You can enable the edge-to-edge display in your app by calling
enableEdgeToEdge
.
This should be sufficient for most apps. This guide describes how to enable
edge-to-edge if your app needs to do so without using enableEdgeToEdge
.
Lay out your app in full screen
Use WindowCompat.setDecorFitsSystemWindows(window,
false)
to lay out your app behind the system bars, as shown in the following code
example:
Kotlin
override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) WindowCompat.setDecorFitsSystemWindows(window, false) }
Java
@Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); WindowCompat.setDecorFitsSystemWindows(getWindow(), false); }
Change the color of the system bars
When operating in an edge-to-edge layout, your app needs to change the colors of the system bars to let the content underneath be visible. After your app performs this step, the system handles all visual protection of the user interface in gesture navigation mode and in button mode.
- Gesture navigation mode: the system applies dynamic color adaptation in which the contents of the system bars change color based on the content behind them. In the following example, the handle in the navigation bar changes to a dark color when it's above light content and to a light color when it's above dark content.
- Button mode: the system applies a translucent scrim behind the system bars (for API level 29 or later) or a transparent system bar (for API level 28 or earlier).
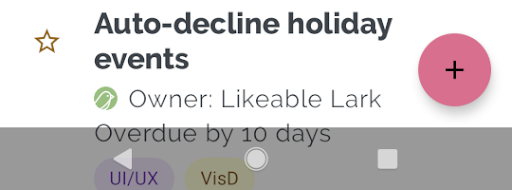
- Status bar content color: controls the color of status bar content, such as the time and icons.
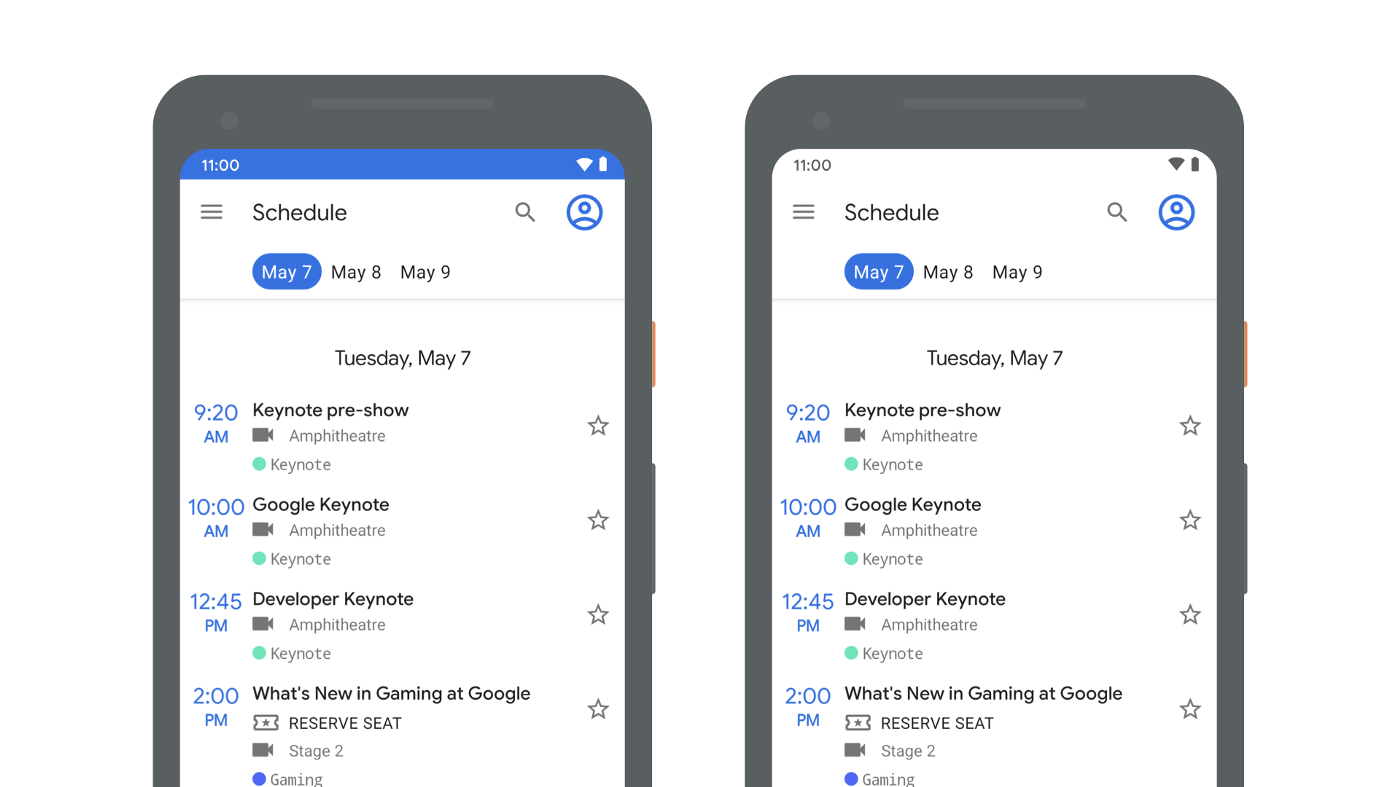
You can edit the themes.xml
file to set the color of the navigation bar and,
optionally, to set the status bar as transparent and status bar content color as
dark.
<!-- values-v29/themes.xml -->
<style name="Theme.MyApp">
<item name="android:navigationBarColor">
@android:color/transparent
</item>
<!-- Optional: set to transparent if your app is drawing behind the status bar. -->
<item name="android:statusBarColor">
@android:color/transparent
</item>
<!-- Optional: set for a light status bar with dark content. -->
<item name="android:windowLightStatusBar">
true
</item>
</style>
You can use the
WindowInsetsController
API
directly, but we strongly recommend using the Support Library
WindowInsetsControllerCompat
where possible. You can use the WindowInsetsControllerCompat
API instead of
theme.xml
to control the status bar's content color. To do so, use the
setAppearanceLightNavigationBars()
function, passing in true
to change the foreground color of the navigation to
a light color or false
to revert to the default color.
Kotlin
val windowInsetsController = ViewCompat.getWindowInsetsController(window.decorView) windowInsetsController?.isAppearanceLightNavigationBars = true
Java
WindowInsetsControllerCompat windowInsetsController = ViewCompat.getWindowInsetsController(getWindow().getDecorView()); if (windowInsetsController == null) { return; } windowInsetsController.setAppearanceLightNavigationBars(true);