Le plug-in Google Play Instant pour Unity configure votre projet Unity pour créer une appli instantanée de votre jeu. Ce guide explique comment installer et utiliser ce plug-in.
Télécharger et importer le plug-in
Ce plug-in fait partie des plug-ins Google Play pour Unity. Importer procédez comme suit:
- Téléchargez la dernière version sur la page des plug-ins Google Play pour Unity.
- Importez le fichier
.unitypackage
en sélectionnant l'option de menu de l'IDE Unity Composants > Importer un package > "Custom Package" et importer tous les éléments.
Fonctionnalités de l'éditeur Unity
Importez le plug-in pour ajouter un Google > Sous-menu "Play Instant" dans Unity. Ce propose les options suivantes.
Paramètres de compilation
Ouvre une fenêtre permettant de basculer entre Installée et Instantanée. de développement d'applications. Le passage à Instantané entraîne les modifications suivantes:
- Il crée un symbole de définition de script appelé
PLAY_INSTANT
qui peut être utilisé pour l'écriture de script avec#if PLAY_INSTANT
et#endif
. - Gère les mises à jour du fichier AndroidManifest.xml pour certaines modifications requises telles que en tant que android:targetSandboxVersion.
Paramètres du lecteur
La boîte de dialogue Player Settings (Paramètres du joueur), illustrée à la figure 1, affiche des suggestions pour vous aider vous optimisez la prise en charge de Google Play Instant, et votre développement sur des plates-formes plus compatibles graphiques et réduire la taille de votre APK.
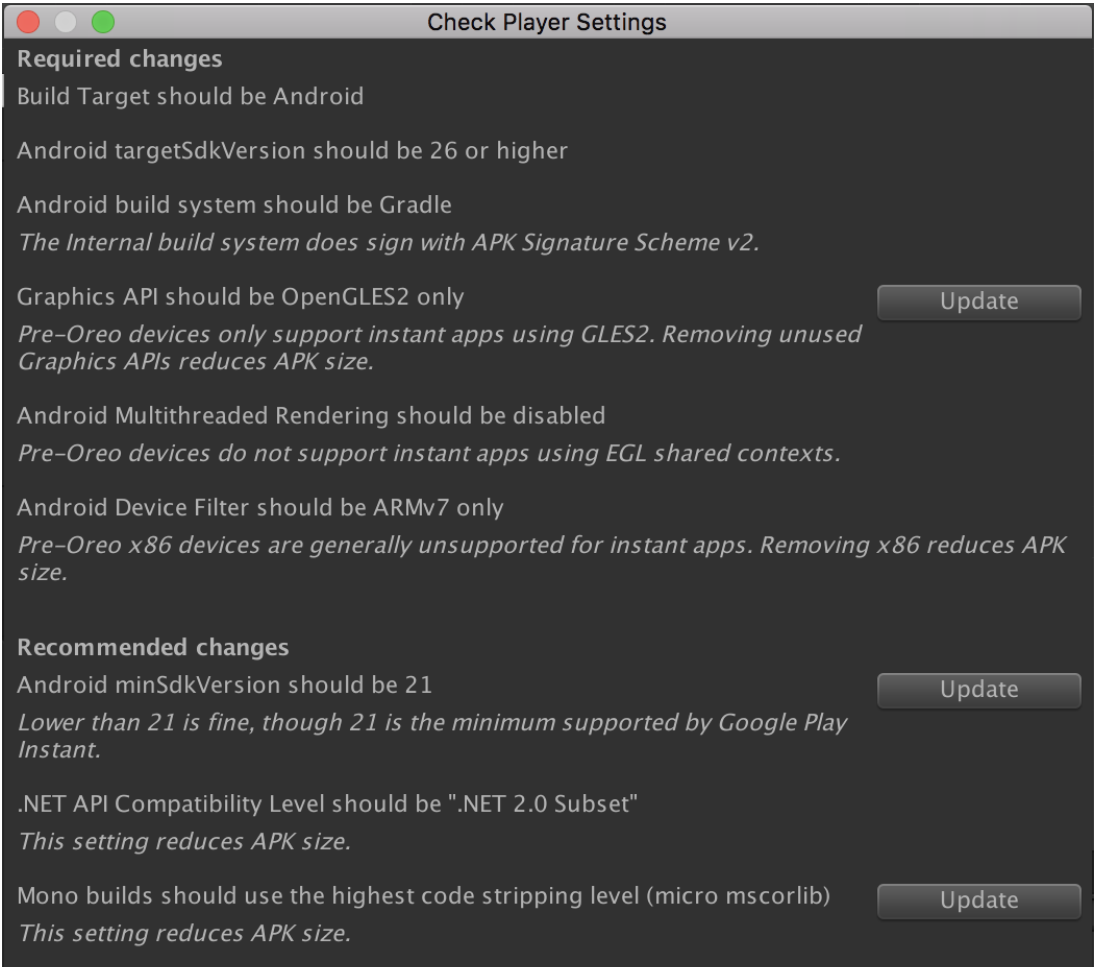
Ces paramètres de lecteur sont répartis dans les catégories Obligatoire et Recommandé. paramètres. Si un paramètre est associé au bouton Mettre à jour, cliquez dessus pour remplacez le paramètre par la valeur préférée.
