すべてのテキスト フィールドには、メールアドレス、電話番号、メールアドレス、電話番号など、特定のタイプのテキスト入力が求められます。 書式なしテキストで処理します。テキスト フィールドごとに入力タイプを指定して、 適切なソフト入力方法(画面キーボードなど)を使用して、
インプット メソッドで使用できるボタンの種類以外にも、 入力方法がスペル候補を提示するか、新しい文を大文字に変換し、 [Done] や [Next] などのアクション ボタンを備えた改行ボタン。このページでは、 これらの特性を指定します。
キーボード タイプの指定
必ず
android:inputType
属性を
<EditText>
要素。
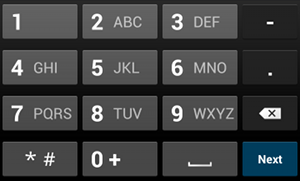
phone
入力タイプ。たとえば、電話番号を入力するための入力方法が必要な場合は、
"phone"
値:
<EditText android:id="@+id/phone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/phone_hint" android:inputType="phone" />
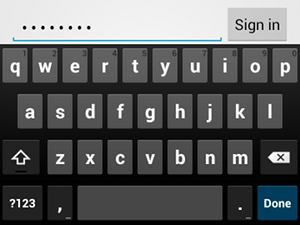
textPassword
入力タイプ。テキスト フィールドがパスワードの場合は、"textPassword"
値を使用して、テキスト フィールドがパスワード用になるようにします。
ユーザーの入力を隠します。
<EditText android:id="@+id/password" android:hint="@string/password_hint" android:inputType="textPassword" ... />
android:inputType
属性で指定できる値はいくつかありますが、
また、いくつかの値を組み合わせて、入力方法の外観や追加の入力方法を指定できます。
サポートします。
スペルの候補と他の動作の有効化
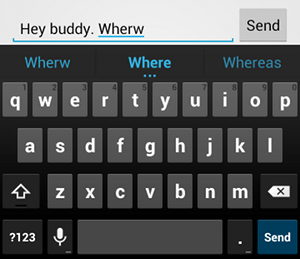
textAutoCorrect
を追加すると、
スペルミスがあります。android:inputType
属性を使用すると、入力にさまざまな動作を指定できます。
メソッドを呼び出します。最も重要なのは、テキスト フィールドが基本的なテキスト入力(例:
テキスト メッセージ - "textAutoCorrect"
で自動スペル修正を有効にする
あります。
Google Meet の設定を使用して、さまざまな動作や入力方法のスタイルを組み合わせることができます。
android:inputType
属性。たとえば、テキスト フィールドを作成し、
文の先頭の単語を大文字に変換し、スペルミスを自動修正します。
<EditText android:id="@+id/message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType= "textCapSentences|textAutoCorrect" ... />
入力方法のアクションの指定
ほとんどのソフト入力方法では、ユーザー アクション ボタンが下隅に用意されています。
現在のテキスト フィールドに表示されます。デフォルトでは、このボタンは [次へ] または
完了: テキスト フィールドが複数行のテキストをサポート
android:inputType="textMultiLine"
- この場合、操作ボタンはキャリッジです。
戻ります。ただし、テキスト フィールドに適した他のアクションを指定することもできます。
[Send] や [Go] を指定します。
キーボード アクション ボタンを指定するには、
android:imeOptions
属性を、"actionSend"
や "actionSearch"
などのアクション値に置き換えます。次に例を示します。

android:imeOptions="actionSend"
。<EditText android:id="@+id/search" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/search_hint" android:inputType="text" android:imeOptions="actionSend" />
アクション ボタンの押下をリッスンするには、
TextView.OnEditorActionListener
EditText
要素の対象:
指定された IME アクション ID に
EditorInfo
クラス、
IME_ACTION_SEND
,
次のように指定します。
Kotlin
findViewById<EditText>(R.id.search).setOnEditorActionListener { v, actionId, event -> return@setOnEditorActionListener when (actionId) { EditorInfo.IME_ACTION_SEND -> { sendMessage() true } else -> false } }
Java
EditText editText = (EditText) findViewById(R.id.search); editText.setOnEditorActionListener(new OnEditorActionListener() { @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { boolean handled = false; if (actionId == EditorInfo.IME_ACTION_SEND) { sendMessage(); handled = true; } return handled; } });
オートコンプリート候補の提供
ユーザーが入力するときに候補を提示する場合は、
EditText
さんが電話をかけました
AutoCompleteTextView
。
予測入力を実装するには、
テキストを提供する Adapter
提案します。データの出所に応じて複数のアダプターが用意されており、
取得できるからです
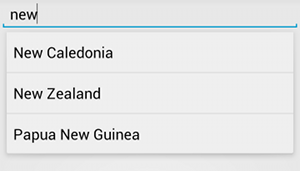
AutoCompleteTextView
の例
提案。次の手順では、AutoCompleteTextView
を設定する方法について説明します。
を使用して配列から候補を表示します。
ArrayAdapter
:
AutoCompleteTextView
をレイアウトに追加します。これはテキストのみのレイアウトです。 フィールド:<?xml version="1.0" encoding="utf-8"?> <AutoCompleteTextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/autocomplete_country" android:layout_width="fill_parent" android:layout_height="wrap_content" />
- すべてのテキスト候補を含む配列を定義します。たとえば、
国名の配列:
<?xml version="1.0" encoding="utf-8"?> <resources> <string-array name="countries_array"> <item>Afghanistan</item> <item>Albania</item> <item>Algeria</item> <item>American Samoa</item> <item>Andorra</item> <item>Angola</item> <item>Anguilla</item> <item>Antarctica</item> ... </string-array> </resources>
Activity
またはFragment
さん、次のコードを使用して 候補を提供するアダプターを指定します。Kotlin
// Get a reference to the AutoCompleteTextView in the layout. val textView = findViewById(R.id.autocomplete_country) as AutoCompleteTextView // Get the string array. val countries: Array<out String> = resources.getStringArray(R.array.countries_array) // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries).also { adapter -> textView.setAdapter(adapter) }
Java
// Get a reference to the AutoCompleteTextView in the layout. AutoCompleteTextView textView = (AutoCompleteTextView) findViewById(R.id.autocomplete_country); // Get the string array. String[] countries = getResources().getStringArray(R.array.countries_array); // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries); textView.setAdapter(adapter);
上記の例では、新しい
ArrayAdapter
が初期化され、countries_array
文字列配列をTextView
simple_list_item_1
レイアウト。これは Android が提供するレイアウトで、 標準の表示形式です。-
以下を呼び出して、アダプターを
AutoCompleteTextView
に割り当てます。setAdapter()
。