لمفاتيح المرور
يمكنك إعداد صف PasskeyWebListener
للتعامل مع
الاتصال باستخدام JavaScript. يجب أن يكتسب هذا الصف من
WebViewCompat.WebMessageListener
. تتلقى هذه الفئة الرسائل من
JavaScript وتنفّذ الإجراءات اللازمة في تطبيق Android.
Kotlin
// The class talking to Javascript should inherit:
class PasskeyWebListener(
private val activity: Activity,
private val coroutineScope: CoroutineScope,
private val credentialManagerHandler: CredentialManagerHandler
) : WebViewCompat.WebMessageListener
// ... Implementation details
Java
// The class talking to Javascript should inherit:
class PasskeyWebListener implements WebViewCompat.WebMessageListener {
// Implementation details
private Activity activity;
// Handles get/create methods meant for Java:
private CredentialManagerHandler credentialManagerHandler;
public PasskeyWebListener(
Activity activity,
CredentialManagerHandler credentialManagerHandler
) {
this.activity = activity;
this.credentialManagerHandler = credentialManagerHandler;
}
// ... Implementation details
}
في PasskeyWebListener
، يمكنك تنفيذ المنطق للطلبات والردود، كما هو موضّح في الأقسام التالية.
معالجة طلب المصادقة
لمعالجة طلبات عمليات WebAuthn navigator.credentials.create()
أو navigator.credentials.get()
، يتم استدعاء طريقة onPostMessage
للفئة PasskeyWebListener
عندما يرسل رمز JavaScript رسالة إلى تطبيق Android:
Kotlin
class PasskeyWebListener(...)... {
// ...
/** havePendingRequest is true if there is an outstanding WebAuthn request.
There is only ever one request outstanding at a time. */
private var havePendingRequest = false
/** pendingRequestIsDoomed is true if the WebView has navigated since
starting a request. The FIDO module cannot be canceled, but the response
will never be delivered in this case. */
private var pendingRequestIsDoomed = false
/** replyChannel is the port that the page is listening for a response on.
It is valid if havePendingRequest is true. */
private var replyChannel: ReplyChannel? = null
/**
* Called by the page during a WebAuthn request.
*
* @param view Creates the WebView.
* @param message The message sent from the client using injected JavaScript.
* @param sourceOrigin The origin of the HTTPS request. Should not be null.
* @param isMainFrame Should be set to true. Embedded frames are not
supported.
* @param replyProxy Passed in by JavaScript. Allows replying when wrapped in
the Channel.
* @return The message response.
*/
@UiThread
override fun onPostMessage(
view: WebView,
message: WebMessageCompat,
sourceOrigin: Uri,
isMainFrame: Boolean,
replyProxy: JavaScriptReplyProxy,
) {
val messageData = message.data ?: return
onRequest(
messageData,
sourceOrigin,
isMainFrame,
JavaScriptReplyChannel(replyProxy)
)
}
private fun onRequest(
msg: String,
sourceOrigin: Uri,
isMainFrame: Boolean,
reply: ReplyChannel,
) {
msg?.let {
val jsonObj = JSONObject(msg);
val type = jsonObj.getString(TYPE_KEY)
val message = jsonObj.getString(REQUEST_KEY)
if (havePendingRequest) {
postErrorMessage(reply, "The request already in progress", type)
return
}
replyChannel = reply
if (!isMainFrame) {
reportFailure("Requests from subframes are not supported", type)
return
}
val originScheme = sourceOrigin.scheme
if (originScheme == null || originScheme.lowercase() != "https") {
reportFailure("WebAuthn not permitted for current URL", type)
return
}
// Verify that origin belongs to your website,
// it's because the unknown origin may gain credential info.
if (isUnknownOrigin(originScheme)) {
return
}
havePendingRequest = true
pendingRequestIsDoomed = false
// Use a temporary "replyCurrent" variable to send the data back, while
// resetting the main "replyChannel" variable to null so it’s ready for
// the next request.
