Material Design introduces elevation for UI elements. Elevation helps users understand the relative importance of each element and focus their attention to the current task.
The elevation of a view, represented by the Z property, determines the visual appearance of its shadow. Views with higher Z values cast larger, softer shadows, and they occlude views with lower Z values. However, the Z value of a view doesn't affect the view's size.
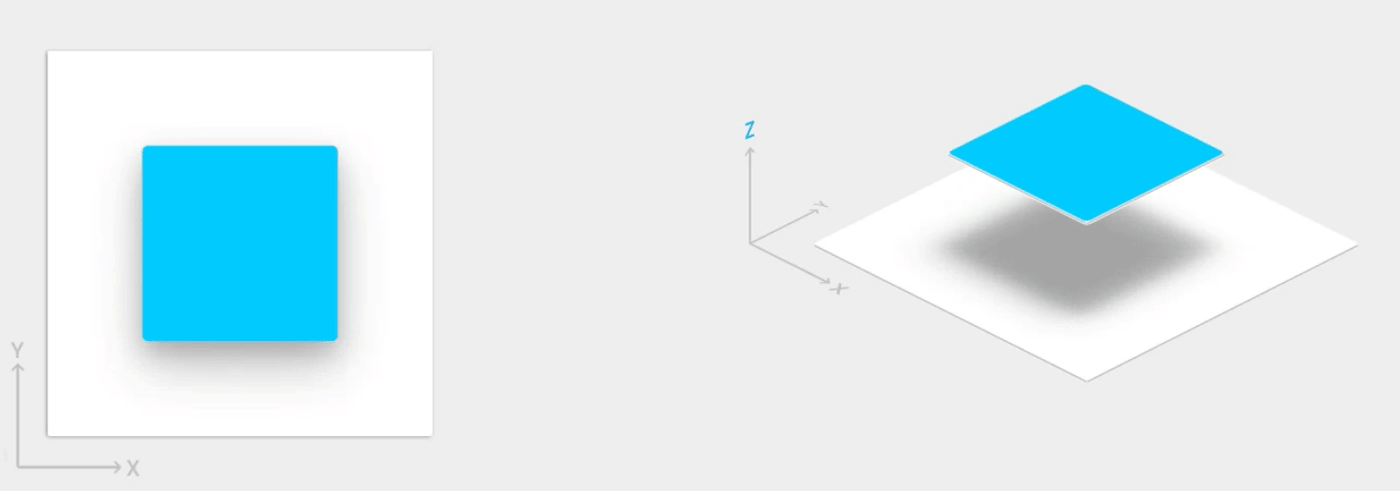
Shadows are drawn by the parent of the elevated view. They are subject to standard view clipping and are clipped by the parent by default.
Elevation is also useful to create animations where widgets temporarily rise above the view plane when performing actions.
For more information, see Elevation in Material Design.
Assign elevation to your views
The Z value for a view has two components:
- Elevation: the static component
- Translation: the dynamic component used for animations
Z = elevation + translationZ
The Z values are measured in dp (density-independent pixels).
