IconMarginSpan
public
class
IconMarginSpan
extends Object
implements
LeadingMarginSpan,
LineHeightSpan
java.lang.Object | |
↳ | android.text.style.IconMarginSpan |
Paragraph affecting span, that draws a bitmap at the beginning of a text. The span also allows setting a padding between the bitmap and the text. The default value of the padding is 0px. The span should be attached from the first character of the text.
For example, an IconMarginSpan
with a bitmap and a padding of 30px can be set
like this:
SpannableString string = new SpannableString("Text with icon and padding"); string.setSpan(new IconMarginSpan(bitmap, 30), 0, string.length(), Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
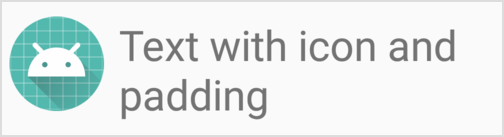
IconMarginSpan
Summary
Public constructors | |
---|---|
IconMarginSpan(Bitmap bitmap)
Creates an |
|
IconMarginSpan(Bitmap bitmap, int pad)
Creates an |
Public methods | |
---|---|
void
|
chooseHeight(CharSequence text, int start, int end, int istartv, int v, Paint.FontMetricsInt fm)
Classes that implement this should define how the height is being calculated. |
void
|
drawLeadingMargin(Canvas c, Paint p, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. |
Bitmap
|
getBitmap()
Returns the bitmap to be used at the beginning of the text |
int
|
getLeadingMargin(boolean first)
Returns the amount by which to adjust the leading margin. |
int
|
getPadding()
Returns the padding width between the bitmap and the text. |
String
|
toString()
Returns a string representation of the object. |
Inherited methods | |
---|---|
Public constructors
IconMarginSpan
public IconMarginSpan (Bitmap bitmap)
Creates an IconMarginSpan
from a Bitmap
.
Parameters | |
---|---|
bitmap |
Bitmap : bitmap to be rendered at the beginning of the text
This value cannot be null . |
IconMarginSpan
public IconMarginSpan (Bitmap bitmap, int pad)
Creates an IconMarginSpan
from a Bitmap
.
Parameters | |
---|---|
bitmap |
Bitmap : bitmap to be rendered at the beginning of the text
This value cannot be null . |
pad |
int : padding width, in pixels, between the bitmap and the text
Value is 0 or greater |
Public methods
chooseHeight
public void chooseHeight (CharSequence text, int start, int end, int istartv, int v, Paint.FontMetricsInt fm)
Classes that implement this should define how the height is being calculated.
Parameters | |
---|---|
text |
CharSequence : the text |
start |
int : the start of the line |
end |
int : the end of the line |
istartv |
int : the start of the span |
v |
int : the line height |
fm |
Paint.FontMetricsInt : font metrics of the paint, in integers |
drawLeadingMargin
public void drawLeadingMargin (Canvas c, Paint p, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. This is called before the margin has been
adjusted by the value returned by getLeadingMargin(boolean)
.
Parameters | |
---|---|
c |
Canvas : the canvas |
p |
Paint : the paint. The this should be left unchanged on exit. |
x |
int : the current position of the margin |
dir |
int : the base direction of the paragraph; if negative, the margin
is to the right of the text, otherwise it is to the left. |
top |
int : the top of the line |
baseline |
int : the baseline of the line |
bottom |
int : the bottom of the line |
text |
CharSequence : the text |
start |
int : the start of the line |
end |
int : the end of the line |
first |
boolean : true if this is the first line of its paragraph |
layout |
Layout : the layout containing this line |
getBitmap
public Bitmap getBitmap ()
Returns the bitmap to be used at the beginning of the text
Returns | |
---|---|
Bitmap |
a bitmap
This value cannot be null . |
getLeadingMargin
public int getLeadingMargin (boolean first)
Returns the amount by which to adjust the leading margin. Positive values move away from the leading edge of the paragraph, negative values move towards it.
Parameters | |
---|---|
first |
boolean : true if the request is for the first line of a paragraph,
false for subsequent lines |
Returns | |
---|---|
int |
the offset for the margin. |
getPadding
public int getPadding ()
Returns the padding width between the bitmap and the text.
This units of this value are pixels.
Returns | |
---|---|
int |
a padding width in pixels This units of this value are pixels. {} |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |