Android には、さまざまな画面サイズやウィンドウ サイズ用のテストの作成に役立つさまざまなツールと API が用意されています。
DeviceConfigurationOverride
DeviceConfigurationOverride
コンポーザブルを使用すると、構成属性をオーバーライドして、Compose レイアウト内の複数の画面サイズとウィンドウ サイズをテストできます。ForcedSize
オーバーライドにより、使用可能なスペースの任意のレイアウトに適合するため、任意の画面サイズで UI テストを実行できます。たとえば、小さなスマートフォン フォーム ファクタを使用して、大型スマートフォン、折りたたみ式デバイス、タブレットの UI テストなど、すべての UI テストを実行できます。
DeviceConfigurationOverride(
DeviceConfigurationOverride.ForcedSize(DpSize(1280.dp, 800.dp))
) {
MyScreen() // Will be rendered in the space for 1280dp by 800dp without clipping.
}
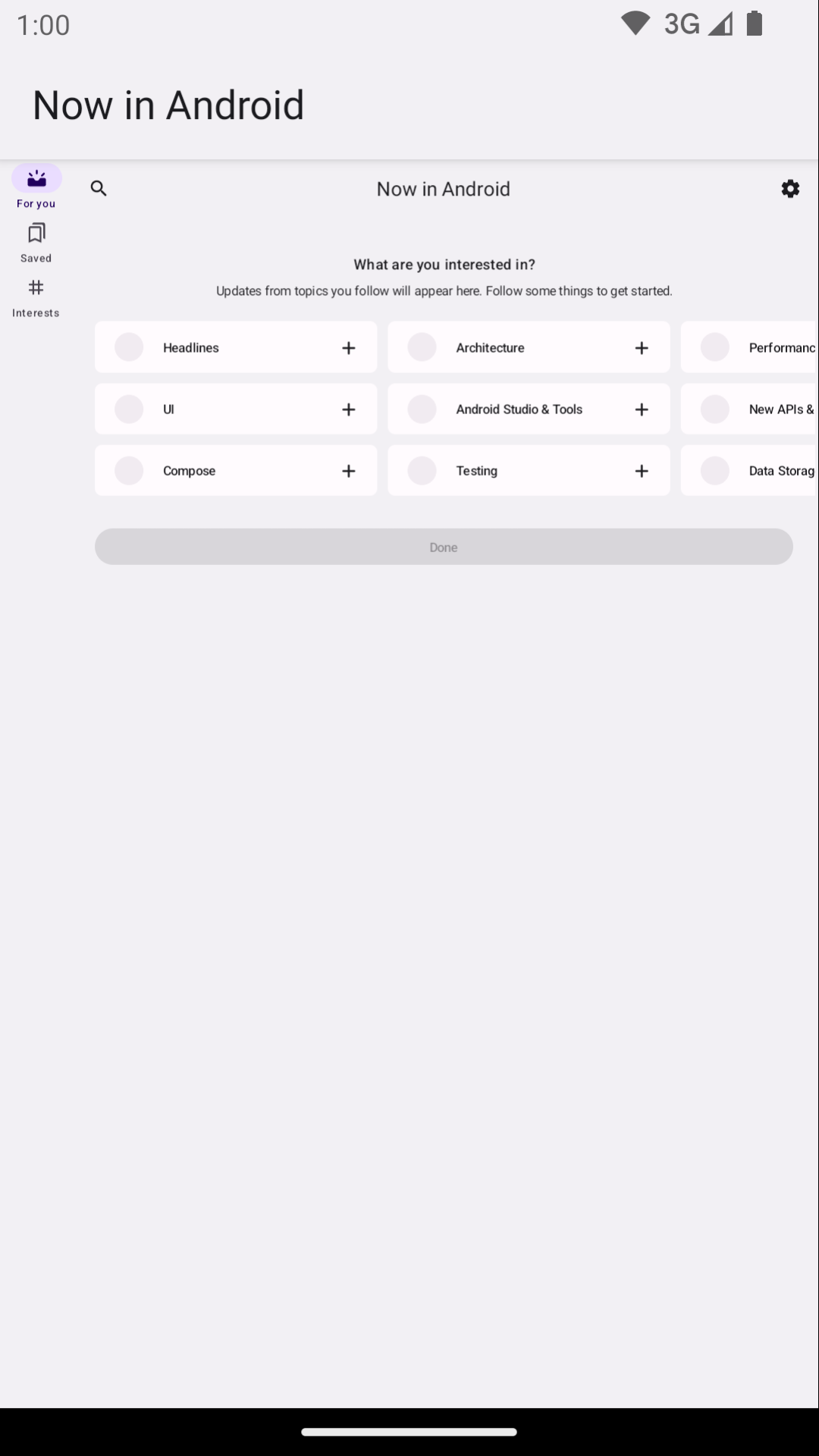
さらに、このコンポーザブルを使用して、フォントのスケールやテーマなどのプロパティを設定して、さまざまなウィンドウ サイズでテストすることもできます。
Robolectric
Robolectric を使用して、JVM 上でローカルに Compose またはビューベースの UI テストを実行できます。デバイスやエミュレータは必要ありません。特定の画面サイズなどの有用なプロパティを使用するように、Robolectric を構成できます。
次の Now in Android の例では、Robolectric が画面サイズ 1,000x1,000 dp、解像度 480 dpi をエミュレートするように構成されています。
@RunWith(RobolectricTestRunner::class)
// Configure Robolectric to use a very large screen size that can fit all of the test sizes.