val replyCurrent = replyChannel
if (replyCurrent == null) {
Log.i(TAG, "The reply channel was null, cannot continue")
return;
}
when (type) {
CREATE_UNIQUE_KEY ->
this.coroutineScope.launch {
handleCreateFlow(credentialManagerHandler, message, replyCurrent)
}
GET_UNIQUE_KEY -> this.coroutineScope.launch {
handleGetFlow(credentialManagerHandler, message, replyCurrent)
}
else -> Log.i(TAG, "Incorrect request json")
}
}
}
private suspend fun handleCreateFlow(
credentialManagerHandler: CredentialManagerHandler,
message: String,
reply: ReplyChannel,
) {
try {
havePendingRequest = false
pendingRequestIsDoomed = false
val response = credentialManagerHandler.createPasskey(message)
val successArray = ArrayList<Any>();
successArray.add("success");
successArray.add(JSONObject(response.registrationResponseJson));
successArray.add(CREATE_UNIQUE_KEY);
reply.send(JSONArray(successArray).toString())
replyChannel = null // setting initial replyChannel for the next request
} catch (e: CreateCredentialException) {
reportFailure(
"Error: ${e.errorMessage} w type: ${e.type} w obj: $e",
CREATE_UNIQUE_KEY
)
} catch (t: Throwable) {
reportFailure("Error: ${t.message}", CREATE_UNIQUE_KEY)
}
}
companion object {
const val TYPE_KEY = "type"
const val REQUEST_KEY = "request"
const val CREATE_UNIQUE_KEY = "create"
const val GET_UNIQUE_KEY = "get"
}
}
Java
class PasskeyWebListener implements ... {
// ...
/**
* Called by the page during a WebAuthn request.
*
* @param view Creates the WebView.
* @param message The message sent from the client using injected JavaScript.
* @param sourceOrigin The origin of the HTTPS request. Should not be null.
* @param isMainFrame Should be set to true. Embedded frames are not
supported.
* @param replyProxy Passed in by JavaScript. Allows replying when wrapped in
the Channel.
* @return The message response.
*/
@UiThread
public void onPostMessage(
@NonNull WebView view,
@NonNull WebMessageCompat message,
@NonNull Uri sourceOrigin,
Boolean isMainFrame,
@NonNull JavaScriptReplyProxy replyProxy,
) {
if (messageData == null) {
return;
}
onRequest(
messageData,
sourceOrigin,
isMainFrame,
JavaScriptReplyChannel(replyProxy)
)
}
private void onRequest(
String msg,
Uri sourceOrigin,
boolean isMainFrame,
ReplyChannel reply
) {
if (msg != null) {
try {
JSONObject jsonObj = new JSONObject(msg);
String type = jsonObj.getString(TYPE_KEY);
String message = jsonObj.getString(REQUEST_KEY);
boolean isCreate = type.equals(CREATE_UNIQUE_KEY);
boolean isGet = type.equals(GET_UNIQUE_KEY);
if (havePendingRequest) {
postErrorMessage(reply, "The request already in progress", type);
return;
}
replyChannel = reply;
if (!isMainFrame) {
reportFailure("Requests from subframes are not supported", type);
return;
}
String originScheme = sourceOrigin.getScheme();
if (originScheme == null || !originScheme.toLowerCase().equals("https")) {
reportFailure("WebAuthn not permitted for current URL", type);
return;
}
// Verify that origin belongs to your website,
// Requests of unknown origin may gain access to credential info.
if (isUnknownOrigin(originScheme)) {
return;
}
havePendingRequest = true;
pendingRequestIsDoomed = false;
// Use a temporary "replyCurrent" variable to send the data back,
// while resetting the main "replyChannel" variable to null so it’s
// ready for the next request.
