In dieser Lektion wird beschrieben, wie Sie die Navigationsleiste ausblenden, Android 4.0 (API-Level 14)
Obwohl es in dieser Lektion darum geht, Navigationsleiste verwenden, sollten Sie Ihre App so gestalten, dass die Statusleiste aus, wie unter Statusleiste ausblenden beschrieben. Navigations- und Statusleiste ausblenden, aber dennoch leicht zugänglich bleiben kann der Inhalt den gesamten Anzeigebereich nutzen, wodurch ein immersiver User Experience aus.
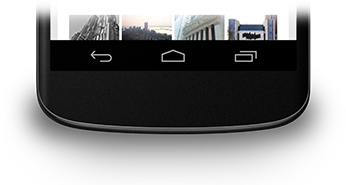
Abbildung 1: Navigationsleiste:
Navigationsleiste ausblenden
Sie können die Navigationsleiste mithilfe der
Flag SYSTEM_UI_FLAG_HIDE_NAVIGATION
. Dieses Snippet blendet sowohl
der Navigationsleiste und der Statusleiste:
Kotlin
window.decorView.apply { // Hide both the navigation bar and the status bar. // SYSTEM_UI_FLAG_FULLSCREEN is only available on Android 4.1 and higher, but as // a general rule, you should design your app to hide the status bar whenever you // hide the navigation bar. systemUiVisibility = View.SYSTEM_UI_FLAG_HIDE_NAVIGATION or View.SYSTEM_UI_FLAG_FULLSCREEN }
Java
View decorView = getWindow().getDecorView(); // Hide both the navigation bar and the status bar. // SYSTEM_UI_FLAG_FULLSCREEN is only available on Android 4.1 and higher, but as // a general rule, you should design your app to hide the status bar whenever you // hide the navigation bar. int uiOptions = View.SYSTEM_UI_FLAG_HIDE_NAVIGATION | View.SYSTEM_UI_FLAG_FULLSCREEN; decorView.setSystemUiVisibility(uiOptions);
Beachten Sie Folgendes:
- Bei diesem Ansatz wird beim Berühren einer beliebigen Stelle auf dem Bildschirm die Navigationsleiste (und Statusleiste) erscheint und bleibt sichtbar. Durch die Nutzerinteraktion werden die Flags gelöscht werden.
- Sobald die Flags gelöscht sind, müssen sie von Ihrer App zurückgesetzt werden, wenn Sie die Balken wieder ausblenden. Unter Auf Änderungen der Sichtbarkeit der Benutzeroberfläche reagieren finden Sie Diskussion darüber, wie Sie Änderungen an der Sichtbarkeit der Benutzeroberfläche überwachen können, damit Ihre App entsprechend reagieren.
- Es macht einen Unterschied, wo Sie die UI-Flags festlegen. Wenn Sie die Systemleisten im Menü
onCreate()
und drückt der Nutzer die Startbildschirmtaste, werden die Systemleisten erscheinen. Wenn der Nutzer die Aktivität wieder öffnet,onCreate()
wird nicht angerufen, sodass die Systemleisten sichtbar bleiben. Wenn Sie möchten, dass Änderungen der System-UI bleiben, während der Nutzer zu Ihrer Aktivität wechselt, und legen Sie UI-Flags inonResume()
oderonWindowFocusChanged()
. - Nur die Methode
setSystemUiVisibility()
wenn die Ansicht sichtbar ist, aus der Sie den Aufruf erstellen. - Markierungen für das Verlassen der Ansicht
festgelegt mit
setSystemUiVisibility()
gelöscht werden.
Inhalte hinter der Navigationsleiste einblenden
Unter Android 4.1 und höher können Sie festlegen, dass die Inhalte Ihrer App hinter
der Navigationsleiste, sodass die Größe des Inhalts nicht geändert wird, wenn die Navigationsleiste ausgeblendet wird
Serien und TV-Sendungen. Verwenden Sie dazu
SYSTEM_UI_FLAG_LAYOUT_HIDE_NAVIGATION
Möglicherweise müssen Sie auch
SYSTEM_UI_FLAG_LAYOUT_STABLE
, damit deine App
Layout zu ändern.
Wenn Sie diesen Ansatz verwenden, liegt es in Ihrer Verantwortung, sicherzustellen, werden nicht durch Systemleisten verdeckt. Weitere Informationen Diskussion zu diesem Thema finden Sie in der Lektion zum Ausblenden der Statusleiste