// This allows enough room to render the content under test without clipping or scaling.
@Config(qualifiers = "w1000dp-h1000dp-480dpi")
class NiaAppScreenSizesScreenshotTests { ... }
また、Now in Android サンプルの次のスニペットに示すように、テスト本文から修飾子を設定することもできます。
val (width, height, dpi) = ...
// Set qualifiers from specs.
RuntimeEnvironment.setQualifiers("w${width}dp-h${height}dp-${dpi}dpi")
RuntimeEnvironment.setQualifiers()
は、新しい設定でシステム リソースとアプリリソースを更新しますが、アクティブなアクティビティやその他のコンポーネントに対するアクションはトリガーしません。
詳しくは、Robolectric のデバイス構成に関するドキュメントをご覧ください。
Gradle で管理されているデバイス
Gradle で管理されているデバイス(GMD)の Android Gradle プラグインを使用すると、インストルメンテーション テストを実行するエミュレータと実際のデバイスの仕様を定義できます。画面サイズが異なるデバイスの仕様を作成して、特定のテストを特定の画面サイズで実行する必要があるテスト戦略を実装します。継続的インテグレーション(CI)で GMD を使用すると、必要に応じて適切なテストを実行し、エミュレータをプロビジョニングして起動し、CI の設定を簡素化できます。
android {
testOptions {
managedDevices {
devices {
// Run with ./gradlew nexusOneApi30DebugAndroidTest.
nexusOneApi30(com.android.build.api.dsl.ManagedVirtualDevice) {
device = "Nexus One"
apiLevel = 30
// Use the AOSP ATD image for better emulator performance
systemImageSource = "aosp-atd"
}
// Run with ./gradlew foldApi34DebugAndroidTest.
foldApi34(com.android.build.api.dsl.ManagedVirtualDevice) {
device = "Pixel Fold"
apiLevel = 34
systemImageSource = "aosp-atd"
}
}
}
}
}
testing-samples プロジェクトには複数の GMD の例が記載されています。
Firebase Test Lab
Firebase Test Lab(FTL)または同様のデバイス ファーム サービスを使用して、アクセスできない可能性のある特定の実際のデバイス(さまざまなサイズの折りたたみ式デバイスやタブレットなど)でテストを実行します。Firebase Test Lab は、無料枠のある有料サービスです。FTL は、エミュレータでのテスト実行もサポートしています。これらのサービスでは、デバイスとエミュレータを事前にプロビジョニングできるため、インストルメンテーション テストの信頼性と速度が向上します。
GMD での FTL の使用については、Gradle で管理されているデバイスを使用したテストのスケーリングをご覧ください。
テストランナーによるテスト フィルタリング
最適なテスト戦略では、同じことを 2 回検証することがあってはならないため、ほとんどの UI テストは複数のデバイスで実行する必要がありません。通常、UI テストをフィルタするには、スマートフォン フォーム ファクタではそのすべてまたは大部分を実行し、画面サイズが異なるデバイスではサブセットのみを実行します。
特定のデバイスでのみ実行する特定のテストにアノテーションを付けて、テストを実行するコマンドを使って引数を AndroidJUnitRunner に渡すことができます。
たとえば、次のようにさまざまなアノテーションを作成できます。
annotation class TestExpandedWidth
annotation class TestCompactWidth
さまざまなテストで使用します。
class MyTestClass {
@Test
@TestExpandedWidth
fun myExample_worksOnTablet() {
...
}
@Test
@TestCompactWidth
fun myExample_worksOnPortraitPhone() {
...
