Le visualizzazioni di scorrimento ti consentono di spostarti tra le schermate gemelle, ad esempio le schede, con un gesto del dito orizzontale o con lo scorrimento. Questo pattern di navigazione è definito anche paging orizzontale.
Questo argomento insegna come creare un layout delle schede con visualizzazioni a scorrimento per passare da una scheda all'altra e come mostrare una barra dei titoli al posto delle schede.
Implementare le visualizzazioni a scorrimento
Puoi creare visualizzazioni a scorrimento utilizzando il
widget ViewPager2
di AndroidX.
Per utilizzare ViewPager2 e le schede, devi aggiungere una dipendenza a ViewPager2 e ai materiali Material al tuo progetto.
Per configurare il layout con ViewPager2
, aggiungi l'elemento <ViewPager2>
al
layout XML. Ad esempio, se ogni pagina nella visualizzazione a scorrimento utilizza l'intero layout, il layout sarà simile al seguente:
<androidx.viewpager2.widget.ViewPager2
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/pager"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Per inserire visualizzazioni secondarie che rappresentano ogni pagina, collega questo layout a un elemento FragmentStateAdapter
.
Ecco come potresti usarlo per scorrere una raccolta di Fragment
oggetti:
Kotlin
class CollectionDemoFragment : Fragment() { // When requested, this adapter returns a DemoObjectFragment, // representing an object in the collection. private lateinit var demoCollectionAdapter: DemoCollectionAdapter private lateinit var viewPager: ViewPager2 override fun onCreateView( inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View? { return inflater.inflate(R.layout.collection_demo, container, false) } override fun onViewCreated(view: View, savedInstanceState: Bundle?) { demoCollectionAdapter = DemoCollectionAdapter(this) viewPager = view.findViewById(R.id.pager) viewPager.adapter = demoCollectionAdapter } } class DemoCollectionAdapter(fragment: Fragment) : FragmentStateAdapter(fragment) { override fun getItemCount(): Int = 100 override fun createFragment(position: Int): Fragment { // Return a NEW fragment instance in createFragment(int). val fragment = DemoObjectFragment() fragment.arguments = Bundle().apply { // The object is just an integer. putInt(ARG_OBJECT, position + 1) } return fragment } } private const val ARG_OBJECT = "object" // Instances of this class are fragments representing a single // object in the collection. class DemoObjectFragment : Fragment() { override fun onCreateView( inflater: LayoutInflater, container: ViewGroup?, savedInstanceState: Bundle? ): View { return inflater.inflate(R.layout.fragment_collection_object, container, false) } override fun onViewCreated(view: View, savedInstanceState: Bundle?) { arguments?.takeIf { it.containsKey(ARG_OBJECT) }?.apply { val textView: TextView = view.findViewById(android.R.id.text1) textView.text = getInt(ARG_OBJECT).toString() } } }
Java
public class CollectionDemoFragment extends Fragment { // When requested, this adapter returns a DemoObjectFragment, // representing an object in the collection. DemoCollectionAdapter demoCollectionAdapter; ViewPager2 viewPager; @Nullable @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { return inflater.inflate(R.layout.collection_demo, container, false); } @Override public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) { demoCollectionAdapter = new DemoCollectionAdapter(this); viewPager = view.findViewById(R.id.pager); viewPager.setAdapter(demoCollectionAdapter); } } public class DemoCollectionAdapter extends FragmentStateAdapter { public DemoCollectionAdapter(Fragment fragment) { super(fragment); } @NonNull @Override public Fragment createFragment(int position) { // Return a NEW fragment instance in createFragment(int). Fragment fragment = new DemoObjectFragment(); Bundle args = new Bundle(); // The object is just an integer. args.putInt(DemoObjectFragment.ARG_OBJECT, position + 1); fragment.setArguments(args); return fragment; } @Override public int getItemCount() { return 100; } } // Instances of this class are fragments representing a single // object in the collection. public class DemoObjectFragment extends Fragment { public static final String ARG_OBJECT = "object"; @Nullable @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { return inflater.inflate(R.layout.fragment_collection_object, container, false); } @Override public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) { Bundle args = getArguments(); ((TextView) view.findViewById(android.R.id.text1)) .setText(Integer.toString(args.getInt(ARG_OBJECT))); } }
Le sezioni seguenti mostrano come aggiungere schede per facilitare la navigazione tra le pagine.
Aggiungere schede utilizzando TabLayout
Un elemento TabLayout
consente di visualizzare
le schede in orizzontale. Se utilizzata insieme a ViewPager2
, TabLayout
può fornire un'interfaccia familiare per la navigazione tra le pagine in una visualizzazione a scorrimento.