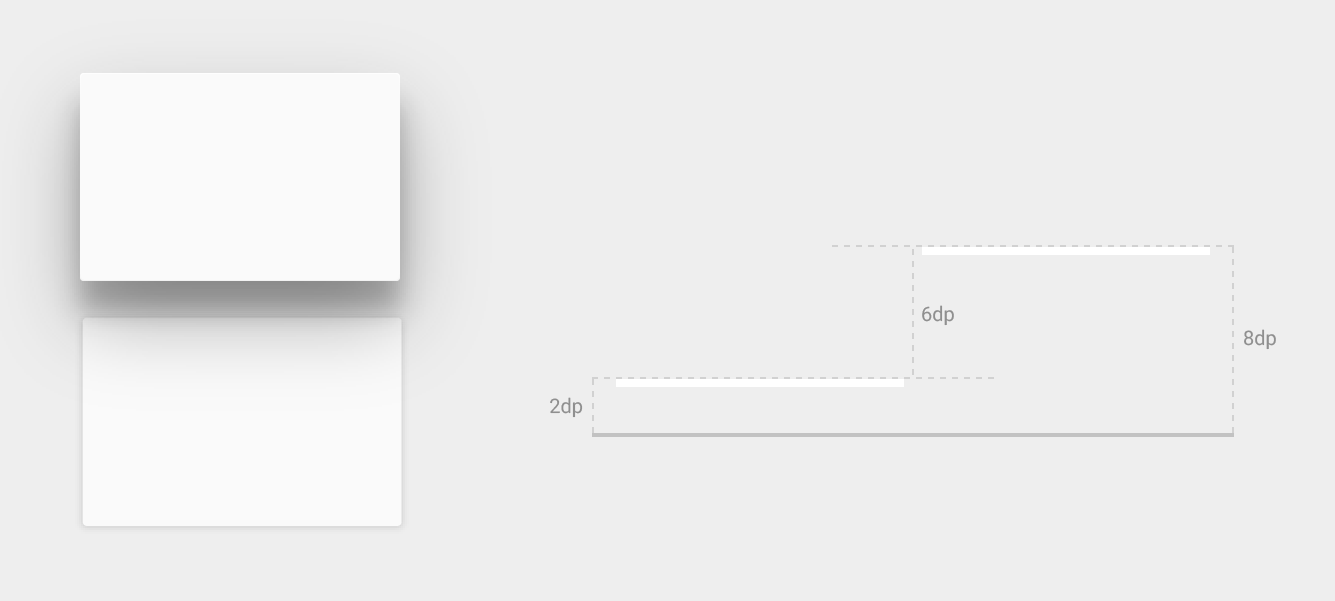
To set the default (resting) elevation of a view, use the
android:elevation
attribute in the XML layout. To set the elevation
of a view in the code of an activity, use the
View.setElevation()
method.
To set the translation of a view, use the
View.setTranslationZ()
method.
The
ViewPropertyAnimator.z()
and
ViewPropertyAnimator.translationZ()
methods let you animate the elevation of views. For more information, see the
API reference for
ViewPropertyAnimator
and the property
animation developer guide.
You can also use a
StateListAnimator
to specify these animations in a declarative way. This is especially useful for
cases where state changes trigger animations, like when the user taps a button.
For more information, see
Animate view
state changes using StateListAnimator.
Customize view shadows and outlines
The bounds of a view's background drawable determine the default shape of its shadow. Outlines represent the outer shape of a graphics object and define the ripple area for touch feedback.
Consider the following view, which is defined with a background drawable:
<TextView android:id="@+id/myview" ... android:elevation="2dp" android:background="@drawable/myrect" />
The background drawable is defined as a rectangle with rounded corners:
<!-- res/drawable/myrect.xml --> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle"> <solid android:color="#42000000" /> <corners android:radius="5dp" /> </shape>
The view casts a shadow with rounded corners, since the background drawable defines the view's outline. Providing a custom outline overrides the default shape of a view's shadow.
To define a custom outline for a view in your code, do the following:
- Extend the
ViewOutlineProvider
class. - Override the
getOutline()
method. - Assign the new outline provider to your view with the
View.setOutlineProvider()
method.
You can create oval and rectangular outlines with rounded corners using the
methods in the
Outline
class. The default outline provider for views obtains the outline from the
view's background. To prevent a view from casting a shadow, set its
outline provider to null
.
Clip views
Clipping views lets you change the shape of a view. You can clip views for
consistency with other design elements or to change the shape of a view in
response to user input. You can clip a view to its outline area using the
View.setClipToOutline()
method. Only outlines that are rectangles, circles, and round rectangles support
clipping, as determined by the
Outline.canClip()
method.
To clip a view to the shape of a drawable, set the drawable as the
background of the view—as shown in the preceding example—and call
the View.setClipToOutline()
method.
Clipping views is an expensive operation, so don't animate the shape you use to clip a view. To achieve this effect, use the reveal animation.