}
}
その後、テストの実行時に android.testInstrumentationRunnerArguments.annotation
プロパティを使用して、特定のものをフィルタリングできます。たとえば、Gradle で管理されているデバイスを使用している場合は、次のようにします。
$ ./gradlew pixelTabletApi30DebugAndroidTest -Pandroid.testInstrumentationRunnerArguments.annotation='com.sample.TestExpandedWidth'
GMD を使用せず、CI でエミュレータを管理している場合は、まず、正しいエミュレータまたはデバイスの準備が整って接続されていることを確認してから、パラメータをいずれかの Gradle コマンドに渡して、インストルメンテーション テストを実行します。
$ ./gradlew connectedAndroidTest -Pandroid.testInstrumentationRunnerArguments.annotation='com.sample.TestExpandedWidth'
Espresso デバイス(次のセクションを参照)では、デバイス プロパティを使用してテストをフィルタすることもできます。
Espresso デバイス
Espresso Device を使用すると、Espresso、Compose、UI Automator など、あらゆる種類のインストルメンテーション テストを使用するテストで、エミュレータでアクションを実行できます。これらのアクションには、画面サイズの設定や、折りたたみ式の状態や形状の切り替えなどがあります。たとえば、折りたたみ式エミュレータを制御してテーブルトップ モードに設定できます。Espresso デバイスには、特定の機能を必要とする JUnit ルールとアノテーションも含まれています。
@RunWith(AndroidJUnit4::class)
class OnDeviceTest {
@get:Rule(order=1) val activityScenarioRule = activityScenarioRule<MainActivity>()
@get:Rule(order=2) val screenOrientationRule: ScreenOrientationRule =
ScreenOrientationRule(ScreenOrientation.PORTRAIT)
@Test
fun tabletopMode_playerIsDisplayed() {
// Set the device to tabletop mode.
onDevice().setTabletopMode()
onView(withId(R.id.player)).check(matches(isDisplayed()))
}
}
なお、Espresso Device はまだアルファ版であり、次の要件があります。
- Android Gradle プラグイン 8.3 以降
- Android Emulator 33.1.10 以降
- API レベル 24 以降を搭載した Android 仮想デバイス
テストをフィルタリングする
Espresso デバイスでは、接続済みデバイスのプロパティを読み取って、アノテーションを使用してテストをフィルタリングできます。アノテーション付きの要件が満たされていない場合、テストはスキップされます。
DeviceMode アノテーションが必要
RequiresDeviceMode
アノテーションを複数回使用して、デバイスですべての DeviceMode
値がサポートされている場合にのみ実行されるテストを示すことができます。
class OnDeviceTest {
...
@Test
@RequiresDeviceMode(TABLETOP)
@RequiresDeviceMode(BOOK)
fun tabletopMode_playerIdDisplayed() {
// Set the device to tabletop mode.
onDevice().setTabletopMode()
onView(withId(R.id.player)).check(matches(isDisplayed()))
}
}
必須 ディスプレイ アノテーション
RequiresDisplay
アノテーションを使用すると、公式のウィンドウ サイズクラスに沿ってディメンション バケットを定義するサイズクラスを使用して、デバイス画面の幅と高さを指定できます。
class OnDeviceTest {
...
@Test
@RequiresDisplay(EXPANDED, COMPACT)
fun myScreen_expandedWidthCompactHeight() {
...
}
}
ディスプレイのサイズ変更
setDisplaySize()
メソッドを使用して、実行時に画面のディメンションのサイズを変更します。このメソッドを DisplaySizeRule
クラスと組み合わせて使用すると、テスト中に加えた変更を次のテストの前に元に戻すことができます。
@RunWith(AndroidJUnit4::class)
class ResizeDisplayTest {
@get:Rule(order = 1) val activityScenarioRule = activityScenarioRule<MainActivity>()
// Test rule for restoring device to its starting display size when a test case finishes.
@get:Rule(order = 2) val displaySizeRule: DisplaySizeRule = DisplaySizeRule()
@Test
fun resizeWindow_compact() {
onDevice().setDisplaySize(
widthSizeClass = WidthSizeClass.COMPACT,
heightSizeClass = HeightSizeClass.COMPACT
)
// Verify visual attributes or state restoration.
}
}
setDisplaySize()
を使用してディスプレイのサイズを変更しても、デバイスの密度は影響しないため、寸法がターゲット デバイスに収まらない場合、テストは UnsupportedDeviceOperationException
で失敗します。この場合にテストが実行されないようにするには、RequiresDisplay
アノテーションを使用してテストを除外します。
@RunWith(AndroidJUnit4::class)
class ResizeDisplayTest {
@get:Rule(order = 1) var activityScenarioRule = activityScenarioRule<MainActivity>()
// Test rule for restoring device to its starting display size when a test case finishes.
@get:Rule(order = 2) var displaySizeRule: DisplaySizeRule = DisplaySizeRule()
/**
* Setting the display size to EXPANDED would fail in small devices, so the [RequiresDisplay]
* annotation prevents this test from being run on devices outside the EXPANDED buckets.
