AndroidJUnitRunner
類別是 JUnit 測試執行器,可讓您在 Android 裝置上執行檢測設備的 JUnit 4 測試,包括使用 Espresso、UI Automator 和 Compose 測試架構的測試。
測試執行程式會負責將測試套件和測試中的應用程式載入至裝置、執行測試,以及回報測試結果。
這個測試執行程式支援多項常見的測試工作,包括:
編寫 JUnit 測試
下列程式碼片段說明如何編寫檢測設備 JUnit 4 測試,驗證 ChangeTextBehavior
類別中的 changeText
作業是否正常運作:
Kotlin
@RunWith(AndroidJUnit4::class) @LargeTest // Optional runner annotation class ChangeTextBehaviorTest { val stringToBeTyped = "Espresso" // ActivityTestRule accesses context through the runner @get:Rule val activityRule = ActivityTestRule(MainActivity::class.java) @Test fun changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()) onView(withId(R.id.changeTextBt)).perform(click()) // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))) } }
Java
@RunWith(AndroidJUnit4.class) @LargeTest // Optional runner annotation public class ChangeTextBehaviorTest { private static final String stringToBeTyped = "Espresso"; @Rule public ActivityTestRule<MainActivity>; activityRule = new ActivityTestRule<>;(MainActivity.class); @Test public void changeText_sameActivity() { // Type text and then press the button. onView(withId(R.id.editTextUserInput)) .perform(typeText(stringToBeTyped), closeSoftKeyboard()); onView(withId(R.id.changeTextBt)).perform(click()); // Check that the text was changed. onView(withId(R.id.textToBeChanged)) .check(matches(withText(stringToBeTyped))); } }
存取應用程式的內容
使用 AndroidJUnitRunner
執行測試時,您可以呼叫靜態 ApplicationProvider.getApplicationContext()
方法,存取測試中應用程式的內容。如果您在應用程式中建立了 Application
的自訂子類別,這個方法會傳回自訂子類別的內容。
如果您是工具導入者,可以使用 InstrumentationRegistry
類別存取低階測試 API。這個類別包含 Instrumentation
物件、目標應用程式 Context
物件、測試應用程式 Context
物件,以及傳入測試的指令列引數。
篩選測試
在 JUnit 4.x 測試中,您可以使用註解設定測試執行作業。這項功能可減少在測試中加入樣板和條件式程式碼的需求。除了 JUnit 4 支援的標準註解外,測試執行器也支援Android 專屬註解,包括以下項目:
@RequiresDevice
:指定測試應僅在實體裝置上執行,而非在模擬器上執行。@SdkSuppress
:禁止測試在低於指定級別的 Android API 級別上執行。舉例來說,如要抑制在所有 API 級別 (低於 23) 上執行的測試,請使用註解@SDKSuppress(minSdkVersion=23)
。@SmallTest
、@MediumTest
和@LargeTest
:將測試執行時間分類,進而決定測試執行頻率。您可以使用這個註解篩選要執行的測試,並設定android.testInstrumentationRunnerArguments.size
屬性:
-Pandroid.testInstrumentationRunnerArguments.size=small
區塊測試
如果您需要並行執行測試,並在多個伺服器之間共用測試,以便加快執行速度,可以將測試分成群組或區塊。測試執行工具支援將單一測試套件分割成多個資料分割,因此您可以輕鬆地將屬於同一資料分割的測試一起執行。每個分割區都會以索引編號識別。執行測試時,請使用 -e numShards
選項指定要建立的個別資料分割數量,並使用 -e shardIndex
選項指定要執行的資料分割。
舉例來說,如要將測試套件分割成 10 個區塊,並只執行第二個區塊中所分組的測試,請使用下列 adb 指令:
adb shell am instrument -w -e numShards 10 -e shardIndex 2
使用 Android Test Orchestrator
Android Test Orchestrator 可讓您透過應用程式自身叫用的 Instrumentation
個別執行每項測試。使用 AndroidJUnitRunner 1.0 以上版本時,您可以存取 Android Test Orchestrator。
Android Test Orchestrator 可為測試環境提供下列優點:
- 最少共用狀態:每項測試都會在各自的
Instrumentation
例項中執行。因此,如果測試共用應用程式狀態,大部分的共用狀態會在每次測試後從裝置的 CPU 或記憶體中移除。如要在每次測試後從裝置的 CPU 和記憶體中移除「所有」共用狀態,請使用clearPackageData
標記。請參閱「從 Gradle 啟用」一節的範例。 - 當機情形會受到隔離:即使有一個測試停止運作,也只會終止自己的
Instrumentation
執行個體。這表示套件中的其他測試仍會執行,並提供完整的測試結果。
由於 Android Test Orchestrator 會在每次測試後重新啟動應用程式,因此這種隔離方式可能會導致測試執行時間增加。
Android Studio 和 Firebase Test Lab 都預先安裝了 Android Test Orchestrator,但您需要在 Android Studio 中啟用這項功能。
透過 Gradle 啟用
如要使用 Gradle 指令列工具啟用 Android Test Orchestrator,請完成下列步驟:
- 步驟 1:修改 Gradle 檔案。在專案的
build.gradle
檔案中加入下列陳述式:
android {
defaultConfig {
...
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
// The following argument makes the Android Test Orchestrator run its
// "pm clear" command after each test invocation. This command ensures
// that the app's state is completely cleared between tests.
testInstrumentationRunnerArguments clearPackageData: 'true'
}
testOptions {
execution 'ANDROIDX_TEST_ORCHESTRATOR'
}
}
dependencies {
androidTestImplementation 'androidx.test:runner:1.1.0'
androidTestUtil 'androidx.test:orchestrator:1.1.0'
}
- 步驟 2:執行下列指令,執行 Android Test Orchestrator:
./gradlew connectedCheck
透過 Android Studio 啟用
如要在 Android Studio 中啟用 Android Test Orchestrator,請將「Enable from Gradle」中顯示的陳述式新增至應用程式的 build.gradle
檔案。
透過指令列啟用
如要在指令列上使用 Android 測試指揮家,請在終端機視窗中執行下列指令:
DEVICE_API_LEVEL=$(adb shell getprop ro.build.version.sdk)
FORCE_QUERYABLE_OPTION=""
if [[ $DEVICE_API_LEVEL -ge 30 ]]; then
FORCE_QUERYABLE_OPTION="--force-queryable"
fi
# uninstall old versions
adb uninstall androidx.test.services
adb uninstall androidx.test.orchestrator
# Install the test orchestrator.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/orchestrator/1.4.2/orchestrator-1.4.2.apk
# Install test services.
adb install $FORCE_QUERYABLE_OPTION -r path/to/m2repository/androidx/test/services/test-services/1.4.2/test-services-1.4.2.apk