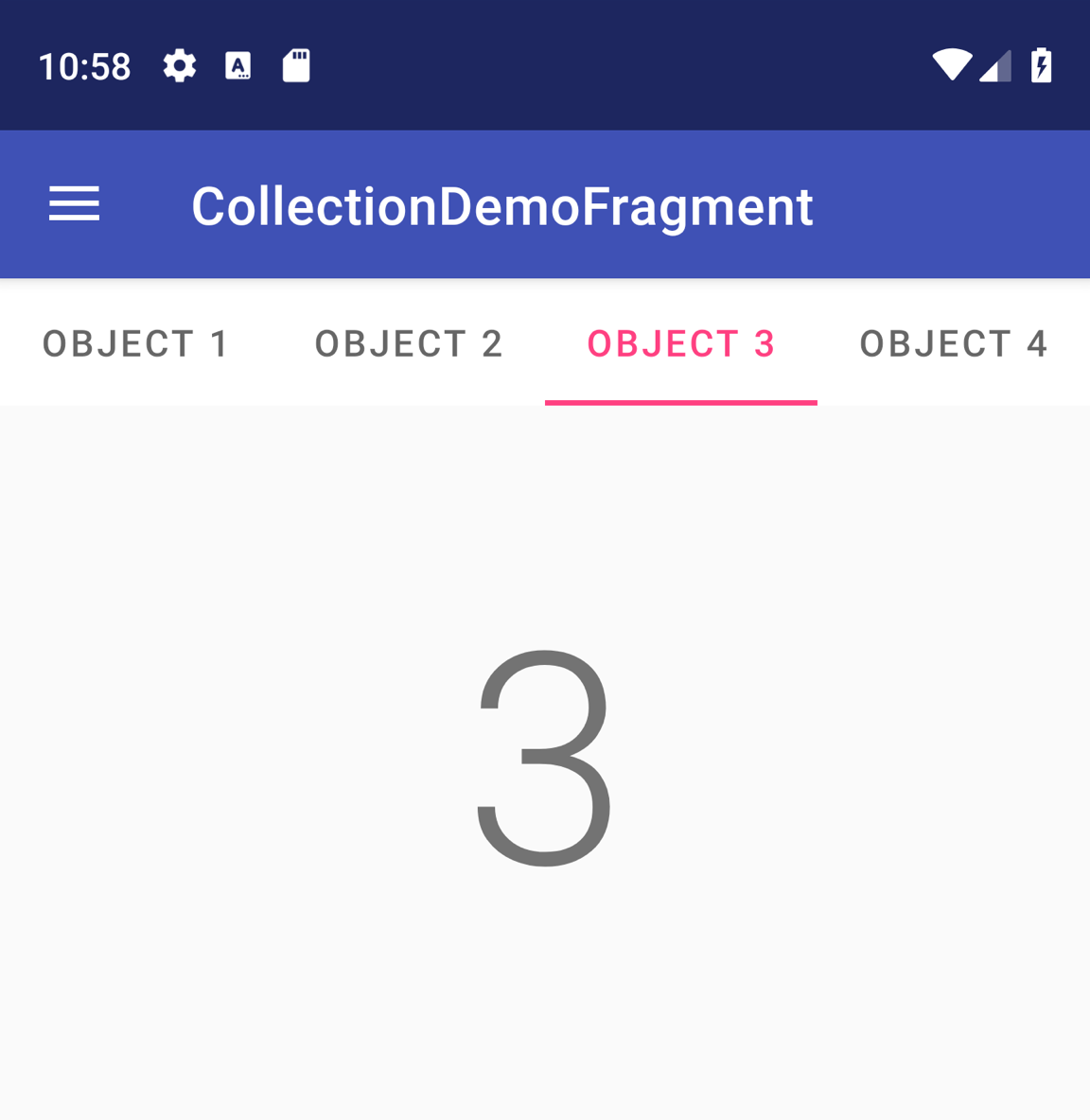
TabLayout
con quattro schede.
Per includere TabLayout
in un elemento ViewPager2
, aggiungi un elemento <TabLayout>
sopra
l'elemento <ViewPager2>
:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.google.android.material.tabs.TabLayout
android:id="@+id/tab_layout"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<androidx.viewpager2.widget.ViewPager2
android:id="@+id/pager"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1" />
</LinearLayout>
A questo punto, crea un elemento TabLayoutMediator
per collegare TabLayout
a ViewPager2
e allegalo, come indicato di seguito:
Kotlin
class CollectionDemoFragment : Fragment() { ... override fun onViewCreated(view: View, savedInstanceState: Bundle?) { val tabLayout = view.findViewById(R.id.tab_layout) TabLayoutMediator(tabLayout, viewPager) { tab, position -> tab.text = "OBJECT ${(position + 1)}" }.attach() } ... }
Java
public class CollectionDemoFragment extends Fragment { ... @Override public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) { TabLayout tabLayout = view.findViewById(R.id.tab_layout); new TabLayoutMediator(tabLayout, viewPager, (tab, position) -> tab.setText("OBJECT " + (position + 1)) ).attach(); } ... }
Per ulteriori indicazioni sulla progettazione dei layout delle schede, consulta la documentazione di Material Design per le schede.
Risorse aggiuntive
Per scoprire di più su ViewPager2
, consulta le seguenti risorse aggiuntive.
Samples
- Esempi di ViewPager2 su GitHub