이 과정에서는 CompatTab
및 TabHelper
추상 클래스의 서브클래스를 만들고 새 API를 사용하는 방법을 보여줍니다. 애플리케이션은 이를 지원하는 플랫폼 버전을 실행하는 기기에서 이 구현을 사용할 수 있습니다.
새로운 API를 사용하여 탭 구현
최신 API를 사용하는 CompatTab
및 TabHelper
의 구체적인 클래스는 프록시 구현입니다. 이전 과정에서 정의된 추상 클래스가 새로운 API(클래스 구조, 메서드 서명 등)를 반영하므로 이러한 최신 API를 사용하는 구체적인 클래스는 메서드 호출과 그 결과를 간단히 프록시합니다.
느린 클래스 로드로 인해 이러한 구체적인 클래스에서 최신 API를 직접 사용할 수 있으며 이전 기기에서는 비정상 종료되지 않습니다. 클래스는 처음 액세스할 때 로드 및 초기화되어 처음으로 클래스를 인스턴스화하거나 클래스의 정적 필드 또는 메서드 중 하나에 액세스합니다. 따라서 Honeycomb 이전 기기에서 Honeycomb 특정 구현을 인스턴스화하지 않는 한 Dalvik VM은 VerifyError
예외를 발생시키지 않습니다.
이 구현의 올바른 이름 지정 규칙은 구체적인 클래스에 필요한 API와 일치하는 API 수준 또는 플랫폼 버전 코드명을 추가하는 것입니다. 예를 들어 네이티브 탭 구현은 CompatTabHoneycomb
및 TabHelperHoneycomb
클래스에서 제공할 수 있습니다. 이러한 클래스는 Android 3.0(API 수준 11) 이상에서 사용 가능한 API에 의존하기 때문입니다.
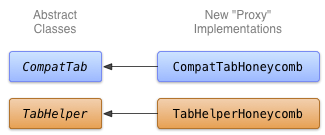
그림 1. 탭의 Honeycomb 구현 클래스 다이어그램
CompatTabHoneycomb 구현
CompatTabHoneycomb
은 TabHelperHoneycomb
이 개별 탭을 참조하는 데 사용하는 CompatTab
추상 클래스의 구현입니다. CompatTabHoneycomb
은 포함된 ActionBar.Tab
객체의 모든 메서드 호출을 간단히 프록시합니다.
다음과 같이 새 ActionBar.Tab
API를 사용하여 CompatTabHoneycomb
구현을 시작합니다.
Kotlin
class CompatTabHoneycomb internal constructor(val activity: Activity, tag: String) : CompatTab(tag) { ... // The native tab object that this CompatTab acts as a proxy for. private var mTab: ActionBar.Tab = // Proxy to new ActionBar.newTab API activity.actionBar.newTab() override fun setText(@StringRes textId: Int): CompatTab { // Proxy to new ActionBar.Tab.setText API mTab.setText(textId) return this } ... // Do the same for other properties (icon, callback, etc.) }
Java
public class CompatTabHoneycomb extends CompatTab { // The native tab object that this CompatTab acts as a proxy for. ActionBar.Tab mTab; ... protected CompatTabHoneycomb(FragmentActivity activity, String tag) { ... // Proxy to new ActionBar.newTab API mTab = activity.getActionBar().newTab(); } public CompatTab setText(int resId) { // Proxy to new ActionBar.Tab.setText API mTab.setText(resId); return this; } ... // Do the same for other properties (icon, callback, etc.) }
TabHelperHoneycomb 구현
TabHelperHoneycomb
은 포함된 Activity
에서 가져온 실제 ActionBar
의 메서드 호출을 프록시하는 TabHelper
추상 클래스의 구현입니다.
다음과 같이 TabHelperHoneycomb
을 구현하여 ActionBar
API 메서드 호출을 프록시합니다.
Kotlin
class TabHelperHoneycomb internal constructor(activity: FragmentActivity) : TabHelper(activity) { private var mActionBar: ActionBar? = null override fun setUp() { mActionBar = mActionBar ?: mActivity.actionBar.apply { navigationMode = ActionBar.NAVIGATION_MODE_TABS } } override fun addTab(tab: CompatTab) { // Tab is a CompatTabHoneycomb instance, so its // native tab object is an ActionBar.Tab. mActionBar?.addTab(tab.getTab() as ActionBar.Tab) } }
Java
public class TabHelperHoneycomb extends TabHelper { ActionBar actionBar; ... protected void setUp() { if (actionBar == null) { actionBar = activity.getActionBar(); actionBar.setNavigationMode( ActionBar.NAVIGATION_MODE_TABS); } } public void addTab(CompatTab tab) { ... // Tab is a CompatTabHoneycomb instance, so its // native tab object is an ActionBar.Tab. actionBar.addTab((ActionBar.Tab) tab.getTab()); } // The other important method, newTab() is part of // the base implementation. }