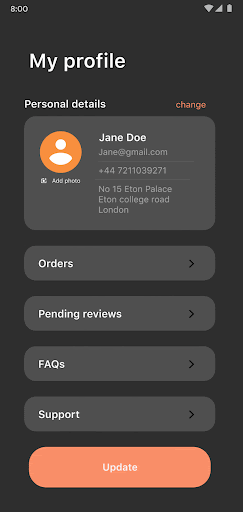
相片挑選工具提供可瀏覽的介面,可讓使用者查看媒體庫,並按照日期由新到舊排序。如「隱私權最佳做法程式碼研究室」所示,相片挑選工具內建安全的權限授予方式,可讓使用者僅授予所選圖片和影片的存取權,不必將整個媒體庫的存取權授予應用程式。
如果使用者的裝置上有符合資格的雲端媒體供應商,也可以從遠端儲存的相片和影片中選取。進一步瞭解雲端媒體供應商。
這項工具會自動更新,讓應用程式使用者持續享有更多新推出的功能,不必變更任何程式碼。
使用 Jetpack Activity 合約
如要簡化相片挑選工具整合功能,請加入 1.7.0 以上版本的 androidx.activity
程式庫。
使用下列活動結果合約啟動相片挑選工具:
如果裝置上沒有相片挑選工具,資料庫會改為自動叫用 ACTION_OPEN_DOCUMENT
意圖動作。搭載 Android 4.4 (API 級別 19) 以上版本的裝置支援這個意圖。您可以呼叫 isPhotoPickerAvailable()
,確認特定裝置是否支援相片挑選工具。
選取單一媒體項目
如要選取單一媒體項目,請使用 PickVisualMedia
活動結果合約,如以下程式碼片段所示:
Kotlin
// Registers a photo picker activity launcher in single-select mode. val pickMedia = registerForActivityResult(PickVisualMedia()) { uri -> // Callback is invoked after the user selects a media item or closes the // photo picker. if (uri != null) { Log.d("PhotoPicker", "Selected URI: $uri") } else { Log.d("PhotoPicker", "No media selected") } } // Include only one of the following calls to launch(), depending on the types // of media that you want to let the user choose from. // Launch the photo picker and let the user choose images and videos. pickMedia.launch(PickVisualMediaRequest(PickVisualMedia.ImageAndVideo)) // Launch the photo picker and let the user choose only images. pickMedia.launch(PickVisualMediaRequest(PickVisualMedia.ImageOnly)) // Launch the photo picker and let the user choose only videos. pickMedia.launch(PickVisualMediaRequest(PickVisualMedia.VideoOnly)) // Launch the photo picker and let the user choose only images/videos of a // specific MIME type, such as GIFs. val mimeType = "image/gif" pickMedia.launch(PickVisualMediaRequest(PickVisualMedia.SingleMimeType(mimeType)))
Java
// Registers a photo picker activity launcher in single-select mode. ActivityResultLauncher<PickVisualMediaRequest> pickMedia = registerForActivityResult(new PickVisualMedia(), uri -> { // Callback is invoked after the user selects a media item or closes the // photo picker. if (uri != null) { Log.d("PhotoPicker", "Selected URI: " + uri); } else { Log.d("PhotoPicker", "No media selected"); } }); // Include only one of the following calls to launch(), depending on the types // of media that you want to let the user choose from. // Launch the photo picker and let the user choose images and videos. pickMedia.launch(new PickVisualMediaRequest.Builder() .setMediaType(PickVisualMedia.ImageAndVideo.INSTANCE) .build()); // Launch the photo picker and let the user choose only images. pickMedia.launch(new PickVisualMediaRequest.Builder() .setMediaType(PickVisualMedia.ImageOnly.INSTANCE) .build()); // Launch the photo picker and let the user choose only videos. pickMedia.launch(new PickVisualMediaRequest.Builder() .setMediaType(PickVisualMedia.VideoOnly.INSTANCE) .build()); // Launch the photo picker and let the user choose only images/videos of a // specific MIME type, such as GIFs. String mimeType = "image/gif"; pickMedia.launch(new PickVisualMediaRequest.Builder() .setMediaType(new PickVisualMedia.SingleMimeType(mimeType)) .build());
