모든 텍스트 입력란에는 이메일 주소, 전화번호 또는 사용할 수 있습니다 시스템이 다음을 표시하도록 앱의 각 텍스트 필드에 입력 유형을 지정해야 합니다. 적절한 소프트 입력 방법(예: 터치 키보드)이어야 합니다.
입력 방법에 사용할 수 있는 버튼 유형 외에도 다음과 같은 동작을 지정할 수 있습니다. 입력 방법이 맞춤법 제안을 제공하고, 새 문장을 대문자로 표시하고, 완료 또는 다음과 같은 작업 버튼이 있는 캐리지 리턴 버튼입니다. 이 페이지에서는 지정할 수도 있습니다
키보드 유형 지정
텍스트 필드의 입력 방법은 항상
android:inputType
드림
속성을
<EditText>
요소
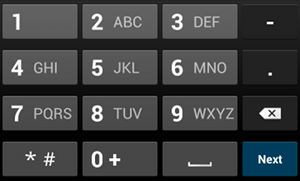
phone
입력 유형예를 들어 전화번호 입력에 사용할 입력 방법을 원하는 경우
"phone"
값:
<EditText android:id="@+id/phone" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/phone_hint" android:inputType="phone" />
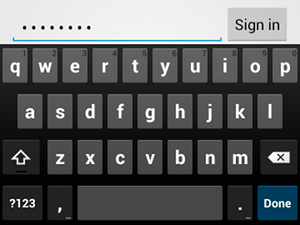
textPassword
입력 유형텍스트 필드가 비밀번호용인 경우 "textPassword"
값을 사용하여 텍스트 필드가
사용자의 입력을 숨깁니다.
<EditText android:id="@+id/password" android:hint="@string/password_hint" android:inputType="textPassword" ... />
android:inputType
속성으로 문서화되는 몇 가지 가능한 값이 있습니다.
일부 값을 결합하여 입력 방법의 모양과 추가 입력을 지정할 수 있습니다.
확인할 수 있습니다
맞춤법 제안 및 기타 동작 사용 설정
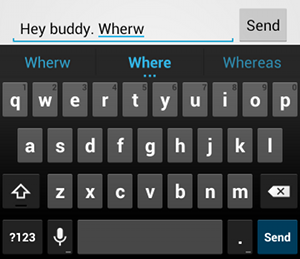
textAutoCorrect
를 추가하면
찾을 수 있습니다android:inputType
속성을 사용하면 입력의 다양한 동작을 지정할 수 있습니다.
메서드를 사용하여 축소하도록 요청합니다. 가장 중요한 것은 텍스트 필드가 기본 텍스트 입력(예:
문자 메시지—"textAutoCorrect"
을(를) 사용하여 자동 맞춤법 교정을 사용 설정합니다.
값으로 사용됩니다.
다음과 같이 다양한 동작과 입력 방법 스타일을 결합할 수 있습니다.
android:inputType
속성 예를 들어,
는 문장의 첫 단어를 대문자로 표시하고 맞춤법 오류도 자동으로 수정합니다.
<EditText android:id="@+id/message" android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType= "textCapSentences|textAutoCorrect" ... />
입력 방법 작업 지정하기
대부분의 소프트 입력 방식은 키보드 하단에 적합한 사용자 작업 버튼을
확인할 수 있습니다. 기본적으로 시스템은 Next 또는
완료 작업(예: 텍스트 필드에서 여러 줄의 텍스트를 지원하지 않는 경우)
android:inputType="textMultiLine"
: 작업 버튼이 캐리지입니다.
반환합니다. 그러나 텍스트 입력란에 더 적합한 다른 작업을 지정할 수도 있습니다.
보내기 또는 이동 중 원하는 옵션을 선택합니다.
키보드 작업 버튼을 지정하려면
android:imeOptions
드림
속성(예: "actionSend"
또는 "actionSearch"
)을 사용합니다. 예를 들면 다음과 같습니다.