ReplyChannel replyCurrent = replyChannel;
if (replyCurrent == null) {
Log.i(TAG, "The reply channel was null, cannot continue");
return;
}
if (isCreate) {
handleCreateFlow(credentialManagerHandler, message, replyCurrent));
} else if (isGet) {
handleGetFlow(credentialManagerHandler, message, replyCurrent));
} else {
Log.i(TAG, "Incorrect request json");
}
} catch (JSONException e) {
e.printStackTrace();
}
}
}
}
بالنسبة إلى handleCreateFlow
وhandleGetFlow
، يمكنك الرجوع إلى المثال على GitHub.
التعامل مع الردّ
لمعالجة الردود التي يتم إرسالها من التطبيق الأصلي إلى صفحة الويب، يمكنك إضافة JavaScriptReplyProxy
ضمن JavaScriptReplyChannel
.
Kotlin
class PasskeyWebListener(...)... {
// ...
// The setup for the reply channel allows communication with JavaScript.
private class JavaScriptReplyChannel(private val reply: JavaScriptReplyProxy) :
ReplyChannel {
override fun send(message: String?) {
try {
reply.postMessage(message!!)
} catch (t: Throwable) {
Log.i(TAG, "Reply failure due to: " + t.message);
}
}
}
// ReplyChannel is the interface where replies to the embedded site are
// sent. This allows for testing since AndroidX bans mocking its objects.
interface ReplyChannel {
fun send(message: String?)
}
}
Java
class PasskeyWebListener implements ... {
// ...
// The setup for the reply channel allows communication with JavaScript.
private static class JavaScriptReplyChannel implements ReplyChannel {
private final JavaScriptReplyProxy reply;
JavaScriptReplyChannel(JavaScriptReplyProxy reply) {
this.reply = reply;
}
@Override
public void send(String message) {
reply.postMessage(message);
}
}
// ReplyChannel is the interface where replies to the embedded site are
// sent. This allows for testing since AndroidX bans mocking its objects.
interface ReplyChannel {
void send(String message);
}
}
احرص على اكتشاف أي أخطاء من التطبيق الأصلي وإعادة إرسالها إلى جانب JavaScript.
الدمج مع WebView
يوضّح هذا القسم كيفية إعداد دمج WebView.
تهيئة WebView
في نشاط تطبيق Android، عليك إعداد WebView
وإعداد WebViewClient
مصاحب. تعالج WebViewClient
عملية الاتصال باستخدام رمز JavaScript الذي تم إدخاله في WebView
.
إعداد WebView وطلب إدارة بيانات الاعتماد:
Kotlin
val credentialManagerHandler = CredentialManagerHandler(this)
// ...
AndroidView(factory = {
WebView(it).apply {
settings.javaScriptEnabled = true
// Test URL:
val url = "https://credman-web-test.glitch.me/"
val listenerSupported = WebViewFeature.isFeatureSupported(
WebViewFeature.WEB_MESSAGE_LISTENER
)
if (listenerSupported) {
// Inject local JavaScript that calls Credential Manager.
hookWebAuthnWithListener(this, this@MainActivity,
coroutineScope, credentialManagerHandler)
} else {
// Fallback routine for unsupported API levels.
}
loadUrl(url)
}
}
)
Java
// Example shown in the onCreate method of an Activity
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
WebView webView = findViewById(R.id.web_view);
// Test URL:
String url = "https://credman-web-test.glitch.me/";
Boolean listenerSupported = WebViewFeature.isFeatureSupported(
WebViewFeature.WEB_MESSAGE_LISTENER
);
if (listenerSupported) {
// Inject local JavaScript that calls Credential Manager.
hookWebAuthnWithListener(webView, this,
coroutineScope, credentialManagerHandler)
} else {
// Fallback routine for unsupported API levels.