*/
@RequiresDisplay(
widthSizeClass = WidthSizeClassEnum.EXPANDED,
heightSizeClass = HeightSizeClassEnum.EXPANDED
)
@Test
fun resizeWindow_expanded() {
onDevice().setDisplaySize(
widthSizeClass = WidthSizeClass.EXPANDED,
heightSizeClass = HeightSizeClass.EXPANDED
)
// Verify visual attributes or state restoration.
}
}
StateRestorationTester
StateRestorationTester
クラスは、アクティビティを再作成せずにコンポーズ可能なコンポーネントの状態の復元をテストするために使用されます。アクティビティの再作成は複数の同期メカニズムを使用する複雑なプロセスであるため、テストの高速化と信頼性が向上します。
@Test
fun compactDevice_selectedEmailEmailRetained_afterConfigChange() {
val stateRestorationTester = StateRestorationTester(composeTestRule)
// Set content through the StateRestorationTester object.
stateRestorationTester.setContent {
MyApp()
}
// Simulate a config change.
stateRestorationTester.emulateSavedInstanceStateRestore()
}
ウィンドウ テスト ライブラリ
Window Testing ライブラリには、アクティビティの埋め込みや折りたたみ式機能など、ウィンドウ管理に関連する機能に依存または検証するテストの作成に役立つユーティリティが含まれています。このアーティファクトは Google の Maven リポジトリから入手できます。
たとえば、FoldingFeature()
関数を使用してカスタム FoldingFeature
を生成し、Compose プレビューで使用できます。Java では、createFoldingFeature()
関数を使用します。
Compose プレビューでは、FoldingFeature
を次のように実装できます。
@Preview(showBackground = true, widthDp = 480, heightDp = 480)
@Composable private fun FoldablePreview() =
MyApplicationTheme {
ExampleScreen(
displayFeatures = listOf(FoldingFeature(Rect(0, 240, 480, 240)))
)
}
また、TestWindowLayoutInfo()
関数を使用して、UI テストのディスプレイ機能をエミュレートすることもできます。次の例では、画面の中央に HALF_OPENED
の垂直ヒンジがある FoldingFeature
をシミュレートし、レイアウトが想定どおりかどうかを確認しています。
Compose
import androidx.window.layout.FoldingFeature.Orientation.Companion.VERTICAL
import androidx.window.layout.FoldingFeature.State.Companion.HALF_OPENED
import androidx.window.testing.layout.FoldingFeature
import androidx.window.testing.layout.TestWindowLayoutInfo
import androidx.window.testing.layout.WindowLayoutInfoPublisherRule
@RunWith(AndroidJUnit4::class)
class MediaControlsFoldingFeatureTest {
@get:Rule(order=1)
val composeTestRule = createAndroidComposeRule<ComponentActivity>()
@get:Rule(order=2)
val windowLayoutInfoPublisherRule = WindowLayoutInfoPublisherRule()
@Test
fun foldedWithHinge_foldableUiDisplayed() {
composeTestRule.setContent {
MediaPlayerScreen()
}
val hinge = FoldingFeature(
activity = composeTestRule.activity,
state = HALF_OPENED,
orientation = VERTICAL,
size = 2
)
val expected = TestWindowLayoutInfo(listOf(hinge))
windowLayoutInfoPublisherRule.overrideWindowLayoutInfo(expected)
composeTestRule.waitForIdle()
// Verify that the folding feature is detected and media controls shown.
composeTestRule.onNodeWithTag("MEDIA_CONTROLS").assertExists()
}
}
回視聴
import androidx.window.layout.FoldingFeature.Orientation
import androidx.window.layout.FoldingFeature.State
import androidx.window.testing.layout.FoldingFeature
import androidx.window.testing.layout.TestWindowLayoutInfo
import androidx.window.testing.layout.WindowLayoutInfoPublisherRule
@RunWith(AndroidJUnit4::class)
class MediaControlsFoldingFeatureTest {
@get:Rule(order=1)
val activityRule = ActivityScenarioRule(MediaPlayerActivity::class.java)
@get:Rule(order=2)
val windowLayoutInfoPublisherRule = WindowLayoutInfoPublisherRule()
@Test
fun foldedWithHinge_foldableUiDisplayed() {
activityRule.scenario.onActivity { activity ->
val feature = FoldingFeature(
activity = activity,
state = State.HALF_OPENED,
orientation = Orientation.VERTICAL)
val expected = TestWindowLayoutInfo(listOf(feature))
windowLayoutInfoPublisherRule.overrideWindowLayoutInfo(expected)
}
// Verify that the folding feature is detected and media controls shown.
onView(withId(R.id.media_controls)).check(matches(isDisplayed()))
}
}
WindowManager プロジェクトにその他のサンプルがあります。
参考情報
ドキュメント
サンプル
- WindowManager のサンプル
- Espresso デバイスのサンプル
- Now In Android
- スクリーンショット テストを使用してさまざまな画面サイズを検証する
Codelab