android:imeOptions="actionSend"
<EditText android:id="@+id/search" android:layout_width="fill_parent" android:layout_height="wrap_content" android:hint="@string/search_hint" android:inputType="text" android:imeOptions="actionSend" />
그런 다음
TextView.OnEditorActionListener
(EditText
요소의 경우)
리스너가 리스너에 정의된 적절한 IME 작업 ID에
EditorInfo
클래스,
예:
IME_ACTION_SEND
,
다음과 같습니다.
Kotlin
findViewById<EditText>(R.id.search).setOnEditorActionListener { v, actionId, event -> return@setOnEditorActionListener when (actionId) { EditorInfo.IME_ACTION_SEND -> { sendMessage() true } else -> false } }
자바
EditText editText = (EditText) findViewById(R.id.search); editText.setOnEditorActionListener(new OnEditorActionListener() { @Override public boolean onEditorAction(TextView v, int actionId, KeyEvent event) { boolean handled = false; if (actionId == EditorInfo.IME_ACTION_SEND) { sendMessage(); handled = true; } return handled; } });
자동 완성 제안 제공
사용자가 입력할 때 추천 단어를 제공하려면
EditText
님이 전화함
AutoCompleteTextView
입니다.
자동 완성을 구현하려면
Adapter
: 텍스트를 제공합니다.
있습니다. 데이터의 출처에 따라 사용할 수 있는 어댑터는 몇 가지가 있습니다.
데이터베이스 또는 배열에서
가져올 수 있습니다
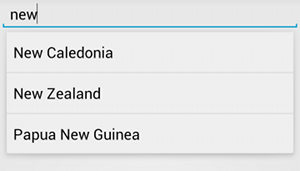
AutoCompleteTextView
의 예
확인할 수 있습니다다음 절차는 다음과 같은 AutoCompleteTextView
를 설정하는 방법을 설명합니다.
는
ArrayAdapter
:
- 레이아웃에
AutoCompleteTextView
를 추가합니다. 다음은 텍스트만 포함된 레이아웃입니다. 필드:<?xml version="1.0" encoding="utf-8"?> <AutoCompleteTextView xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/autocomplete_country" android:layout_width="fill_parent" android:layout_height="wrap_content" />
- 모든 텍스트 제안을 포함하는 배열을 정의합니다. 예를 들면 다음과 같습니다.
국가 이름 배열:
<?xml version="1.0" encoding="utf-8"?> <resources> <string-array name="countries_array"> <item>Afghanistan</item> <item>Albania</item> <item>Algeria</item> <item>American Samoa</item> <item>Andorra</item> <item>Angola</item> <item>Anguilla</item> <item>Antarctica</item> ... </string-array> </resources>
Activity
또는Fragment
인 경우 다음 코드를 사용하여 제안을 제공하는 어댑터를 지정합니다.Kotlin
// Get a reference to the AutoCompleteTextView in the layout. val textView = findViewById(R.id.autocomplete_country) as AutoCompleteTextView // Get the string array. val countries: Array<out String> = resources.getStringArray(R.array.countries_array) // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries).also { adapter -> textView.setAdapter(adapter) }
자바
// Get a reference to the AutoCompleteTextView in the layout. AutoCompleteTextView textView = (AutoCompleteTextView) findViewById(R.id.autocomplete_country); // Get the string array. String[] countries = getResources().getStringArray(R.array.countries_array); // Create the adapter and set it to the AutoCompleteTextView. ArrayAdapter<String> adapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, countries); textView.setAdapter(adapter);
이전 예에서는 새
ArrayAdapter
이 초기화되어countries_array
문자열 배열을TextView
가simple_list_item_1
레이아웃 이 레이아웃은 Android에서 제공하는 레이아웃으로 표준 모양을 사용합니다.-
다음을 호출하여
AutoCompleteTextView
에 어댑터를 할당합니다.setAdapter()
입니다.