}
webView.loadUrl(url);
}
يمكنك إنشاء كائن عميل WebView جديد وإدخال JavaScript في صفحة الويب:
Kotlin
// This is an example call into hookWebAuthnWithListener
val passkeyWebListener = PasskeyWebListener(
activity, coroutineScope, credentialManagerHandler
)
val webViewClient = object : WebViewClient() {
override fun onPageStarted(view: WebView?, url: String?, favicon: Bitmap?) {
super.onPageStarted(view, url, favicon)
// Handle page load events
passkeyWebListener.onPageStarted();
webView.evaluateJavascript(PasskeyWebListener.INJECTED_VAL, null)
}
}
webView.webViewClient = webViewClient
Java
// This is an example call into hookWebAuthnWithListener
PasskeyWebListener passkeyWebListener = new PasskeyWebListener(
activity, credentialManagerHandler
)
WebViewClient webiewClient = new WebViewClient() {
@Override
public void onPageStarted(WebView view, String url, Bitmap favicon) {
super.onPageStarted(view, url, favicon);
// Handle page load events
passkeyWebListener.onPageStarted();
webView.evaulateJavascript(PasskeyWebListener.INJECTED_VAL, null);
}
};
webView.setWebViewClient(webViewClient);
إعداد أداة معالجة رسائل الويب
للسماح بنشر الرسائل بين JavaScript وتطبيق Android، عليك إعداد
أداة معالجة رسائل الويب باستخدام طريقة WebViewCompat.addWebMessageListener
.
Kotlin
val rules = setOf("*")
if (WebViewFeature.isFeatureSupported(WebViewFeature.WEB_MESSAGE_LISTENER)) {
WebViewCompat.addWebMessageListener(
webView, PasskeyWebListener.INTERFACE_NAME, rules, passkeyWebListener
)
}
Java
Set<String> rules = new HashSet<>(Arrays.asList("*"));
if (WebViewFeature.isFeatureSupported(WebViewFeature.WEB_MESSAGE_LISTENER)) {
WebViewCompat.addWebMessageListener(
webView, PasskeyWebListener.INTERFACE_NAME, rules, passkeyWebListener
)
}
الدمج على الويب
للتعرّف على كيفية إنشاء صفحة الدفع في عملية دمج الموقع الإلكتروني، يمكنك إنشاء مفتاح مرور لتسجيل الدخول بدون كلمة مرور وتسجيل الدخول باستخدام مفتاح مرور من خلال الملء التلقائي للنموذج.
الاختبار والنشر
اختبر التدفق بالكامل بدقة في بيئة خاضعة للرقابة لضمان التوصيل المناسب بين تطبيق Android وصفحة الويب والخلفية.
انشر الحل المتكامل في الإنتاج مع التأكد من أن الخلفية يمكنها التعامل مع طلبات التسجيل والمصادقة الواردة. يجب أن ينشئ رمز الخلفية جهود JSON المبدئية لعمليات التسجيل (الإنشاء) والمصادقة (الحصول). كما يجب أن يعالج التحقق من صحة الردود الواردة من صفحة الويب والتحقق منها.
تحقَّق من توافق عملية التنفيذ مع اقتراحات تجربة المستخدم.
ملاحظات مهمة
- يمكنك استخدام رمز JavaScript الذي قدّمته للتعامل مع عمليات
navigator.credentials.create()
وnavigator.credentials.get()
. - الفئة
PasskeyWebListener
هي جسر بين تطبيق Android ورمز JavaScript في WebView. تتعامل مع تمرير الرسائل والتواصل وتنفيذ الإجراءات المطلوبة. - اضبط مقتطفات الرمز المقدَّمة لتناسب بنية مشروعك واصطلاحات التسمية وأي متطلبات محددة قد تكون لديك.
- يمكنك رصد الأخطاء من جانب التطبيق الأصلي وإرسالها مرة أخرى إلى جهة JavaScript.
يمكنك من خلال اتّباع الإرشادات الواردة في هذا الدليل ودمج Credential Manager API في تطبيق Android الذي يستخدم WebView توفير تجربة تسجيل دخول آمنة وسلسة باستخدام مفتاح مرور للمستخدمين وإدارة بيانات اعتمادهم بفعالية.