選取多個媒體項目
如要選取多個媒體項目,請設定可選取的媒體檔案數量上限,如以下程式碼片段所示。
Kotlin
// Registers a photo picker activity launcher in multi-select mode. // In this example, the app lets the user select up to 5 media files. val pickMultipleMedia = registerForActivityResult(PickMultipleVisualMedia(5)) { uris -> // Callback is invoked after the user selects media items or closes the // photo picker. if (uris.isNotEmpty()) { Log.d("PhotoPicker", "Number of items selected: ${uris.size}") } else { Log.d("PhotoPicker", "No media selected") } } // For this example, launch the photo picker and let the user choose images // and videos. If you want the user to select a specific type of media file, // use the overloaded versions of launch(), as shown in the section about how // to select a single media item. pickMultipleMedia.launch(PickVisualMediaRequest(PickVisualMedia.ImageAndVideo))
Java
// Registers a photo picker activity launcher in multi-select mode. // In this example, the app lets the user select up to 5 media files. ActivityResultLauncher<PickVisualMediaRequest> pickMultipleMedia = registerForActivityResult(new PickMultipleVisualMedia(5), uris -> { // Callback is invoked after the user selects media items or closes the // photo picker. if (!uris.isEmpty()) { Log.d("PhotoPicker", "Number of items selected: " + uris.size()); } else { Log.d("PhotoPicker", "No media selected"); } }); // For this example, launch the photo picker and let the user choose images // and videos. If you want the user to select a specific type of media file, // use the overloaded versions of launch(), as shown in the section about how // to select a single media item. pickMultipleMedia.launch(new PickVisualMediaRequest.Builder() .setMediaType(PickVisualMedia.ImageAndVideo.INSTANCE) .build());
平台會限制使用者可在相片挑選工具中選取的檔案數目上限。如要使用這項限制,請呼叫 getPickImagesMaxLimit()
。在不支援相片挑選工具的裝置上,系統會忽略此項限制。
適用裝置
裝置必須符合以下條件,才能使用相片挑選工具:
- 搭載 Android 11 (API 級別 30) 以上版本
- 透過 Google 系統更新接收模組化系統元件的變更內容
搭載 Android 4.4 (API 級別 19) 至 Android 10 (API 級別 29) 版本的舊型裝置,以及搭載 Android 11 或 12 版本且支援 Google Play 服務的 Android Go 裝置,皆可安裝相片挑選工具的向後移植版本。如要透過 Google Play 服務自動安裝向後移植的相片挑選工具模組,請在應用程式資訊清單檔案中的 <application>
標記加入以下內容:
<!-- Trigger Google Play services to install the backported photo picker module. -->
<service android:name="com.google.android.gms.metadata.ModuleDependencies"
android:enabled="false"
android:exported="false"
tools:ignore="MissingClass">
<intent-filter>
<action android:name="com.google.android.gms.metadata.MODULE_DEPENDENCIES" />
</intent-filter>
<meta-data android:name="photopicker_activity:0:required" android:value="" />
</service>
保留媒體檔案存取權
根據預設,系統會授予應用程式對媒體檔案的存取權,直到裝置重新啟動或應用程式停止為止。如果應用程式執行的是長時間執行的工作 (例如在背景上傳大型檔案),則可能需要延長此存取權的保留時間。如要延長,請呼叫 takePersistableUriPermission()
方法:
Kotlin
val flag = Intent.FLAG_GRANT_READ_URI_PERMISSION context.contentResolver.takePersistableUriPermission(uri, flag)
Java
int flag = Intent.FLAG_GRANT_READ_URI_PERMISSION; context.contentResolver.takePersistableUriPermission(uri, flag);