Pour réduire davantage la taille de l'APK, ouvrez le gestionnaire de packages Unity et supprimez les packages inutilisés.
Déploiement rapide
Quick Deploy peut réduire la taille d'une appli instantanée basée sur Unity en empaquetant certaines éléments d'une AssetBundle. Lorsque vous utilisez Quick Deploy, le moteur de jeu Unity et un écran de chargement sont empaquetés en un APK d'appli instantanée. Une fois lancée, l'appli récupère le AssetBundle d'un serveur.
Prendre en charge les workflows d'installation
L'objectif de nombreuses applis instantanées est de donner aux utilisateurs l'occasion de découvrir l'application avant d'installer la version complète. Le plug-in Google Play Instant pour Unity fournit des API pour afficher une boîte de dialogue d'installation sur le Play Store et pour transférer l'état depuis l'appli instantanée à l'application installée.
Afficher une invite d'installation
Une appli instantanée avec un bouton Installer peut afficher une installation depuis le Play Store en appelant la commande suivante à partir d'un gestionnaire de clics sur le bouton d'installation:
Google.Play.Instant.InstallLauncher.ShowInstallPrompt();
La méthode ShowInstallPrompt()
présente une surcharge qui permet d'exécuter un ou plusieurs des éléments suivants :
les éléments suivants:
- Déterminer si l'utilisateur annule le processus d'installation. Remplacement
onActivityResult()
dans l'activité principale de l'appli instantanée et rechercherRESULT_CANCELED
sur lerequestCode
spécifié. - En transmettant une chaîne de provenance de l'installation via le paramètre
referrer
. - Transmission de l'état concernant la session de jeu en cours via
PutPostInstallIntentStringExtra()
Ces éléments sont illustrés dans l'exemple suivant:
using Google.Play.Instant;
...
const int requestCode = 123;
var sessionInfo = /* Object serialized as a string representing player's current location, etc. */;
using (var activity = UnityPlayerHelper.GetCurrentActivity())
using (var postInstallIntent = InstallLauncher.CreatePostInstallIntent(activity))
{
InstallLauncher.PutPostInstallIntentStringExtra(postInstallIntent, "sessionInfo", sessionInfo);
InstallLauncher.ShowInstallPrompt(activity, requestCode, postInstallIntent, "test-referrer");
}
Si l'utilisateur termine l'installation de l'application, le Play Store la relancera.
à l'aide du postInstallIntent
fourni. L'application installée peut récupérer une valeur
défini dans postInstallIntent
à l'aide du code suivant:
var sessionInfo = InstallLauncher.GetPostInstallIntentStringExtra("sessionInfo");
Remarques :
- Il est possible que les éléments supplémentaires inclus dans l'
postInstallIntent
ne soient pas transmis à l'appli installée application si l'utilisateur installe l'application, mais annule le lancement après installation. Les extras d'intent de transmission sont mieux adaptés pour conserver l'état d'une session active que pour conserver un état persistant ; pour ces derniers, API Cookie. - Tout le monde peut créer un intent avec des champs supplémentaires pour lancer le Par conséquent, si la charge utile apporte quelque chose de valeur, concevez-la de sorte que il ne peut être utilisé qu'une seule fois, le signer de manière cryptographique et vérifier sur un serveur.
Utiliser l'API Cookie
L'API Cookie fournit des méthodes pour transmettre un cookie (par exemple, l'ID de joueur ou le niveau
données de saisie) d'une appli instantanée à son application installée correspondante. Retirer le "J’aime"
postInstallIntent
: l'état du cookie est disponible même si l'utilisateur
ne lance pas immédiatement l'application installée. Par exemple, une appli instantanée pourrait
Appelez le code suivant à partir d'un gestionnaire de clics sur le bouton d'installation:
using Google.Play.Instant;
...
var playerInfo = /* Object serialized as a string representing game levels completed, etc. */;
var cookieBytes = System.Text.Encoding.UTF8.GetBytes(playerInfo);
try
{
var maxCookieSize = CookieApi.GetInstantAppCookieMaxSize();
if (cookieBytes.Length > maxCookieSize)
{
UnityEngine.Debug.LogErrorFormat("Cookie length {0} exceeds limit {1}.", cookieBytes.Length, maxCookieSize);
}
else if (CookieApi.SetInstantAppCookie(cookieBytes))
{
UnityEngine.Debug.Log("Successfully set cookie. Now display the app install dialog...");
InstallLauncher.ShowInstallPrompt();
}
else
{
UnityEngine.Debug.LogError("Failed to set cookie.");
}
}
catch (CookieApi.InstantAppCookieException ex)
{
UnityEngine.Debug.LogErrorFormat("Failed to set cookie: {0}", ex);
}
Si l'utilisateur termine l'installation de l'application, celle-ci peut récupérer les les données des cookies à l'aide du code suivant:
var cookieBytes = CookieApi.GetInstantAppCookie();
var playerInfoString = System.Text.Encoding.UTF8.GetString(cookieBytes);
if (!string.IsNullOrEmpty(playerInfoString))
{
// Initialize game state based on the cookie, e.g. skip tutorial level completed in instant app.
}