# Replace "com.example.test" with the name of the package containing your tests.
# Add "-e clearPackageData true" to clear your app's data in between runs.
adb shell 'CLASSPATH=$(pm path androidx.test.services) app_process / \
androidx.test.services.shellexecutor.ShellMain am instrument -w -e \
targetInstrumentation com.example.test/androidx.test.runner.AndroidJUnitRunner \
androidx.test.orchestrator/.AndroidTestOrchestrator'
如同指令語法所示,您必須先安裝 Android Test Orchestrator,然後才能直接使用。
adb shell pm list instrumentation
使用不同的工具鍊
如果您使用其他工具鍊測試應用程式,仍可透過完成下列步驟使用 Android Test Orchestrator:
建築
Orchestrator 服務 APK 會儲存在與測試 APK 和測試中應用程式的 APK 分開的程序中:
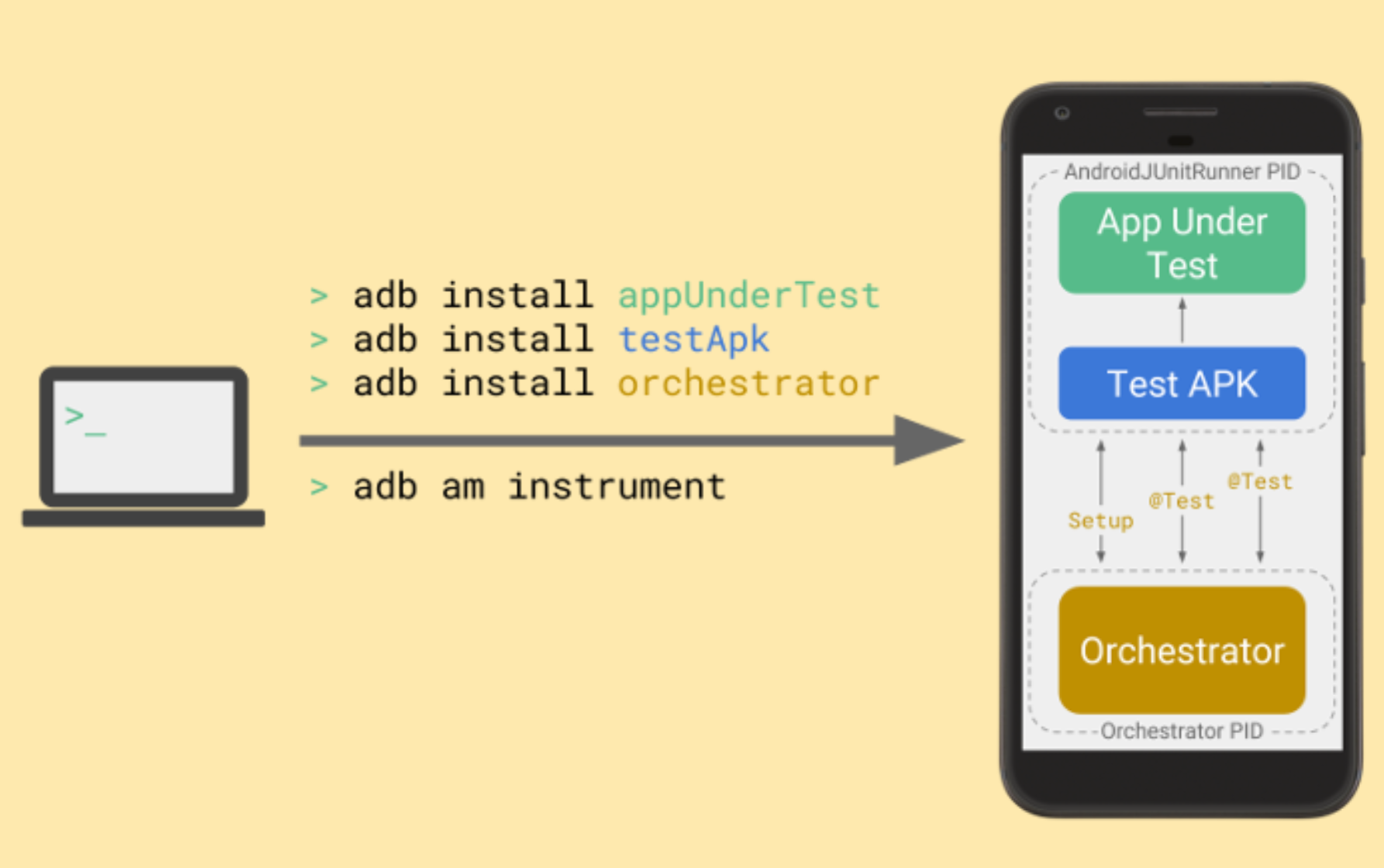
Android Test Orchestrator 會在測試套件執行的開頭收集 JUnit 測試,但會在 Instrumentation
的個別例項中個別執行每項測試。
更多資訊
如要進一步瞭解如何使用 AndroidJUnitRunner,請參閱 API 參考資料。
其他資源
如要進一步瞭解如何使用 AndroidJUnitRunner
,請參閱下列資源。
範例
- AndroidJunitRunnerSample:展示測試註解、參數化測試和測試套件建立作業。